The JavaScript trim() method removes white space characters from the beginning and end of a string. The trimLeft() and trimStart() methods trim white space characters from the beginning of a string. trimRight() and trimEnd() trim white spaces from the end of a string.
You may want to remove white spaces, such as spaces, tabs, or newlines, from the start or the end of a string. For example, suppose you have a string of customer names. You may want to make sure there are no extra spaces at the end of each name.
That’s where the JavaScript trim() function comes in. The trim() function removes white space characters from the start and end of a string.
There are a few functions related to trim() that you may want to use:
- trimLeft()
- trimStart()
- trimRight()
- trimEnd()
In this tutorial, we will explore the JavaScript trim() function and how it works. We will also look at the four related functions that you may want to use instead of or in addition to trim().
JavaScript trim() String
The JavaScript trim() method removes any white spaces from the start and end of a string. The trim() method creates a new string. trim() does not accept any arguments. This method is added to the end of a string.
Here is the syntax for the trim() method:
var ourString = "Test ": ourString.trim();
This code removes all the white spaces from the start and end of our string. There are only white spaces at the end of our string so these are the only characters removed. trim() returns a new string. This is because JavaScript strings are immutable so they cannot be changed.
It’s common to encounter a string that starts or ends with an unnecessary white space character. White space characters include spaces, tabs, and newline characters.
Trimming strings can come in handy in a range of situations.
For example, say you have a preformatted string of text that includes spaces. You may need to remove those spaces for your code to function as intended. To learn more about formatting strings, check out our guide to JavaScript string interpolation.
trim() JavaScript Example
Here’s an example of the JavaScript trim() function in action:
var studentName = "Benjamin "; console.log(studentName.trim());
The value assigned to our JavaScript variable “studentName” includes a number of spaces at the end. By using the trim function, we are able to remove those spaces.
The trim() method returns a new string to the JavaScript console:
“Benjamin”
The trim() method removes white space characters at the end of our string. trim() does not change our original string. If we wanted to store our string in its trimmed form, we would need to create a new variable to store our string:
var studentName = "Benjamin "; var trimmedStudentName = studentName.trim(); console.log(trimmedStudentName);
Our code returns: Benjamin. Now our trimmed student name is stored within the variable trimmedStudentName. We can refer to the string throughout our program by using this variable.
JavaScript trim() Additional Methods
It’s important to note that the trim() function removes spaces from both the start and the end of a string.
If you only want to remove spaces from the start or the end of a string, you should not use the trim() function. There are custom functions you can use for this purpose.
Say you want to remove white space characters from the start of a string. You can do this using trimStart() or trimLeft(). If you want to remove white space characters from the end of a string, you can use trimEnd() or trimRight().
JavaScript trimStart() and trimLeft()
Let’s suppose we want to remove white space characters from the beginning of a string. We have a name and we want to remove some white space characters from that name. Here’s an example of trimStart() and trimLeft() in action to help us accomplish this task:
var studentName = " Benjamin "; console.log(studentName.trimStart()); console.log(studentName.trimLeft());
Our code returns:
" Benjamin" " Benjamin"
Notice that our code only removed the spaces from the start of our string because we used the trimStart() and trimLeft() methods.
JavaScript trimEnd() and trimRight()
Similarly, say we wanted to remove the white space from the end of our string. We could use the trimEnd() or trimRight() methods to do this:
var studentName = " Benjamin "; console.log(studentName.trimEnd()); console.log(studentName.trimRight());
Our code returns:
"Benjamin " "Benjamin "
We have successfully removed the white spaces from the end of our string. But, the white space at the start of the string remains.
Conclusion
The JavaScript trim() function removes white spaces from the start and end of a string. trim() is added to the end of a string. The method does not accept any arguments.
You can remove white spaces from specific ends of a string using the trimStart(), trimLeft(), trimEnd(), and trimRight() methods.
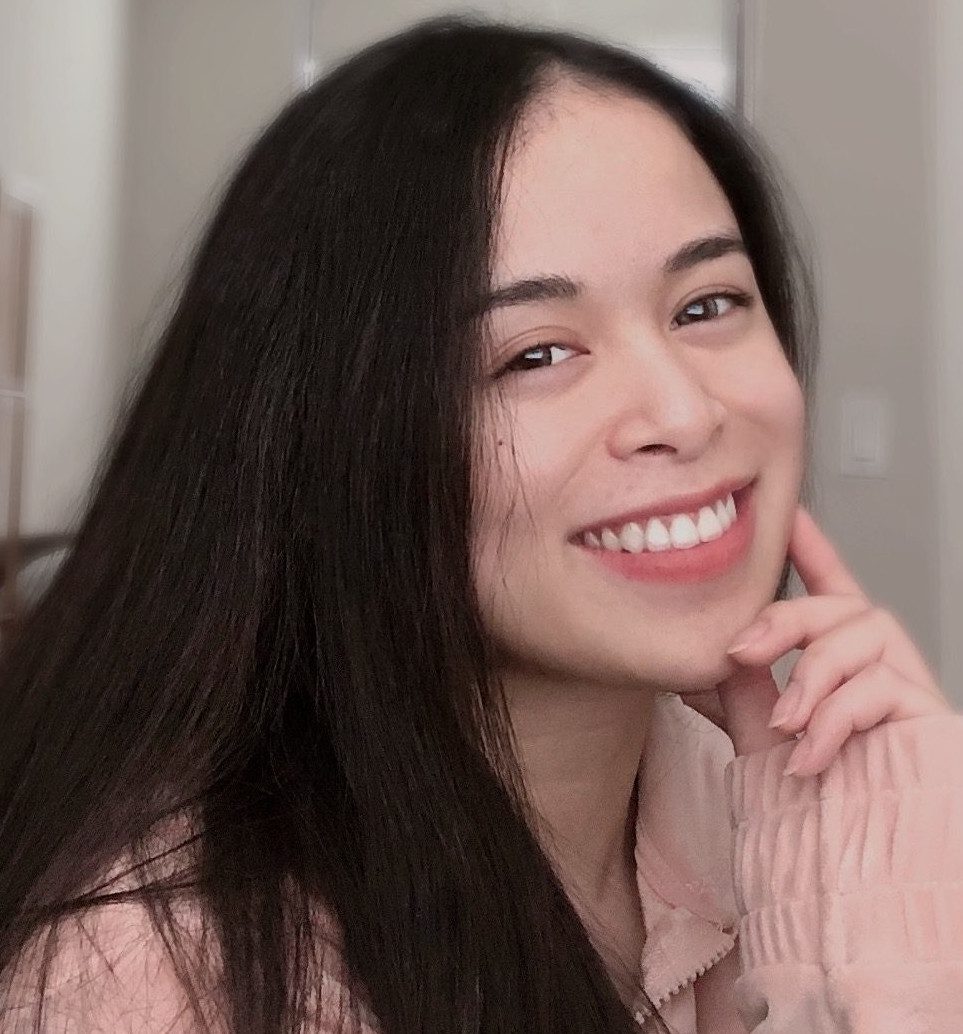
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Are you interested in learning more about JavaScript? Read our How to Learn JavaScript guide. You’ll find expert tips for learning JavaScript as well as guidance on top courses and online learning resources.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.