The JavaScript toLowerCase()
method returns a string without any uppercase letters. Similarly, the toUpperCase()
method returns a string without any lowercase characters. Both accept a string and return that string in a different case.
When you’re working with a string in JavaScript, you may encounter a situation where you want to change the case of the string. For instance, if you’re creating a sign-up form that collects a user’s email address, you may want the email address to appear in all-lowercase.
That’s where the toUpperCase()
and toLowerCase()
JavaScript functions come in. These functions allow you to convert a string to all-uppercase and all-lowercase, respectively.
In this tutorial, we’ll explore how to use the toUpperCase()
and toLowerCase()
string methods in JavaScript. We’ll also walk through an example of each of these methods being used in a real program.
After reading this article, you’ll be an expert in the use of these string methods! Let’s get started.
JavaScript Strings and Case Sensitivity
Strings are a data type, in Java, used to store text. Strings can include letters, symbols, numbers, and white spaces. The string data type is important because it allows developers to communicate text-based data through a computer program.
In JavaScript, strings can be declared using single quotes (‘’
), double quotes (“”
), or backticks (``
). Single quotes and double quotes can be used interchangeably, although you should keep your usage of either consistent throughout a program.
Here’s an example of a string in JavaScript:
var email_address = "linda.carlton@google.com";
Strings are case sensitive. This means that when you are comparing strings, searching through strings, or otherwise manipulating strings, the case of each individual character will have an impact on how you work with the string. For instance, if you compare two strings in different cases, the strings will not be considered the same by JavaScript.
The toUpperCase()
and toLowerCase()
methods are useful because they allow you to convert a string to a specific case. This means that you can perform equal comparisons and otherwise manipulate the contents of a string easily by converting the string into a specific case.
JavaScript toUpperCase
The toUpperCase()
method converts a string to uppercase letters. toUpperCase()
does not change the value of a string, instead, it returns a copy of the string in all uppercase.
Here’s the syntax for the toUpperCase()
method:
string_name.toUpperCase();
The toUpperCase()
does not accept any arguments and returns a copy of the string in all uppercase. Let’s walk through an example to illustrate how this works.
Suppose we are operating a restaurant and we want to write a program that helps the reservation attendant check whether someone is on the VIP list. We have a list of VIPs stored in uppercase.
Esther McKay has just arrived and our attendant wants to check if she is on the VIP list. The following program would allow our attendant to do so:
var vips = ['ESTHER MCKAY', 'HARRY JOHNSON', 'DAVID ANDREWS', 'SALLY BRANT']; var customer = 'Esther McKay'; if (vips.includes(customer.toUpperCase())) { console.log(customer + ' is on the VIP list.'); } else { console.log(customer + ' is not on the VIP list.'); }
When we run our code, the following response is returned:
Ester McKay is on the VIP list.
Let’s break down our code. On the first line, we declare a list called vips
which stores all the VIP names. Then, we declare a variable called customer
which stores the name of our customer. In this case, customer
is assigned the value Esther McKay
.
Then, we create an if
statement that checks whether the value of customer
is in the vips
list. To do so, we use toUpperCase()
to retrieve our customer’s name in all uppercase, then we use the includes()
method to check if vips
includes the customer’s name.
In this case, ESTHER MCKAY
is in our VIPs list, so our program prints the message Esther McKay is on the VIP list.
to the console. If the name was not on the VIP list, the message Esther McKay is not on the VIP list.
would have been returned.
JavaScript toLowerCase
The JavaScript toLowerCase()
method converts a string to lowercase letters. Like the toUpperCase()
method, toLowerCase()
does not alter the original string. Instead, it returns a copy of the string where the letters are converted to lowercase characters.
Here’s the syntax for the toLowerCase()
method:
string_name.toLowerCase();
The toLowerCase()
method functions in exactly the same way as toUpperCase()
, but instead of converting a string to uppercase, it converts the string to lowercase.
Let’s walk through an example to illustrate this method in action. Suppose you are creating a login form for a website and you store all email addresses in lowercase. So, before you save a user’s email address, you want to convert it to lowercase.
This is common practice because when a user signs in, you’ll want to search for the email address they inserted in your database. If a user initially submitted an email address with case-sensitive characters, it may affect whether you can find that email in your database.
You could convert the email address a user has submitted to lowercase using this code:
var email = "Emily.Saunders@google.com"; var lowercase_email = email.toLowerCase(); console.log(lowercase_email);
Our code returns: “emily.saunders@gmail.com”. As you can see, the email address has been converted to lower case.
Conclusion
In this guide, we discussed how to use the toUpperCase()
and toLowerCase()
to convert a string to all-uppercase and all-lowercase, respectively. We also walked through an example of each of these methods in action.
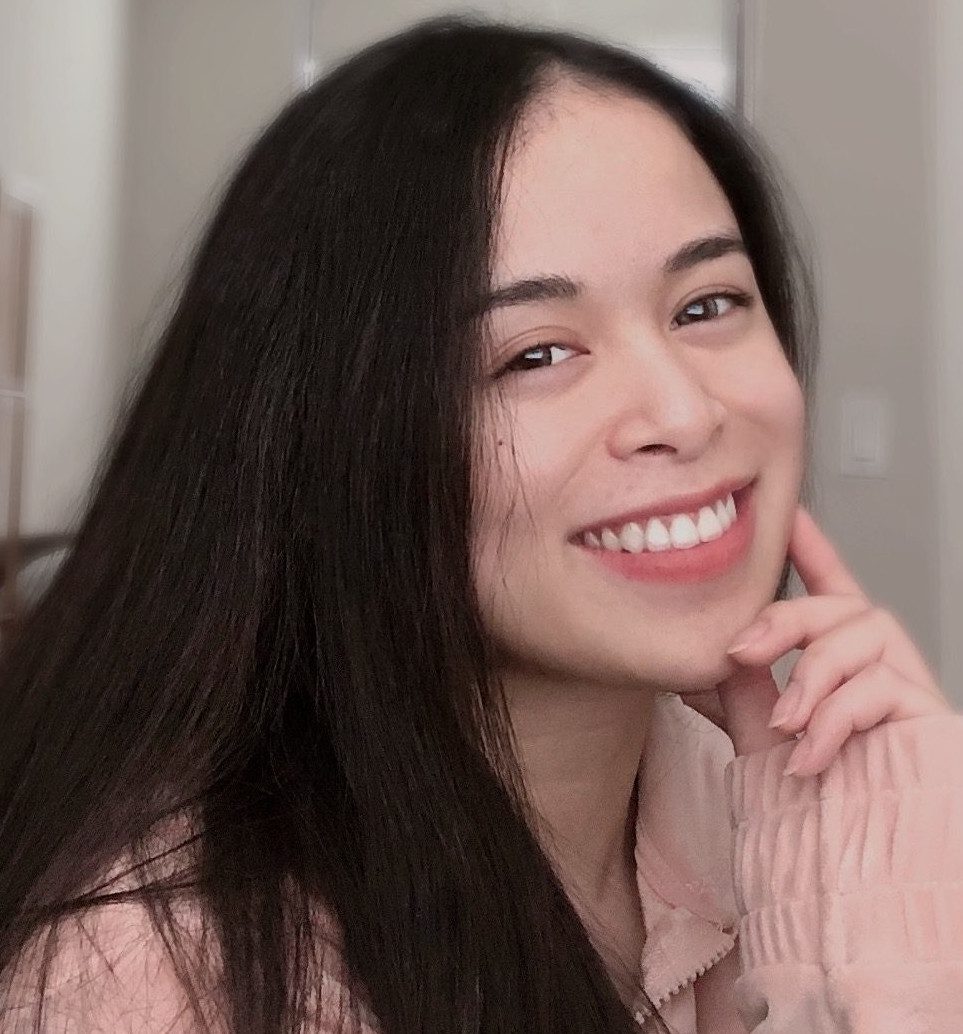
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
You’re now ready to start using toUpperCase()
and toLowerCase()
to convert the cases of JavaScript strings like an expert.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.