JavaScript string interpolation is the process of embedding an expression into part of a string. A template literal is used to embed expressions. You can add values such as variables and mathematical calculations into a string using interpolation.
Do you need to add a value inside a JavaScript string? The template literal syntax has you covered. Template literals make it easier to work with multiline strings and embed values inside a JavaScript string. Template literals are the JavaScript string interpolation syntax.
In this guide, we’re going to talk about JavaScript string interpolation. We’ll discuss what it is, how it works, and walk through an example of string interpolation to help you get started.
Without further ado, let’s begin!
JavaScript String Interpolation
You can add values into a JavaScript string using a template literal. This is a dollar sign followed by a pair of curly brackets. Within the curly brackets should be the expression whose value you want to embed in the string.
Template literals let you embed values into a string without relying on concatenation. To declare a template literal, your string must be enclosed in back ticks (“) instead of quotation marks.
Consider the following syntax:
`This is a string. Here is the value of 9 + 10: ${9 + 10}.`
We have declared a template literal. Our expression is:
${9 + 10}
This expression evaluates 9 + 10. The result of this expression is added to the end of our string, before the full stop. This is because we have written our expression before the full stop.
JavaScript String Interpolation Example
Template literals allow you to embed values directly into a string. Consider this code:
const address_number = "10 Downing Street"; const prime_minister_address = `The Prime Minister's address is ${address_number}.`; console.log(prime_minister_address)
The first line of code defines the Prime Minister’s address number. Next, we use template literals to create the full address. The ${} syntax is used to embed a value into our string. We have added the value of “address_number” into the string.
Let’s run our code:
The Prime Minister's address is 10 Downing Street
Our code has merged our two strings.
When you are using template literals, you can embed any value inside the literal. This includes a string, a number, or a the result of a JavaScript math calculation. You don’t need to embed a template literal inside a template literal.
One of the major advantages of string literals is that its syntax is clear. We can embed our JavaScript code directly into our string.
Merge Strings Using Concatenation
We can merge strings using concatenation. Let’s say that we have two strings:
const full_address = `The Prime Minister's address is `; const address_number = `10 Downing Street`;
You can do concatenate two strings using the plus sign (+):
const prime_minister_address = full_address + address_number + "."; console.log(prime_minister_address);
The JavaScript console prints out the following value:
The Prime Minister's address is 10 Downing Street
We have successfully created a string that combines our two strings. At the end, we use the concatenation operator to add a full stop (“.”) to our sentence.
But, this syntax has a drawback: we can only add values to the end of a string. This is a big reason behind the introduction of template literal syntax. It is now easier to add values in the middle of a string.
String Interpolation JavaScript: Calculations
The code inside a template literal is a JavaScript statement. This means that you can use template literals to perform calculations and embed their values into a program.
Let’s create a string that calculates the price of two drinks at a café and places the result inside a string:
var drink1 = 2.30; var drink2 = 2.20; var bill = `The total cost of your drinks is $${drink1 + drink2}.`;
Inside the ${} syntax, we have added a calculation. This calculation adds the values of two JavaScript variables together. Our variables are drink1 (2.30) and drink2 (2.20) together. Our code returns a string with the sum of these values:
The total cost of your drinks is $4.50.
String Interpolation JavaScript: Ternary Operators
JavaScript ternary operators let you evaluate whether a statement is True or False. They are a more concise way of writing an if statement. Ternary operators are particularly useful if the result of an if statement is only going to take up one line of code.
If a customer spends over $4.00 on their coffees, they should be presented with their bill. Then, they should be asked to join the coffee loyalty card club. Otherwise, they should just be presented with their bill. We could use a ternary operator to check the cost of a customer’s bill:
var drink1 = 2.30; var drink2 = 2.20; var total = drink1 + drink2; var bill = `The total cost of your drinks is $${total}. ${total > 4.00 ? "Would you like to join our coffee club? It will earn you a free coffee for every ten that you buy." : ""}`; console.log(bill);
Our template literal contains two embedded expressions. First, we embed the value of “total” inside our string. Next, we use a ternary operator to check whether a customer should be asked to join the coffee house’s loyalty card club.
If the total purchase price is over $4.00, the following message is printed to the console:
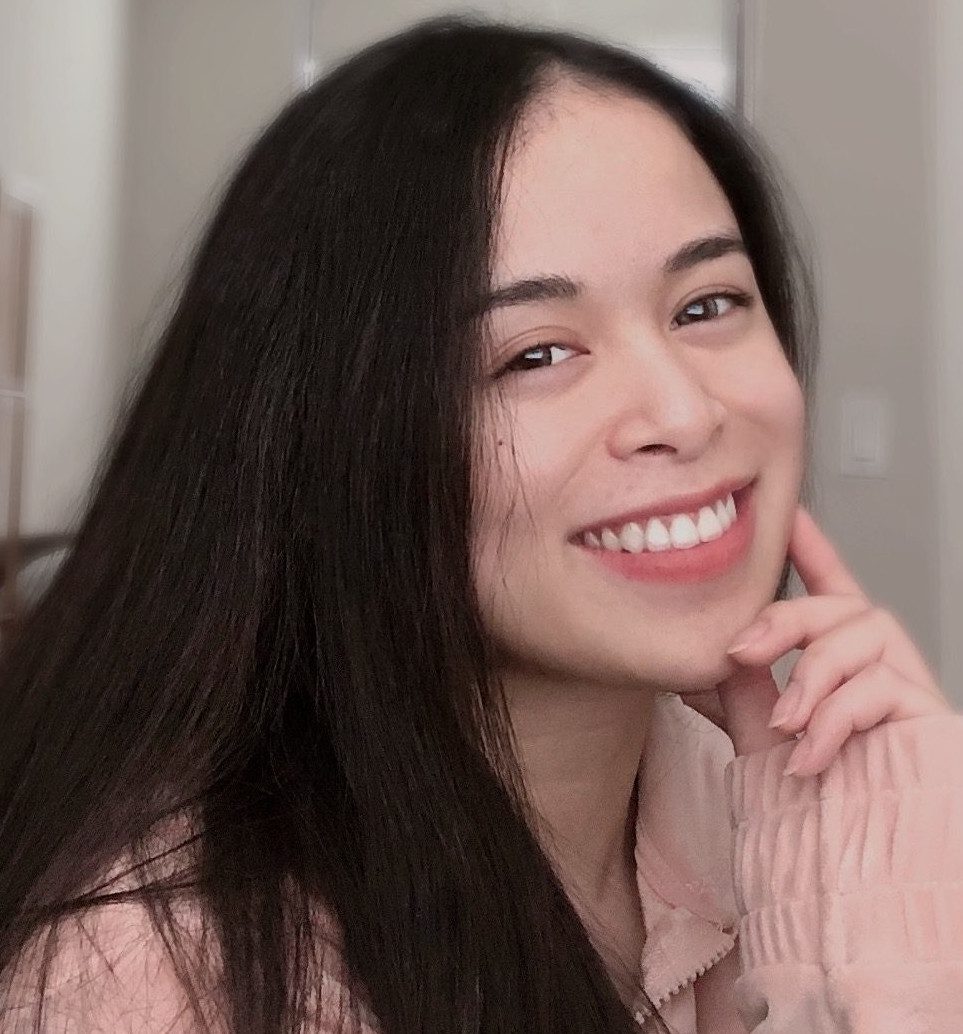
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The total cost of your drinks is $4.50. Would you like to join our coffee club? It will earn you a free coffee for every ten that you buy.
Otherwise, our ternary operator will return the value after the colon (“:”). This is the value that is returned when an expression evaluates to False.
In our example, the value after the colon is an empty string. This means that if a customer’s purchase is under $4.00, only the first sentence of our template literal is returned:
The total cost of your drinks is $4.50.
Conclusion
Template literals allow you to embed a value inside a string. You can perform calculations inside a template literal. We declare template literals using backticks. Statements to evaluate are denoted by a dollar sign and a pair of curly brackets within which the statement appears.
For advice on learning more about JavaScript, read our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.