The JavaScript startsWith
method will return true or false if a string starts with a specified character or string. JavaScript endsWith
works the same way but applies to the end of a string. Both accept parameters that effect where the methods search.
In JavaScript, you may want to determine whether a string starts with or ends with a particular character or set of characters. For instance, if you’re operating a cafe and want to find out whether key lime pie is the most popular flavor, you would want to check if your list of pie flavors ordered by popularity starts with key lime pie.
The JavaScript startsWith()
method is used to determine whether a string starts with a particular substring. The endsWith()
method is used for the same purpose but checks whether a string ends with a specified substring.
This tutorial will discuss how to use the startsWith()
and endsWith()
methods, and walk through an example of each of these functions step-by-step. By the end of this tutorial, you’ll be an expert at using the startsWith()
and endsWith()
methods.
JavaScript startsWith
The startsWith()
method determines whether a string begins with the characters of a specified substring, and returns true or false. The syntax for the startsWith()
method is as follows:
string_name.startsWith(substring, position);
The startsWith()
method accepts two parameters:
- substring is the characters to be searched for at the start of the string object. (required)
- position is the position at which the search should begin. (optional)
startsWith()
returns a Boolean value—true or false—depending on whether the string starts with the substring you have specified.
Let’s go through an example to explain how the startsWith
method works.
Suppose you are operating a cafe and you want to determine whether key lime was the most popular pie sold in your store last month. You remember that a lot of key lime pies were ordered, and if it turns out to be the most popular flavor, you may want to run a new promotion on the pies to attract customers.
You could use the startsWith()
method to check whether key lime was the most popular pie ordered at your store. Here’s an example of a program that accomplishes this task:
var pie_rankings = "Key Lime Apple Blueberry Cherry Strawberry Pecan Peach"; console.log(pie_rankings.startsWith("Key Lime"));
When we run our code, the following response is returned:
true
Let’s break down our code. On the first line, we declare a string called pie_rankings
which stores all the pies we sold last month. The first pie in our list is the most popular, the second pie is the second-most popular, and so on. Then, we use the startsWith()
method to check if the string begins with Key Lime
, and we use console.log()
to print out the response to the console.
In this case, our pie_rankings
string starts with Key Lime
, and so our program returned true.
Now, suppose that we want to figure out whether apple pie was the second-most popular pie ordered last month. We recall that a lot of apple pies were ordered, and if apple was the second-most popular flavor, that next month we could raise the prices slightly.
We could use the startsWith()
method and its optional position
parameter to determine whether apple pie was the second most popular pie ordered at our cafe.
Now, in order for our program to work, we need to know the index value at which Key Lime
ends (notice there is a space after Key Lime
, because it is one entry of many in our list of pies). In this case, Key Lime
lasts 9 characters—seven are letters and two are spaces.
So, we know that our search should start at index position 8 in our list. Here’s an example of a program that allows us to check whether our list starts with Apple
at the index position 9 (which is where the second pie starts in our list):
var pie_rankings = "Key Lime Apple Blueberry Cherry Strawberry Pecan Peach"; console.log(pie_rankings.startsWith("Apple", 9));
Our code returns: true. Apple
starts at the index position 9 in our list, so our code returned true.
JavaScript endsWith
endsWith()
is the opposite of the startsWith()
method. The endsWith()
method determines if a string ends with a particular set of characters. Here’s the syntax for the endsWith()
method:
string_name.endsWith(substring, length);
The endsWith()
method accepts two parameters:
- substring is the string to search for in the string. (required)
- length is the length of the string to search. The default value for this parameter is the length of the string. (optional)
Let’s explore an example of the endsWith()
method.
Suppose that we are looking to reduce the size of our menu by one pie because the baker who makes the pies is overwhelmed by the number of different pies they have to make. We think that peach pies were the least popular last month, and we want to check that against our list of pies ordered by popularity.
We could use the following code to check if our list of pies ends with Peach
:
var pie_rankings = "Key Lime Apple Blueberry Cherry Strawberry Pecan Peach"; console.log(pie_rankings.endsWith("Peach"));
Our code returns: true. As you can see, our pie_rankings
string ends with Peach
. So, the endsWith()
method returns true
.
In addition, we can specify the length
argument if we want our string to stop searching at a certain index value. For instance, say we wanted to check whether Strawberry
was the second-least popular pie sold last month.
We know that the length of our string is 54, and if we subtract the length of Peach
from the string, we can get the length of our string minus the last pie. Peach
is 5 characters long, and if we subtract 5 from 54 we get 49.
In addition, there is a space before Peach
in our string, so we’ll have to subtract another 1 from our string’s length, which means the position at which the second last pie ends in the string is 48.
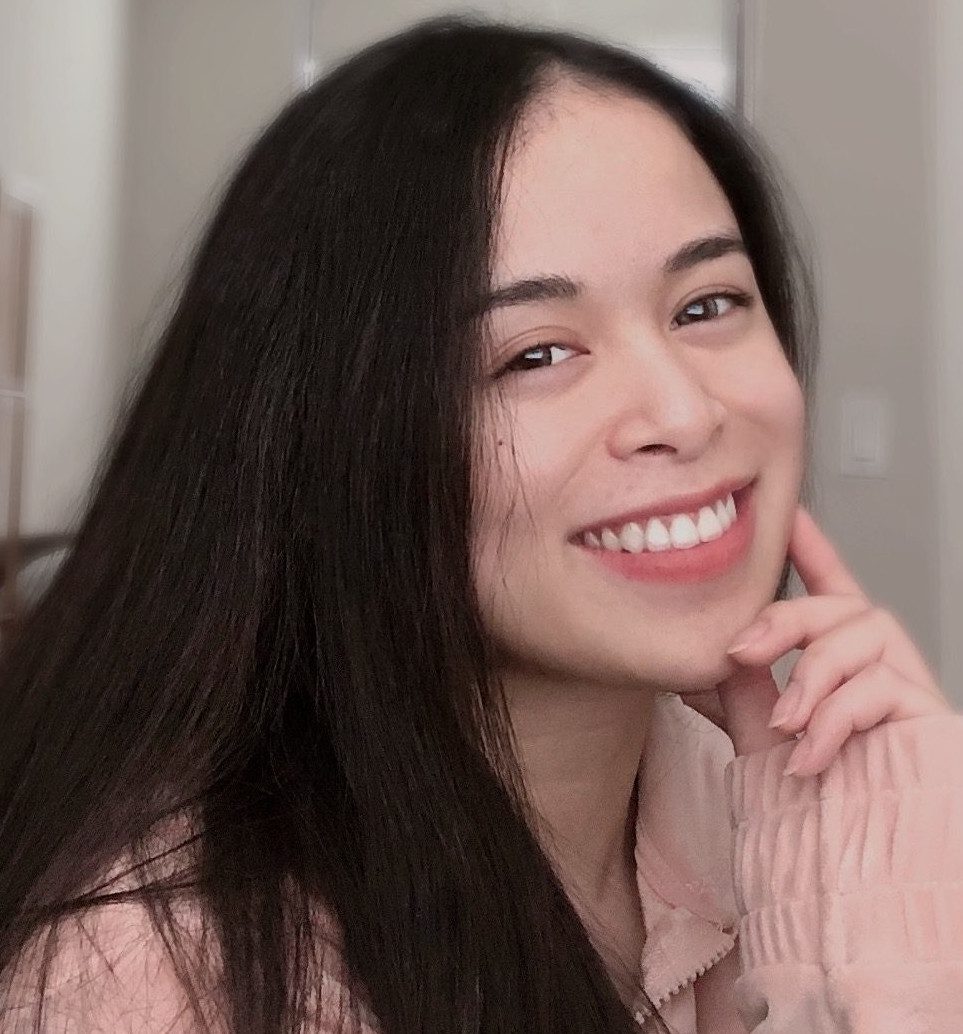
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s the code we could use to check whether Strawberry
is the second-least popular pie in the pie_rankings
string:
var pie_rankings = "Key Lime Apple Blueberry Cherry Strawberry Pecan Peach"; console.log(pie_rankings.endsWith("Strawberry", 48));
Our code returns: false. As you can see, Pecan
comes at the end of our list before the value Peach
and Strawberry
appears before Pecan
in the list. As a result — Strawberry
is not the second-last pie on the list — our code returns false
.
Conclusion
The startsWith()
method is used to determine whether a string starts with a particular sequence of characters. The endsWith()
method is used to check whether a string starts with a sequence of characters.
This tutorial explored how to use the startsWith()
and endsWith()
methods in JavaScript, and walked through an example of each of the methods in action. You’re now equipped with the knowledge you need to use the startsWith()
and endsWith()
methods like an expert.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.