How to Use Sets in JavaScript
Do you have a list of data that should only contain unique values? This is where the JavaScript Set data type really shines. It’s a new type of object that allows you to create a collection of unique values; no duplicates are allowed within a set.
In this guide, we’re going to talk about what sets are, how they work, and when they can be useful. We’ll walk through a few examples of each of the Set methods available in JavaScript.
What Is a Set?
A Set is a set of values that cannot contain duplicates. This data type was introduced in ECMAScript 6. Notably, Sets are a feature of many other programming languages.
Lists are useful because they allow you to store multiple values. Lists support storing duplicate values, which can be useful in a myriad of scenarios.
For instance, if you’re storing a list of orders made at a coffee shop, you’ll need to be able to store duplicate values. Two customers may order the same drink.
There are some instances where you’ll want to store only unique values in a list. That’s where you’d want to use the Set object. Values in Sets can only appear once.
In JavaScript, a set is declared like any other object. Let’s create a Set which stores a list of the favorite cakes reported by customers at a local tea room:
let cakes = new Set(); console.log(cakes)
Our code returns: Set []
. We’ve just created a Set. It has no values at the moment, as you can see from the output of our code; we’ll add those in next.
Initialize a Set with Default Values
Sets can be initialized from an array, string, or another iterable. This is useful because it means that you don’t have to add values manually to your Set; you can work from existing ones.
Consider the following list:
let cake_list = ["Lemon Cake", "Carrot Cake", "Strawberry Cheesecake"];
These values are currently stored in an array. To convert them into a Set, we can pass our array when we create a new Set object:
let cakes = new Set(cake_list); console.log(cakes)
Our code returns:
Set(3) ["Lemon Cake", "Carrot Cake", "Strawberry Cheesecake"]
Adding Values to a Set
The Set object comes with a method called add()
which makes it easy to add items into a set. The name of the method used to add an item to a set is even easy to remember: add()
.
Let’s say that we want to add “Boston Cream Pie” to our list of cakes that are being sold at a local bakery. Let’s use the add()
method to add this value:
cakes.add("Boston Cream Pie"); console.log(cakes);
Our code returns:
Set(4) ["Lemon Cake", "Carrot Cake", "Strawberry Cheesecake", "Boston Cream Pie"]
Our Set now contains four objects. If you try to add an item that is already in a Set, that item will not be added. Sets cannot store duplicate values.
Checking if a Value Exists in a Set
We need to check whether or not “Boston Cream Pie” is on our list of cakes. It was a new addition to the list of cakes and the baker wants to double-check we have added it.
That’s where the has()
method comes in. The has()
method allows you to check to see whether a Set has a particular value. Again, the method has a name that’s easy to remember!
Consider the following code:
var has_boston_cream_pie = cakes.has("Boston Cream Pie"); console.log(has_boston_cream_pie);
Our code returns: true
. The has()
method returns a boolean value—either true or false—depending on whether a Set contains a particular value. This method is similar to includes()
that you’d use on an array but has()
only works with Sets.
Removing a Value from a Set
The baker has decided that strawberry cheesecakes will no longer be served at the bakery. The strawberry cheesecake took longer to make than other cakes and was not very popular.
You can remove a value from a Set using the aptly-named delete()
method. Let’s remove the value “Strawberry Cheesecake” from our Set:
cakes.delete("Strawberry Cheesecake"); console.log(cakes);
Our code returns:
Set(3) ["Lemon Cake", "Carrot Cake", "Boston Cream Pie"]
Strawberry cheesecake was removed from our Set.
You can remove all the values from a Set using the clear()
method.
Iterate Over a Set
Sets, like lists, are iterable objects. You know what that means: you can iterate over them.
We’re going to use a for-each loop to iterate over our cakes set. Our for-each loop will go through every item in our list—for each item in the list—and print it to the console:
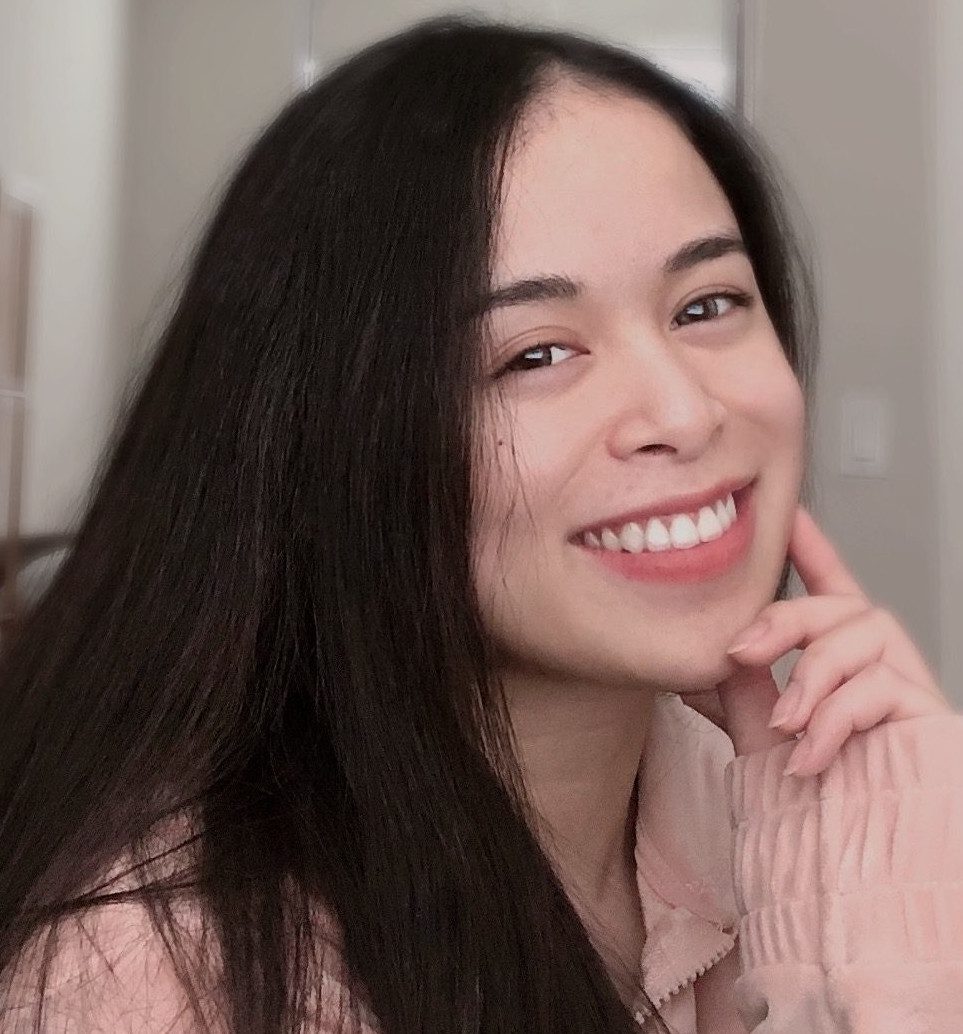
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
cakes.forEach(cake => { console.log(cake); }
Our code returns an iterator which prints out each value in our Set to the console:
Lemon Cake Carrot Cake Boston Cream Pie
It’s that simple! You could also use a for-of loop:
for (let cake of cakes) { console.log(cake); }
This code returns the same output as our forEach
loop:
Lemon Cake Carrot Cake Boston Cream Pie
You could opt to use a for loop to iterate through a Set but because Sets are iterable objects, it’s easier to just use a for-each or for-of loop.
Removing Duplicate Values Using Set
When you initialize a Set with an array, all duplicate values will be automatically removed. This is an incredibly useful feature.
Let’s say that we have a list which contains a number of cake orders:
var cakes = ["Lemon Cake", "Carrot Cake", "Strawberry Cheesecake", "Lemon Cake", "Chocolate Cake", "Chocolate Fudge Cake", "Chocolate Cake", "Red Velvet Cake"];
This Set contains all the cake orders made to the bakery. In total, there are eight values; two values are duplicates.
Suppose that the baker wants to know what different types of cakes have been ordered, so she can start to prepare her kitchen. We could find this out by converting our Set into a list:
var unique_cakes = new Set(cakes); console.log(unique_cakes);
Our code returns:
Set(6) [ "Lemon Cake", "Carrot Cake", "Strawberry Cheesecake", "Chocolate Cake", "Chocolate Fudge Cake", "Red Velvet Cake" ]
Our list has been pared down to six values. These are all of the unique values from our list of orders. There’s one step left.
We’ve got to convert our Set of cakes back to a list so that we can interact with it using standard list methods. We can do so using the Array.from()
method:
let final_cakes = Array.from(unique_cakes); console.log(final_cakes);
Our code returns:
[ "Lemon Cake", "Carrot Cake", "Strawberry Cheesecake", "Chocolate Cake", "Chocolate Fudge Cake", "Red Velvet Cake" ]
While the output of this code is similar to our Set, there is one big difference: our data is now stored as an array. This means that we can interact with it using all the built-in JavaScript array methods.
Conclusion
The Set object allows you to store a list of items that can only contain unique values. Sets can be initialized from an existing iterable object, such as a list or a string.
Because the Set object removes duplicate values, you can use it as a way to remove any duplicates from an array. Then, once you’re done, you can convert your Set back to an array.
Now you’re ready to start using Sets in JavaScript like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.