So, you want to select an element on a web page in JavaScript? That’s the specialty of the getElementById
method, the so-called king of the element getters
in JavaScript.
getElementById
allows you to select an element based on its ID. It’s quite a self-explanatory method name when you think about it.
In this guide, we’re going to talk about what getElementById
is, how it works, and when you should use it in your code. We’ll walk through an example of a “Show/hide” text button in our document.getElementById demo.
Let’s dive in!
What is getElementById?
getElementById()
is a Document method that selects an element from the HTML DOM on a page based on the element ID attribute that you have specified.
Consider the following code:
index.html <button id="mainButton">This is a button!</button> scripts.js const button = document.getElementById("mainButton");
In our HTML code, we’ve defined a <button>
element which has the ID mainButton
. Our JavaScript code will retrieve this component using the getElementById()
method.
This method is useful if you want to retrieve a single element on a page. If you think back to learning HTML and CSS, you’ll know that IDs have to be unique. This makes getElementById()
perfect for retrieving single elements.
You may opt to use this method if you’ve assigned an ID to an element. Instead of using a broader getter
like querySelector
or getElementByClass
, which returns the first element object that meets a particular criteria, you can retrieve an element using getElementById()
.
getElementById()
is a common method used to retrieve an item on a web page.
What You Need to Know About getElementById
Before we delve into an example, we’re going to talk about the mistakes that beginners often make when using this method.
It’s always frustrating when you are learning something new and it doesn’t work, so let’s chat about what common errors you may make while you’re getting the hang of getElementById()
.
First, getElementById()
is case sensitive. While the term ID
is usually capitalized in programming, you need to use the camel case Id
when you’re using this method. Otherwise, this method will not work.
Second, the ID that you specify should not include a pound sign (hashtag). Consider this code:
const button = document.getElementById("#mainButton");
This example may look like it will retrieve the element with the ID mainButton
, but that’s not the case. You should only specify the ID of the element you want to retrieve:
const button = document.getElementById("mainButton");
Now that’s out of the way, we can go through an example.
How to Use getElementById
When you’re browsing the web, you may encounter tags that say “Read more”. A web page may show a few sentences of a piece of text, and then ask you to press that button so that you can see the rest of the text.
We’re going to build that feature using the getElementById()
getter. You can view the final code for this tutorial on GitHub.
Creating the Front-End
Let’s get started by creating a simple front-end using HTML and CSS. Create a new file called index.html and paste in the following code:
<!DOCTYPE html> <html> <head> <title>An Introduction to getElementById()</title> <link rel="stylesheet" href="styles.css" /> </head> <body> <div> <h1>JavaScript getElementById()</h1> <p>Are you looking to learn more about getElementById()? You've come to the right place.</p> <span id="showMore">Read more</span> <p id="hiddenText">getElementById() is a JavaScript method that allows you to select an element on a web page. This method is commonly referred to as a "getter" because it "gets" an element from a page.</p> </div> </body> <script src="scripts.js"></script> </html>
In this document, we’ve defined a box which contains information about the getElementById()
method. Our box contains a title, two paragraphs of text, and a span HTML tag that we will use to reveal the last paragraph.
Open up a file called styles.css and paste in this code:
<!DOCTYPE html> <html> <head> <title>An Introduction to getElementById()</title> <link rel="stylesheet" href="styles.css" /> </head> <body> <div> <h1>JavaScript getElementById()</h1> <p>Are you looking to learn more about getElementById()? You've come to the right place.</p> <span id="showMore">Read more</span> <p id="hiddenText">getElementById() is a JavaScript method that allows you to select an element on a web page. This method is commonly referred to as a "getter" because it "gets" an element from a page.</p> </div> </body> <script src="scripts.js"></script> </html>
This code gives our page some color to make it more visually appealing. When you open up the web page, you’ll see the following:
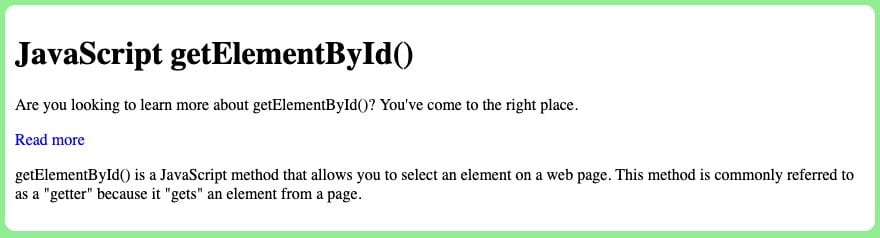
There’s one problem: our “Read more” tag does nothing, and our last paragraph of text is still visible. That’s because we have not yet added in our JavaScript code. Let’s write the JavaScript functionality for our web page.
Adding JavaScript Code
Let’s start by selecting the elements with which we are going to be working. The elements we need to select are our <span>
tag (which contains our “Read more” text), and the paragraph that we want to toggle between showing and hiding.
Create a file called scripts.js and paste in this code:
var paragraph = document.getElementById("hiddenText"); paragraph.style.display = "none";
In our code, we’ve used getElementById()
to retrieve our paragraph from the DOM document. selects the hiddenText document object and hides it. Next, we’ll create a function that toggles our text:
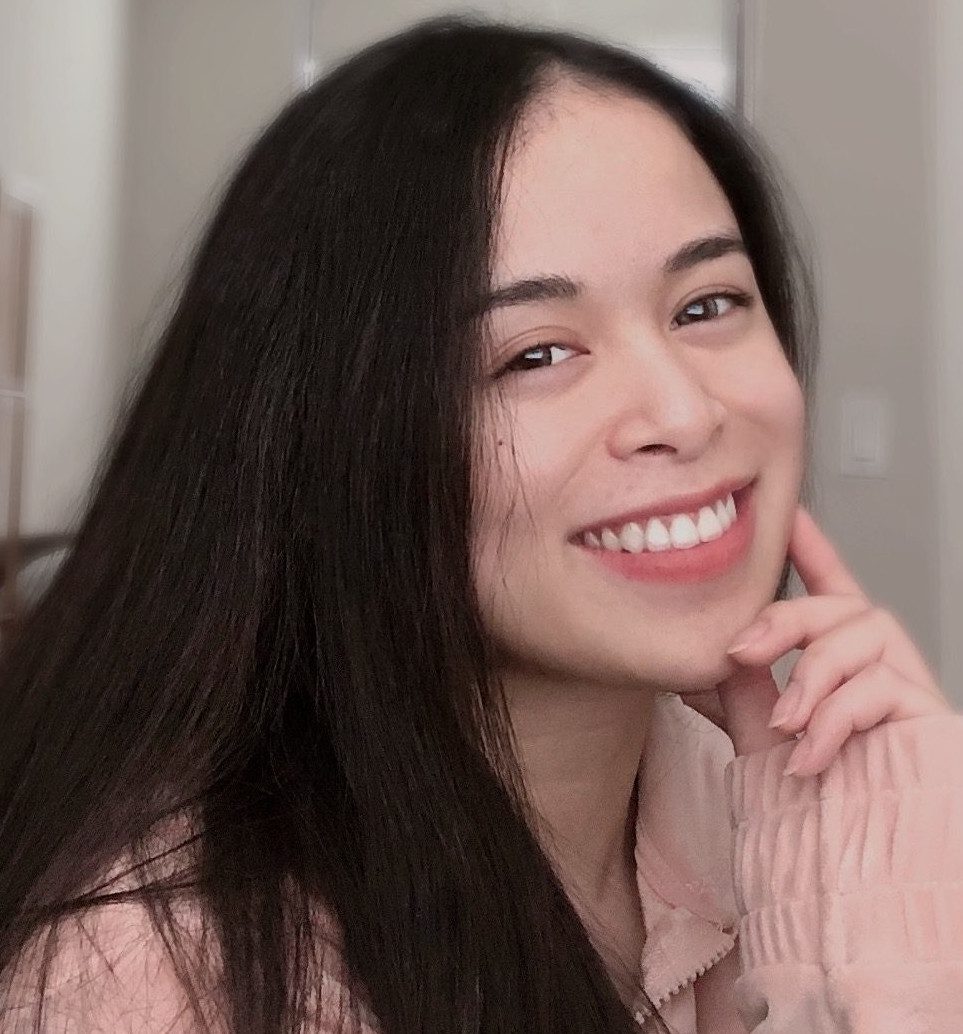
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
function toggleText() { if (paragraph.style.display === "none") { paragraph.style.display = "block"; } else { paragraph.style.display = "none"; } }
We’ve started by selecting the element with the ID hiddenText
. This refers to the paragraph of text that we want to appear or disappear when “Read more” is clicked.
We’ve written an if
statement which checks whether that paragraph is visible. If it is not visible, the display
value of the paragraph will be set to block
, which will make it appear; otherwise, the paragraph’s display
value will be set to none
, and so it will disappear.
Our code isn’t finished yet. Next, we’re going to create en event listener which will activate when we click on our “Read more” text:
var showButton = document.getElementById("showMore"); showButton.addEventListener("click", toggleText);
This code selects the Read more
text on our page. It then sets up an event listener which will trigger our toggleText()
method when the “Read more” text is clicked.
Now, let’s open up our web page and try to click on the Read more
text:
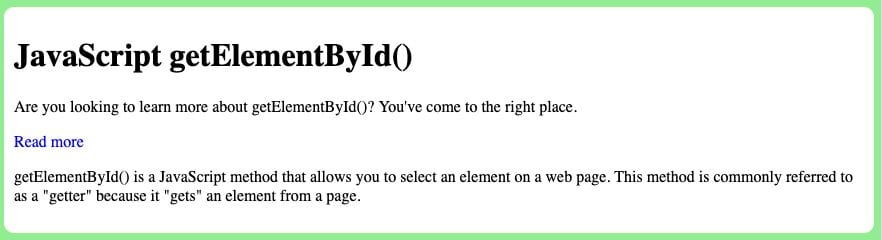
Our code is functional! When you click on the “Read more” text, the text appears. When you click on it again, the text disappears.
Conclusion
The getElementById()
getter allows you to retrieve an element based on its ID from a web page.
Are you looking for a challenge? Use the getElementById()
getter to change the value of “Read more” to “Read less” when the text is visible. To go further, you could add a few images to the page and create a button which hides all those images when the button is clicked.
Now you’re ready to start using the getElementById()
getter like a professional coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.