JavaScript comments are used to write notes in your code or to disable sections of code without deleting them. Comments are made in JavaScript by adding //
before a single line, or /*
before and */
after multiple lines.
Commenting on code is a core feature of a number of programming languages. The idea behind code comments is that while your code will be read by the computer, your code will also be read by humans, and so it is important that it is easy for people to understand the code that you write.
Comments allow you to keep track of your code as you are programming, and give you the ability to take notes that may be helpful further down the line. In addition, if you are working on a team, writing comments will help ensure that your code is readable for everyone who may have to contribute to your work.
In this tutorial, we are going to discuss how to write comments in JavaScript. We will start by discussing the value of commenting in JavaScript, then we will look at the syntax you can use to write comments in JavaScript.
Writing Comments on Code
Commenting is an essential part of writing readable and maintainable code. The truth is that while you may understand the code you are writing today, there is no guarantee that you will be able to effectively describe what it does tomorrow. In addition, if you are working with other people, there is a high chance that they may not understand some of the code you write.
That’s where comments come in. Comments are statements which are ignored by the compiler and can be used to explain your JavaScript code.
As a developer, writing comments on your own code will allow you to more effectively keep track of what you are doing. As you are writing your code, you can write down notes which will help you better understand your code and figure out the intent behind code you have previously written. Writing comments will also be helpful for any other people who may see your code, as the comment will likely help them understand how your code works in more depth.
Every developer writes comments differently, but the general rule is that comments should be used to explain the intent behind your code. After all, your code speaks for itself, but the reasons behind writing code in a certain way often do not. So, by writing comments, you can explain why you approached a problem in a certain way, making it easier for both you and others to understand how your program works further down the line.
JavaScript Comments
There are two types of comments used in JavaScript: single-line comments and block comments.
Single-line comments are written using two forward slashes (//) and are written on one line of code. Every character following the “//”
statement will be ignored until the end of the line. Here’s an example of a single-line comment in JavaScript:
// This is a single-line comment
Block comments are written with opening and closing tags and allow you to write comments that span across multiple lines. The opening and closing tags for block comments are:
“/*”
for opening a comment“*/”
for closing a comment
Block comments are sometimes referred to as multi-line comments and resemble CSS multi-line comments. Here’s an example of a block comment in JavaScript:
/* This is a multi-line block comment */
All code between our open and closing tags will be ignored by the compiler.
Each type of comment can be used to explain your code and discuss the intention behind your code. Here’s an example of a comment being used to explain a basic variable declaration:
// Declare “chocolate” variable to store chocolate types as an array
var chocolate = [‘Dark’, ‘Milk’, ‘White’, ‘Hazelnut’, ‘Caramel’];
Comments in any programming language should be updated frequently as the code changes. So, if we decided that our variable name was going to be renamed to “chocolate_types,” we should update our comment to ensure that it reflects the new variable name. Indeed, as programs get larger, they often change significantly, so it’s important to update your comments alongside your code.
Commenting Out Code
Comments are often used for one of two purposes. The first, as we have discussed throughout this article, is to explain the intent behind your code and clarify any confusing procedures. The second is to prevent code from running while you are testing.
Commenting out code allows you to stop a line or multiple lines of code from running without removing the code from your program entirely. This usage of comments is common when debugging, as you may want to try out multiple solutions to a problem and retain your code for later.
Here’s an example of a comment that comments out a line of code:
var chocolate = ['Dark', 'Milk', 'White', 'Hazelnut', 'Caramel']; // var flavors ['Strawberry Deluxe', 'Hazelnut Praline', 'Vanilla', 'Triple Truffle'];
When we execute our program, the compiler will only execute the line of code that declares the variable “chocolate.”
We have commented out the “flavors”
variable because it contains an error and we need to debug the problem. In this case, we have forgotten to place an equals sign between our variable name (“flavors”)
and the value of the variable (our array of flavors).
Let’s break down each of the two types of comments we have discussed and explore how they can be used.
Single-Line Comments
Single-line comments, also known as inline comments, are used to comment on part of a line of code or a full line of code. Here’s an example of a JavaScript single-line comment:
var first_type = chocolate[0]; // Get first value of chocolate array for later reference
In this comment, we declare a variable “first_type”
which stores the first value in our “chocolate”
array. Then, we add an inline comment at the end of our line to explain the intent behind our code.
Single-line comments are generally used to make short comments on your code, or to describe the intent behind a specific line of code. However, single-line comments are not the only type of comment you should use. If you have to explain a larger function, it’s best to write block comments.
Block Comments
Block comments are written across multiple lines and are used to explain a larger section of code. Whereas single-line comments usually explain one line of code, a block comment may explain a function or the purpose of a file.
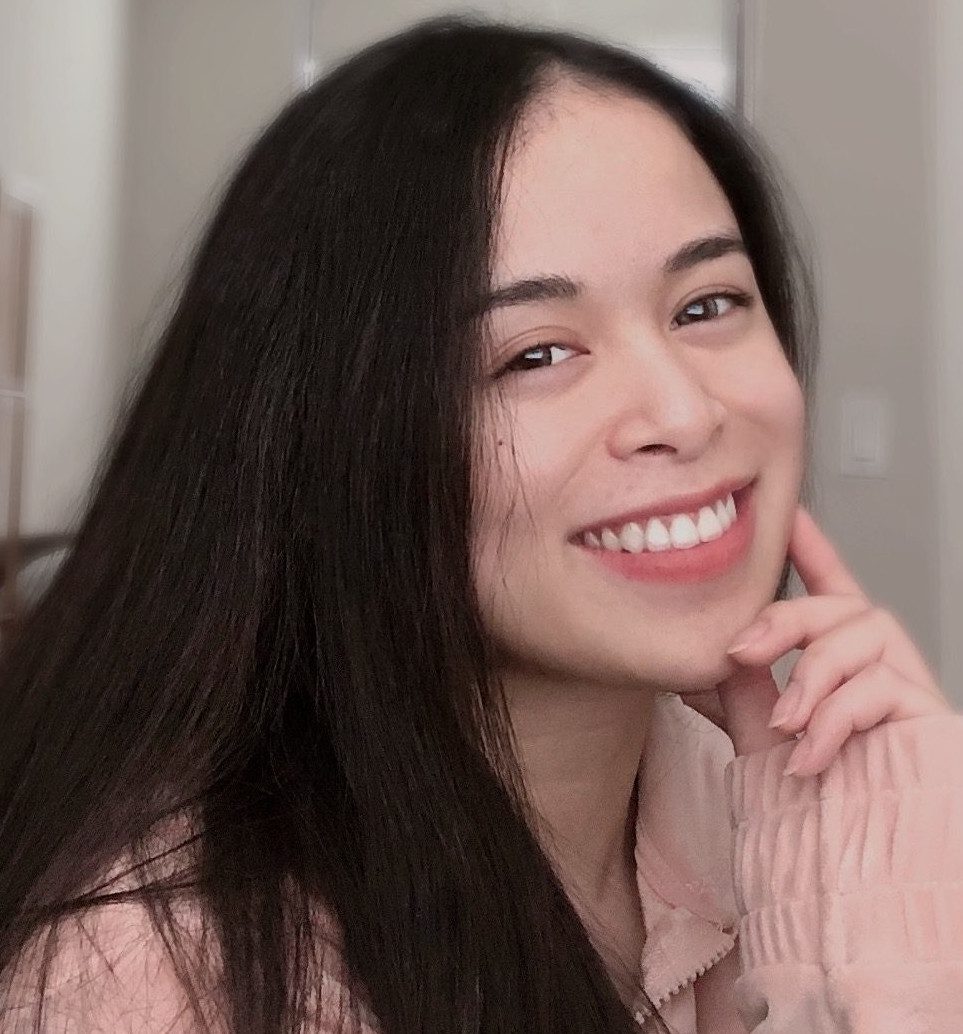
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Multi-line comments start either at the top of a function or a file, in most cases. Here’s an example of a block comment being used to explain a for loop:
var chocolate = ['Dark', 'Milk', 'White', 'Hazelnut', 'Caramel']; /* Create a for loop to iterate through chocolate array and print out each individual value in the array */ for (i = 0; i < chocolate.length; i++) { console.log(chocolate[i]); };
Our block comment spans across three lines and is being used to describe the code written in our “for” loop.
Block comments can also be found at the start of a file to explain the purpose of a file, or to include information about the author and version of the file. Here’s an example of a snippet that includes the author of a file and the date it was created in JavaScript:
/* Author: John Appleseed Date: January 1, 2020 */
Conclusion
Writing comments on your code allows you to write more readable and maintainable code. You can use comments to both keep track of your code for your future self, and to make it easy for other people working with your code to understand its purpose.
In this tutorial, we discussed the basics of commenting and then explored how to write a comment in JavaScript. We also discussed the two main types of comments—single-line and multi-line—and showed examples of each type of code comment in action. Finally, we discussed the differences between commenting on and commenting out code.
Now you’re ready to write comments in JavaScript like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.