JavaScript is based on prototypes. Every time you declare an object, a prototype property is created which extends the properties and methods associated with that object.
Over the last few years, many JavaScript developers have sought ways to incorporate object-oriented design into their code. This has given rise to a new type of object in JavaScript called a class, which extends a prototype.
In this guide, we’re going to discuss what classes are, why they are used, and how you can implement a class in your code. We’ll walk through an example to help you get started with classes.
What is a JavaScript Class?
Classes are a way to declare a template for an object.
If you’re familiar with object-oriented programming languages, you’ll know that they depend heavily on classes and objects. A class is like a blueprint for a type of data: it says what data can be stored and what methods it can access. An object is an instance of that class.
An example of a class would be Book
. This class would contain the blueprint for what information could be stored on books and how that data could be changed. An object could be A Streetcar Named Desire, which is an individual book.
In JavaScript, classes are what we call syntactic sugar. The class syntax is sweet to have, but they do not necessarily add in any new functionality.
Classes are commonly used in modern JavaScript frameworks such as React and Next.js.
Classes vs. Functions
If you remember one thing from this article, let it be this: classes are just normal functions.
The only difference between a class and a function expression is how they are declared; functions use the function
keyword and classes use the class
keyword. Technically, you could declare a class without even using the class keyword.
We can tell that they are the same by creating two functions and printing out their prototypes:
const PlayerFunction = function() {} const PlayerClass = class {} console.log(Object.getPrototypeOf(PlayerFunction)); console.log(Object.getPrototypeOf(PlayerClass));
Our class expression and function returns:
function ()
function ()
Both our functions and classes use the same prototype-based architecture.
How to Define a Class
How do you define a class? That’s a great question.
You can define a class using the class
keyword. Once you’ve defined a class, you can use the constructor() function to initialize it with some default values.
Open up a JavaScript console and paste in this code:
class Book { constructor(title, author) { this.title = title; this.author = author; } }
This creates a class called Book
. Our class can store two values, title and author, which we defined in our class constructor method. To create an object of this class, we could use this code:
var streetcar = new Book("A Streetcar Named Desire", "Tennessee Williams");
We can retrieve values from our class like we would from any object:
console.log(streetcar.title);
Our code returns: A Streetcar Named Desire.
How to Define a Class Method
One of the benefits of using classes over prototypes is that you can declare a method inside a class. This means that you don’t need to use the prototype
syntax to add a new method to an existing function.
Consider this example:
class Book { constructor(title, author) { this.title = title; this.author = author; } getDetails() { console.log(`${this.title} is a book written by ${this.author}.`); } }
We’ve declared a method called getDetails()
which exists inside our class. This is nice because it keeps all of our code grouped together. Let’s call our getDetails()
function:
var streetcar = new Book("A Streetcar Named Desire", "Tennessee Williams"); streetcar.getDetails();
Our code returns:
A Streetcar Named Desire is a book written by Tennessee Williams.
Inheriting Classes
Like in any object-oriented structure, classes can inherit properties from other classes.
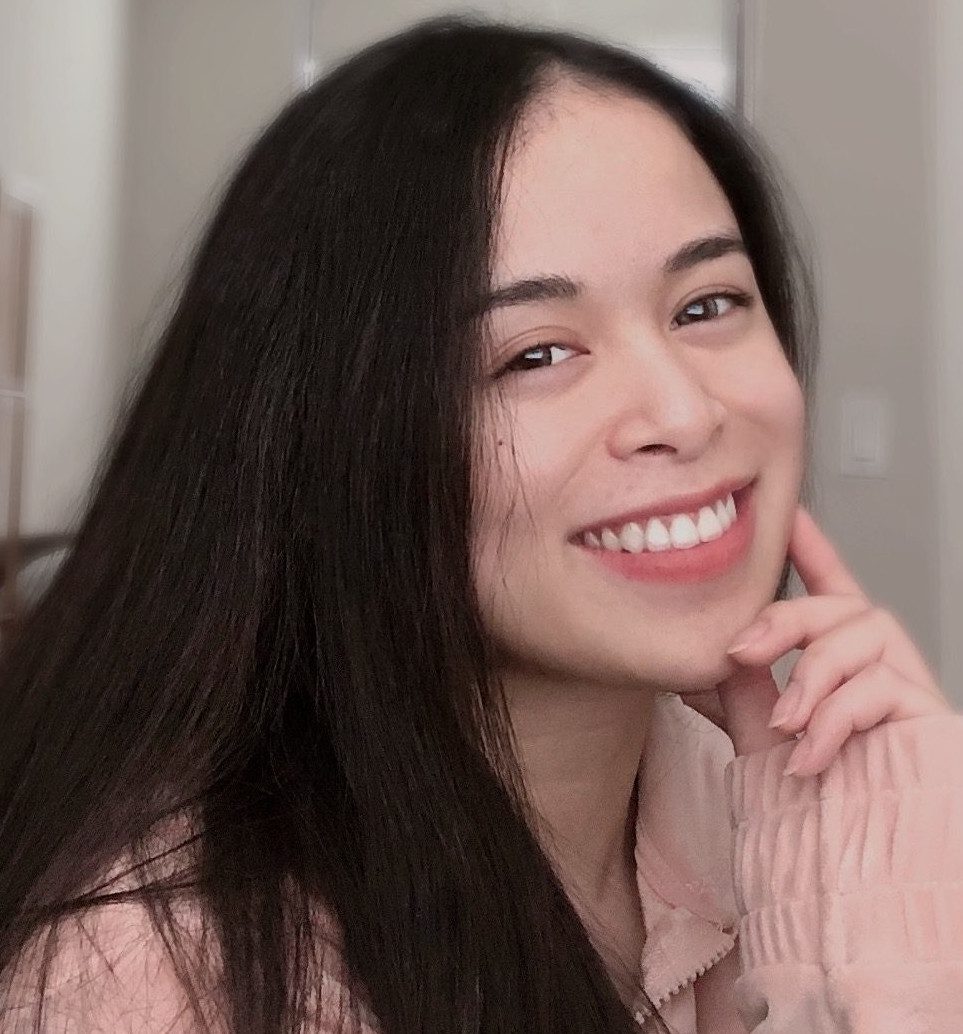
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Inheritance refers to a process where a child class can access methods and data from a parent class. To inherit a class, you can use the extends
keyword. Create a JavaScript file or open your JavaScript console and paste in this code:
class Fiction extends Book { constructor(title, author, fiction) { super(title, author); this.fiction = true; } }
We’ve created a class called Fiction which uses the Book class we declared earlier as a blueprint. In our code, we’ve used the super()
keyword to inherit the values title and author from the parent class, which is Book. Then, we’ve defined a new attribute, fiction
, whose value is set to true by default.
Let’s create an object of our new Fiction class:
var gatsby = new Fiction("The Great Gatsby", "F. Scott Fitzgerald"); console.log(gatsby.fiction);
Our code returns: true. The Fiction class stores an item called fiction
which is true by default. If we tried to access this value from an instance of the Book class, nothing would be returned. That’s because “fiction” is only accessible in our Fiction class.
Getters and Setters
When you’re working with classes, you should make use of the getter and setter functions.
Getter functions allow you to retrieve methods from a class. Setter functions allow you to change the value of a particular item in a class. These functions are denoted using the get
and set
keywords, respectively.
Consider the following code:
class Book { constructor(title, author) { this.title = title; this.author = author; } get author() { return this.author; } set author(author) { this.author = author; } }
In this example, we’ve declared two methods called author
. One of these methods allows us to retrieve the value of author
; that’s our get
method. The other method allows us to change the value of author
.
We can see this in action using the following code:
var streetcar = new Book("A Streetcar Named Desire", ""); streetcar.author = "Tennessee Williams"; console.log(streetcar.author);
Our code returns: Tennessee Williams.
We’ve initialized an object called streetcar
and gave it the title A Streetcar Named Desire
. We then set the value of author
to Tennessee Williams
using the setter that we defined in our code. Finally, we printed out the value of author
to the console using the getter method.
Conclusion
While classes do not add any new functionality into JavaScript, they do make writing functions easier to understand if you’re used to object-oriented programming.
Classes abstract the prototype-based design of JavaScript and make it seem more object-oriented. The word “seem” is crucial because, remember, classes are functions. Classes are nothing more than syntactic sugar.
Now you’re ready to start using classes like a JavaScript expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.