You’ve probably seen a few code snippets use the word “callback” inside a function. Callbacks are a special type of function that are passed inside another function.
Callbacks are often used inside event handlers to run a block of code. In this guide, we’re going to talk about what callback functions are and how they work. We’ll walk through an example of a callback function to help you learn how to use them in your code.
What is a Callback Function?
A callback function is a function that is passed as a parameter into another function.
Callback functions are run within the function in which they are declared. When you execute a function, its callback function, if one is specified, will execute. Once it has run, the callback function will return a response to the main function.
Callback functions work because in JavaScript, every function is an object. This means that we can work with them like any other object. We can assign functions to variables, or pass them as arguments, just like we would with any other value.
Let’s use a simple example to show how callbacks work. We’re going to create a function which prints out a user’s name for a video game to the console, followed by their character type. Let’s start by declaring a function:
function printName(name, callback) { console.log(`Player Name: ${name}`); callback(); }
This is an anonymous JavaScript function which contains a callback. Anonymous functions are functions without a name. They usually appear inside other functions, like in the example above.
This callback is the second parameter in our code. When this function is run, the name of our player is printed to the console. Then, the contents of our callback() function are executed.
Now, let’s use this function:
printName("Violet", function() { console.log("Character Type: Mage"); })
We have called our function printName()
in this code. We have specified “Violet” as the player’s name. We have specified a function which prints out the character type as the callback. Let’s run this code and see what happens:
Player Name: Violet
Character Type: Mage
The contents of the printName()
function are executed, followed by the contents of our callback function. Callback functions do not need to be declared when you are passing them as a parameter. We could refactor our code so that our callback function is on its own:
function printCharacterType() { console.log("Character Type: Mage"); } printName("Violet", printCharacterType())
This code will return the same response. We have created a function called printCharacterType()
which prints the character type of a player to the console. This function is executed when we run printName()
, as it is specified as a callback.
Using Callbacks with Events
Callbacks are commonly used with JavaScript events.
Events listen out for an action, like a user clicking a button, and run a block of code when that action is taken. Let’s create a callback which runs when a user hovers over an image. Open up an HTML file and paste in the following code inside a <body> tag:
<img src=“
https://careerkarma.com/favicon.ico
” id=“image” />
This defines an image on the web page which a user can click. Then, enclose the following JavaScript code within a <script>
tag:
var image = document.querySelector("#image") image.addEventListener("mouseover", function() { console.log("The user has moused over the image."); });
This code selects the element with the ID “image”. It then uses the addEventListener method to listen out for when the user “mouses over” (hovers over) the image. When the user hovers over the image with their cursor, a message will be printed to the console:
The user has moused over the image.
Using Callbacks with Web Requests
Web requests usually need to be executed in a synchronous way. This is because most web applications need to retrieve particular data before the rest of a page can be loaded.
Let’s create a program which makes a request to the JSON Placeholder API, an API with dummy data that we can use for testing purposes. Our program should print the response to the console. We’ll start by declaring a function which makes the request:
function makeRequest(url, callback) { var query = await fetch(url).then(res => res.json()); callback(query); }
This function will make a web request using the fetch() API. The response is assigned to the variable “query”. We return the result of this web request to our callback by passing “query” as an argument in our callback function. Now, let’s call our function and print out its response to the console:
makeRequest("https://jsonplaceholder.typicode.com/posts/1", function(data) { console.log(data); });
This code instructs the makeRequest method to make a request to the JSON Placeholder API. Our callback function prints the data returned from this request to the console:
{ "userId": 1, "id": 1, "title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit", "body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto." }
Great! Our code has made a web request and printed its response to the console.
Conclusion (and Challenge)
Callbacks allow you to pass a function as a parameter inside another function. They are commonly used within event handlers to run code when an event is executed or to make web requests.
Are you looking for a challenge? Write a callback function which executes when a button is pressed on a web page. If you’ve already done this, try to write a function which sorts a list of items and uses a callback function to print each one to the console.
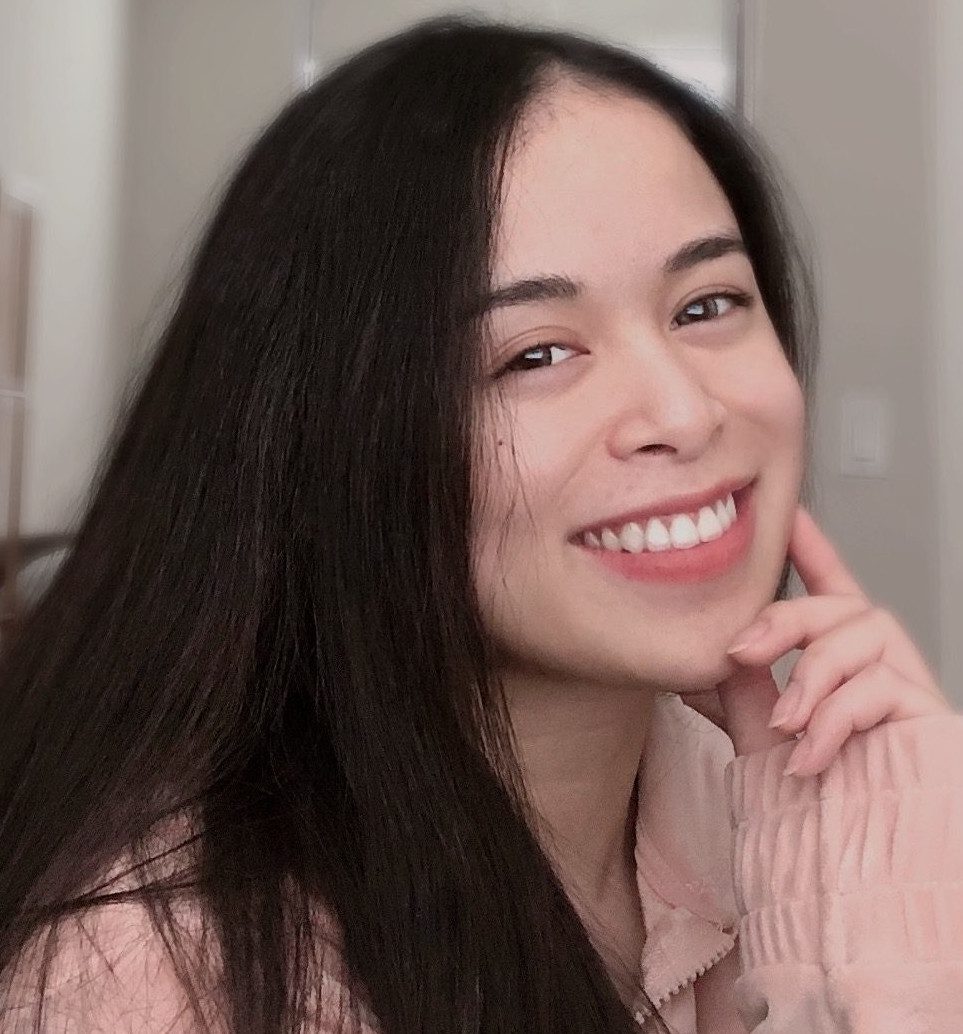
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now you’re ready to start using JavaScript callbacks for asynchronous programming like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.