How to Write a JavaScript Bubble Sort
Do you have a list of values that you need to sort? The bubble sort could be for you. Bubble sorts compare adjacent elements in a list and swap their positions if they are not in the correct order.
In this guide, we’re going to talk about what bubble sorts are and how they work. We’ll walk through how to write a bubble sort in JavaScript so you can quickly get started with this sort.
Let’s get started!
What is a JavaScript Bubble Sort?
A bubble sort, or a “sinking sort,” is a simple sorting algorithm that compares a pair of adjacent elements in a list. If an element is not in the right order, we swap the element with the one before. Otherwise, the element remains in the same place.
The bubble sort got its name because it loops through a list and moves all the largest values to the end. Another way of thinking about this is that the largest values “bubble up” at the end of the list. Bubble sorts work in both ascending or descending order.
There are two types of bubble sorts: regular and optimized.
Regular bubble sorts make all possible comparisons irrespective of whether an array is sorted. Optimized bubble sorts stop executing after an iteration has finished if no swapping has occurred.
Bubble Sort JavaScript Walkthrough
We’ll begin by talking about how bubble sorts work, and then we will implement one in JavaScript. Consider the following list of items:
9 | 3 | 2 | 11 |
To start our sort, we will compare the first and second numbers. If the first number is greater than the second number, we swap the items. Otherwise, the items stay in the same place.
9 is greater than 3 so the positions of the first two elements swap:
3 | 9 | 12 | 2 |
This process continues until we compare every item in the list.
9 is not greater than 12, so these items stay in the same place. 12 is greater than 2, so these items swap:
3 | 9 | 2 | 12 |
Our list is now starting to look more ordered. Our algorithm has iterated through the list once. It will continue to do so until we order every item. In the next iteration, our program makes the following comparisons:
- Is 3 greater than 9? No, so nothing happens.
- Is 9 greater than 2? Yes, so the items swap.
- Is 9 greater than 12? No, so nothing happens.
After this iteration, our list looks like this:
3 | 2 | 9 | 12 |
We’re almost there. In the next iteration we swap the first two elements, which gives us a completely sorted list:
2 | 3 | 9 | 12 |
We did it! We have sorted a list using a bubble sort. Now comes the tricky part: implementing this algorithm in JavaScript.
How to Write a Bubble Sort in JavaScript
We can write a bubble sort algorithm in JavaScript. We’ll create two bubble sorts: a regular bubble sort and an optimized one.
Regular Bubble Sort
Let’s start by defining a JavaScript function that conducts our bubble sort:
function sortItems(array) { for (let i = 0; i < array.length; i++) { for (let j = 0; j < array.length; j++) { if (array[j] > array[j + 1]) { let temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; } } } return array; }
This function takes in an array of numbers and sorts it using the bubble sort algorithm. To start, the algorithm creates a for loop which iterates through each item in the list.
Our code uses the array length attribute to calculate the length of the list. Then, we declare another for loop. This for loop makes comparisons between each element in the list.
For each iteration of our inner loop, our program executes an if statement. This JavaScript if statement checks if the number on the left of a comparison is greater than the number on the right. If it is, our program swaps the numbers. Otherwise, nothing happens.
We return the array to the main program after sorting it. Let’s call our function and give it an example array:
var numbersToSort = [9, 3, 2, 11]; var sortedList = sortItems(numbersToSort); console.log(sortedList);
We have declared a JavaScript variable called numbersToSort which contains the numbers we want to sort. We have then called our sortItems() method and passed this variable as a parameter. This sorts our list. We print the newly-sorted list to the browser JavaScript console: [2, 3, 9, 11].
This code sorts our list in ascending order. We can change this behavior by replacing the “greater than” sign in our “if” statement with a “less than” sign:
if (array[j] < array[j + 1]) {
We’re almost done! Let’s make our code more efficient by implementing a bubble sort with a swapped variable.
Optimized Bubble Sort
Optimized bubble sorts introduce a new variable. This variable keeps track of whether a swap occurs. The sort will stop if no swaps have occurred.
To make our bubble sort more efficient, we will replace our outer for loop with a while loop:
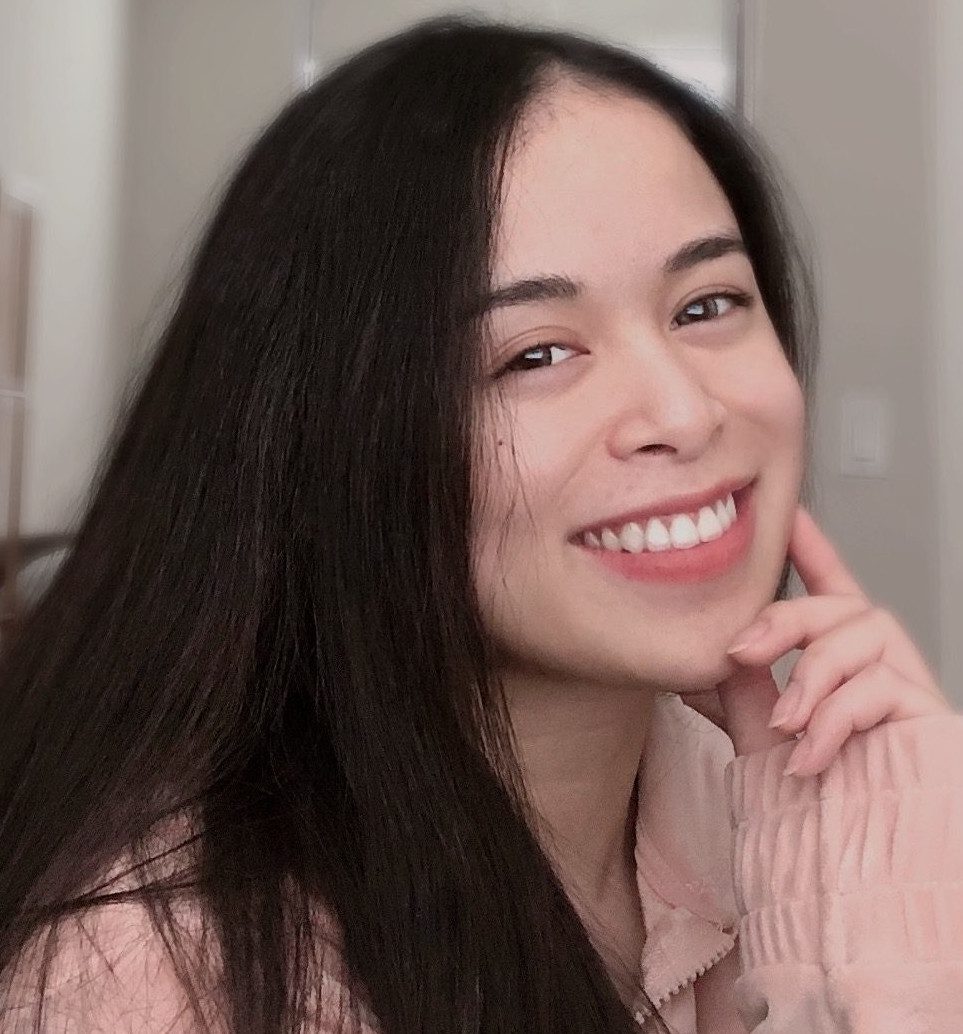
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
function sortItems(array) { let swapped = true; do { swapped = false; for (let j = 0; j < array.length; j++) { if (array[j] > array[j + 1]) { let temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; swapped = true; } } } while (swapped); return array; }
The while loop will execute until “swapped” is equal to false. By default, the value of “swapped” is true. In each iteration of our list, we set the value of “swapped” false. If a swap occurs, the value of “swapped” returns to true.
This allows us to keep track of whether a swap was made in an iteration. If no swap was made, it means our list is sorted. If this is the case, we can stop our bubble sort.
Let’s try to use this bubble sort:
var numbersToSort = [9, 3, 2, 11]; var sortedList = sortItems(numbersToSort); console.log(sortedList);
Our code returns: [2, 3, 9, 11]. Our list is sorted. This algorithm is more efficient because it does not perform any unnecessary comparisons. As soon as the list is sorted, the algorithm stops running.
Conclusion
Bubble sorts are a simple way to sort a list. They compare adjacent items in a list and swap them if they are not in the right order.
There are more efficient sorts available such as an insertion sort or a merge sort. These sorts are more advanced. Bubble sorts are usually the best way to start learning about sorting algorithms.
To learn more about coding in JavaScript, read our How to Learn JavaScript guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.