JavaScript arrow functions are a way of declaring functions. Arrow functions can be used to write JavaScript functions more efficiently and using more concise syntax. Arrow function syntax supports implicit returns for single expressions.
A function is a block of code that performs a specific action and/or returns a value. Functions are used by programmers to create code that can be reused multiple times. This helps reduce the number of lines in a program, thereby making it more efficient.
Traditionally, JavaScript offered one way to declare a function: using the function
keyword. However, since ES6, JavaScript supports arrow functions
which can be used to more efficiently define a function.
In this guide, we are going to explore the basics of functions in JavaScript and discuss how the JavaScript arrow function can be used. We’ll also discuss where you may want to use an arrow function, and explore a few examples to illustrate how arrow functions can be used in practice.
JavaScript Function Refresher
The most common method used to declare a function in JavaScript is by using the regular function
keyword. The function
keyword allows coders to declare a function that can be referenced throughout their code.
Here’s the syntax for a JavaScript function:
function nameOfTheFunction() { // Code }
Our function contains a number of parts. Firstly, we use the function
keyword to tell our program that we are going to declare a function. Then, we specify the name of our function, which in the above example is nameOfTheFunction
. It’s important to note that function names use the same rules as variables, and so they are written in camel case.
Our function then is followed by a set of brackets, which can optionally be used to parse parameters. Then, our code is contained within a set of curly brackets ({}
).
Let’s use an example to illustrate how a function works in JavaScript. The following code creates a function called wakeUpRoutine
which prints a morning message to the console:
function wakeUpRoutine() { console.log("Good morning! Today is a great day."); }
Right now, if we run our program, nothing will happen. This is because our function has been declared, but we have not yet called the function. If we want to run our function, we can call it by writing out the name of the function:
wakeUpRoutine();
Our program returns:
Good morning! Today is a great day.
We can use our function as many times as we want throughout our program by calling it where we want it to be executed.
JavaScript functions can also take parameters. Parameters are input that are passed into a function and act as local variables within the function. So, let’s say that we wanted our wake up message to include our name. We could use the following code to include our name in the wake up message:
function wakeUpRoutine(name) { console.log(`Good morning, ${name}! Today is a great day.`); }
In our code, we have not defined our name
parameter. That’s because we assign it a value when we call our function. Let’s say that our name is Peter
. In order to make our message appear with our name, we would use the following code:
wakeUpRoutine("Peter");
Our program returns:
Good morning, Peter! Today is a great day.
The value of our name
parameter is passed into the wakeUpRoutine function, which in this case is Peter
. We then use the name
variable in our function to reference the name our program has specified.
JavaScript Arrow Function
JavaScript arrow functions, sometimes referred to as fat arrow
functions, are one of the most popular features introduced in ES6. These function expressions can be used to write functions more efficiently and using more concise syntax.
The syntax for a JavaScript arrow function is as follows:
functionName = (parameters) => { // Code }
Let’s use the wake up routine example to illustrate how the JavaScript arrow functions. In our above code, we had to use the “function” keyword.” However, with an arrow function, we use the =>
syntax instead. Here’s an example of our wake up routine function written as an arrow function:
wakeUpRoutine = (name) => { console.log(`Good morning, ${name}! Today is a great day.`); }
In order to call our function, we use the same syntax as we would with a traditional function:
wakeUpRoutine("Sophia")
Our function returns:
Good morning, Sophia! Today is a great day.
The structure for arrow functions is very similar to that of a traditional function. The only differences are that we don’t use the function
keyword in an arrow function, and instead we use a =>
arrow.
In addition, notice that we did not need to use the return
keyword in our arrow function, which makes our code even more concise.
Implicit Returns
Arrow functions are particularly useful if the function body is a single expression. If the function body is a single expression, you can remove the curly brackets and create an implicit return to simplify your function. This is different from a traditional function where the number of lines used in your function did not affect how the function was defined.
Here’s an example of a one-line arrow function:
const multiplyNumbers = (a, b) => a * b; multiplyNumbers(2, 2);
Our code returns: 4
. As you can see, our arrow function has multiplied our two numbers.
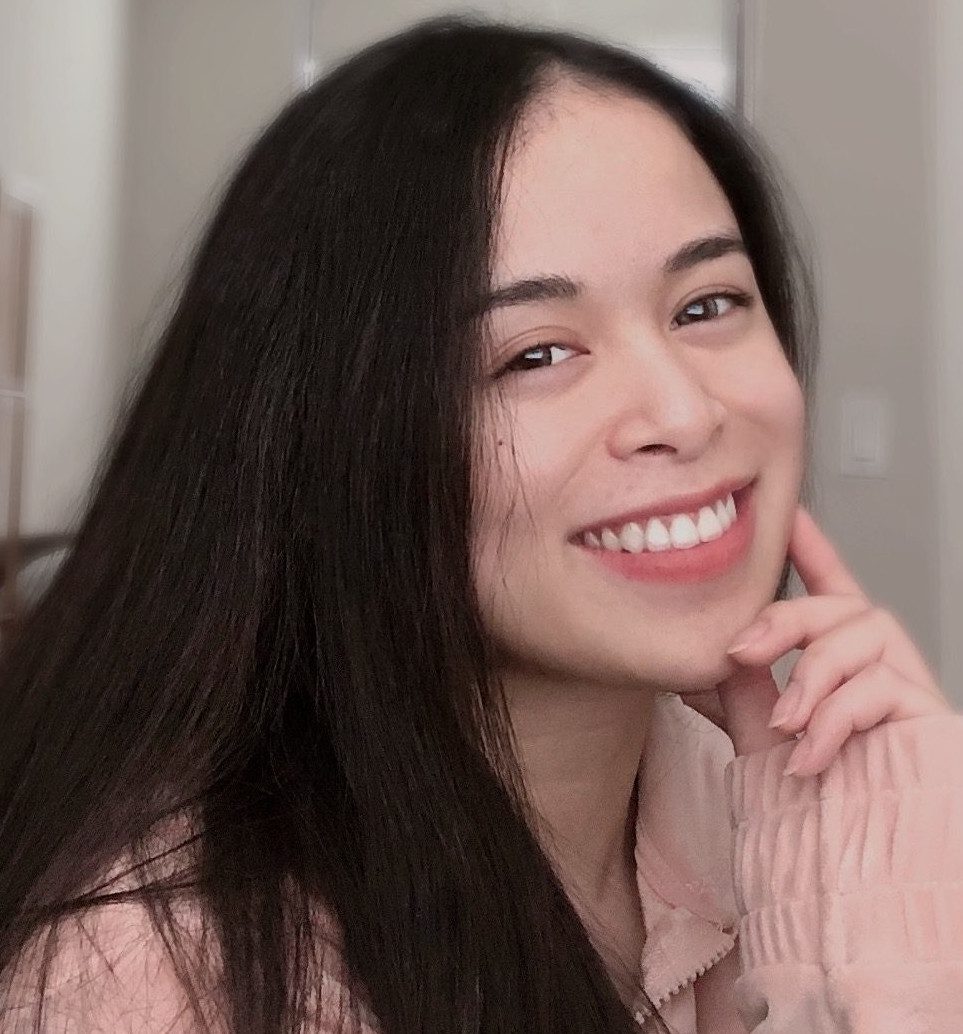
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The implicit return syntax is usually used if you are returning values, like we did above. However, you can also use the syntax to execute code that will not return any values. So, if you wanted to use implicit syntax to recreate our wake up routine function, you could use this code:
const wakeUpRoutine = name => console.log(`Good morning, ${name}! Today is a great day.`); wakeUpRoutine("Ava");
Our code returns the following:
Good morning, Ava! Today is a great day.
Removing Parentheses
JavaScript arrow functions only need parenthesis around the parameters if there are no parameters or more than one. So, if your arrow function only has one parameter, you can remove the parentheses like this:
wakeUpRoutine = name => { console.log(`Good morning, ${name}! Today is a great day.`); }
As you can see, our name
parameter is no longer surrounded by parentheses.
Arrow Functions with Map, Filter, and Reduce
Arrow functions are commonly used with the map, filter, and reduce JavaScript array methods, as arrow functions allow you to transform an array in only one line.
Let’s say that we have an array of student grades. We want to add 1
to each student grade because there was an error in a recent test that meant students could not answer a certain question, and so nobody could get a perfect score of 30
. Here’s an example of an arrow function we could use to fix the test scores:
const grades = [25, 26, 22, 28]; const fixStudentGrades = grades.map( score => score + 1 ); console.log(fixStudentGrades);
Our code returns:
[26, 27, 23, 29]
As you can see, our code has added 1
to each grade in our grades
array.
Conclusion
Arrow functions are a feature introduced in ES6 JavaScript that can be used to write more efficient and concise code. Arrow functions allow you to use the =>
arrow in place of a function
statement, and also facilitate writing one-line functions in certain circumstances.
In this tutorial, we explored the basics of how functions work in JavaScript, then we discussed how to write arrow functions. In addition, we also explored implicit returns, removing parentheses, and using arrow functions with the map
, filter
, and reduce
functions.
Now you’re equipped with the knowledge you need to write an arrow function like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.