The innerHTML property lets you set the contents of an element using JavaScript.
If you specify an invalid element with which to use the innerHTML method, or if you place your script before the element appears on the page, you’ll encounter the “Uncaught TypeError: cannot set property ‘innerHTML’ of null” error.
In this guide, we discuss what this error means and why you may encounter it. We’ll walk through an example of this error so you can learn how to resolve it in your JavaScript code.
Uncaught TypeError: cannot set property ‘innerHTML’ of null
The innerHTML property is associated with any web element that you are working with in JavaScript. You may have selected a web element from the page using a “getter” like getElementById(), or you may have created an element in JavaScript that you want to modify.
The “cannot set property ‘innerHTML’ of null” error is a type error. This means we are trying to apply a property or a function to a value that does not support a property or function.
In this case, we’re trying to set the value of innerHTML on an element that is equal to null. Null values do not have an innerHTML property.
There are two common causes of this error:
- Placing a script before an element appears on the web page
- Referencing the wrong element ID.
We’re going to walk through the first cause, which is arguably the most common mistake made by beginners.
An Example Scenario
We’re going to write a simple website that displays the time. To start, define a basic HTML page. This HTML page will be the canvas on which we display the time:
<!DOCTYPE html> <html lang="en"> <head> <title>Time</title> </head> <body> <p>The time is <span id="show_time"></span>.</p> </body> </html>
This HTML page contains a <head>
and a <body>
tag. The <head>
tag contains a <title>
tag which defines the title for the web page. The <body>
tag contains a paragraph.
Within the paragraph, we have added a <span> tag. We’re going to replace the contents of this tag with the current time in our JavaScript code.
Next, write a script to retrieve the time. We’ll add this JS code inside the <head>
tag:
… <title>Time</title> <script> var show_time = document.getElementById("show_time"); var current_date = new Date(); var pretty_time = `${current_date.getHours()}:${current_date.getMinutes()}` show_time.innerHTML = pretty_time; </script> …
Our document now contains HTML and JavaScript. The getElementById()
method retrieves our show_time
span tag. We then retrieve the current date using the JavaScript Date module.
On the next line of code, we create a string which displays the current hour of the day and the minute of the hour. This string uses the following structure:
HH:MM
There is a colon that separates the hour and minute values. We create this string using a technique called JavaScript string interpolation. This lets us embed multiple values into the same string. Finally, we use the innerHTML method to display this time on our web page.
Let’s open up our web page in the web browser:
Our web page fails to display the time. If we look at the JavaScript console, we can see an error:
Uncaught TypeError: show_time is null
This is another representation of the “cannot set property ‘innerhtml’ of null”. It tells us the element on which we are trying to set the value of innerHTML is null.
The Solution
Because the value of show_time
is null, we can infer that JavaScript was unable to successfully retrieve the element whose ID is show_time
.
In this case, the cause is that we have placed our <script>
tag too early in our program. Our <script>
tag appears in the header of our page. This is a problem because without using an onload()
function, we can only select elements that come before our <script>
.
To solve this error, we need to move our <script>
below our paragraph:
... <body> <p>The time is <span id="show_time"></span>.</p> </body> <script> var show_time = document.getElementById("show_time"); var current_date = new Date(); var pretty_time = `${current_date.getHours()}:${current_date.getMinutes()}` show_time.innerHTML = pretty_time; </script> …
We have moved our <script>
tag below the <body>
tag. Let’s try to open our web page again:
The web page successfully displays the time.
If this does not solve the error for you, make sure that you correctly select the element that you want to work with in your code. For instance, consider this line of code:
var show_time = document.getElementById("show_times");
If we used this line of code to select our element, we would see the same error that we encountered before. This is because there is no element whose ID is “show_times”.
Conclusion
The “Uncaught TypeError: cannot set property ‘innerHTML’ of null” error is caused by trying to set an innerHTML value for an element whose value is equal to null.
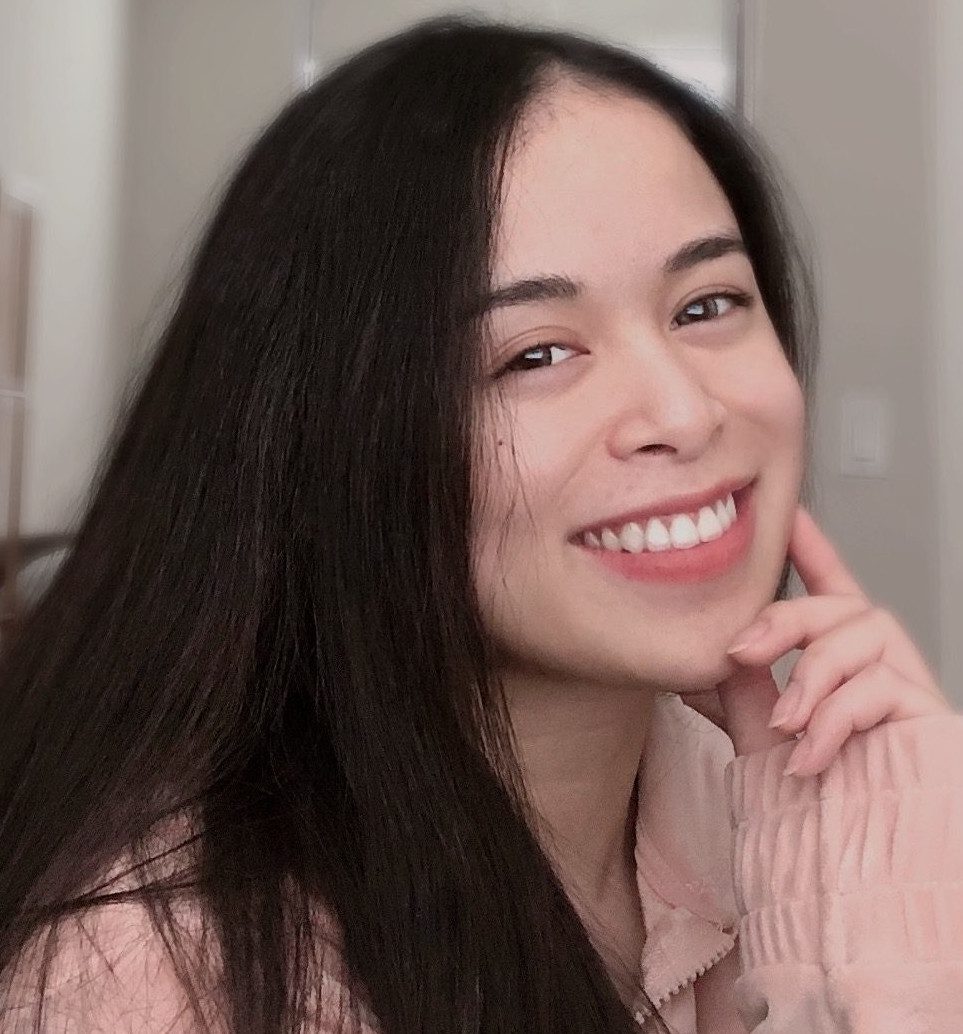
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
To solve this error, make sure your <script>
tag comes after the element that you want to select in your script. If this does not solve the issue, check to make sure you are selecting a valid element in your program.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
Well Explained .