The JavaScript getElementById method selects an element from the HTML Document Object Model (DOM). If you incorrectly spell this method, you’ll encounter the document.getElementByID is not a function
error in your program.
This tutorial discusses what this error means and why it is raised. We’ll walk through an example of this error so you can learn what you need to know to fix it.
document.getElementByID is not a function
The document.getElementById()
method is one of the JavaScript selector methods. The method retrieves an object based on its ID from the HTML DOM.
This method is used to manipulate the contents of an element or retrieve information about a particular element, such as its contents or its attributes.
IDs are defined in HTML. An ID must be unique to a particular element:
<header id=“top_header”></header>
The <header>
tag has the ID top_header
. This ID is unique to the <header>
tag. IDs are often used to apply styles to particular elements using CSS.
document.getElementById()
is case-sensitive. This method is not:
- document.getelementbyid()
- document.getElementByID()
- document.GetElementById()
Any other variations on the method name will not be accepted by JavaScript.
While it is tempting to write ID in capital letters because it is shorthand for another word, the method requires that you use an uppercase I and a lowercase d.
To learn more about the getElementById method, read our guide on JavaScript getElementById.
An Example Scenario
We’re going to build a code snippet that welcomes a user to our application. The welcome message will be in a title that appears at the top of our page.
For the purposes of this example, we’re going to use a demo name.
To start, define our body HTML code for the welcome section of the webpage:
<html> <body> <header> <h1 id="welcome_title">Welcome</h1> </header> </body> </html>
By default, our <h1>
tag will contain the contents Welcome
. The <header>
tag is the parent tag in which our title is located. Next, we’re going to add a <script>
tag below our <header> tag. The script tag will contain all of the JavaScript code for our welcome message:
… </header> <script> </script> </body> ...
Within our script, we are going to select our title and change its contents:
var title = document.getElementByID("welcome_title"); title.textContent = "Welcome, Luke!";
The getElementById()
method selects our title. We assign this HTML element to the JavaScript variable called title
. The contents of our title will be changed to display “Welcome, Luke!” We use the textContent
method to change the title.
Let’s run our code and see what happens. If we open our JavaScript console, we can see an error:

Our code is not functioning correctly. Our title has not been changed:
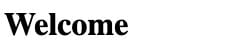
The Solution
We’ve used the incorrect case for the document.getElementByID
selector. Instead of using an uppercase I and a lowercase d, we have used an uppercase I and D.
JavaScript does not register our program as calling the getElementById
function because the function name is case sensitive.
We need to change our code so that we use the correct cases for our function:
var title = document.getElementById("welcome_title"); title.textContent = "Welcome, Luke!";
We are now using Id
instead of ID
in our function call. Let’s run our new code:

Our program successfully changes the contents of our title to include the name of our user.
Conclusion
The document.getElementById()
method selects an element from the web page. This method lets you view and change the contents of an element.
When you use this method, you should make sure that you use the correct cases. ID is not in all capital letters. You need to use an uppercase I and a lowercase d for the method to work.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.