In programming, there are often instances where you have a repetitive task you want to execute multiple times. Loops are used to automate these repetitive tasks and allow you to create more efficient code.
The while
and do...while
loops in Java are used to execute a block of code as long as a specific condition is met. These loops are similar to conditional if
statements, which are blocks of code that only execute if a specific condition evaluates to true. Unlike an if
statement, however, while
loops run until a condition is no longer true.
This tutorial will discuss the basics of the while
and do...while
statements in Java, and will walk through a few examples to demonstrate these statements in a Java program.
Java While Loop
The while
loop loops through a block of code as long as a specified condition evaluates to true. The syntax for the while
loop is similar to that of a traditional if
statement. Here’s the syntax for a Java while
loop:
while (condition_is_met) { // Code to execute }
The while
loop will test the expression inside the parenthesis. If the Boolean expression evaluates to true, the body of the loop will execute, then the expression is evaluated again. The program will continue this process until the expression evaluates to false, after which point the while
loop is halted, and the rest of the program will run.
Let’s walk through an example to show how the while
loop can be used in Java. Say we are a carpenter and we have decided to start selling a new table in our store. We only have the capacity to make five tables, after which point people who want a table will be put on a waitlist.
We want to create a program that tells us how many more people can order a table before we have to put them on a waitlist. We could do so by using a while
loop like this which will execute the body of the loop until the number of orders made is not less than the limit:
int limit = 5; int orders_made = 0; while (orders_made < limit) { orders_made++; int capacity = limit - orders_made; String message = capacity + "more tables can be ordered."; System.out.println(message); }
Our code returns:
4 more tables can be ordered. 3 more tables can be ordered. 2 more tables can be ordered. 1 more tables can be ordered. 0 more tables can be ordered.
Let’s break down our code. On the first line, we declare a variable called limit
that keeps track of the maximum number of tables we can make. Then, we declare a variable called orders_made
that stores the number of orders made.
Our program then executes a while
loop, which runs while orders_made
is less than limit
. Then, we use the orders_made++
increment operator to add 1 to orders_made
.
After the increment operator has executed, our program calculates the remaining capacity of tables by subtracting “orders_made
from limit
. Then, it prints out the message [capacity] more tables can be ordered.
to the console.
Our “while” statement stops running when orders_made
is larger than limit
.
Java Do While Loop
The do…while
loop is a type of while
loop. The do...while
loop executes the block of code in the do
block once before checking if a condition evaluates to true. Then, the program will repeat the loop as long as the condition is true.
The difference between while
and do...while
loops is that while
loops evaluate a condition before running the code in the while
block, whereas do…while loops evaluate the condition after running the code in the do
block.
The syntax for the do…while loop is as follows:
do { // Code to execute } while (condition_is_met);
Let’s use an example to explain how the do…while loop works.
Say that we are creating a guessing game that asks a user to guess a number between one and ten. We want our user to first be asked to enter a number before checking whether they have guessed the right number. If the user enters the wrong number, they should be promoted to try again. We could accomplish this task using a do…while loop.
Here’s an example of a program that asks a user to guess a number, then evaluates whether the user has guessed the correct number using a do…while loop:
import java.util.Scanner; class GuessingGame { public static void main(String[] args) { int number = 8; int guess = 0; Scanner input = new Scanner(System.in); do { System.out.print("Enter a number between 1 and 10: "); guess = input.nextInt(); } while (number != guess); System.out.println("You're correct!"); } }
When we run our code, we are asked to guess the number first, before the condition in our do...while
loop is evaluated. Here’s what happens when we try to guess a few numbers before finally guessing the correct one:
Enter a number between 1 and 10: 9 Enter a number between 1 and 10: 1 Enter a number between 1 and 10: 4 Enter a number between 1 and 10: 5 Enter a number between 1 and 10: 8 You're correct!
Let’s break down our code. First, we import the util.Scanner
method, which is used to collect user input. Then we define a class called GuessingGame
in which our code exists.
We then define two variables: one called number
which stores the number to be guessed, and another called guess
which stores the users’ guess.
Then, we use the Scanner method to initiate our user input. We print out the message Enter a number between 1 and 10:
to the console, then use the input.nextInt()
method to retrieve the number the user has entered.
After this code has executed, the do…while loop evaluates whether the number the user has guessed is equal to the number the user is to guess. If the user has guessed the wrong number, the contents of the do
loop run again; if the user has guessed the right number, the do…while loop stops executing and the message You’re correct!
is printed to the console.
Infinite Loops
Infinite loops are loops that will keep running forever. If you have a while
loop whose statement never evaluates to false, the loop will keep going and could crash your program. So, it’s important to make sure that, at some point, your while loop stops running.
Here’s an example of an infinite loop in Java:
while (true) { // Code to execute }
This loop will run infinitely. However, we can stop our program by using the break
statement. Let’s say we are creating a program that keeps track of how many tables are in-stock. We only have five tables in stock. When there are no tables in-stock, we want our while
loop to stop. We could create a program that meets these specifications using the following code:
Boolean tables_in_stock = true; int orders_made = 0; while (tables_in_stock) { orders_made++; if (orders_made == 5) { System.out.println("We are out of stock."); break; } else { int tables_left = 5 - orders_made; String message = "There are " + tables_left + " tables in stock."; System.out.println(message); } }
When we run our code, the following response is returned:
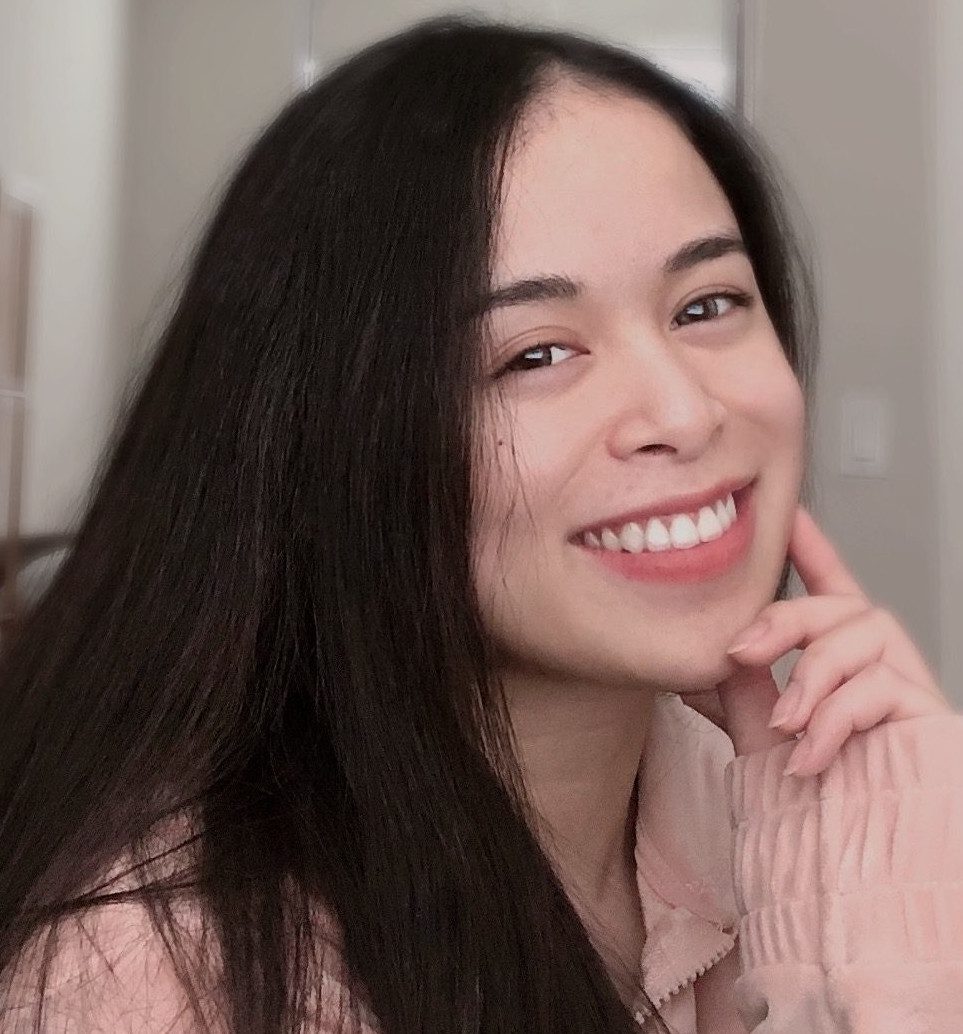
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
There are 4 tables in stock. There are 3 tables in stock. There are 2 tables in stock. There are 1 tables in stock. We are out of stock.
In our example, the while
loop will continue to execute as long as tables_in_stock
is true. But we never specify a way in which tables_in_stock
can become false.
So, in our code, we use a break
statement that is executed when orders_made
is equal to 5.
This means that when fewer than five orders have been made, a message will be printed saying, There are [tables_left] tables in stock.
But when orders_made
is equal to 5, a message stating We are out of stock.
will be printed to the console, and the break statement is executed. When the break statement is run, our while
statement will stop.
Conclusion
The while
loop is used in Java executes a specific block of code while a statement is true, and stops when the statement is false. The do...while
loop executes a block of code first, then evaluates a statement to see if the loop should keep going.
This tutorial discussed how to use both the while
and do...while
loop in Java. We also talked about infinite loops and walked through an example of each of these methods in a Java program. You’re now equipped with the knowledge you need to write Java while
and do...while
loops like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.