JavaScript is one of the standard languages on the Web today and controls the activity of your website. So anything that moves, refreshes, pops up, or changes your screen without refreshing the page itself. It can also be used for solving algorithms in dynamic programming.
Yep. JavaScript.
Whenever you do a Google search or click a button on a website, more than likely the coding behind that web interaction is the result of JavaScript. When JavaScript came out in 1995, the founders branded JavaScript as a language that creates web pages that “come alive.”
But today, it has taken on a life of its own and has become a very important step to someone breaking into the tech industry. JavaScript, along with HTML and CSS, makes up the “Power of 3” in programming and standard web technologies. Every developer has to learn these three languages when starting out in tech.
You’re going to need to learn JavaScript at the start of your tech career. So let’s go ahead and take a look into the basics to get an understanding of what JavaScript is all about.
So, What is JavaScript?
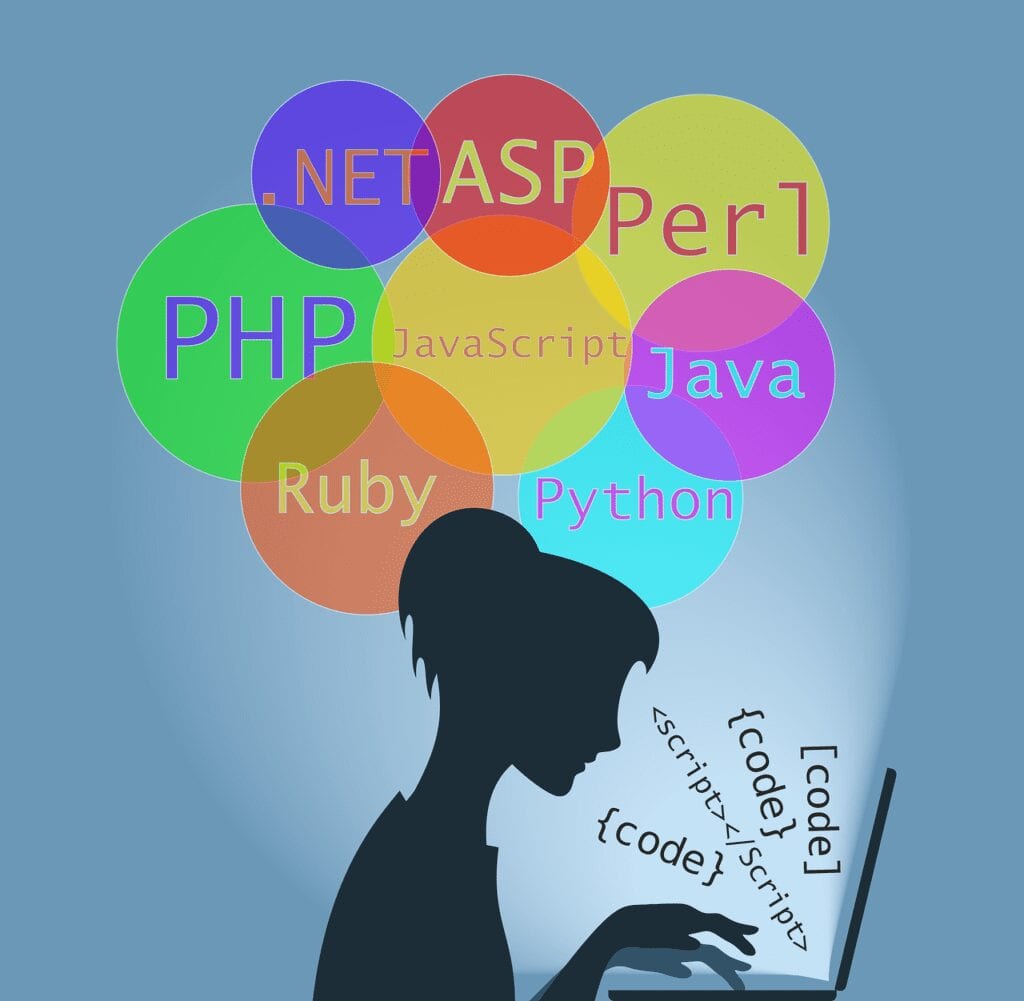
To know how to use JavaScript in your budding career as a developer, you first have to know what it is.
JavaScript is a scripting language that allows you to interact with various items on a web page in real-time. It gives you the ability to create dynamic interactions with your web page rather than stay still on the page (or remain static). JavaScript can do things like animate images, control videos and audio, and change the text within your web page.
Now we know you are probably saying “Oh! I know about Java. That’s what it is, right?”
JavaScript (initially named “LiveScript”) was branded in 1995 as the “younger sibling” of Java to leverage the popularity of Java at the time. As the years went by, it evolved into a language of its own and even has its own specification called ECMAScript. With its growth and rise in the tech world, it no longer has any relationship or association with Java despite the name.
So we mentioned earlier how JavaScript is one of the “Power of 3” in web technology and programming, along with HTML and CSS. In order for you to understand the importance of the three languages, let’s imagine it’s like building a house.
- HTML (HyperText Markup Language) is the programming language that structures and gives meaning to the content on your page. This means that it defines where your paragraphs, links, images, headings, and other items show up on your web page. It sets up the foundation for how your web page (or your house) is laid out. Think of it as your blueprint.
- CSS (Cascading Style Sheets) is a programming language we use to control the look and style of the layout of the HTML. In other words, this would be our materials like paint, wood, bricks, metals, and concrete used to apply the overall look of our blueprint (the HTML). It creates the design of the fonts, colors, borders, columns, and sections in your HTML. It changes your web page from being a boring text page to a beauty of presentation for everyone to enjoy.
- JavaScript (or JS for short) does what HTML and CSS will never be able to do. It’s the magic and activity inside your house. JavaScript is like the electricity running through your house and the water running within the pipes. It controls what happens within your framework and manipulates it according to actions made by a user on the web page. It tells your web page to do something or react in a certain way when an action happens.
When you head to a web page where you have to sign in, more than likely that sign-in button is coded through JavaScript. If it opens up a pop-up screen on the web page for you to enter your login information—probably JavaScript.
JavaScript is so powerful that it is supported by most modern web browsers and some mobile web browsers on Android and iOS.
So to sum it up, JavaScript code can do the following:
- Change HTML content (yes, it can change the words on the page)
- Change HTML attribute values (such as your tags and sections)
- Change HTML styles from your CSS (you can manipulate your IDs and classes)
- Hide HTML elements (if you want something to be removed through the action of a button, JavaScript can do it!)
- Show HTML elements (such as typing a search topic in and providing its results)
You will run into ready-made sets of code that connect two applications together using a set of rules through coding such as JavaScript. These sets of code are called Application Programming Interfaces (or API). The best description of APIs is like the easy to build furniture kits you get from the store to build bookshelves and tables. APIs make it easier to link the parts together instead of having to build everything from scratch.
There are two types of APIs. Browser APIs, which run within your web browser, allow you to expose data from surrounding environments to do complex actions. The DOM, or Document Object Model, will be the most common browser API you use. Third-party APIs, which are not built into your browser, are provided through code blocks and information from another location on the Web. For example, having a Twitter widget on your page to show your latest tweets in real-time is possible through third-party APIs.
Both APIs are built on top of JavaScript code, emphasizing the importance of JavaScript in our web technologies today.
How Does It Work?
All three languages (CSS, HTML, and JavaScript) work together in a collaborative manner through a web interface called the Document Object Model (or DOM). The DOM is built in most major web browsers available today. It gives developers a chance to manipulate actively within the HTML and CSS of a web page using JavaScript.
First, the web browser loads your web page and examines the HTML to create the DOM from the contents. The DOM presents the real-time view of the page to the JavaScript code. Then the browser grabs everything linked to the HTML, which would be your media, images, and CSS rules. The DOM combines the HTML and CSS together to create the web page for the viewer. In the background, the JavaScript engine loads the JavaScript code but only after the HTML and CSS has completely loaded on the live website.
After the webpage is live with the HTML and CSS, JavaScript does its work. It performs the code on the JS file in the order it was written and the DOM reads the results and updates the webpage with the JS code in the browser.
MakeUseOf provides an awesome chart of how the process works between the three languages and the DOM.
How Do I Use It?
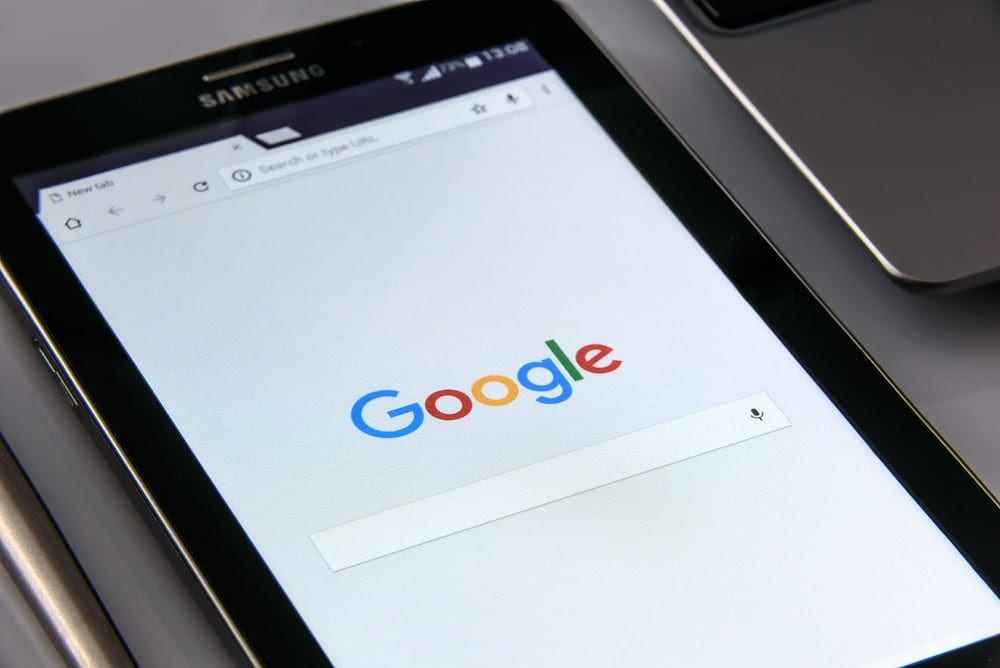
JavaScript is composed of blocks of code called scripts. These can either run directly into the HTML code and run automatically as the page loads, or they can run in the background of the HTML and CSS through a separate file (with a .js extension) and interact with the HTML and CSS through the DOM.
Internal JavaScript is when you run your JavaScript code within your HTML page. This is like the way CSS uses the <link> element to apply stylesheets to the HTML. JavaScript uses the <script> element to apply its code to the HTML.
You should only use this technique if you are dealing with a couple of lines of JavaScript code. It is not recommended to take this approach for lots of JS code because you can clutter your HTML, making it difficult to find errors if you have any. If you want your JavaScript to run on several pages within your application or website, then you should use external JavaScript.
External JavaScript is code that lives in a JS file separate from the HTML and CSS page. The HTML page calls this file through the <script> element in the HTML file by linking the source (or src) attribute to the .js file.
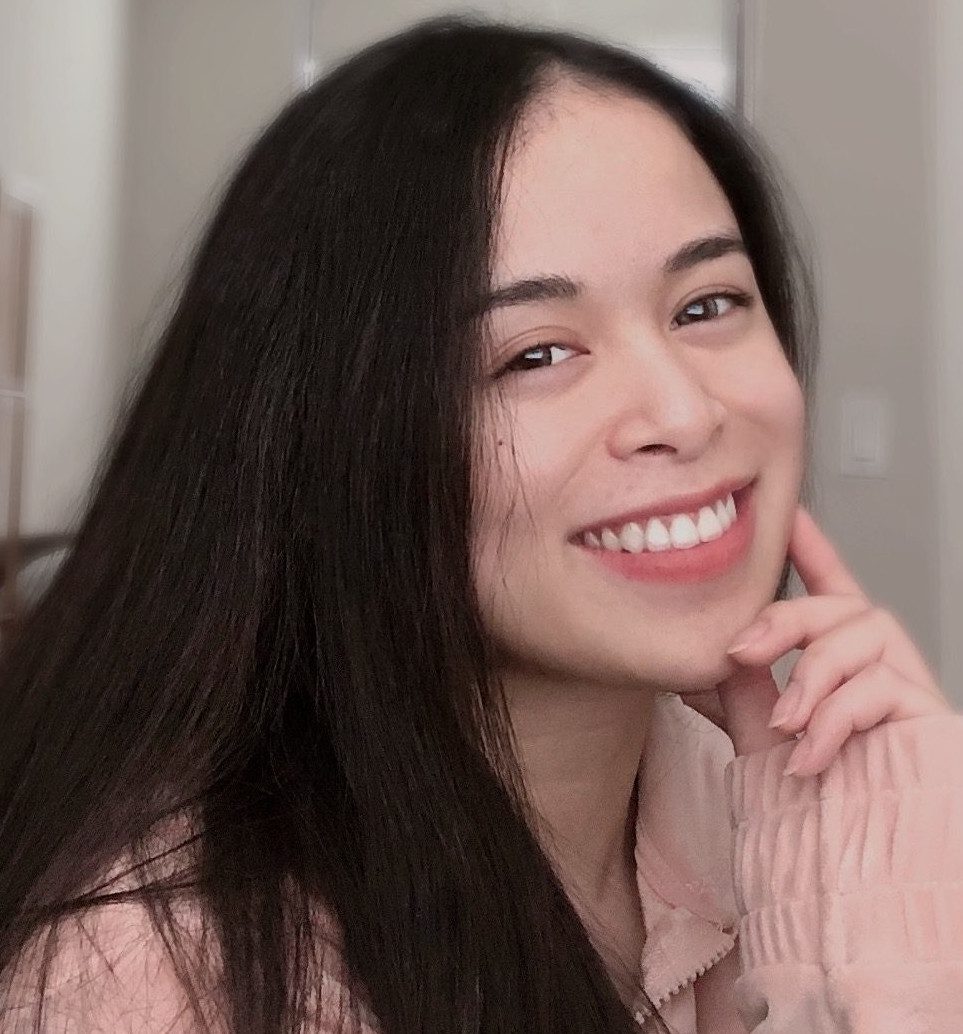
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
JavaScript runs in order from top to bottom through variables. Variables are containers for storing data values of various data types.
The most common data types in JavaScript are:
- Numbers, which are self-explanatory. They can be written with or without decimals and are stored as double-precision, floating-point numbers. But the floating-point system is not always accurate, so you have to keep that in mind when using that format in your code.
- Strings, which store text in your JavaScript. These are identified with quotes, using either single quotes or double quotes (whichever you prefer).
- Objects, which are variables containing several data values types.
- Arrays, which are used to store a group of many values in a single variable. Think of this as a list of items represented by one label—like a shopping list or a wish list.
- Functions, which are code blocks that perform an action when something calls it.
In order for your code to work in your HTML, you have to make sure that your variables are set before providing any functions within your code. Mixing up the order or having your code out of order causes errors to occur on your web page. More than likely, the actions you want to happen on your web page will not happen if your code is out of order and everything isn’t defined.
Also, it’s important to note, in order for your code to work on your web page, you must call your JavaScript code last in your HTML, not first. Errors will occur if you try to run your JavaScript before your HTML and CSS. Remember that the DOM has to recognize your HTML and CSS first before JavaScript can even touch your HTML for interactivity.
How Can I Start Learning JavaScript?
For anyone interested in web development and programming, it is always best to start with HTML and CSS first. Those languages will help you build a basic static web page and help develop structure. As you advance on those, you are going to be hungry for interactivity. That’s where JavaScript comes in handy. Learning JavaScript will definitely improve your tech skills and open many doors for your career.
There are many resources out there that can help you learn to dig deep into the world of JavaScript. First, we have a list of the best JavaScript books recommended by programmers today that will help you get started.
Next, applications like Grasshopper, SoloLearn, freeCodeCamp, and Codecademy will help you with JavaScript. They provide activities and tutoring modules to help teach you step-by-step the world of JavaScript. As you advance towards the more complex languages out there, you’ll realize that JavaScript and Python, as well as other coding languages, connect. It may seem like it is complex in itself, but JavaScript will build you into a well-equipped developer for the future.
Lastly, if you really want to give your career a kickstart, you can look into enrolling in job training programs called coding bootcamps. Career Karma is a great resource to help you build a network with others who are changing their careers and lives by breaking into tech. They also help you get enrolled in these coding bootcamps to provide you access to a path to that dream tech job of your choice.
Summary
So now that you know what JavaScript is and how it works, you see the importance that it has in our growing modern world of technology. Now you know that anything that you’ve done on a web page or application—filling out interactive forms, interacting with multimedia and animations, flipping through photo slideshows online and even that autocorrect feature that we love to hate at times—is all because of JavaScript.
We should not take JavaScript for granted, especially in this growing digital era we currently live in.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.