An API should fill four types of functions. It should be able to create new data and read, update, and delete existing data. In computer science, we refer to these options as CRUD.
The CRUD method is essential in building web applications because it allows you to structure the models you build for your APIs. But how does CRUD work? How do you interact with APIs using CRUD?
In this article, we answer those questions. We’ll start by discussing the basics of CRUD and then walk you through a few examples of how to interact with an API using CRUD.
Why Do We Need CRUD?
Let’s say we are building an API that stores the coffees sold at a cafe we own. This API should be able to store different coffee objects. A coffee object may include:
- A unique identifier for the coffee
- The name of the coffee
- The price of the coffee
We want to make sure the users can interact with and change the data stored on our API. That’s why we need to follow the CRUD structure. After all, what’s an API that stores coffees good for if the company can’t change the price of their coffees? Why would a company use an API that doesn’t allow it to remove records?
What is CRUD?
CRUD is an acronym for Create, Read, Update, and Delete.
When you’re working with web services, CRUD corresponds to the following HTTP methods, which are used to tell a web server how you want to interact with a website:
- Create: POST
- Read: GET
- Update: PUT
- Delete: DELETE
Let’s look at each one individually.
We’re going to use the cURL command line utility to create example queries to a coffee API so that you can see how these operations work. Before we get started, run the following command in a terminal on your computer to ensure cURL is installed:
curl
If an error “curl: command not found” appears in your terminal, you’ll need to install cURL. You can do so by following this tutorial. Otherwise, you’re ready to get started!
Create
An API that stores data for our cafe should allow us to add new records to the menu. In this case, we should be able to create a new coffee item by specifying its name and price.
To add a new coffee to the menu, we can use a POST request, which allows us to submit data to our API that will be stored in a database.
These are details of the coffee item we want to add:
- Name: Espresso
- Price: 1.95
The following cURL command allows us to create a coffee with these details using our API:
curl -X POST http://localhost:5000/add -d name=Espresso -d price=1.95
In this command, we use the -X flag to structure our request as a POST request. We then use -d flags to specify the data we want to send to our API. In this case, we use two -d flags: one to specify the name of the coffee, and the other to specify its price. Our code returns:
{"name":"Espresso","price":"1.95"}
This command creates a new coffee in our database with the name “Espresso” and the price “1.95”.
Read
Our API should allow us to see a list of all the coffees on our menu. To see this data, we can use a GET request. This allows us to see a list of the coffees on our menu without making changes to the data stored on our API.
This command allows us to retrieve a list of coffees from our API:
curl -X GET http://localhost:5000/coffees
Our command returns:
{ "coffees": [ { "id": 1, "name": "Espresso", "price": 1.95 }, { "id": 2, "name": "Latte", "price": 2.6 } ] }
As you can see, this resource allows us to retrieve a list of coffees. An API that uses the CRUD structure often allows you to retrieve individual records too. For instance, the following command allows us to retrieve data on one coffee item:
curl -X GET http://localhost:5000/coffees/1
Our code returns:
{ "coffees": [ { "id": 1, "name": "Espresso", "price": 1.95 } ] }
You can see that our command has returned the coffee whose id is equal to 1. No changes have been made to the data because GET requests can only retrieve data from an API.
Update
We also need to support updating records in our API. The corresponding HTTP method for updating a resource using CRUD is PUT.
If we want to change the price of our Latte to $2.75, we could use the following PUT request:
curl -X PUT http://localhost:5000/update/2 -d name=Latte -d price=2.75
Our code returns:
{ "coffees": [ { "id": 2, "name": "Latte", "price": 2.75 } ] }
This request changes the name and the price of the Latte record to the values we specified in our cURL command.
Delete
The final operation in the CRUD structure is Delete, whose corresponding HTTP method is DELETE (this one’s really easy to remember!)
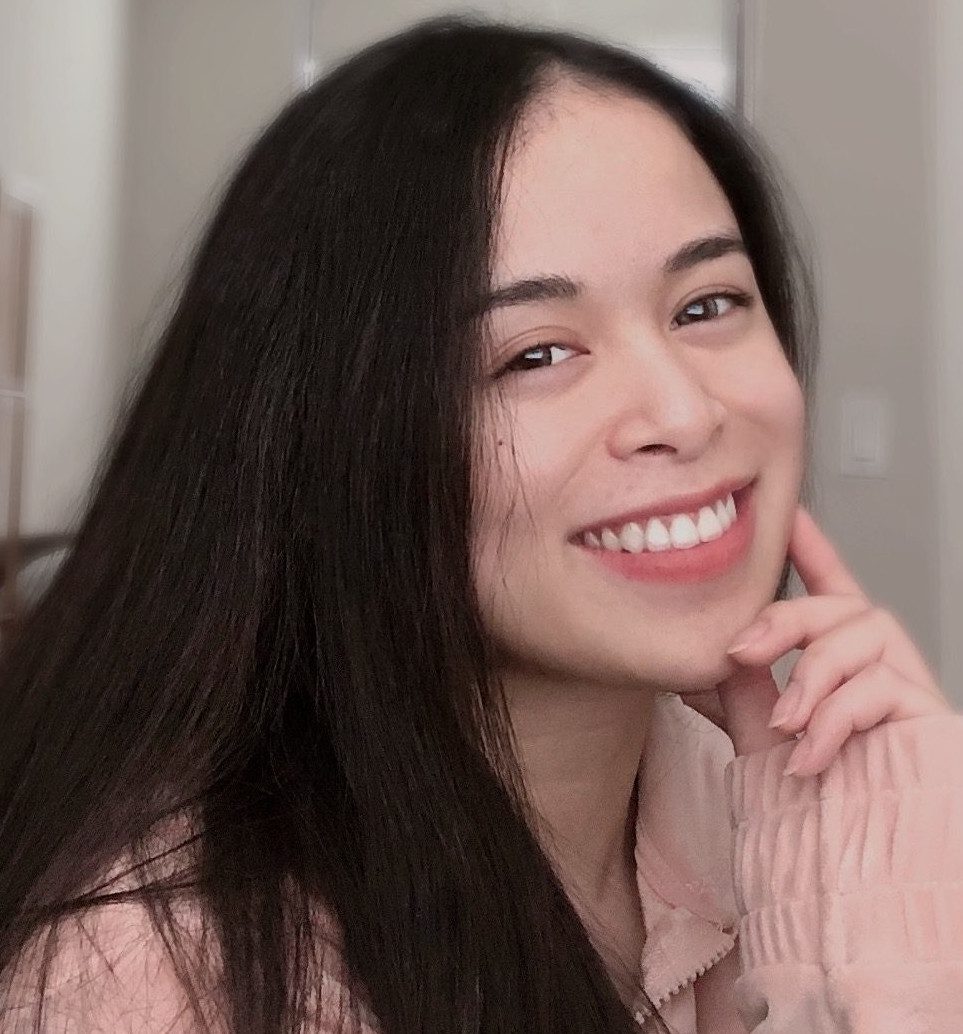
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose we ran out of espresso powder and we have to remove it from our menu. We could do so using the following request:
curl -X DELETE http://localhost:5000/delete/1
When we run this code, no response is returned. This is because the coffee we have specified is deleted from our server. If we run a GET request to see the list of coffees again, we see that the record with the value 1—the Espresso—has been removed.
CRUD Next Steps
Do you want to explore CRUD further? That’s great news!
You now know the basics. CRUD is a structure that states the four main operations that a web service should have. To advance your knowledge of CRUD, think of a hypothetical API you can design and make a list of routes.
For example, an API to track your favorite songs could have the following routes:
- /songs (GET)
- /songs/new (POST)
- /songs/:id (GET)
- /songs/:id (PUT)
- /songs/:id (DELETE)
Try making a list of routes for your own API, and write down their corresponding HTTP methods.
If you want to play around with the web requests we used in this tutorial, you can view the code we used to host the API on GitHub. Now you’re ready to start working with CRUD APIs like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.