If you decide to explore the question of which programming language is the most widely-used, a common answer you’ll come across is Java. Since its introduction by James Gosling of the Oracle corporation in 1996, Java has found use in applications ranging from machine learning to web development, and everything in between.
It even does pretty well when compared against another rockstar language: Python.
For this reason, anyone aspiring to a fantastic career in technology would do well to learn the basics of Java. An understanding of classes is an important step in this process.
In this piece, we’re going to explore what classes are and the vital role they play in Java programming.
What Is a Class?
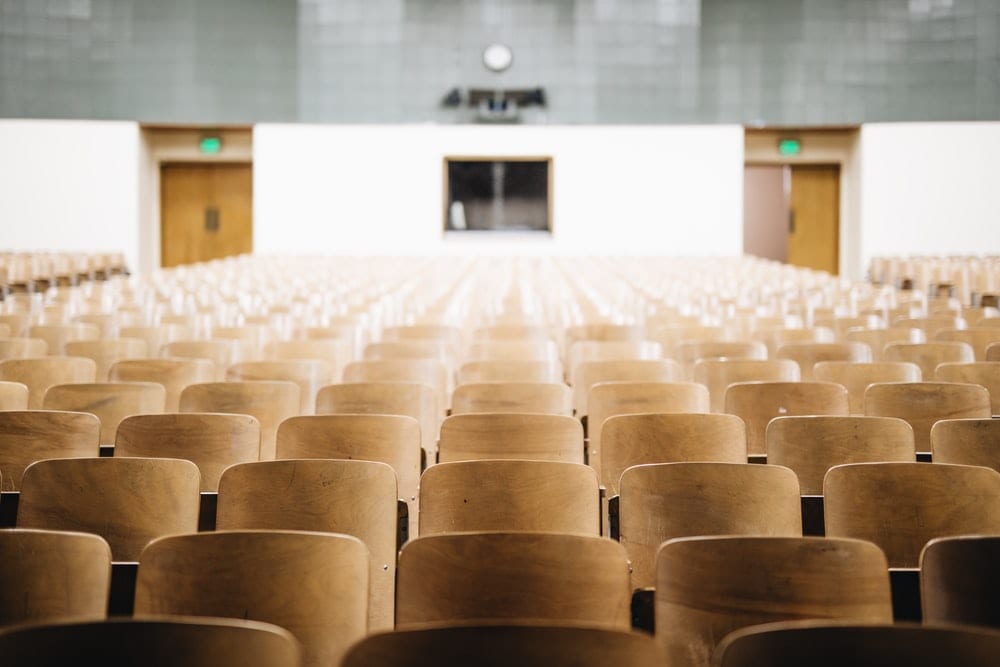
In object-oriented programming, classes can be thought of as modifiable templates which allow for the creation of an unlimited number of instances, each of which possesses the attributes and methods of the class definition.
In order to concretize this a little bit, let’s consider an example. Say I’ve defined a class called ‘Car’, with an attribute ‘color’ and a function ‘make_sound’. Now I can use this class definition to make instances of Car, which I’ll name ‘red_car’ and ‘blue_car’. By default, ‘red_car’ and ‘blue_car’ have access to the attribute and function defined in the ‘Car’ class.
The advantage here should be obvious. If I need to make a large number of objects which share certain features in common, it doesn’t make any sense to define each of them individually. By instead writing a class and using it to make instances, I can save myself a lot of effort.
But this isn’t all. Classes can inherit from one another. I could now define a ‘Motorcycle’ class which borrows the ‘make_sound’ method from the Car class, saving myself the necessity of building that function again.
Though this is a trivial example, it does illustrate the awesome power of abstraction in programming. The best coders don’t get to where they are by writing lots and lots of pointless code. They get there by being as parsimonious as they can be with their thinking and work.
Classes are an important part of the process of becoming a more efficient programmer.
How Do Classes Work In Java?
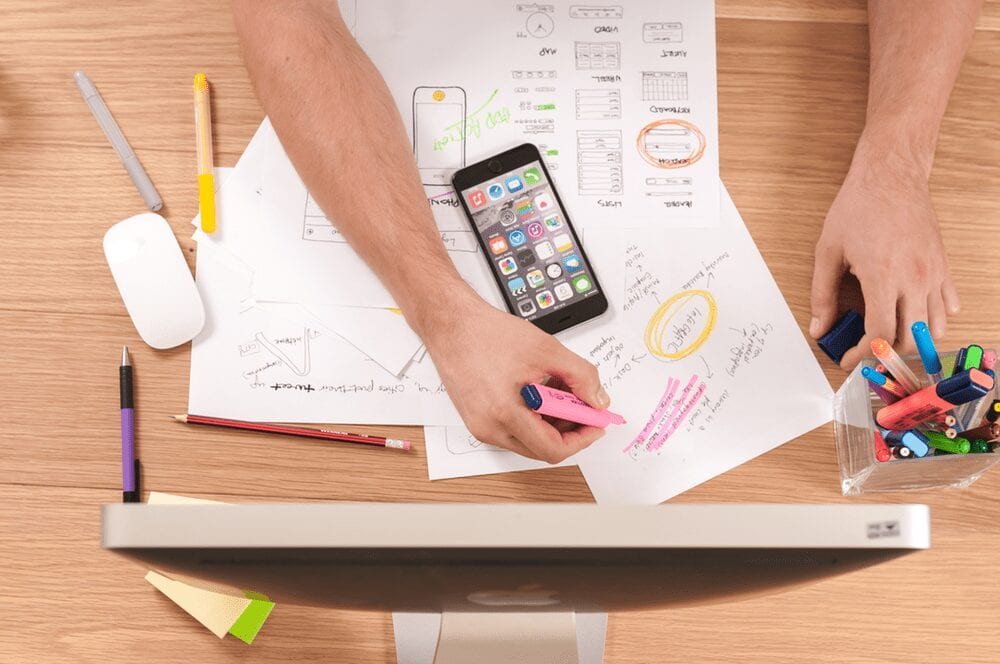
All of the comments made so far about classes apply to classes in Java as well. There are a handful of features which you’ll see in almost all Java class definitions:
- Modifiers: Java allows for the creation of a couple of different kinds of classes, each of which requires a special keyword to precede the class name. In order to make a private, protected, or public class we must add the appropriate word to the class definition, like so:
- public class Test
- private class Test
- protected class Test
- If no modifier is provided, Java creates a default class.
- Class name: Of course every class has to have a name, and by convention these are always capitalized.
- Body: This contains the actual code implementing the class.
In all the object-oriented programming languages I’ve seen, a class definition is only the crucial first step in actually using a class. The next step is to instantiate the class, which means creating an object based on the class template. In Java this tends to look like:
MyClass x = new MyClass(“argument_1″,”argument_2”);
The variable ‘x’ now has whatever properties, methods, and attributes we gave ‘MyClass’ in its definition. We call ‘x’ an instance of ‘MyClass’.
Learning More About Classes In Java
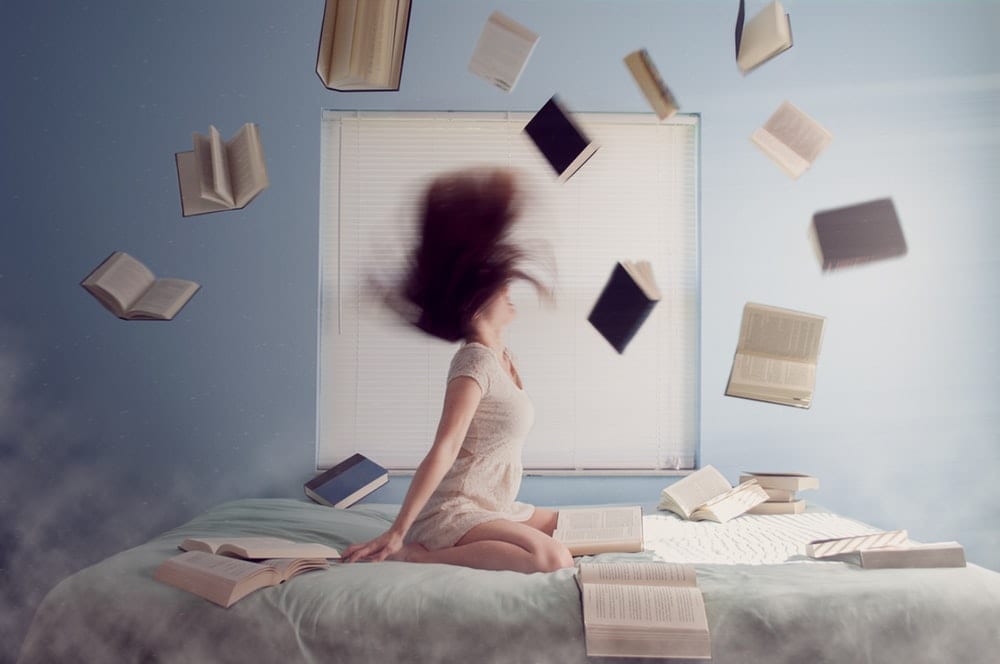
No doubt by now you’re burning with the desire to learn more about classes in Java. To that end, here are some fantastic tutorials to take your knowledge to the next level:
- Geeks for Geeks also has good tutorials, featuring simple explanations and lots of code snippets.
- It’s hard to go wrong consulting the official documentation for a language. It can take a while to be able to read the docs fluently, so don’t feel bad if these don’t help you a whole lot as a beginner.
- For visual learners, try this edureka video on Java classes, complete with diagrams and pithy explanations.
Classes are an ideal way to extend your range as a programmer and become more valuable. Hopefully this article has made them a little more accessible.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.