React has grown significantly in popularity over the last few years.
According to Stack Overflow’s 2019 Developer Survey, which studied the tools and development practices used by over 90,000 programmers, React.js was the second most popular web framework among respondents.
React has become so popular because it provides developers with the ability to build scalable applications quickly, a value that many companies around the world have recognized. As a result, questions about React are common in web development job interviews.
In this guide, we explore the top 15 React.js interview questions common in job interviews, and give you advice on how to answer them like an expert. We’ll focus on theoretical questions designed to test your knowledge of the React ecosystem.
#1: What is React JS?
During an interview, this may sound like a simple question to ask; you may even think it’s a trick. However, this is a very common question in technical job interviews precisely because it is so simple.
These are a few points you should cover in your answer:
- React is a front end JavaScript library for building web applications.
- React is declarative, which means you can design simple views for each state in a web application.
- React uses components, which allow you to create reusable featured on a web page.
#2: What is the difference between props and state?
Props and state are two essential concepts with which you need to be familiar to pass data through components.
State refers to the default data value in a React component. The state of a component can change over time, and can be manually changed by a developer. Props, on the other hand, describes how a React component is configured. Props do not change.
#3: What are React components?
Components are an essential feature of React—they are the building blocks of all React-based applications. You should know this and be able to describe what a component is.
Components allow developers to break down a user interface into smaller parts that can be reused. React renders each component on a web page only when it is needed, which allows applications to render quicker.
#4: When are keys used in React?
Keys are used in React to identify unique elements in the virtual DOM. By using keys, React can optimize rendering as the framework can recycle existing elements loaded to the DOM.
Suppose you have two instances of a component on a web page. Without keys, React would render both elements in the DOM, and would not re-order the DOM elements. But, when you use keys, React will re-order the DOM elements, which provides a number of performance benefits to an application.
#5: What are the most common approaches for styling a React component?
There are a couple of ways you can style a React component, and you should be able to describe how each works. Here are the main ways in which React components are styled:
- CSS Classes: React allows you to use traditional CSS class names to define styles for a component.
- Inline CSS: Inline CSS allows you to scope styles to a particular element on a web page.
- JavaScript Modules: JavaScript modules like styled-jsx and styled components are also used to style components.
- Preprocessors: Preprocessors like Sass and Less are another option to style a component.
#6: When should you use a class component instead of a functional component?
There are two types of components in React: class and functional.
Functional components are defined by a component with unchanging properties (props). Class components, on the other hand, are more complex components that allow you to manage a component’s state.
You should use class components over a functional component when you need to manage the state of a component, or use component lifecycle methods.
#7: What happens during the lifecycle of a React component?
The lifecycle of a React component are the events that occur from the creation of a component all the way to its destruction.
Three main parts make up the lifecycle of a React component: initialization, state updated, and destruction.
For instance, when you are creating a component, you may use methods like componentDidMount() to add event listeners to the component. This occurs after a component has been initialized. Then, you may use componentWillUnmount() to remote an event listener when the component is going to be removed from the web page.
#8: What is a virtual DOM? How does it work?
When a HTML document is rendered by a web browser, a representational tree is created called a Document Object Model (DOM). This gives the browser all the information it needs to render a web page successfully.
By default, a web page will use HTML to update its DOM to make changes to the page (such as updates to content that appear on a website). React, on the other hand, creates a virtual DOM, which is a copy of the web page’s “real” DOM.
This virtual DOM can be used to change how a web page appears based on an action taken by a user. Then, once a change is made in the virtual DOM, the virtual DOM will be compared to the “real” DOM and the “real” DOM will be updated with the relevant changes.
The virtual DOM offers a number of performance benefits for a React application, because the entire “real” DOM does not need to be reloaded every time a change is made on a site.
#9: What is JSX?
JSX is a tool that allows you to embed raw HTML templates inside JavaScript code.
JSX cannot be read by the browser, and so you need to use tools like webpack and Babel in order to translate it into browser-readable JavaScript.
JSX is used because it makes it easier to work with both HTML and JavaScript in a website. Developers do not need to use JSX, but it is a great way to reduce complexity in an application.
#10: What are stateless components?
Stateless components are a type of reusable component that render based solely on the properties passed to the component.
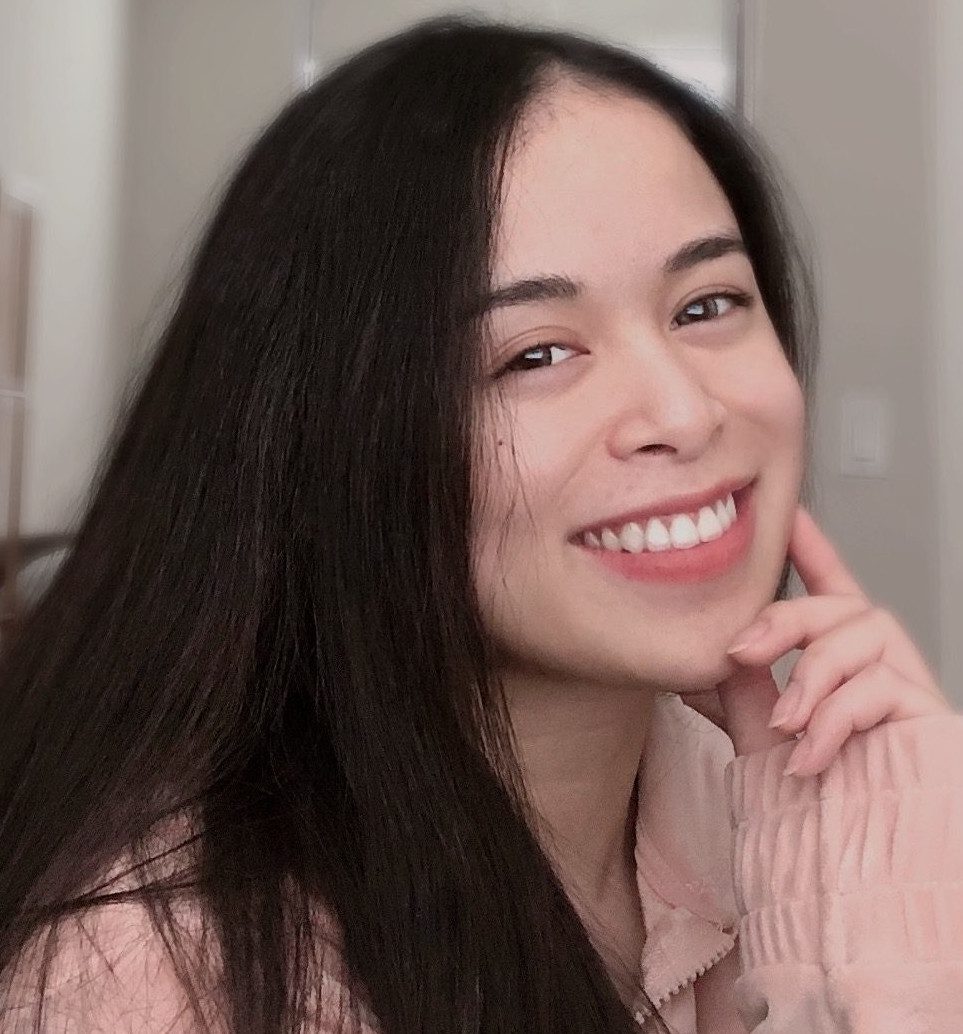
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
While regular components use both props and state, stateless components only use props. This means that a component will render based on the properties initially provided to it, and will not change over time.
#11: What tools can you use to make a React application more accessible?
There are two main types of tools you can use to help identify accessibility problems in an application. The first is a static analysis tool, such as ESLint, which allows you to analyze your code at a component level.
The second type of tool you may use is a browser accessibility tool like Google Lighthouse, which performs accessibility tests on the level of the application. These plugins allow you to discover larger problems with accessibility because they simulate how a user will interact with a given web application.
#12: What is the difference between React and React Native?
Both frameworks have the word “React” in the name, but they have different purposes.
React JS is a front end JavaScript library used to build seamless user interfaces. On the other hand, React Native is an open source framework that enables developers to use React to create mobile applications for iOS and Android. React Native is built on top of React, but is a separate framework.
#13: What is Redux? Why is it used?
Redux is a library that complements React and allows developers to build web applications that are easy to test. Redux can be used with React or any other JS user interface library, and comes with pre-packaged debugging tools.
The Redux library is often used because it adds more structure to your code, thereby making it easier to maintain your codebase. In addition, the developer tools offered by Redux can help with performance tracking such as events and state changes. This data can give you more insight into how your application works, and how it can be made more efficient.
#14: Why would you use React over other user interface frameworks like Ember?
During a job interview, the employer wants to know if you understand not just how React is used but why it is used in the first place.
These are a few of the reasons you could mention:
- React allows you to create reusable components that help reduce repetition in your code.
- Coding with HTML and JavaScript is made easier using JSX.
- The virtual DOM model makes web pages render and re-render faster.
- React has a supportive developer community with a wide range of open source packages to quickly get you started building a new application.
#15: Explain the purpose of two lifecycle methods commonly used in React.
React offers a wide range of methods that execute at different points in a component’s lifecycle. Here is a list of some of these methods and descriptions of how they work:
- componentDidMount(): Executes code on the client side after a component has rendered.
- componentDidUpdate(): Executes code as soon as the component’s DOM updates.
- componentWillMount(): Executes code before rendering begins.
- componentWillUpdate(): Executes code before rendering starts in the DOM.
There are other lifecycle methods offered by React, and you are free to describe any you are familiar with if asked this question.
Conclusion
An effective way to prepare for a technical job interview is to practice your answers to the most common questions.
Practicing the questions covered in this article should help you prepare for your next technical interview and increase your chances of success.
You can also ask a friend or a co-worker to quiz you. This is effective because the other person can give you feedback on your answers.
Keep in mind that in a job interview for a web developer position, you will likely be asked about more than just React. They could ask you about JavaScript or another framework that the company uses and lists on the job description. However, if you’re about to participate in a job interview for a position that requires React as a skill, the questions we’ve covered in this article will give you a good idea of what to expect.
Now all you have to do to nail your interview is to practice!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.