Manipulating text is a common operation in many programming languages, and Ruby is no exception. For example, you may want to capitalize someone’s name before it is printed on an invoice, or you may want to replace someone’s apartment number if they have moved.
In this guide, we break down the most useful string methods in Ruby. These include: finding out the length of a string, splitting strings into substrings, changing the case of a string, and finding and replacing text. By the end of this tutorial, you’ll be a master at working with Ruby strings.
Accessing String Characters
Strings are a data type that can contain letters, numbers, symbols, and whitespace characters. Here’s an example of a string in Ruby:
our_string = "I am a Ruby string!"
Each item in our string, like an array, has its own index number, starting with 0. We can use these index numbers to access individual items from our string. Here is a breakdown of the index values of the word Career
:
C | a | r | e | e | r |
0 | 1 | 2 | 3 | 4 | 5 |
Right now, though, our string is stored in one variable, as one value. But what if we wanted to get a few characters from our string? That’s where the slice()
method comes in.
The slice()
method allows us to retrieve a single character or a range of characters from our string. Here’s an example of slice()
in action:
"Career".slice(0)
Our code returns the following:
C
If we pass a single value, we’ll get the character at that index position in the string. If we want to get more than one character, we need to pass two values. Here’s an example of a slice()
function that gets the characters between the index values 1
and 3
from our string:
"Career".slice(1..3)
Our code returns:
are
If we wanted, we could also access our string using its negative index value. This allows us to count backwards from the end of our string, which is useful if we have a long string. The negative index values for the string Career
would be as follows:
C | a | r | e | e | r |
-6 | -5 | -4 | -3 | -2 | -1 |
So, if we wanted to get the last character in our string, we could use the following code:
"Career".slice(-1)
Our code would return:
r
In addition, if we want to turn our string into an array of characters, we can use the chars
method. Here’s an example of the chars method in action:
"Career".chars
Our code returns the following:
["C", "a", "r", "e", "e", "r"]
Now that we know the basics of how to retrieve characters from a string and how strings are indexed, we can move on to explore more string operations.
String Length
The length
method allows us to get the number of characters in a string. This method can be useful if you have a minimum or maximum character limit that you need to impose on a string. For example, you may limit the size of email addresses to 100 characters to prevent people from abusing a form.
Here’s an example of the length method in action:
name = "John Appleseed" print name.length
Our code returns 14
. Remember that every character, including spaces, will be included in our total.
Changing Cases
You may want to change the cases in a string with which you are working. For example, you may want all usernames to be in lowercase so that people cannot create two accounts with the same characters capitalized differently.
The functions upcase
and downcase
can be used to change the cases of a string to uppercase and lowercase, respectively. Here’s an example of the upcase function being used:
name = "John Appleseed" print name.upcase
Our code returns:
JOHN APPLESEED
We could also replace upcase
with downcase
in the above example to make our string appear in all lowercase letters.
You can also use the swapcase
method if you want to invert the cases in a string. Here’s an example of the swapcase method in action:
name = "John Appleseed" print name.swapcase
Our code returns the following:
jOHN aPPLESEED
There is also a function—capitalize
—that allows us to change the first letter of a string to a capital letter. This may be useful if we want every name in our program to appear with the first letter capitalized, even if a user enters a lowercase character at the start of their name.
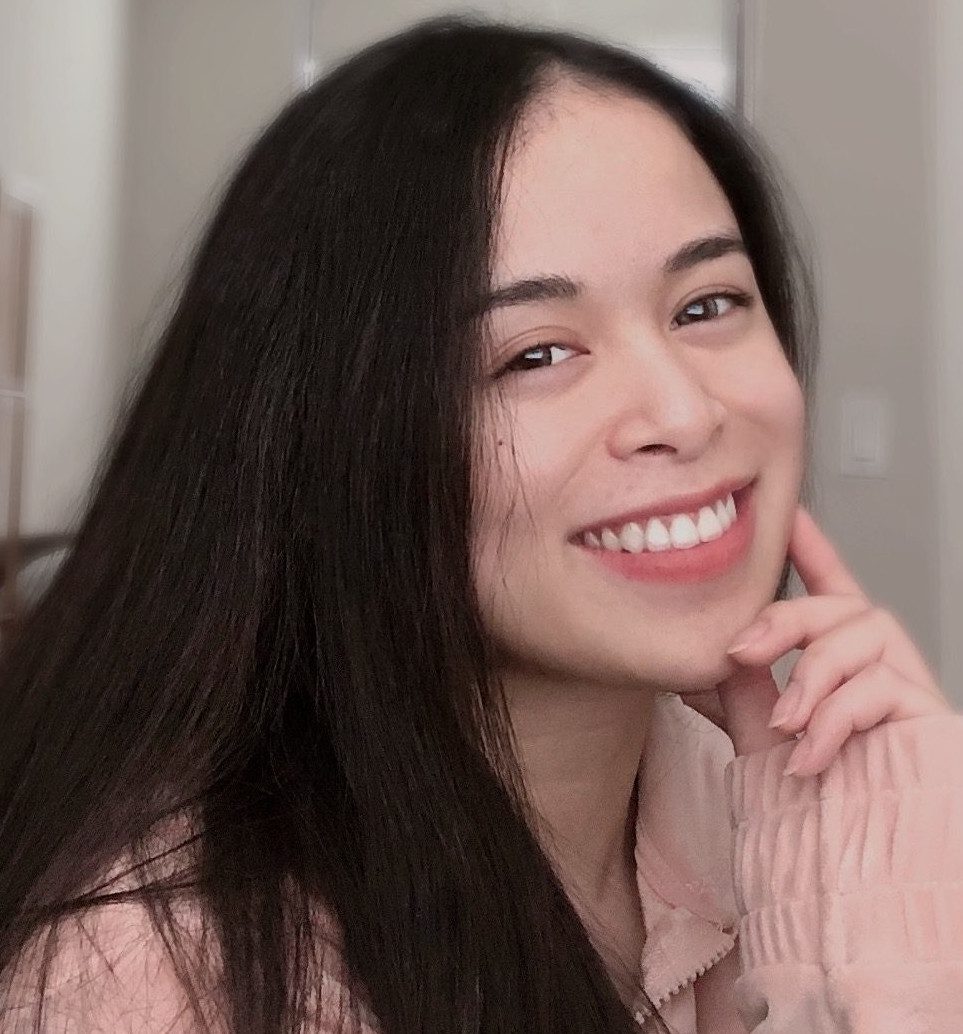
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s an example of the capitalize function in action:
"john".capitalize
Our code returns the following:
John
However, the capitalize function only capitalizes the first character in our string, so if we were to include a surname, it would not be capitalized.
These functions do not modify our strings; instead, they create new ones. If you want to modify the original string, you can use the !
operator. Here’s an example of a program that will convert all letters in a name to uppercase:
name = "Erica" name = name.upcase! print name
Our code returns the following:
ERICA
Finding Text
There may be times when you need to check whether a string contains a substring. We can use the include?()
method to do this. The method returns true
if the substring can be found within the string, and false
if it cannot.
Here’s an example of the include?()
method in action:
"John Appleseed".include?("Apple")
Our code returns true
, because our string contains Apple
.
On the other hand, if we want to get the index value of the character at which a certain substring starts, we can use the index()
function, like so:
"John Appleseed".index("Apple")
Our code returns 5
, which is the index value of the character at which our substring begins. That said, the index function only returns the first occurance of a value in a string, so if we were looking for multiple occurances of a value, we would not be able to use the index function.
In addition, if we are looking to find out whether our text starts with a particular string, we can use the start_with()
method. The start_with()
method accepts one or more strings and will return true
if any of those strings can be found in the larger string.
Here’s an example of the start_with()
method:
name = "John Appleseed" name.start_with("John", "Graham")
Our code returns true
, because our name starts with John
, which is one of the values we specified.
Replacing Text
If you want to replace text in Ruby, you can do so using the sub()
and gsub()
methods. Let’s say that we have a sentence and we want to replace the word quick
with slow
. We could use the sub()
function to do this, like so:
text = "The quick brown fox. The quick white bear." print text.sub("quick", "slow")
Our program returns:
The slow brown fox. The quick white bear.
If we wanted to change every instance of the word quick
to slow
, then we would need to use gsub()
, like so:
text = "The quick brown fox. The quick white bear." print text.gsub("quick", "slow")
Our code returns:
The slow brown fox. The slow white bear.
As you can see, our program has replaced every instance of the word quick
with slow
.
It’s worth noting that sub()
and gsub()
are case sensitive, so make sure you employ the use of the right cases in your substrings.
Like in other string functions, sub()
and gsub()
do not replace a string unless you use the !
flag. Here’s an example of a text replace function that will edit our original string:
text = "The quick brown fox. The quick white bear." text = text.gsub!("quick", "slow") print text
Our code returns:
The slow brown fox. The slow white bear.
Our original text
variable has been replaced with the updated one.
For advanced Ruby coders, you may want to consider using regex
(regular expressions) to conduct a search and replace function. This gives us more control over our replace function. Here’s an example of a gsub that will replace the letters t
and b
with X
:
text = "The quick brown fox. The quick white bear." text = text.gsub /[tb]/, "X" print text
Our code returns:
The quick Xrown fox. The quick whiXe Xear.
If you’re looking to find out more about Regex and to experiment with different operations, check out RegExr.
Padding and Stripping
If you want to format text, you may find yourself in a situation where you want spaces between your strings. Or perhaps you want to remove extra spaces from a string so that you can format your string a certain way.
There are a number of built-in functions in Ruby that make it easy to format strings. Let’s break these down.
Center and Adjust
If you want a string to be centered and surrounded by spaces, you can use the center()
function. Here’s an example of the center()
function in action:
"John".center(20)
Our code returns the following:
" John "
The center()
method works with two parameters: (1) the number of spaces you want to add to your string (20 in the below example), and (2) the character you want to use instead of a space if you do not want to use a space. Here’s an example of center()
being used with both parameters:
"John".center(20, "*")
Our code returns the following:
********John********
You can also use the ljust()
and rjust()
functions if you want to add spaces to the left or right of a string, respectively. Here’s an example of the ljust()
function in action:
"John".ljust(20, "*")
Our code returns:
John****************
Strip and Chomp
The center()
, ljust()
, and rjust()
functions can be used to add spaces or other characters to a string. But what if you want to remove characters from a string? That’s where the strip
, chop
and chomp()
functions come in.
Strip works in the same way as the center()
, ljust()
, and rjust()
functions do. The strip
, lstrip
, and rstrip
functions remove all spaces, spaces on the left, and spaces on the right in a string, respectively. Here’s an example of these in action:
" John ".strip # "John" "John ".lstrip # "John" " John".rstrip # "John"
In addition, we can use chop to remove the last character in a string. Here’s an example of chop in action:
"Pauline".chop
Our code returns: Paulin
.
We can also use chomp
to remove multiple characters from the end of a string. Here’s an example of chomp being used to remove the last two characters from our string:
"Pauline".chomp("ne")
Our code returns: Pauli
.
If you don’t specify the characters you want to remove with chomp()
, it will remove a newline character (“\n
”). If there are no newline characters in your string, chomp()
will return the original string.
Conclusion
String methods allow us to manipulate a string according to our needs. In this tutorial, we explored how to manipulate strings using several built-in Ruby functions. We discussed how strings are made in Ruby, how to access strings using their index values, how to replace text, how to find text within a string, how to convert cases in a string, and other functions.
Now you’re an expert at working with Ruby string methods!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.