As developers, our goal is to write succinct and effective code. Loops are one way to cut down on unnecessary code. In Ruby, there are several types of loops including `while`, `for`, `do..while`, and `until` loops. In this article, we’ll discuss how to implement a `for` loop while writing code in Ruby.
What is a Loop?
A loop is an instruction to repeat an action until a condition is met. Let’s look at a real-life analogy:
At a New Year’s Eve party, guests count down to midnight and shout, “Happy New Year!” In this situation, the guests are repeating an action (counting out loud) until a condition is met (the clock strikes midnight.) The guests don’t continue counting down past, “One!” and start shouting negative numbers, because the condition has been met.
For Loops
There are different loops that work better in different situations, and loops vary between programming languages. In Ruby, the `for` loop works best when the number of times the action will be completed is definite.
The New Year’s Eve analogy continues to work here: the guests will shout from the number ten to the number one, before shouting, “Happy New Year.” The number of times the action will repeat is limited. Whereas in `while` loops, the action will continue as long as the conditional is true, and will stop when the conditional is false (or continue as an infinite loop.) You can read more about the Ruby While Loop” here.
The syntax of a `for` loop is:
for element in collection #execute code end
If we take a look at the code, it says: for each element in this collection, apply this change to each element of the code. When using a `for` loop, the keywords you need to include are for and in; the rest is interchangeable based on what your code accomplishes.
Similarity to the Each Method
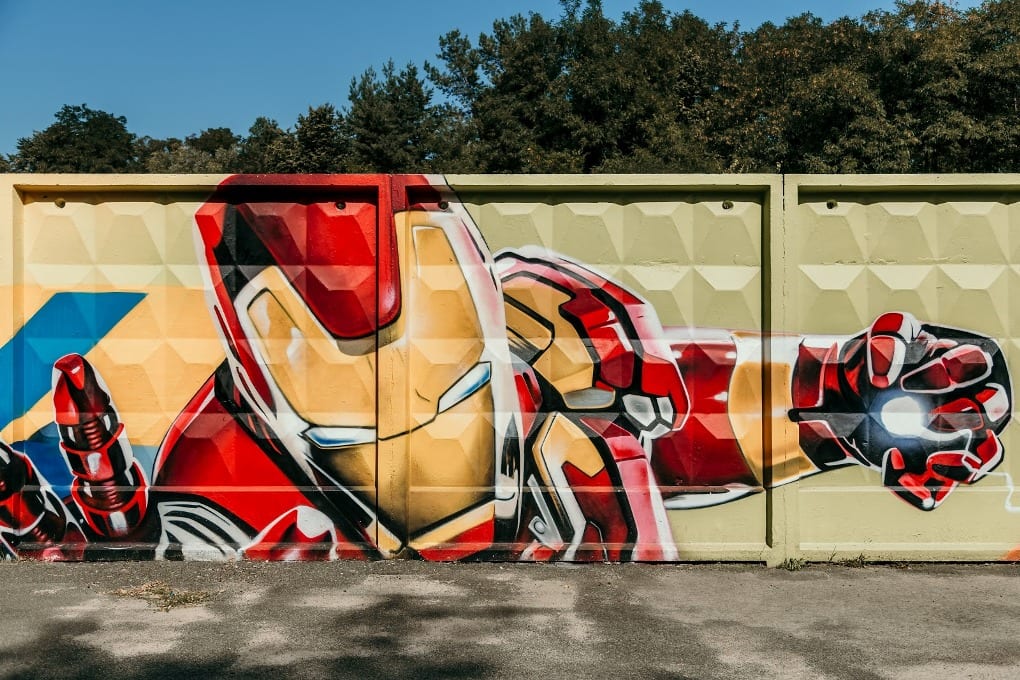
Here’s a second example of using a `for` loop with an array.
avengers = ["Iron Man", "Captain America", "Hulk", "Thor", "Black Widow", "Hawkeye"] for member in avengers puts "Good morning, I'm #{member}." end
This code uses a `for` loop to iterate through each member in the avengers array and output a string with a greeting and the member information—in this case, their name.
The output of the code is:
Good morning, I'm Iron Man. Good morning, I'm Captain America. Good morning, I'm Hulk. Good morning, I'm Thor. Good morning, I'm Black Widow. Good morning, I'm Hawkeye.
You might notice using a `for` loop with Ruby is similar to using the .each method. Here is a similar code using the .each method with a similar output.
avengers = ["Iron Man", "Captain America", "Hulk", "Thor", "Black Widow", "Hawkeye"] avengers.each do |member| puts "Good afternoon, I'm #{member}." end
The output of the code is:
Good afternoon, I'm Iron Man. Good afternoon, I'm Captain America. Good afternoon, I'm Hulk. Good afternoon, I'm Thor. Good afternoon, I'm Black Widow. Good afternoon, I'm Hawkeye.
Conclusion
In this article, we learned about `for` loops in Ruby, which are the preferred loop when the number of times the action will repeat is set at the beginning of the loop. We also learned how the `for` loop is similar to the .each method.
If you are curious about what you can do using Ruby, check out our article, ”What Is Ruby Code Used For?”
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.