If you’ve looked into different frontend frameworks, the phrase “React Redux” has probably come up. What is React/Redux? Are they the same thing?
Redux is a JavaScript library for managing an application’s state. It works alongside another JavaScript library, such as React, to contain the application’s state.
Say you’re building an e-commerce app. Most likely, the cart component tracks the items in the app’s state. Redux manages the cart component’s state as the single source of truth.
What single source of truth means is Redux keeps track of the individual React component’s states. This is helpful because some apps are complex and searching inside individual React components for their state can be overwhelming.
What is Redux?
In the introduction, we established that although you’ll hear the words “React” and “Redux” together, they are separate JavaScript libraries. Redux is designed to work alongside other libraries, such as React. React has its own state management system, but as with our e-commerce example app, state management can become confusing.
When that much data is changing over time, Redux is a helpful tool to be able to access the data from one location. That location, referred to as a store, organizes the application’s state and makes it available to individual components.
Redux comes with its own methods to access state. The connect() method allows the developer to connect a React component to the Redux store. With a few lines of code, the component now has access to the state of the entire app.
Now that we have a better idea of what Redux is, let’s see how we can use Redux.
What is Redux used for?
To better understand the benefits of Redux, let’s take a closer look at an app’s state. In React, the state object stores the components’ property values. These values are then rendered on the user’s screen. If the state changes, the component re-renders with the new values.
This means each React class component can manage its own state. Thinking back to our e-commerce app, we will have a lot of components and state to track. Redux makes managing state much simpler.
Redux solves the issue of tracking each individual component’s state by creating a store in which all state resides. A developer can access the entire application’s state through the Redux store. Redux uses reducers to add and remove data from the store. A handy combineReducers() method accesses all reducers in the store. The connect() method grants all components access to the Redux store.
Above outlines the basic flow of data without diving too deep into specifics. If you want to take a deeper dive into Redux, let’s talk about learning Redux.
Learning Redux
When shopping online, there are many things happening behind the scenes from adding an item to a shopping cart and checking out. Most sites have the ability to add items, remove items, clear whole orders, and check out.
Who keeps track of all of this? As discussed above, state refers to the application’s changing data. An e-commerce site will have a lot of changing data from so many users buying from the site. Redux keeps track of the entire application’s state in a single place known as a store.
How Long Does it Take to Learn Redux?
Before deciding to learn Redux, ask yourself how well you know React or a similar library? If your React knowledge is based in a solid understanding of JavaScript fundamentals then go for it!
Learning Redux can be done in a matter of hours, but like all new things, it will take some time to get used to. Actions like sending data to the Redux store with multiple reducers will take a little practice.
Redux can be learned and implemented quickly inside a few days with daily practice.
How to Learn Redux: Step-by-Step
Here are some steps to consider before taking on Redux:
- Learn JavaScript fundamentals. React is a JavaScript library so it makes sense to be fluent in JavaScript.
- Familiarize yourself with JSX. This is how you write components in React. JSX combines HTML and JavaScript in its syntax.
- Understand React basics. Knowing the difference between state and props, React data flow, class components vs functional components will prove essential in quickly implementing Redux.
- Knowing how much state will change in your application. If there is not much state change, use React’s built-in state management. Otherwise, if your application’s state changes frequently across multiple components, using Redux will help manage the state.
- Build a few small React projects and practice hooking Redux into them. Practice makes progress and why not beef up your portfolio while learning Redux?
Once the basics of frontend web development are mastered, learning Redux is quick. It may take a few tries to get everything working together properly, but it is a painless process.
The Best Redux Courses and Training
As online learning gains popularity, the quality of resources to learn web development appreciates as well. Learning Redux and implementing it into your React web app is meant to be quick, so you can get back to building your app. Below is a list of the top Redux courses for beginner and intermediate developers to learn Redux.
Online Redux Courses
Modern React with Redux
- Provider: Udemy
- Instructor: Stephen Grider
- Cost: $119
- Audience: Intermediate
Udemy has some fantastic instructors with in-depth lessons. Stephen Grider is a React veteran with a skill for clearly explaining the React process. This course is continually updated to be the most modern implementation of Redux in a React app available.
Grider guides you in building a single page app in 52 hours. With a clear teaching style, you’ll understand the lessons and walk away from this course as a more advanced developer. He not only covers React/Redux basics, but talks about Babel, NPM, Webpack, and throws in a little about React hooks!
UI Dev Redux
- Provider: UI Dev
- Cost: $350 per year ($40 monthly)
- Audience: Intermediate
The Redux course at UI Dev takes a project based approach. Consisting of several small projects per lesson, it aims to teach through actively coding. The Redux course – packed with lessons, quizzes, and growing projects – progresses from fundamentals to real world implementation.
UI Dev continually updates their courses, so they are always current. The Redux course clocks in at 31 topics, 357 minutes of video, 1,182 words of text, six quizzes, and three projects. Here, you can learn Redux in a couple of days.
React and Redux Masterclass
- Provider: Udemy
- Cost: Free
- Audience: Intermediate
The Redux masterclass on Udemy assumes you have prior knowledge in modern JavaScript and at least some familiarity with React. Taking just two hours and six minutes to complete, this may be the most accessible Redux courses.
This course covers both React and Redux basics, it will get beginning React developers up to speed quickly.
Online Resources
Getting Started with Redux
Redux creator Dan Abramov shares a full video series on how to get started with Redux. He uses an example app of a to-do list to clearly demonstrate Redux capabilities.
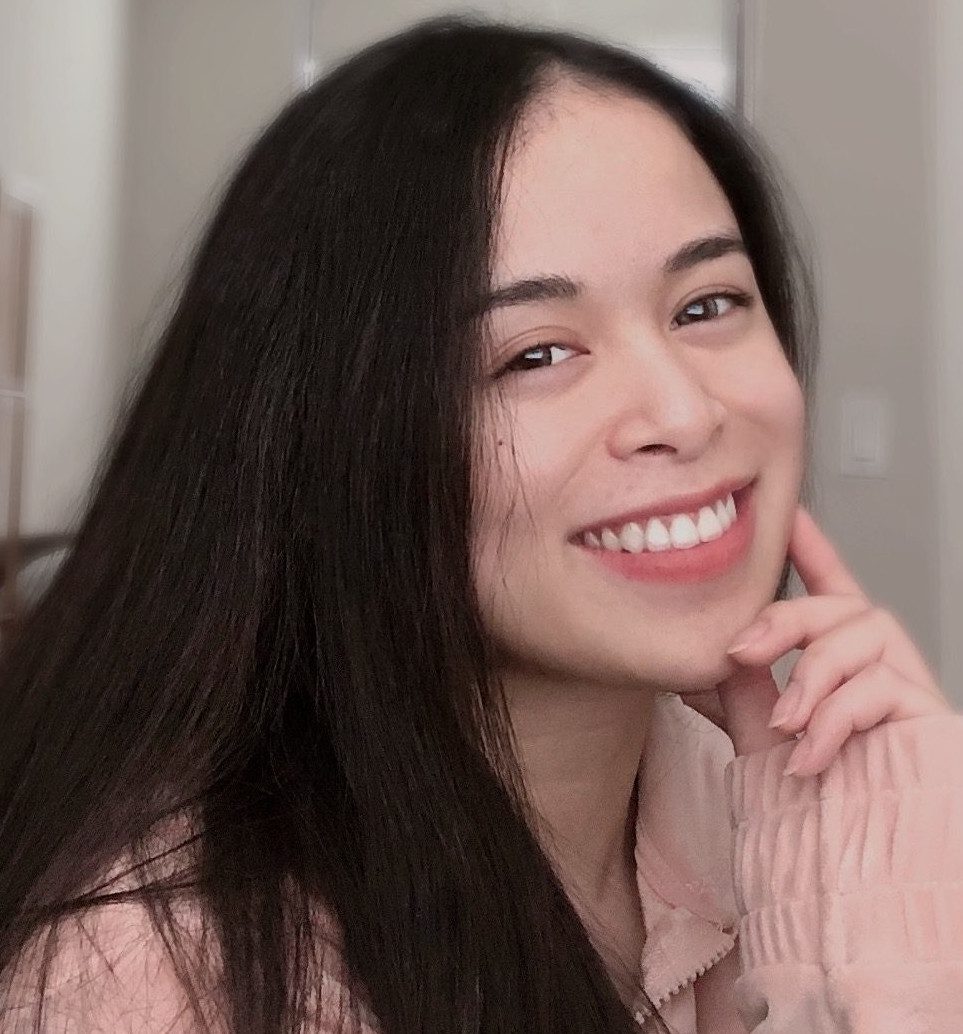
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Learning from the creator himself is a great way to understand the thought process behind how Redux functions.
Official Redux Tutorial
One of the great things about Redux is how the official documentation is written. It includes a getting started guide as well as an official tutorial section to help you get Redux up and running quickly.
It does, however, assume you have prior knowledge of modern JavaScript (ES6 syntax, AJAX requests) and a working understanding of React.
React Redux Tutorial for Beginners: A 2020 Guide
A Redux tutorial for complete beginners by Valentino Gagliardi outlines the basics from “What is Redux?” to “What problem does Redux solve?” The guide is split up into several easy to read sections. Reading this guide from start to finish will take about an hour or so, but you’ll be on your way to understanding Redux.
Should I learn Redux?
Learning Redux as an intermediate developer with fluency in modern JavaScript and at least some familiarity with React is a relatively quick process. Something to consider is the application you’re building. Is it a large, complex app? Or is it a compact React app?
If your React app has few components and not a lot of data changes, then use React’s built-in state management. Sometimes adding libraries can be redundant and overly complicate your app.
If the app is a complex app with several data changes happening throughout, then Redux is a fantastic tool to learn. It will keep these state changes organized and accessible through its singular store.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.