Have you ever heard of state
in the JavaScript library React but don’t know how to use it? State refers to “how each object on a web page appears.” The concept is simple — it’s a collection of data related to the component’s current state, which can be modified by changing the data that describes it. Each time the data changes, it re-renders on the computer screen.
The most difficult part about state is knowing how and when to use it. But don’t worry because this tutorial covers how state is used.
To give you a brief overview, to set the initial state, you use this.state
in a JavaScript constructor. To update the state, use the setState
method. We will talk more about state and some ways to use state in this tutorial.
What is React State?
React state is an object with a set of properties you designate for a component, and that contributes to how the component is rendered. A component that has state
is referred to as stateful. You generally use it if a component needs to keep track of information related to it between renderings.
An example would be a “like” button component that needs to track the number of times it’s clicked on. This has to be re-rendered on the screen each time the like-button is hit to show the updated number of likes. And for that, we have to make sure the like-button component keeps track of the information, instead of just repeatedly rendering the information we coded with it. After all, you don’t want to lose those likes.
A component’s state data can be accessed via this.state
and changed by using the .setState
method. State is private as it can only be changed by the component that contains the state.
Many people confuse state with props, another react object, but there are major differences between the two. One of them is that state is dynamic, meaning data it holds can change and thereby affect the component’s behavior. Props, on the other hand, are immutable, which means that “its state cannot change after it is constructed.”
React State Syntax
State is initially defined in the function named constructor. This is because even though state can change, we want to make sure there’s an initial value present. The general format for defining state is:
this.state={ attribute:"value");
For example, if you are making a timer component, you must define the starting-off state as this.state={seconds:0}. You know that a timer will change its state every second, but it has to start its count somewhere. The component you are coding in is referred to as “this”. This statement changes the component’s state by setting one of its attributes (“seconds”) to be equal to the value you choose to define (“0” in this case).
Here is a sample index.js file for a component named “Person”. We will add four values — name, occupation, hair, and age — to the state object in the component:
//basic React import statements
import React from 'react'; import ReactDOM from 'react-dom';
//Component we named “Person”, with state properties defined in the constructor:
class Person extends React.Component { constructor(props) { super(props); this.state = { name: "Tom Hiddleston", occupation: "Actor", hair: "black", age: 40 }; }
//A render function that defines what will be rendered on the screen:
render() { return ( <div> <h1> Person </h1> </div> ); } } ReactDOM.render(<Play />, document.getElementById('root'));
The last line is saying that the code will go through your HTML document and look for the element that has the id “root”, and render what you have instructed it to do in the render()
method above. This is why you must make sure to add the following inside of the document body of your index.html file:
<div id="root"> </div>
This HTML tag has the ID attribute set to “root”. This is where everything you asked the component to render will go.
Referring to the State Object
You can refer to the state object by writing this.state.attributeName. Doing this will replace “attributeName” with the attributes defined.
Here is an example using the properties we defined earlier to make up the state of our “Person” component. We will use the {this.state.attribute} format to call on the attribute value for the component we are writing our code in.
In this case, we will copy the following method into our “Person” component, which will be what “this” refers to. The screen will show the value belonging to the attribute we wrote. Replace the render()
statement from our “Person” component in the last example with this one:
render() { return ( <div> <h1> {this.state.name} </h1> <p> {this.state.name} is an {this.state.occupation} and is {this.state.age} years old. His hair is currently {this.state.hair}. </p> </div> ); }
The screen will render a heading with the value of the attribute “name”, which is Tom Hiddleston. Under that will be a paragraph stating, “Tom Hiddleston is an Actor and is 40 years old. His hair is currently black.”
The .setState() Method
Use this.setState() to update a state object’s values. This method takes one argument, which will be a new attribute and its value: {attribute: “new value” }. Methods are used to do this because React will call the render method behind the scenes so your new content is always rendering.
Here’s an example using our “Person” component from earlier. Add the following code to the “Person” component code we saw at the beginning of the article.
- Add an occupation changing function to “Person” in the class’ body. This function will call the
.setState
method. The attribute written here is the occupation attribute and we write the value we want to change it to. Recall from the “Person” component code that the occupation is currently “actor”.
occupation = () =>{this.setState({occupation :”model”});} - Insert the following button code inside of the
.render()
method’s main <div>. It will add a button that calls the occupation function when a user clicks it, hence the “onClick” attribute. The button will have “Occupation Change!” written inside it.
<button type=”button” onClick={this.occupation}>Occupation Change!</button>
And there you have it! Now, you can see a button that calls the function from step one. The method contains a .setState()
method wherein the occupation attribute is set to have a new value, “model”.
Multiple .setState()
methods are not processed in order, so this may present conflicts you should be aware of — read more about those here. This is important because when your code grows bigger and there are more lines and more React states written, you have to think of how they will work together. This can be hard to do, especially with React’s asynchronous nature, where you can’t predict in what order your setState methods are going to be processed.
Do You Have to Use State?
The simple answer is no, you don’t. There are two main types of React components: functional components and class components. Components without state are functional components. By default, there is no State in a component — you have to create it yourself.
Without state, your app won’t be very interactive, however. Even if you do opt for implementing state, it is recommended that you aren’t overzealous in your use of it. This is because excessively using State in components can make it hard to foresee possible conflicts due to the complexity involved.
Wrapping Up
State and Props are two important objects to keep in mind when using React. State keeps track of a component’s current state as it changes depending on the update to the data that defines a state. Props, on the other hand, don’t change. A simple rule of thumb regarding which to use is to ask “Will this component’s attributes be changed at any point?”. If the answer is “yes”, then you want to use State category, since Props is immutable.
Before diving fully into React, make sure you’re familiar with the JavaScript ES6 syntax, since React makes use of many of its features, such as arrow functions, classes, and variable types. Also, there were changes to React regarding using state and other features without having to write a class in the recent React update, React Hooks.
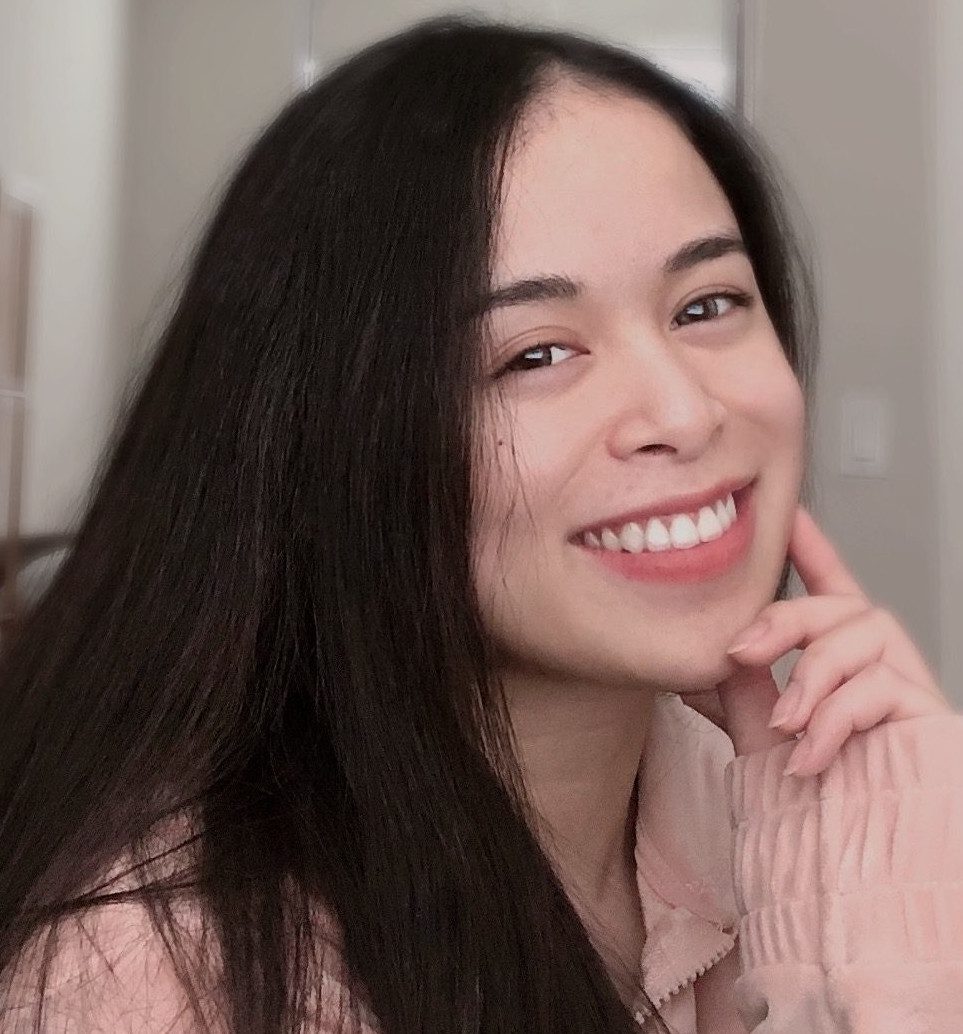
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.