Have you ever seen the word “props” in React, perhaps passed into a function? Ever wondered what it means? Due to the inherent importance of this object in React applications, it’s important you understand why and how props and state are used.
If you are confused about props you are not alone! Many of us are just used to seeing props passed in at certain points and go along with it almost subconsciously. Hopefully, this basic tutorial will help demystify why and how React props are used.
What is React Props?
React props (“properties”), are used as a way to pass data from one component (a parent component) to another (a child component). Props are immutable, which means that you can’t change props passed to a component from within the component. Components receive data from other components with props. Props received from another component can’t be modified.
Props Syntax
Here is a basic index.js file example showing props being passed in as an argument:
//Basic React import statements
import React from 'react'; import ReactDOM from 'react-dom';
//Movie component with a render function
class Movie extends React.Component { render() { return <h2>Tonight we'll watch {this.props.title} !</h2> } }
Notice that we have not assigned the “title” property a value yet. We will do that in this next line:
//constant variable name “myelement”
const myelement = <Movie title="Casablanca" />;
Here we ask our “Movie” component to display (by use of <… />) with the value “Casablanca” assigned to the property named “title”.
//React statement to render “myelement” to the HTML element with the id named “root”.
ReactDOM.render(myelement, document.getElementById('root'));
“Tonight we’ll watch Casablanca !” will be displayed.
Sending Props to the Constructor and to super()
You may have seen props passed to constructor functions as well as into super() and been super (pun intended) confused about why. Here is a simplified example:
class Computer extends React.Component{ constructor(props){ super(props); //we passed in props to super() console.log(props); console.log(this.props); }
Why do we call super and why pass props to it or the constructor? Super refers to the parent class constructor. If you have had significant programming experience, you’ve dealt with constructors before. Our “Computer” class component extends from (in other words, it is constructed using) the basic React component (as you can see in the first line).
So above we are initializing the React component’s this.props. If we had not passed in props to super(), the last console.log statement would return as undefined. Basically, inside the constructor, you can’t use “this” unless you use super(props). If that last console.log statement was written before super(props) it would not work.
Even if you do not call super(), you’ll still be able to access this.props in other parts of your component. This is because behind the scenes, React assigns this.props after the constructor runs. The issue is if you wanted to call a method from within the constructor itself. Coders include super(props) for code maintainability. Your code might change in the future and you want to be prepared if it does. Let’s see the following code wherein we have a “Person” class component with a property (“name”):
class Friend{ constructor(name){ this.name=name; } }
Let’s add a class “GoodFriend” which extends Friend:
class GoodFriend extends Friend{ constructor(name){ this.complimentYou(); //you shouldn't do this before super()! super(name); }
//the definition to the “complimentYou” function we called above:
complimentYou() { alert('You look nice today'); // an alert that shows up on your screen } }
The above works okay. However, if you later wanted to actually use name and add
alert('Compliment brought to you by your friend '+ this.name);
to the complimentYou() function, you’d encounter a problem: the function is called in the constructor before we used super(name) to set up the parent’s (“Friend”) this.name, so it would not work. This is why people call super() and pass props!
Sending Props into a Component
Here is a way you can send props into a component:
First, define the attribute/value pair you want to send into the component:
const elementToAdd = <Band name="Velvet Underground" />;
Then pass along the argument you defined in the first step to the component as a props object:
class Band extends React.Component { render(){ return <h1> The band name is {this.props.name}. </h1>; } } ReactDOM.render(elementToAdd, document.getElementById('root'));
Passing Data to Another Component with Props
You can send data through props into another component. A reason to do this is code cleanliness. React is all about breaking things up into reusable, manageable sections after all. Let’s look at this example wherein we pass a name value.
The “Band” component’s render() function returns the value of the property named “name” (we will give it a value in the next component!) along with the string “from New York City!”:
class Band extends React.Component { render() { return <h3> {this.props.name} from New York City! </h3>; } }
The “Venue” component’s render() function returns a heading stating, “Band Playing Today:”. Under that we see our “Band” component we wrote above being called to be rendered; “name” is assigned the value of “Velvet Underground”:
class Venue extends React.Component { render() { return ( <div> <h1>Band Playing Today:</h1> <Band name="Velvet Underground" /> </div> ); } } ReactDOM.render(<Venue />, document.getElementById('root'));
Now a heading saying “Band Playing Today:” will be displayed along with “Velvet Underground from New York City!” In the last line, we only called <Venue /> component to be rendered since “venue” will already display our “band” component.
Similarly, you can pass other forms of data.
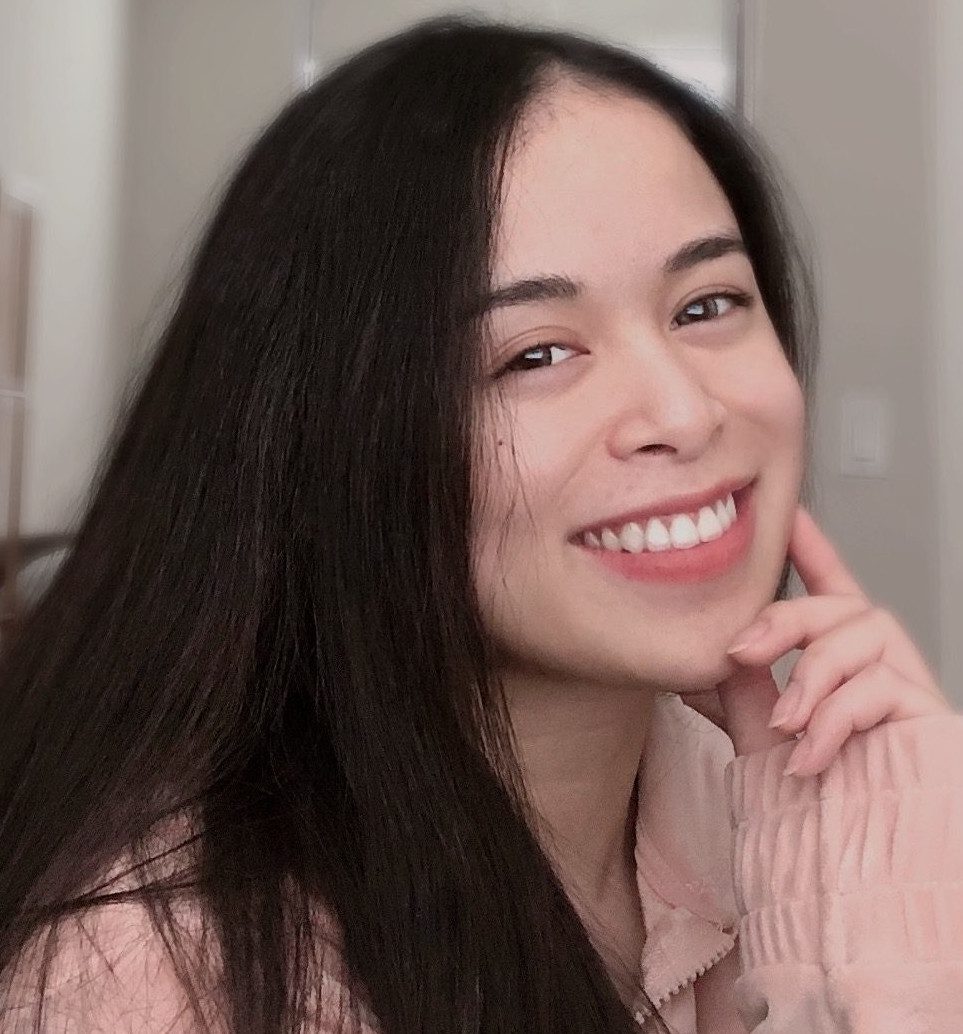
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Sudoku Board Section Example with Props
Imagine you have a sudoku game board you want to build with React. It’s made up of nine, three-by-three square sections. Let’s focus on one of those three-by-three square sections. It’s basically a square board made of nine smaller squares, and each square has a number.
First, you create a “Square” class with a render() method that would return a button. In your CSS file, you make it into a square shape using the className we tied to the button so you can refer to it. Notice also that the button content (what’s displayed inside the button) is currently assigned to {this.props.value}, but “value” is not yet defined:
class Square extends React.Component { render() { return ( <button className="smallSquare"> {this.props.value} </button> ); } }
Now that we have the small squares taken care of, let’s think of our sudoku board. We want to arrange these squares into three-by-three rows, and we want to fill these squares with numbers. Let’s make our board component!
We add the component heading and a function we’ll name “showSquare”. Look at the function’s return statement. It calls our Square component and sets its value to “i” (remember we did not assign a value to the “value” property in our Square component).
class Board extends React.Component { showSquare(i) { return <Square value={i} />; }
Now that we have the function that will use the square class and input a value “i”, let’s use that in a render function to show our desired three-by-three board. We will call the renderSquare method on “this” (meaning this component we are writing on, the Board component), and we will pass a number as the argument (the “i” in our function above):
render() { return ( <div> <div className="board-row"> {this.renderSquare(9)} {this.renderSquare(5)} {this.renderSquare(2)} </div> <div className="board-row"> {this.renderSquare(3)} {this.renderSquare(4)} {this.renderSquare(1)} </div> <div className="board-row"> {this.renderSquare(6)} {this.renderSquare(7)} {this.renderSquare(8)} </div> </div> ); } }
Notice also the className attribute I included here for each row <div>. You can, of course, call it something different than “board-row”; I included it just to remind you to use that in your CSS file to format the square rows. This is an example of what the CSS section for the board row formatting might look like:
.board-row:after { display: table; content: ""; clear: both; }
The last step would be to tell React to look at the DOM (Document Object Model) and render our board component to the HTML element that has the id set to “root”:
ReactDOM.render( <Board />, document.getElementById('root') );
After this, you would want to build the interactive functionality of your game in another component, incorporating the two stateless components you built in this example. Interactive components are stateful, which means they have a state object that remembers and handles a collection of properties about a component. You’d need state in your sudoku game to accept user input and keep track of numbers as well as to do other things, such as tell the user when they’ve made a mistake or won the game.
Props vs State
Both props and state are objects in React which hold information about the component. It is what they do with that information that marks their differences. Props are immutable and are passed down from parent to child components, and child components can’t modify the props. On the other hand, state stores information about the component which can be changed and updates itself accordingly. State can be used to store important information about a program.
Conclusion
State and props are two concepts React users will come across. Remember that props are used to pass on information, or properties, from one component to the next. Although using state in React will add some more interactivity to your application, the tradeoff is that it will also add a lot of complexity.
Stateless components are less complex and thereby easier to test and work with. When working on your next React project, definitely try to get more familiar with all you can do with props.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.