A lifecycle in React can be thought of in three different phases: mounting, updating, and unmounting. Mounting occurs when the React component renders to the DOM (Document Object Model). When changes are made to that component, it enters the updating phase.
In the update phase of the lifecycle, a series of internal methods are run to check for new information and re-render that component. Unmounting is simply when a component is removed from the DOM.
The course in this article discusses the broad implications of the lifecycle and some of the commonly used methods. There are more lifecycle methods that can be used that will not be discussed here. For more information about other lifecycle methods, refer to this introduction.
What is React Lifecycle?
When a component is loaded into the DOM, it enters its first of three phases, or lifecycle. This first phase is called mounting. In this phase, the component is rendered as it is originally defined. It is now ready to interact with the user and enter into the second phase.
In the second phase, the user has interacted with the component and made some changes. In most cases, the user has performed an action that has changed the components state. We are now in the update phase of the lifecycle.
In the update phase, React will re-render the component to display the changes. In our example above of changing state to trigger the update phase, the setState()
method will be called to change the initial state and start the re-rendering process. For more on setState, read this introductory article.
Finally, when we navigate away from a specific component, we can clear it from the DOM. This is known as unmounting and is the third phase of the lifecycle. Though this phase is not required, it is recommended for component heavy apps.
Common Lifecycle Methods
Now that we’ve gone over the lifecycle sequence of a React component, let’s look at some common lifecycle methods. Lifecycle methods are React methods that are called at the different lifecycle stages. Some of them are required while some are optional. We are going to look at the required and commonly used optional methods.
Mounting
When mounting a class component, the first commonly used optional method is the constructor()
method. It’s here in the constructor method that the component’s initial state can be directly declared.
class App extends React.Component { constructor(props) { super(props); this.state= { currentUser : " " } } }
In this example, we are setting an initial state as an object for a current user. The constructor()
method requires the boilerplate super()
method. For more on the base constructor super()
, read our setState guide.
For our purposes, when this component is mounted, we will assume no one has logged in yet. In order for a user to log in, we need a login form. This brings us to the first required method, render()
.
A required method in mounting a component is the render()
method. After the class component is constructed with its initial state set, we can render either another component or HTML.
render(){ return ( <LoginForm /> ) }
The syntax of render()
and its return statement are slightly different than other methods. Notice we use parentheses after return and not curly brackets. Here in the return statement, we are rendering the LoginForm component.
When a user logs in, we will have access to the credentials. In a functioning app, a log in requires a fetch request to a server to retrieve the matching credentials. In the context of this introductory article on lifecycle, we will set the state directly.
In this simple example, we have a user, John. He enters his password and pushes the login button. This sends a fetch request to the server and the server responds with John’s credentials. Now that John is logged in, we want him to stay logged in until he decides to log out.
To do this, we can use another quite common optional method, componentWillMount()
. If this lifecycle method is called, it will execute after the component renders. This is a good place to set our new state.
componentWillMount() { this.setState({ currentUser: "John" }) }
Now, after rendering the component and before mounting, the component’s state is set with a current user of John. Even if the page is refreshed, the state will be set with John, so our user stays logged in.
Using the seState()
method also brings us into the update phase of lifecycle. When setState()
is called, it signals to React that a change has been made to the component. React will now re-render the component to show any changes made to the user. In our case, it will show that John is logged in.
Updating
The update stage in the lifecycle has quite a few optional lifecycle methods that are beyond the scope of this introduction. Suffice it to say for our purposes that when a change is detected (commonly with setState), React will call the required render()
method again. Then the component will re-render to reflect the changes made.
Unmounting
The final stage in a component’s lifecycle is called unmounting. It’s here that a component is removed from the DOM. Although not required, it helps a component heavy app perform more efficiently.
Unmounting has only one optional method, componentWillUnmount()
. Our user, John, now wants to log out. When he clicks the logout button, we can use componentWillUnmount()
to remove his user profile from the DOM.
This example is a bit contrived, but shows clearly what is happening at each stage of the lifecycle.
class UserContainer extends React.Component { constructor(props) { super(props); this.state = { currentUser: " " } } componentWillMount() { this.setState({ currentUser: "John" }) } logout = () => { this.setState({ currentUser: " " }) } render() { if this.state.currentUser === "John" { return ( <UserProfile /> <button type="button" onclick={this.logout}>Logout</button> ) } else { return( <button type="button>">Login</button> ) } } } class UserProfile extends React.Component { componentWillUnmount() { alert("Logged Out") } render() { return ( <h1>Welcome back, John!</h1> ) } }
We have two components. The first is UserContainer and it simply holds the component UserProfile. UserProfile contains the user information.
In UserContainer, we construct our component and set the initial state like before. The component renders with a login button very briefly. Right before we mount the component, we log in John. This causes React to update the component and re-render it with John’s credentials.
Now that John is logged in, we render the UserProfile component. This contains all of John’s user information, so we need to remove it from the DOM as soon as he logs out.
When the logout button is clicked, it executes our logout function that simply returns the current user to an initial state of an empty string. Because our logout function calls setState, React will re-render the container component. Now, it will not show the UserProfile component and we will get a pop-up alert notifying the user he or she has logged out.
Conclusion
There is a lot more to React lifecycle than is covered here. The goal of this article is to introduce the basic concepts with some simple code examples. Now that the concept of lifecycle has been introduced, the official React documentation will be more clear.
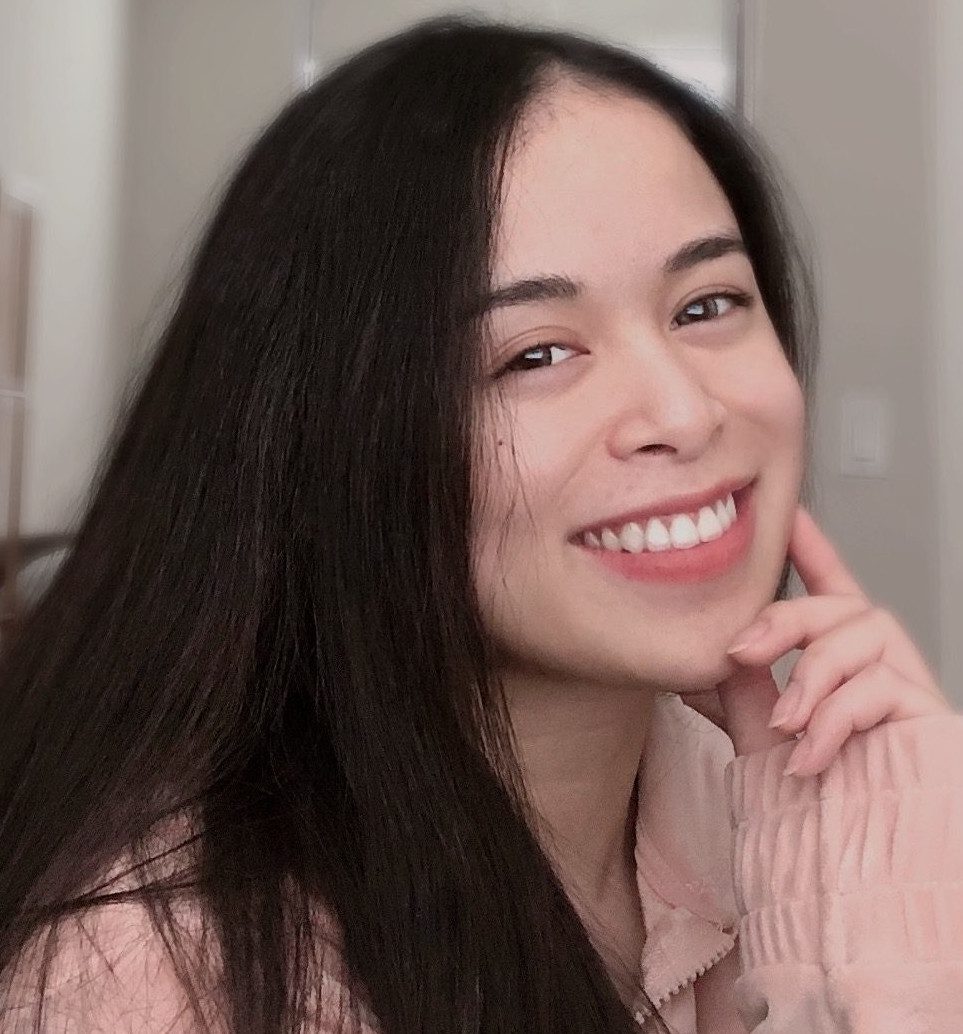
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
After reading this guide and the supplemental articles linked, you now have a grasp on what lifecycle is in React as well as the ability to go deeper on your own. As with all complex concepts in programming, daily practice helps in working out the details.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.