React is a program that contains prewritten lines of code that enable developers to create interactive user interfaces or single-page web applications. Platforms like Facebook, Netflix, Atlassian, and Salesforce were built with React. This library comes with class components and functional components that facilitate the development of user interfaces. For React developers, React exercises are a great way of building skills with the program.
Learning React requires prior knowledge of programming languages like JavaScript, HTML, and CSS. To make your learning process faster and more productive, you need practice exercises. “Where can I practice React?” you may ask. In this article, we curated a list of React exercises followed by code solutions. We also go over where you can get help with React and find answers to common questions you may have.
React Exercises to Help You Learn React
The exercise list below should provide answers to additional questions you may have about React. If you’re trying to specialize as a React developer and get better at building user interfaces, these should be useful to you. Each exercise explores a major concept in React and is followed by the solution. These exercises can help you with common interview questions if you’re on the job hunt.
10 React Exercises and Practice Problems (With Solutions)
1. How is virtual DOM used in the reconciliation process?
Virtual DOM is a lightweight virtual copy of a user interface in memory. A Virtual DOM can be easily updated or manipulated. In React, each HTML DOM has a corresponding virtual DOM. Both copies are synced with each other through a process called reconciliation. So, how is virtual DOM used in the reconciliation process?
Solution: To reconcile the virtual DOM and real DOM objects, React creates a component tree consisting of nodes. React uses an algorithm to compare Real and Virtual root elements and remounts the elements with changed states. To properly identify which nodes need to be remounted, React uses the key attributes assigned to each node element.
2. How do you build a header in React?
A header is a component in React and one of the major elements in web design. Building headers is a very common task for UI developers. It is a navigation component that enables visitors to access the different pages, actions, and information on a website. React can be used to design responsive and interactive website headers. So, how do you build a website header in React?
Solution: You can create a header with React.js and material UI. First, create your web app and install material UI modules. Then, create a Header.js file in the “src” folder. Build the header with the app bar component from your material UI modules, then import the header and modify it in App.js. Once you’ve done this, you can run the app with the command; “npm start”, from the root directory of your project.
3. How do you convert Figma to React?
Figma is a cloud-based UX/UI design application that comes with robust features for easily creating reusable UI components. This free design tool makes it easy for developers and designers to collaborate on a project, as Figma designs made by designers can be converted into React projects with a few manipulations. So, how do you convert Figma to React?
Solution: To convert a Figma design project to React, you can use several Figma integration tools such as the Pagedraw tool. Using Pagedraw, click “import Figma” then copy and paste the file URL. Click “D” to set elements like text and images as dynamic. Use the stress tester to review the design layout based on screen size and data. Lastly, input the variable names matching code in the Pagedraw sidebar then import the components from your code.
4. How do you mount a component in React?
A React lifecycle is a series of stages in the development of user interfaces using React. The three stages all components in React have are mounting, updating, and unmounting. In the mounting stage, the component elements are imputed in the React DOM. In the updating stage, the component is updated. In the last stage, the component is removed. So, how do you mount a component in React?
Solution: There are four built-in methods in React to mount a component. These are render(), constructor(), componentDidMount(), and getDerivedStateFromProps(). Of all these methods, render() is automatically called. The others are only called when you define them. It is also the method that outputs the HTML components to the ReactDOM.
5. How do you implement conditional rendering in React using conditional rendering with enum?
Conditional rendering is the ability to create and render multiple UI elements that depend on specific conditions. There are different methods of applying conditional renderings such as if/else, switch case operator, conditional rendering with enums, and inline IF with logical && operator. So, how do you implement conditional rendering using conditional rendering with enums?
Solution: The conditional rendering with enums applies when an object is used as a map containing key-value pairs. For example, in the case where Const ENUMOBJECT = {a: ‘1’, b: ‘2’, c: ‘3’,}, you would first need to build an enum object, then create an “EnumState” function with a state property key. After that, you’d create an enum component that would take the values of a state to the “EnumState” function.
6. How do you create a functional component in React?
Functional components do not follow a lifecycle. They are stateless JavaScript functions that accept properties then return JSX elements when creating React applications. They are easier to read and debug because they are created with fewer codes. So, how do you create a functional component in React?
Solution: To create a functional component in React, you write this syntax: import React from ‘react’; function counter ({n}) { return ( <div>{n}</div> ); } export default counter;. A counter function is a pure function used to count the values in a state and return the outcome based on the inputs without side effects.
7. How do you impute expressions in JSX code?
A JSX code is an acronym for JavaScript XML. It is a JavaScript syntax extension in React which provides elements that make it visually easier to write an HTML code within a JavaScript code. It makes it easy for React to display relevant error messages that can help your app development. So, how do you impute expressions in JSX code?
Solution: To implement expressions in JSX code, we declare a variable titled “name”. This variable is placed inside a JSX code and then closed with curly braces. The syntax will look like this: const name = ‘maggie’; const RootElement = <h1>Hello, {name}</h1>; ReactDOM.render( element, document.getElementById( ‘root’) );
8. How are the class properties used in React in practice?
Class properties are properties used to implement classes in React components. You can define static properties on a class using this method. You can also use it to maintain the React context if you need to bind event handlers. You don’t need to define a state in a constructor if you use class properties. So, how do we use class properties in a React component in practice?
Solution: To use class properties, you need to compile your source code using Babel. It comes with a ‘transform class properties’ plugin that allows you to implement class properties in your React component.
9. How do you troubleshoot a web program in React?
Troubleshooting is the step-by-step process of analyzing, identifying, and resolving technical errors and issues in computer software or systems. After building a user interface in React and using the ‘npm start’ to launch the program, you may encounter a list of issues that require troubleshooting. So, how do you troubleshoot a React program?
Solution: To troubleshoot a web program in React, you need to first identify the problem. For example, if the problem is that the program couldn’t be minified, it could be because you’re using an older model of React scripts, which you can correct by upgrading your react script. Otherwise, you might be using a dependency that doesn’t support a modern syntax.
10. How is the state of a component updated?
The state of a component is a JavaScript object that is used to define the situation of a component. It is used to store the property values of a class-based component. These values control how the class component behaves. The state of a component can change if the component triggers changes to its state. So, how do you update the state of a component?
Solution: updating the state of a component in React.js depends on the type of component you’re trying to update. You first have to clear your App.js file. Then, you need to import the component from React and create and name a class component. Then, you must create and name a state object and add a value using ‘this.state syntax’. Lastly, you need to create a new method in the class and update the component state using the ‘this.setState()’ method.
How to Get Help with React
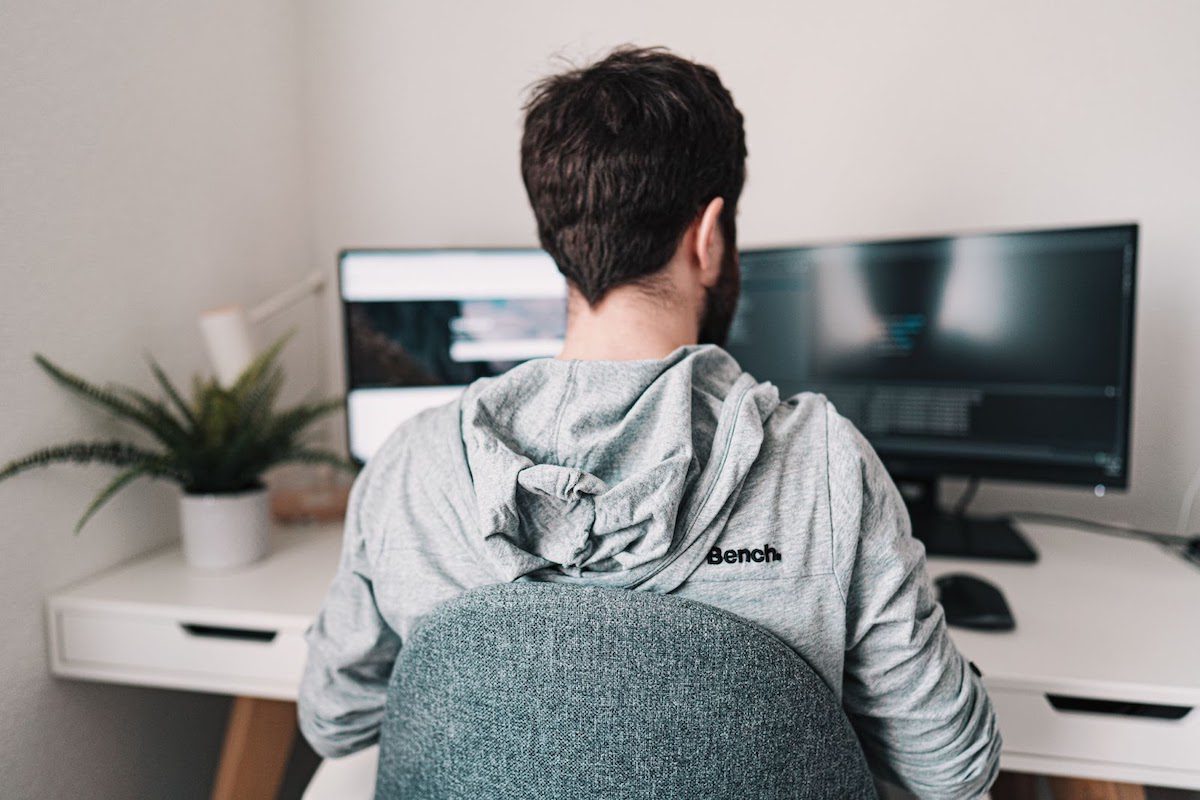
If you want to get better at using React, you should use a method that best suits your learning style. There are many methods you could use, but most of these complement each other, meaning you can easily combine them to hasten your learning progress.
React Exercises
Learning React through exercises can help you build your competence with this JavaScript library. These React exercises are sample questions that require explanatory answers. Since React exercises help you focus on one aspect of React at a time, you can gain a deeper understanding of the processes involved in developing and maintaining a web application lifecycle.
React Projects
Learning through React project development is an ideal way to build professional skills while also producing tangible results. React projects allow you to perform a series of tasks that lead to the development of a web app. They can also help you develop a portfolio so you can prove your development skills to prospective employers.
React Quizzes
React quizzes are random, timed test questions that test your knowledge of React app development processes. At the end of a React quiz, you get a score that represents your knowledge proficiency. React quizzes from sites like W3School come with tutorials so you can learn from your errors. React quizzes are best when you are preparing for an interview.
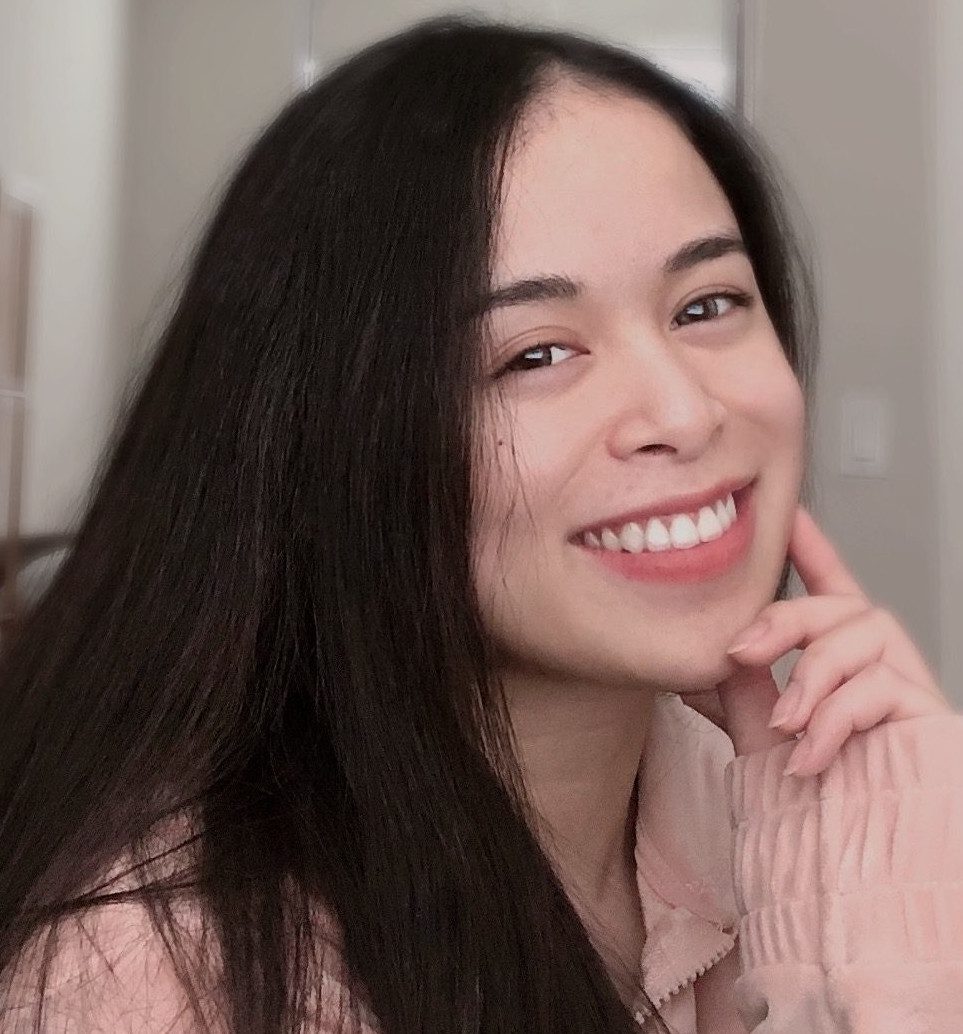
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
React Forums and Blogs
React forums and blogs are great resources for improving your React skills. You can sometimes gain access to learning resources and career opportunities through React forums. You can use online React forums to stay up to date on the latest trends, best practices, and find answers to complex React questions. StackOverflow and Dev’s React community are popular forums.
Where Can I Practice React?
If you’re ready to start writing stronger blocks of code in React, here are some platforms you can use to improve your learning process through practice exercises, quizzes, and projects. Some of these platforms combine tutorials, courses, and exercises to help the students measure their training progress.
Websites to Practice React
- Educative. Educative provides aspiring React developers the opportunity to learn the software through comprehensive courses. Here, you can practice using lessons, quizzes, and challenging projects. You can build your professional skills so you’re ready for whatever technical questions an interviewer might have for you.
- TestDome. TestDome is a platform ideal for both entry-level and senior developers. You can prepare for your next big web development interview through its common interview questions. The skill assessments used by this platform are also used by companies. At the end of your practice, you can receive a certificate if you score within the top 25 percent.
- W3School. This platform makes it easy to learn React and other front end languages without having a user login. You can learn how to better structure your code in React through its practice exercises and quizzes and get scored. The site give a code solution for each exercise so that you can learn from your mistakes and access to React tutorials to help you build your programming skills.
- TestGorilla. TestGorilla is an online practice platform to help you learn React. This platform offers candidates the opportunity to evaluate their proficiency in React concepts. If you’re a front end or full stack developer, this is an ideal place to prepare for your next interview. The skills covered here include advanced concepts, hoops, state and design patterns in React.
- ReactTutorial. ReactTutorial offers an interactive environment for candidates to learn and practice modern applications development in React. You’ll gain access to comprehensive courses that allow you to build React skills and learn the program’s best practices through projects, lessons, and challenges. You’ll get to improve your learning through a one-time payment that lasts for up to five years.
What’s the Best Way to Learn React?
The best way to learn React is through any method that allows you to apply what you’ve learned in practice. Web development is a practical field, so you’ll need hands-on skills if you’re hoping to create a career in it. Participating in exercises, projects, and quizzes allows you to master React faster and increase your chances of working with top employers and clients in this field. You should also consider participating in a React bootcamp.
React Exercises FAQ
The fastest way to learn React is through a combination of learning methods. This includes tutorials, training courses, project tests, and quizzes. You’ll need more than a couple of minutes of practice a day if you’re trying to get hired or promoted. This article contains useful resources to help you boost your React skills.
Yes, you can learn the basic concepts in React in a day. However, if you want to learn more advanced concepts in React, you need regular practice. The best way to practice is by completing React exercises in your spare time. The positive effects of exercise programs cannot be understated.
In some cases, yes. While Angular is a component-oriented framework that enables you to build interactive web applications, it doesn’t outperform React when it comes to front end development. React is the more functional choice for building large and flexible web apps that come with frequent variable data.
React is a front end development library for building user interfaces. It enables users to create single-page web applications using dynamic web components. React comes with multiple features and allows the integration of component packages such as material UI, React bootstrap, React motion and React router to facilitate building user interfaces.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.