getElementById
. getElementByClass
. You may have heard about these functions before. They both allow you to select a particular element in JavaScript.
Once you’ve selected an element you can change its contents, modify its state, or even make it disappear.
There’s another function you can use to select an item on a webpage in JavaScript: querySelector
. This function has a sister called querySelectorAll
, which returns all of the elements that meet a particular query.
In this guide, we’re going to discuss how to use the querySelector
and querySelectorAll
methods. We’ll walk through an example of these methods in action so that you’ll be ready to use them in your code.
What Is querySelector?
querySelector
is a function that returns an element that matches a CSS query. Note the querySelector
method only returns the first instance of a selector. When using querySelector
, you can specify any CSS selector that you want. For instance, you could retrieve an element which has a specific ID set, or an element by its tag name.
You can use this function like this:
var elementName = document.querySelector('#thisElement');
We’ve specified the CSS selectors to match in quotation marks. In addition, you can use the method to select an element within another element:
var parentElement = document.querySelector('main');
var childElement = document.querySelector('#childElement');
This will return the element with the ID #childElement which is contained within the <main> tag in our HTML file.
The querySelectorAll
method works in the same way. The difference is that it returns all the matches found by the query selector, rather than just the first one:
var allParagraphs = document.querySelectorAll('p');
This will return a list of all the <p> tags on an HTML page.
Here are a few examples of selectors you could use with this function:
- p: Selects all <p> tags on the page.
- #name_field: Selects the element with the id “name_field”
- .list: Selects all elements with the class name “list”
- div > p: Selects all <p> elements contained within a <div> element.
You can specify multiple selectors using the querySelector
and querySelectorAll
methods. To learn more about CSS selectors, check out our guide to CSS and HTML attribute selectors.
If matches are not found, null is returned.
How to Use Query Selector
Let’s build a simple web application to illustrate how the querySelector
method works. We’re going to create a web page that displays a fact about HTML. We are going to use querySelector
to add a button which, when clicked, will enable a “dark mode”.
Setting Up the Front End
We’ll start by setting up the front-end for our page:
<!DOCTYPE html>
<html>
<head>
<title>HTML Facts!</title>
<link rel="stylesheet" href="./facts.css">
</head>
<body>
<div class="mainBox">
<h1 class="text">HTML Facts!</h1>
<p class="text">Did you know that HTML is actually based on another markup language, SGML, or Standard Generalized Markup Language?</p>
<button type="button">Enable dark mode</button>
</div>
<script src="./facts.js"></script>
</body>
</html>
We have created a simple HTML webpage that displays a fact about HTML. Right now, our page looks like this:
While our page does give our user a fact about HTML, it is missing two key features: styles and a functional dark mode button. Let’s add the following styles to a file called facts.css:
.mainBox {
margin: auto;
width: 50%;
text-align: center;
background: hotpink;
padding: 5%;
}
body {
background: lightblue;
}
button {
padding: 10px;
background: lightgreen;
border-radius: 10px;
border: none;
}
These styles make our site look more aesthetically pleasing:
That’s all we need to do on the front-end. Now we’re going to focus on adding our dark mode using JavaScript.
Adding Dark Mode Using JavaScript
Our dark mode function should include two features:
- The heading and paragraph inside our box should be changed to white.
- The blue background of our site should be changed to black.
Let’s get started by selecting the DOM elements (webpage elements) that we want to target: the heading, the paragraph, and the main body of our page. We’re going to do this in a file called facts.js:
const heading = document.querySelector("h1");
const paragraph = document.querySelector("p");
const body = document.querySelector("body");
This code returns the first element in the document that has the tag <h1>, <p>, and <body>Now we’re going to create a function that changes the colors of our text and body tag. We’ll change our heading and paragraph text to white and the background of our site to black.
function enableDarkMode() {
heading.setAttribute("style", "color: white;");
paragraph.setAttribute("style", "color: white;");
body.setAttribute("style", "background-color: black;");
}
We’re almost there. The next step is to set up an event listener. This listener will listen for whenever a user clicks our button. When the user clicks the button, the dark mode will be enabled:
document.querySelector("button").addEventListener("click", enableDarkMode);
Now, let’s see what happens when we click our button:
Our site has successfully changed into a dark mode.
Conclusion (and Challenge)
You’ll notice that our code only allows a user to enable dark mode. What gives? This presents a challenge for you: write the code that will allow a user to disable dark mode on the site.
The querySelector
method allows you to select HTML elements based on a CSS selector query. This method returns the first instance of a match. You can use the querySelectorAll
method to retrieve a list of all the elements that match a specified selector
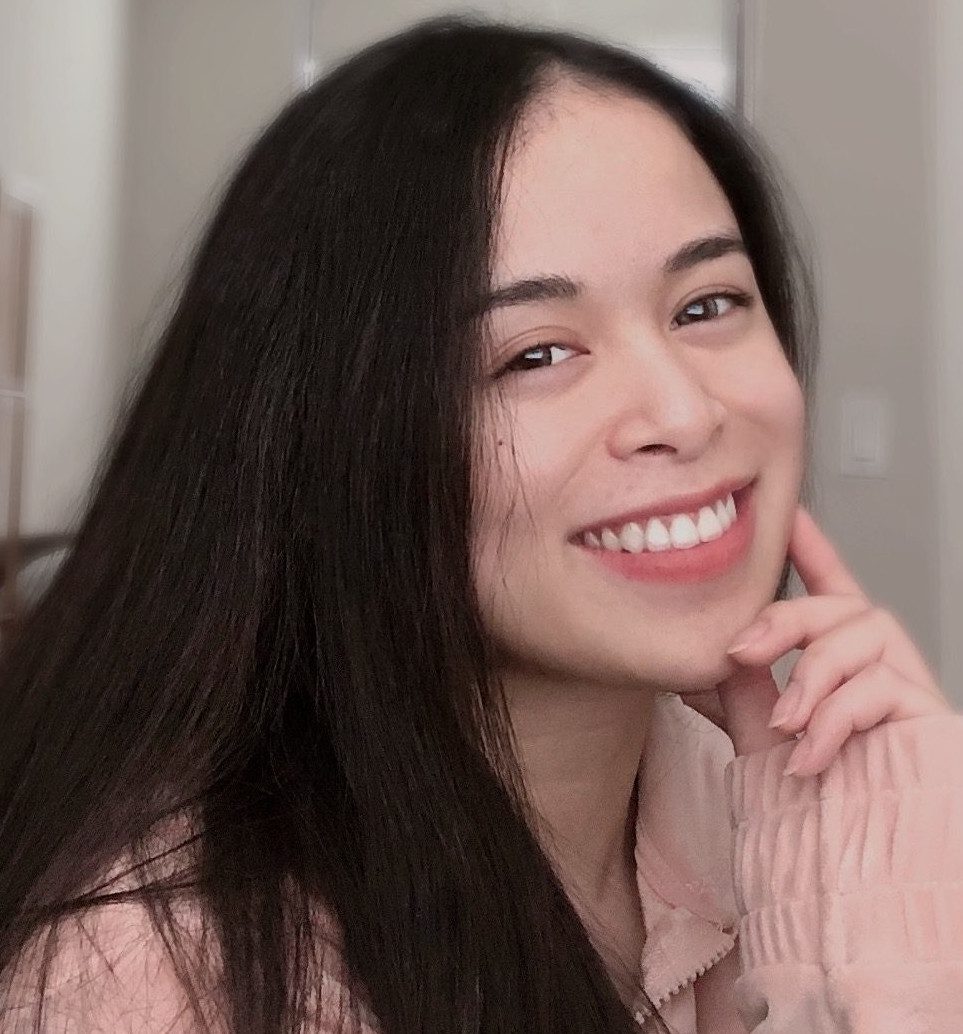
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now you’re ready to start using the querySelector
method like a JavaScript pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.