The Python zip() function accepts iterable items and merges them into a single tuple. The resultant value is a zip object that stores pairs of iterables. You can pass lists, tuples, sets, or dictionaries through the zip() function.
Python has a number of built-in functions that allow coders to loop through data. One of these functions is Python zip. The zip() function creates an iterator that will merge elements from two or more data sources into one.
In this tutorial, we are going to break down the basics of Python zip(). We’ll also discuss how to iterate over a zip and how to unzip a zipped object.
Python Iteration Refresher
In Python, we use the term iterate to describe when a program is running through a list. For example, say you have a for loop that prints out the name of every branch a company operates. We would say that our program is iterating through the list of names.
An iterable, on the other hand, is an object that can return its member items individually. Arrays are iterables because you can print out each item individually by using a for loop.
Python zip Function
The zip() function combines the contents of two or more iterables. zip() returns a zip object. This is an iterator of tuples where all the values you have passed as arguments are stored as pairs.
Python’s zip() function takes an iterable—such as a list, tuple, set, or dictionary—as an argument. The function will generate a list of tuples that contain elements from each iterable you have passed into the function.
The syntax for the zip() function is as follows:
zip(iterable1, iterable2, ...)
You can include as many iterables as you want. You must specify the iterables you want to merge as arguments to the zip() function.
The zip() method continues executing until the iterable objects are fully zipped. This happens when the iterables are exhausted. In other words, the zip() method stops when all of the possible pairs are created.
zip Python Example
Let’s say you have two Python lists. One list contains employee names and the other list contains employee numbers. You want to merge both lists all into an array of tuples. This will let you store employee names and numbers side-by-side.
We can use the zip() function to merge our two lists. Here is an example program that will merge this data:
employee_numbers = [2, 9, 18, 28] employee_names = ["Candice", "Ava", "Andrew", "Lucas"] zipped_values = zip(employee_names, employee_numbers) zipped_list = list(zipped_values) print(zipped_list)
Our zip function returns the following:
[('Candice', 2), ('Ava', 9), ('Andrew', 18), ('Lucas', 28)]
Our program has created an array of tuple items. Each tuple contains the name of an employee and the respective employee number.
On the first two lines of our code, we declare variables that store our employee numbers and employee names.
Next, we perform a zip() function. This function merges our two lists together and creates a new array of tuples.
We convert our zip item into a list. This is because the zip() function returns a zip object. We can iterate over a zip object. However, we cannot print out a zip to the console and see its contents in a readable form. We must convert our zip object to a list, so we can view the contents of the zip from the console.
We can tell that our zipped_values is a zip() item by adding the following code to our above program:
print(type(zipped_values))
Our code returns the following zipped class:
<class 'zip'>
In the above example, we zipped two items together. However, if we had more that we wanted to zip, we could also do that. The only change we would make is to pass another list of items to our zip() function.
Looping Over Iterables Using zip in Python
The zip() function returns an iterator. This means we can view the contents of each zipped item individually.
Working with multiple iterables is one of the most popular use cases for the zip() function in Python. For example, if you want to go through multiple lists, you may want to make use of the zip() function.
Let’s use the zip() function to iterate over both our employee_numbers and employee_names lists:
employee_numbers = [2, 9, 18, 28] employee_names = ["Candice", "Ava", "Andrew", "Lucas"] for name, number in zip(employee_names, employee_numbers): print(name, number)
Our code returns the following:
Candice 2 Ava 9 Andrew 18 Lucas 28
Our program iterates through the list of tuples that zip() returns, and divides them into two values: name and number.
This makes it easy for us to iterate through multiple iterable objects at once. We can now view each employee name alongside their respective employee number. If we wanted to, we could use this to iterate through three or more iterable objects.
Unzip Values in Python
In our code, we have zipped various types of data. But how do we restore data to its previous form? If you have a list of tuples—or zipped values—that you want to divide, you can use the zip() function’s unpacking operator. This is an asterisk * used in conjunction with the zip() function.
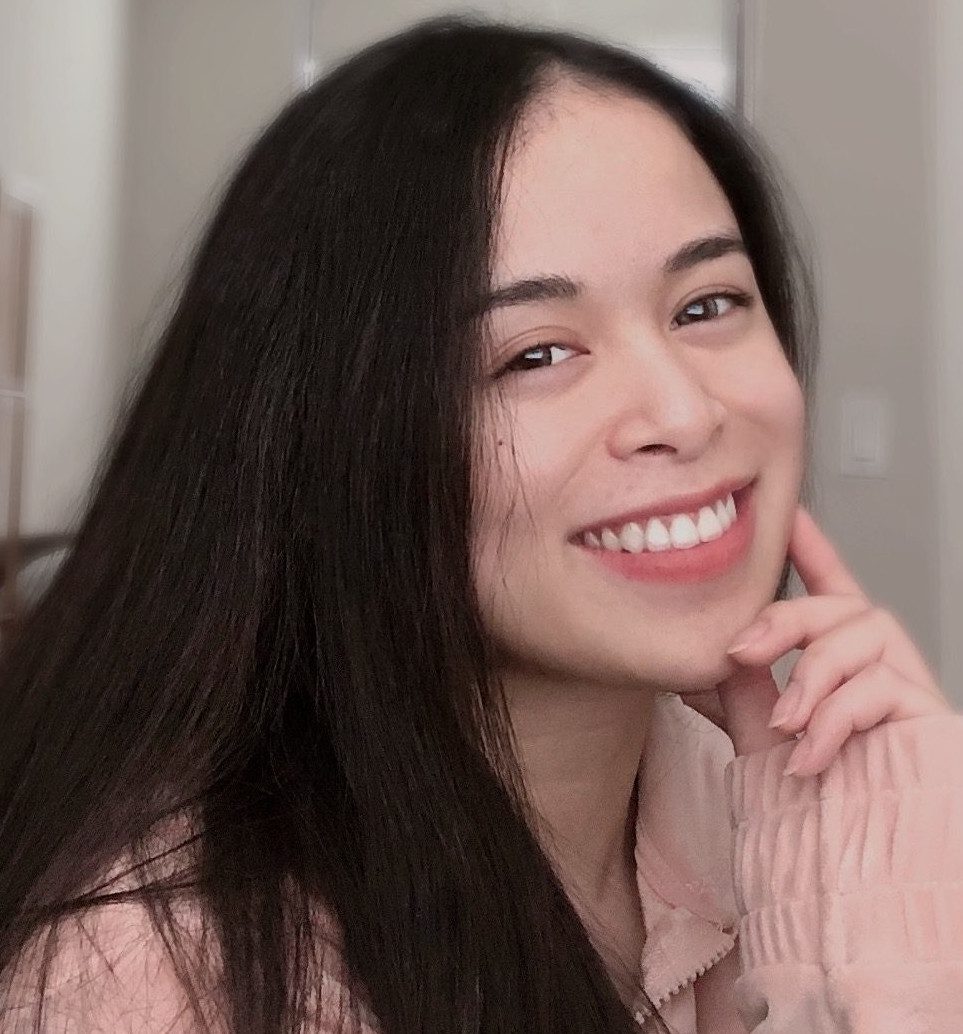
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here is an example of the zip() unpacking operator in action:
employees_zipped = [('Candice', 2), ('Ava', 9), ('Andrew', 18), ('Lucas', 28)] employee_names, employee_numbers = zip(*employees_zipped) print(employee_names) print(employee_numbers)
Our code returns the following output:
('Candice', 'Ava', 'Andrew', 'Lucas') (2, 9, 18, 28)
On the first line of our code, we define a variable that includes a list of tuples. Then, on the next line, we define two variables. These variables are employee_names and employee_numbers. We assign these variables values from our unzip function.
The unzip function is a zip function that takes our employees_zipped variable unpacks the zip using uses the unpacking operator *. In our above example, we print out our two new variables that contain our employee names and employee numbers.
Conclusion
The zip() function receives iterable objects as an input and combines them into a zip() object. This zip() object displays values from each iterable input side-by-side. This can be useful if you have two or more arrays or tuples that you want to merge into one.
We have discussed how to use the zip() function with zero or one input. You can use zip() to loop over iterables and you can unzip data that you have already zipped. You are now on your way to becoming a master of the Python zip() function.
Read our How to Code in Python guide for more tips and advice on learning the Python software development language.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.