A Python variable stores a value in a program. Variables have two parts: a label and a value. These two parts are separated by an equals sign (=). You can use the label to refer to the value assigned to the variable throughout your program. Variables can change in value.
Variables are embedded in programming. Variables allow you to store data in a program that is associated with a particular name.
For instance, you could have a variable called chocolate, which stores a list of chocolates sold at a local grocery store. Or, you could have a variable called username, which stores a user’s name for a gaming application.
This tutorial will discuss, with examples, the basics of variables, and how you can use them in your Python code.
What is a Python Variable?
A Python variable is a unique identifier for a value. The name assigned to a variable can be used to reference the value assigned to that variable. You can change the value of a variable as many times as you want in your program.
One way of thinking about this is that a variable is a label, which is bound to a particular value in your Python program.
The syntax for a Python variable is:
chocolate = "Hazelnut"
Our variable has three parts:
- chocolate is the name of our variable.
- = is the assignment operator. This operator tells our program we want to assign a value to the label “chocolate”.
- “Hazelnut” is the value we want to assign to our variable. In this case, the value is a Python string object. The double quotes denote a string. Strings can be declared between single quotes, too.
Variable types include Python Booleans, Python dictionaries, integers, and floating-point numbers.
Python: Declare Variable
Suppose we are building a math app, and we are going to be using the number 3652 many times over in our code. Instead of writing it out manually each time, we could store it in a variable, like so:
big_number = 3652
We have declared a variable called big_number. This variable has the value 3652. As a result, when we reference the variable big_number in our code, we can access the value 3652.
We have “declared” a variable.
Now that we have a variable declared in our code, we can use it throughout our program. So, if we want to see what is stored in our variable, we could use this code:
print(big_number)
Python Variables: String Example
Variables can store any data type, not just numbers. Suppose we want to store a user’s email address in a variable. We could do so using the same approach we used to declare our big_number variable from earlier. Here’s an example of a variable which stores a string:
email = "user.email@gmail.com"
In our code, we have created a variable called email which stores the value user.email@gmail.com. If we want to see the value assigned our variable, we can do so by printing it out to the Python console:
print(email)
Our code returns:
user.email@gmail.com<
Reassign a Variable
So far, we have talked through how to declare a variable. This is the process of assigning an initial value to a variable.
Variables can be changed in Python. This means that after you have declared a variable, you can change the value that it stores.
Changing the value stored in a variable is an incredibly useful function. For instance, say you are receiving user input in your code. You may want to change the default values to those inserted by the user.
Take the following variable:
username = "lassiter202" print(username)
Our variable is called “username”. Our code returns: lassiter202. Now, suppose we want to change our user’s username to lassiter303. We could do so using this code:
username = "lassiter202" print(username) username = "lassiter303" print(username)
Our code returns: lassiter303. At the start of our program, the variable username stores the value lassiter202. So, when we go to print the variable out to the console, the value lassiter202 is returned.
Later in our code we reassign the value of the username variable to lassiter303. This changes the value of our variable. So, when we print out the username variable again, the value lassiter303 is returned, because we reassigned our variable a new value.
Assign Values to Multiple Variables
In our code above, we assigned one value to a variable per line of code.
However, it is possible to assign values to multiple variables on one line of code. To do so, we can use a technique called multiple assignment.
Suppose we want to assign the following values to these variables:
- espresso should be equal to 2.10.
- latte should be equal to 2.75.
- cappuccino should be equal to 2.60.
We could use the following code to assign these values to their corresponding variables:
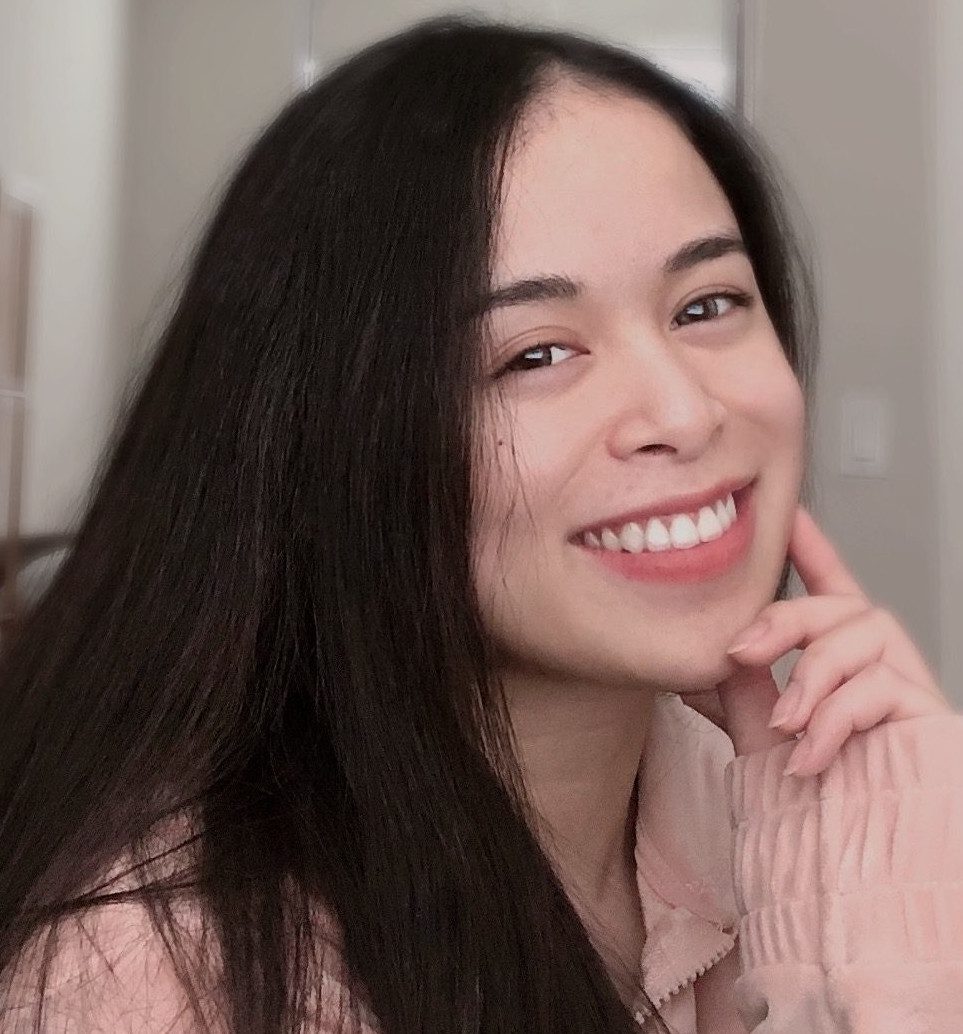
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
espresso, latte, cappuccino = 2.10, 2.75, 2.60 print(espresso) print(latte) print(cappuccino)
Our code returns:
2.10 2.75 2.60
We were able to assign values to our three variables on one line. We did so by separating out the names of our variables, and the values we wanted to assign to those variables, using commas.
Similarly, you can assign the same value to multiple variables on one line.
Suppose we want to assign the value 1 to the variables count1, count2, and count3. We could do so using this code:
count1 = count2 = count3 = 1 print(count1) print(count2) print(count3)
Our code returns:
1 1 1
Each of our variables has been assigned the value 1.
These approaches to assigning variables are useful because they allow you to reduce the length of your code. However, you should use these methods of assigning values to variables with caution.
If your code looks confusing with too many multiple assignment statements, you may want to remove a few. This will help you preserve the readability of your work.
The number of values you specify should be equal to the number of labels you identify. Or, you should specify multiple labels and one value. You should not, for instance, specify two labels and seven values. Python would not know what values should be assigned to what labels.
Delete a Python Variable
There may be a scenario where you want to delete a variable in Python.
To do so, you can use the Python del statement. The del keyword allows you to delete objects in Python, such as lists, list items, and variables.
Suppose we have a variable called username that we want to delete. We could delete the variable using the following code:
username = "linda101" del username print(username)
Our code returns:
NameError: name ‘username’ is not defined
Our code returns an error when we try to print out the value of the username variable. This is because we deleted our variable using the del keyword. As soon as del username is executed, our variable is deleted and no longer stores any value in our code.
How to Name a Python Variable
Every programming language has its own best practices around naming variables.
Style Rules
Here are the main rules you should keep in mind when naming variables in Python:
- They should only contain numbers, letters, and underscore characters.
- Variables cannot begin with a number.
- They cannot include spaces.
So, if you’re storing a user’s email address, the variable name email or email_address would be acceptable. However, the variable name email address or &email_address would not work.
Variable Style
In Python, there are a few style rules that you should keep in mind. These are:
- Variables should not start with an uppercase letter.
- Using camel case is not preferred. Instead, separating words with underscores is used.
So, the variable EmailAddress is not considered the best practice, because the variable starts with an uppercase character. Technically, this variable name is valid, but most developers prefer to start their variable names with a lowercase character.
The variable emailAddress, while also still valid, is also not preferred. This is because the variable uses camel case (the second word starts with a capital letter). Our variable does not separate each word in the variable by using an underscore.
Case Sensitivity
It’s also worth noting that variables are case-sensitive in Python. For instance, the variables email_address and emailAddress would be considered two different values, because both variables use different cases. However, you should err on the side of caution anyway and avoid writing variables that are too similar, otherwise you may confuse them.
Choosing the Right Name
Assuming you have followed the rules above, your variable name will be accepted in Python. However, you should still make sure that you pick the right name for a variable. The name you choose should be easy to understand and reflect the purpose of the variable.
If you want to store a user’s email address, email is a good variable name. user_identifier is not as clear. Almost anything could be used to identify a user. This would not make for such a good variable name to store a user’s email address.
Conclusion
Python variables store values in a program. You can refer to the name of a variable to access its value. The value of a variable can be changed throughout your program. Variables are declared using this syntax: name = value.
Python variables are useful because they allow you to reduce the need to repeat the same value in your code.
This tutorial discussed how you can use variables in your Python code. Now you’re ready to start working with variables like a Python expert.
Challenge
As a challenge, declare a variable with the following attributes:
- Its name should be “score”.
- Its value should be 9.
Then, use a math operator to multiply the value of “score” by two. You should print out the value to the console when you are done.
Next, try to declare three variables called:
- student1grade
- student2grade
- student3grade
These variables should all have the value “A”. You should declare all of these variables on the same line because they share the same value.
For advice on top online Python books, courses, and other learning resources, read our complete How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.