Python does not unpack bags well. When you see the error “valueerror: too many values to unpack (expected 2)”, it does not mean that Python is unpacking a suitcase. It means you are trying to access too many values from an iterator.
In this guide, we talk about what this error means and why it is raised. We walk through an example code snippet with this error so you can learn how to solve it.
The Problem: valueerror: too many values to unpack (expected 2)
A ValueError is raised when you try to access information from a value that does not exist. Values can be any object such as a list, a string, or a dictionary.
Let’s take a look at our error message:
valueerror: too many values to unpack (expected 2)
In Python, “unpacking” refers to retrieving items from a value. For instance, retrieving items from a list is referred to as “unpacking” that list. You view its contents and access each item individually.
The error message above tells us that we are trying to unpack too many values from a value.
There are two mistakes that often cause this error:
- When you try to iterate over a dictionary and unpack its keys and values separately.
- When you forget to unpack every item from a list to a variable.
We look at each of these scenarios individually.
Example: Iterating Over a Dictionary
Let’s try to iterate over a dictionary. The dictionary over which we will iterate will store information about an element on the periodic table. First, let’s define our dictionary:
hydrogen = { "name": "Hydrogen", "atomic_weight": 1.008, "atomic_number": 1 }
Our dictionary has three keys and three values. The keys are on the left of the colons; the values are on the right of the colons. We want to print out both the keys and the values to the console. To do this, we use a for loop:
for key, value in hydrogen: print("Key:", key) print("Value:", str(value))
In this loop, we unpack “hydrogen” into two values: key and value. We want “key” to correspond to the keys in our dictionary and “value” to correspond to the values.
Let’s run our code and see what happens:
Traceback (most recent call last): File "main.py", line 8, in <module> for key, value in hydrogen: ValueError: too many values to unpack (expected 2)
Our code returns an error.
This is because each item in the “hydrogen” dictionary is a value. Keys and values are not two separate values in the dictionary.
We solve this error by using a method called items(). This method analyzes a dictionary and returns keys and values stored as tuples. Let’s add this method to our code:
for key, value in hydrogen.items(): print("Key:", key) print("Value:", str(value))
Now, let’s try to run our code again:
Key: name Value: Hydrogen Key: atomic_weight Value: 1.008 Key: atomic_number Value: 1
We have added the items()
method to the end of “hydrogen”. This returns our dictionary with key-value pairs stored as tuples. We can see this by printing out the contents of hydrogen.items()
to the console:
print(hydrogen.items())
Our code returns:
dict_items([('name', 'Hydrogen'), ('atomic_weight', 1.008), ('atomic_number', 1)])
Each key and value are stored in their own tuple.
A Note for Python 2.x
In Python 2.x, you use iteritems()
in place of items()
. This achieves the same result as we accomplished in the earlier example. The items()
method is still accessible in Python 2.x.
for key, value in hydrogen.iteritems(): print("Key:", key) print("Value:", str(value))
Above, we use iteritems()
instead of items()
. Let’s run our code:
Key: name Value: Hydrogen Key: atomic_weight Value: 1.008 Key: atomic_number Value: 1
Our keys and values are unpacked from the “hydrogen” dictionary. This allows us to print each key and value to the console individually.
Python 2.x is starting to become deprecated. It is best to use more modern methods when available. This means items()
is generally preferred over iteritems()
.
Example: Unpacking Values to a Variable
When you unpack a list to a variable, the number of variables to which you unpack the list items must be equal to the number of items in the list. Otherwise, an error is returned.
We have a list of floating-point numbers that stores the prices of donuts at a local donut store:
donuts = [2.00, 2.50, 2.30, 2.10, 2.20]
We unpack these values into their own variables. Each of these values corresponds to the following donuts sold by the store:
- Raspberry jam
- Double chocolate
- Cream cheese
- Blueberry
We unpack our list into four variables. This allows us to store each price in its own variable. Let’s unpack our list:
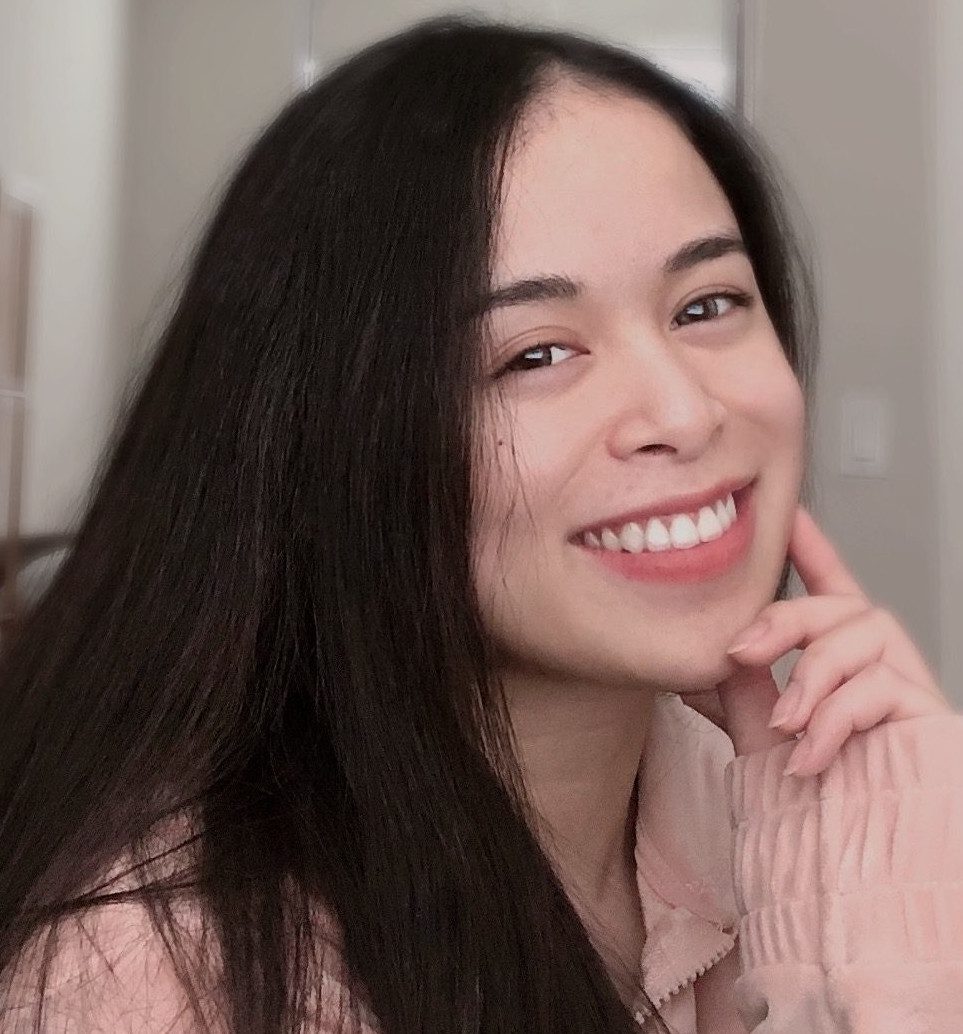
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
raspberry_jam, double_chocolate, cream_cheese, blueberry = [2.00, 2.50, 2.30, 2.10, 2.20] print(blueberry)
This code unpacks our list into four variables. Each variable corresponds with a different donut. We print out the value of “blueberry” to the console to check if our code works. Run our code and see what happens:
Traceback (most recent call last): File "main.py", line 1, in <module> raspberry_jam, double_chocolate, cream_cheese, blueberry = [2.00, 2.50, 2.30, 2.10, 2.20] ValueError: too many values to unpack (expected 4)
We try to unpack four values, our list contains five values. Python does not know which values to assign to our variables because we are only unpacking four values. This results in an error.
The last value in our list is the price of the chocolate donut. We solve our error by specifying another variable to unpack the list:
raspberry_jam, double_chocolate, cream_cheese, blueberry, chocolate = [2.00, 2.50, 2.30, 2.10, 2.20] print(blueberry)
Our code returns: 2.1. Our code successfully unpacks the values in our list.
Conclusion
The “valueerror: too many values to unpack (expected 2)” error occurs when you do not unpack all the items in a list.
This error is often caused by trying to iterate over the items in a dictionary. To solve this problem, use the items()
method to iterate over a dictionary.
Another common cause is when you try to unpack too many values into variables without assigning enough variables. You solve this issue by ensuring the number of variables to which a list unpacked is equal to the number of items in the list.
Now you’re ready to solve this Python error like a coding ninja!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.