You can only read from and write to a Python file if the file is open. If you try to access or manipulate a file that has been closed, the “ValueError : I/O operation on closed file” appears in your code.
In this guide, we talk about what this error means and why it is raised. We walk through two examples of this error so you can learn how to solve it.
ValueError : I/O operation on closed file
It has become good practice in Python to close a file as soon as you have finished working with the file. This helps you clean up your code in the Python interpreter. Once a file has been closed in a Python program, you can no longer read from or write to that file directly.
There are two common scenarios where the “ValueError : I/O operation on closed file” is encountered:
- When you forget to indent your code correctly in a “with” statement
- When you try to read a file after it has been closed using the
close()
statement
Let’s walk through each of these scenarios and discuss them in detail.
Cause #1: Improper Indentation
Let’s write a program that reads a list of student grades from a CSV file. Our CSV file is called students.csv. It currently stores the following data:
Andrew,73,84,92 Linda,76,77,72 Samantha,64,63,75
To start, we import the csv library in our code so we can read our CSV file. We then use a with
statement to open our file:
import csv with open("students.csv", "r") as students: read_file = csv.reader(students)
This code opens the file “students.csv” in read (“r”) mode. We assign the contents of the file to the variable “read_file”.
Let’s print each student’s record to the console:
for s in read_file: print("Name: " + s[0]) print("Test 1 Score: " + s[1]) print("Test 2 Score: " + s[2]) print("Unit Assessment Score: " + s[3])
We use a “for” loop to iterate over every item in the “read_file” variable. This variable stores our CSV file in a list. Next, we use indexing to access each value from each student record. We print each of these values to the console.
Run our code:
Traceback (most recent call last): File "main.py", line 6, in <module> for s in read_file: ValueError: I/O operation on closed file.
Our code returns an error. This is because we’ve tried to iterate over “read_file” outside of our with
statement. The “read_file” variable can only read inside the with
statement. After the with
statement is executed, the file is closed.
To solve this problem, we need to indent our for loop so that it is within our with
statement:
import csv with open("students.csv", "r") as students: read_file = csv.reader(students) for s in range(1, read_file): print("Name: " + s[0]) print("Test 1 Score: " + s[1]) print("Test 2 Score: " + s[2]) print("Unit Assessment Score: " + s[3])
Run this revised code:
Name: Andrew Test 1 Score: 73 Test 2 Score: 84 Unit Assessment Score: 92 Name: Linda Test 1 Score: 76 Test 2 Score: 77 Unit Assessment Score: 72 Name: Samantha Test 1 Score: 64 Test 2 Score: 63 Unit Assessment Score: 75
Our code successfully executes.
Cause #2: Accessing a Closed File
While with
statements are the most common way of accessing a file, you can use an open()
statement without a with
statement to access a file.
Read the contents of our “students.csv” file:
students = open("students.csv", "r") read_file = csv.reader(students) students.close()
We use the open()
method to open the “students.csv” file in read mode. We then use the csv.reader()
method to read our CSV file. We then close our file because we have read its contents into a variable.
Next, print each record from our file to the console using a for loop:
for s in read_file: print("Name: " + s[0]) print("Test 1 Score: " + s[1]) print("Test 2 Score: " + s[2]) print("Unit Assessment Score: " + s[3])
This for loop is the same as the one from our last example. The loop prints out all the information about each record in our CSV file. Let’s test out our code:
Traceback (most recent call last): File "main.py", line 7, in <module> for s in read_file: ValueError: I/O operation on closed file.
Our code raises an error. This is because we try to iterate over “read_file” after we have closed our file. To solve this error, we should close our file after we have iterated over “read_file”:
students = open("students.csv", "r") read_file = csv.reader(students) for s in read_file: print("Name: " + s[0]) print("Test 1 Score: " + s[1]) print("Test 2 Score: " + s[2]) print("Unit Assessment Score: " + s[3]) students.close()
Let’s run our code again:
Name: Andrew Test 1 Score: 73 Test 2 Score: 84 Unit Assessment Score: 92 Name: Linda Test 1 Score: 76 Test 2 Score: 77 Unit Assessment Score: 72 Name: Samantha Test 1 Score: 64 Test 2 Score: 63 Unit Assessment Score: 75
Our code executes successfully. The values in “read_file” are accessible until we close our file. Because we’ve used our for
loop before our close()
statement, our code executes without an error. Once we print each student record to the console, we close our file.
Conclusion
The “ValueError : I/O operation on closed file” error is raised when you try to read from or write to a file that has been closed.
If you are using a with
statement, check to make sure that your code is properly indented. If you are not using a with
statement, make sure that you do not close your file before you read its contents.
Now you’re ready to solve this error like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
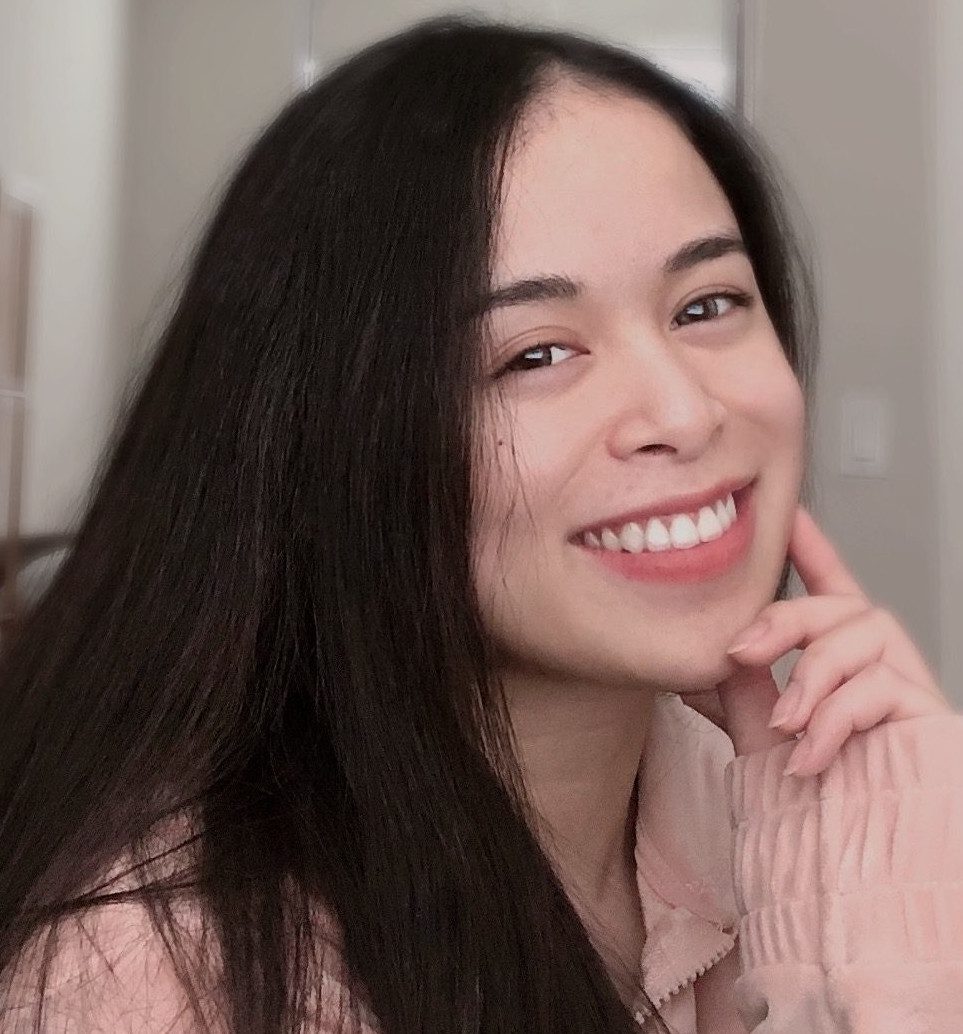
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot