Like lists, Python strings are indexed. This means each value in a string has its own index number which you can use to access that value. If you try to access an index value that does not exist in a string, you’ll encounter a “TypeError: string index out of range” error.
In this guide, we discuss what this error means and why it is raised. We walk through an example of this error in action to help you figure out how to solve it.
TypeError: string index out of range
In Python, strings are indexed starting from 0. Take a look at the string “Pineapple”:
P | i | n | e | a | p | p | l | e |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
“Pineapple” contains nine letters. Because strings are indexed from 0, the last letter in our string has the index number 8. The first letter in our string has the index number 0.
If we try to access an item at position 9 in our list, we’ll encounter an error. This is because there is no letter at index position 9 for Python to read.
The “TypeError: string index out of range” error is common if you forget to take into account that strings are indexed from 0. It’s also common in for loops that use a range() statement.
Example Scenario: Strings Are Indexed From 0
Take a look at a program that prints out all of the letters in a string on a new line:
def print_string(sentence): count = 0 while count <= len(sentence): print(sentence[count]) count += 1
Our code uses a while loop to loop through every letter in the variable “sentence”. Each time a loop executes, our variable “count” is increased by 1. This lets us move on to the next letter when our loop continues.
Let’s call our function with an example sentence:
print_string("String")
Our code returns:
S t r i n g Traceback (most recent call last): File "main.py", line 8, in <module> print_string("test") File "main.py", line 5, in print_string print(sentence[count]) IndexError: string index out of range
Our code prints out each character in our string. After every character is printed, an error is raised. This is because our while loop continues until “count” is no longer less than or equal to the length of “sentence”.
To solve this error, we must ensure our while loop only runs when “count” is less than the length of our string. This is because strings are indexed from 0 and the len()
method returns the full length of a string. So, the length of “string” is 6. However, there is no character at index position 6 in our string.
Let’s revise our while loop:
while count < len(sentence):
This loop will only run while the value of “count” is less than the length of “sentence”.
Run our code and see what happens:
S t r i n g
Our code runs successfully!
Example Scenario: Hamming Distance Program
Here, we write a program that calculates the Hamming Distance between two sequences. This tells us how many differences there are between two strings.
Start by defining a function that calculates the Hamming distance:
def hamming(a, b): differences = 0 for c in range(0, len(a)): if a[c] != b[c]: differences += 1 return differences
Our function accepts two arguments: a and b. These arguments contain the string values that we want to compare.
In our function, we use a for loop to go through each position in our strings to see if the characters at that position are the same. If they are not the same, the “differences” counter is increased by one.
Call our function and try it out with two strings:
answer = hamming("Tess1", "Test") print(answer)
Run our code and see what happens:
Traceback (most recent call last): File "main.py", line 10, in <module> answer = hamming("Tess1", "Test") File "main.py", line 5, in hamming if a[c] != b[c]: IndexError: string index out of range
Our code returns an error. This is because “a” and “b” are not the same length. “a” has one more character than “b”. This causes our loop to try to find another character in “b” that does not exist even after we’ve searched through all the characters in “b”.
We can solve this error by first checking if our strings are valid:
def hamming(a, b): differences = 0 if len(a) != len(b): print("Strings must be the same length.") return for c in range(0, len(a)): if a[c] != b[c]: differences += 1 return differences
We’ve used an “if” statement to check if our strings are the same length. If they are, our program will run. If they are not, our program will print a message to the console and our function will return a null value to our main program. Run our code again:
Strings must be the same length. None
Our code no longer returns an error. Try our algorithm on strings that have the same length:
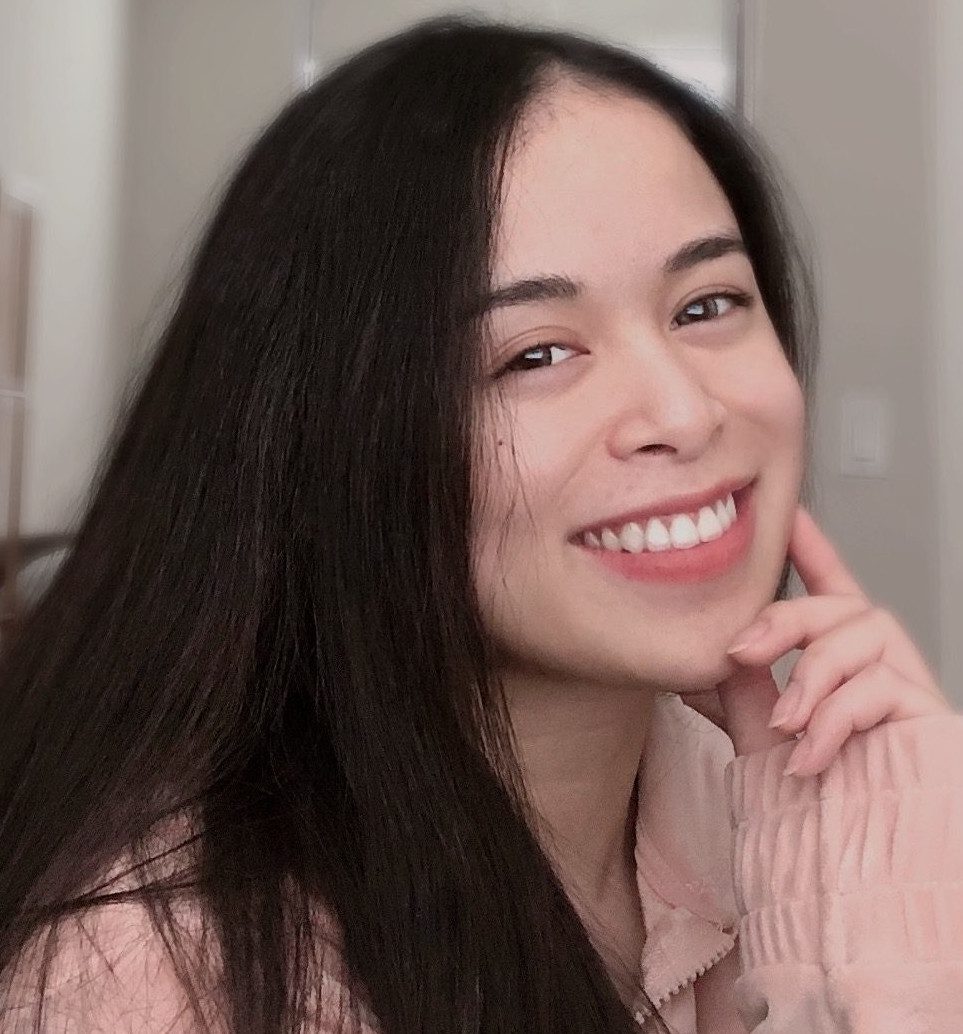
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
answer = hamming("Test", "Tess") print(answer)
Our code returns: 1. Our code has successfully calculated the Hamming Distance between the strings “Test” and “Tess”.
Conclusion
The “TypeError: string index out of range” error is raised when you try to access an item at an index position that does not exist. You solve this error by making sure that your code treats strings as if they are indexed from the position 0.
Now you’re ready to solve this common Python error like a professional coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.