Mistakes are easily made when you are naming variables. One of the more common mistakes is calling a variable “str”. If you try to use the Python method with the same name in your program, “typeerror: ‘str’ object is not callable” is returned.
In this guide, we talk about what the Python error means and why it is raised. We walk through two examples of this error in action so you learn how to solve it.
The Problem: typeerror: ‘str’ object is not callable
Our error message is a TypeError. This tells us we’re trying to execute an operation on a value whose data type does not support that specific operation.
Take a look at the rest of our error message:
typeerror: 'str' object is not callable
When you try to call a string like you would a function, an error is returned. This is because strings are not functions. To call a function, you add () to the end of a function name.
This error commonly occurs when you assign a variable called “str” and then try to use the str()
function. Python interprets “str” as a string and you cannot use the str()
function in your program.
Let’s take a look at two example scenarios where this error has occurred.
Example Scenario: Declaring a Variable Called “str”
We write a program that calculates how many years a child has until they turn 18. We then print this value to the console.
Let’s start by collecting the current age of the young person by using an input() statement:
str = input("What is your age? ")
Next, we calculate how many years a young person has left until they turn 18. We do this by subtracting the value a user has given our program from 18:
years_left = 18 - int(str)
We use the int()
method to convert the age integer. This allows us to subtract the user’s age from 18.
Next, we convert this value to a string and print it to the console. We convert the value to a string because we need to concatenate it into a string. To do so, all values must be formatted as a string.
Let’s convert the value of “years_left” and print it to the console:
years_left = str(years_left) print("You have " + years_left + " years left until you turn 18.")
This code prints out a message informing us of how many years a user has left until they turn 18. Run our code and see what happens:
What is your age? 7 Traceback (most recent call last): File "main.py", line 5, in <module> years_left = str(years_left) TypeError: 'str' object is not callable
Our code returns an error. We have tried to use the str()
method to convert the value of “years_left”. Earlier in our program, we declared a variable called “str”. Python thinks “str” is a string in our program, not a function.
To solve this error, we rename the variable “str” to “age”:
age = input("What is your age? ") years_left = 18 - int(age) years_left = str(years_left) print("You have " + years_left + " years left until you turn 18.")
As we have renamed our variable, Python will use the str()
function when we call it. There is no longer a variable occupying that name. Let’s run our code again:
What is your age? 7 You have 11 years left until you turn 18.
Our code successfully calculates how many years a young person has left until they turn 18.
Example Scenario: String Formatting Using %
This error is also caused by a mistake in string formatting.
Let’s add a few new features to our last program. First, we ask a user for their name. Second, we’re going to craft the message that tells a user how many years they have left until they turn 18 using the % formatting syntax.
Begin by asking a user for their name:
name = input("What is your name? ")
Next, we revise our print()
statement to use the % string formatting syntax. We do this because the % syntax is easier to read when formatting multiple values into a string. Here is the code we use to format the message we will show a user:
print("%s, you have %s years left until you turn 18."(name, age))
Our final program looks like this:
name = input("What is your name? ") age = input("What is your age? ") years_left = 18 - int(age) years_left = str(years_left) print("%s, you have %s years left until you turn 18."(name, age))
Our code replaces the string type %s symbol with the values “name” and “age”, respectively. Let’s run our code and see what happens:
Traceback (most recent call last): File "main.py", line 7, in <module> print("%s, you have %s years left until you turn 18."(name, age)) TypeError: 'str' object is not callable
Our code returns an error because we have forgotten to use the % operator to separate our string and the values we want to add to our string.
Our code thinks we are trying to call “%s, you have %s years left until you turn 18.” as a function because the string is followed by parenthesis.
To solve this error, we add a percentage sign between “%s, you have %s years left until you turn 18.” and (name, age) in our code:
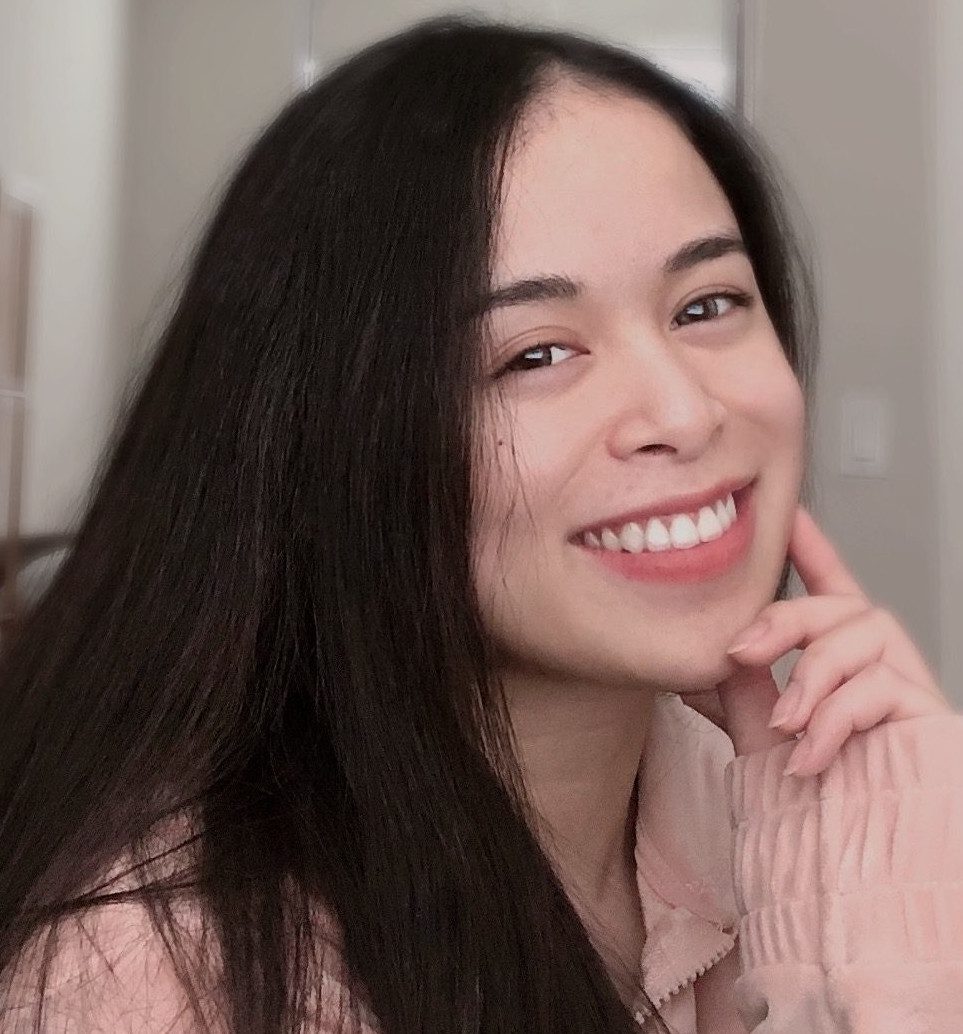
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
print("%s, you have %s years left until you turn 18." % (name, age)) This revision fixes the error: What is your name? Alex What is your age? 7 Alex, you have 11 years left until you turn 18.
Conclusion
The “typeerror: ‘str’ object is not callable” error is raised when you try to call a string as a function. To solve this error, make sure you do not use “str” as a variable name.
If this does not solve the problem, check if you use the % operator to format strings. A % sign should appear between a string and the values into which you want to add to a string.
Now you’re ready to solve this common Python error like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.