Lists are indexed using whole numbers. Whole numbers are known integers. If you try to slice a list using a value that is not an integer, you’ll encounter the “TypeError: slice indices must be integers or None or have an __index__ method” error.
In this guide, we discuss what this error means and why it is raised. We’ll explore an example of this error in action so you can learn how to fix it in your program.
TypeError: slice indices must be integers or None or have an __index__ method
Indexing lets you uniquely identify all the items in a list. Consider the following example:
Python | Ruby | Java |
0 | 1 | 2 |
Each item in our list has its own index number. We can use these index numbers to retrieve an individual item in a list, or a range of items in a list.
Index numbers increment by one for each value on a list. All the index numbers assigned to a list are stored as an integer.
You cannot access items from a list using a floating-point number. Although floating-points are still numbers, they are treated differently to integers. A floating point value does not correspond to any index number in a list.
An Example Scenario
We’re going to write a program that lets a teacher calculate how many times a student has earned a grade of over 75 in their class. We will give the teacher the option to restrict how many grades are searched.
To start, define a list of grades for a student:
grades = [73, 72, 90, 69, 83]
This list contains five values. We are going to ask the teacher how many grades the program should take into consideration. The grades are ordered by the time on which a test was taken. The last grade in the list is the most recent.
Let’s ask the user how many grades they want to review:
to_review = float(input(“How many grades would you like to take into consideration? ”))
We convert the value the user inserts to a floating-point number. This is because we need a number to access values from a list using their index numbers.
Next, use slicing to retrieve those items from our list:
list_of_grades_to_consider = grades[-to_review:]
This code retrieves the last X values from a list, where X is equal to the value that the teacher inserts into the program. For instance, this code retrieves the last two grades from the grades
list if a teacher inserts 2 into the input field that we defined earlier.
Next, let’s write a for loop that calculates how many of these grades are over 75:
high_grades = 0 for g in list_of_grades_to_consider: if g > 75: high_grades += 1
We have defined a variable called high_grades
which tracks how many times a student has earned a grade of over 75. We use a for loop to iterate over every grade in our list of grades to consider. If a grade is over 75, we add 1 to the high_grades
value.
Finally, print out a message to the console that tells us how many times a student has earned a grade of over 75:
print(“This student has earned a grade of over 75 {} times.”.format(high_grades))
Let’s run our program and see what happens:
How many grades would you like to take into consideration? 3 Traceback (most recent call last): File "main.py", line 5, in <module> list_of_grades_to_consider = grades[-to_review:] TypeError: slice indices must be integers or None or have an __index__ method
Our code asks our user how many grades they would like the program to consider. Then, our program stops and returns an error.
The Solution
We surrounded our input()
statement with the float()
method. This converts the value that the teacher inserts into our program to a floating-point number.
Our program returns an error because we’re trying to slice a list using a floating-point number. This is not possible because lists are indexed using integers.
To solve this problem, we’re going to use the int()
method to convert the value a user inserts into our program into an integer:
to_review = int(input(“How many grades would you like to take into consideration? ”))
We’ve replaced float()
with int()
. Run our program and see if it works:
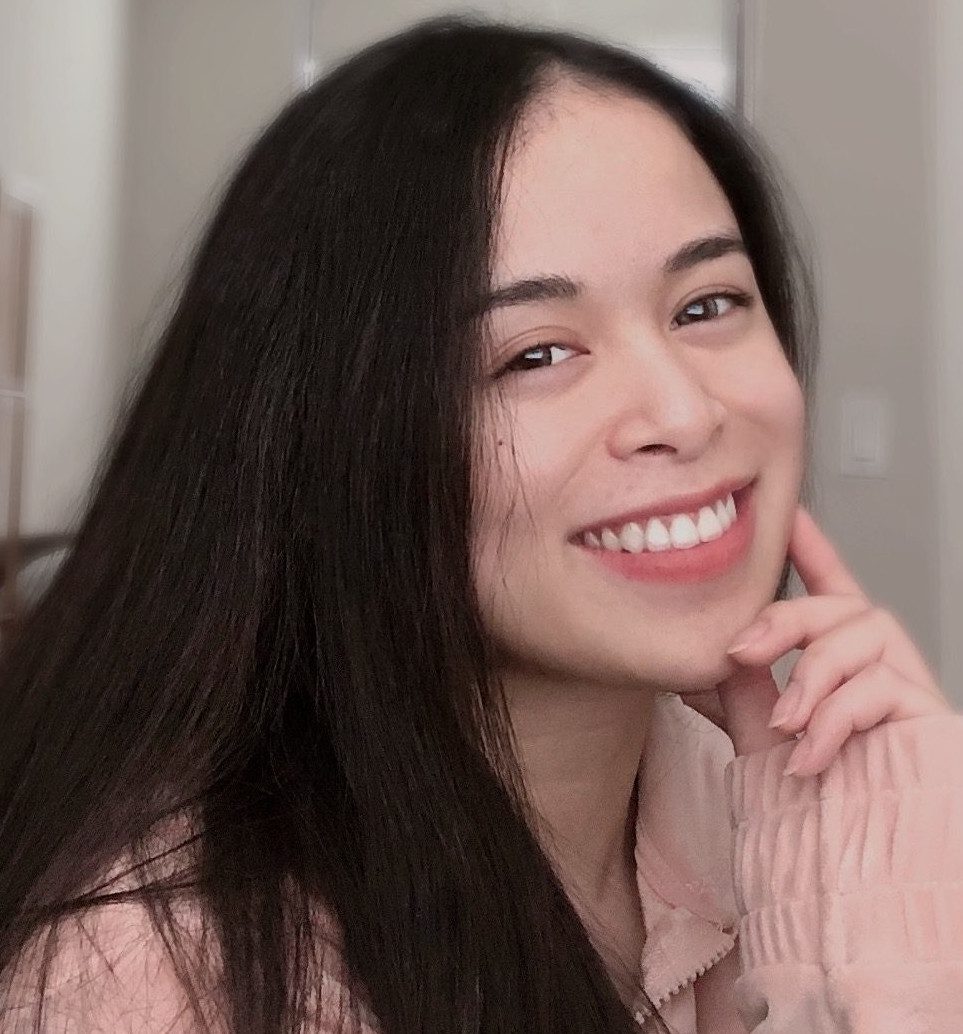
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
How many grades would you like to take into consideration? 3
This student has earned a grade of over 75 2 times.
Our code works. Our code tells us that in the last three tests, a student has earned a grade of over 75 on two occasions.
Conclusion
The “TypeError: slice indices must be integers or None or have an __index__ method” error is raised when you try to slice a list using a value that is not an integer, such as a float.
To solve this error, make sure any values you use in a slicing statement are integers. Now you have the knowledge you need to fix this error like a professional Python coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.