Python is a stickler for the rules. One of the main features of the Python language keeps you in check so that your programs work in the way that you intend. You may encounter an error saying “not all arguments converted during string formatting” when you’re working with strings.
In this guide, we talk about this error and why it pops up. We walk through two common scenarios where this error is raised to help you solve it in your code.
Without further ado, let’s begin!
The Problem: typeerror: not all arguments converted during string formatting
A TypeError is a type of error that tells us we are performing a task that cannot be executed on a value of a certain type. In this case, our type error relates to a string value.
Python offers a number of ways that you can format strings. This allows you to insert values into strings or concatenate values to the end of a string.
Two of the most common ways of formatting strings are:
- Using the % operator (old-style)
- Using the {} operator with the .format() function
When you try to use both of these syntaxes together, an error is raised.
Example: Mixing String Formatting Rules
Let’s write a program that calculates a 5% price increase on a product sold at a bakery.
We start by collecting two pieces of information from the user: the name of the product and the price of the product. We do this using an input() statement:
name = input("Enter the name of the product: ") price = input("Enter the price of the product: ")
Next, we calculate the new price of the product by multiplying the value of “price” by 1.05. This represents a 5% price increase:
increase = round(float(price) * 1.05, 2)
We round the value of “increase” to two decimal places using a round() statement. Finally, use string formatting to print out the new price of the product to the console:
print("The new price of {} is ${}. " % name, str(increase))
This code adds the values of “name” and “increase” into our string. We convert “increase” to a string to merge it into our string. Before we convert the value, “increase” is a floating-point number. Run our code and see what happens:
Traceback (most recent call last): File "main.py", line 6, in <module> print("The new price of {} is {}" % name, str(discount)) TypeError: not all arguments converted during string formatting
It appears there is an error on the last line of our code.
The problem is we mixed up our string formatting syntax. We used the {} and % operators. These are used for two different types of string formatting.
To solve this problem, we replace the last line of our programming with either of the two following lines of code:
print("The new price of {} is ${}.".format(name, str(increase))) print("The new price of %s is $%s." % (name, str(increase)))
The first line of code uses the .format()
syntax. This replaces the values of {} with the values in the .format()
statement in the order they are specified.
The second line of code uses the old-style % string formatting technique. The values “%s” is replaced with those that are enclosed in parenthesis after the % operator.
Let’s run our code again and see what happens:
Enter the name of the product: Babka Enter the price of the product: 2.50 The new price of Babka is $2.62.
Our code successfully added our arguments into our string.
Example: Confusing the Modulo Operator
Python uses the percentage sign (%) to calculate modulo numbers and string formatting. Modulo numbers are the remainder left over after a division sum.
If you use the percentage sign on a string, it is used for formatting; if you use the percentage sign on a number, it is used to calculate the modulo. Hence, if a value is formatted as a string on which you want to perform a modulo calculation, an error is raised.
Take a look at a program that calculates whether a number is odd or even:
number = input("Please enter a number: ") mod_calc = number % 2 if mod_calc == 0: print("This number is even.") else: print("This number is odd.")
First, we ask the user to enter a number. We then use the modulo operator to calculate the remainder that is returned when “number” is divided by 2.
If the returning value by the modulo operator is equal to 0, the contents of our if
statement execute. Otherwise, the contents of the else
statement runs.
Let’s run our code:
Please enter a number: 7 Traceback (most recent call last): File "main.py", line 2, in <module> mod_calc = number % 2 TypeError: not all arguments converted during string formatting
Another TypeError. This error is raised because “number” is a string. The input()
method returns a string. We need to convert “number” to a floating-point or an integer if we want to perform a modulo calculation.
We can convert “number” to a float by using the float() function:
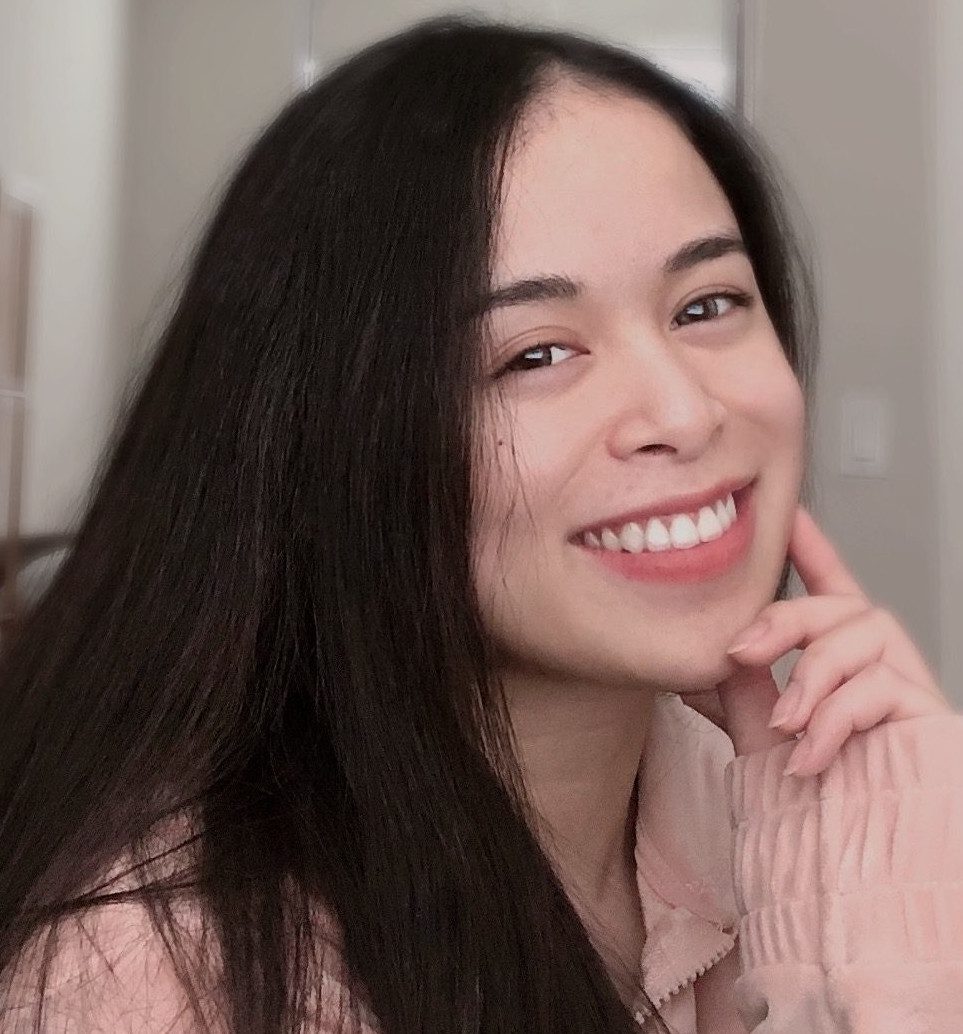
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
mod_calc = float(number) % 2
Let’s try to run our code again:
Please enter a number: 7 This number is odd.
Our code works!
Conclusion
The “not all arguments converted during string formatting” error is raised when Python does not add in all arguments to a string format operation. This happens if you mix up your string formatting syntax or if you try to perform a modulo operation on a string.
Now you’re ready to solve this common Python error like a professional software engineer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.