Python modules are confusing, especially when you define your own. “TypeError: ‘module’ object is not callable” is one of the most common mistakes that Python developers make when working with classes.
In this guide, we talk about what this error means and why it is raised. We walk through an example code snippet to help you overcome this error. Let’s begin!
The Problem: TypeError: ‘module’ object is not callable
Any Python file is a module as long as it ends in the extension “.py”.
Modules are a crucial part of Python because they let you define functions, variables, and classes outside of a main program. This means you can divide your code up into multiple files and categorize it more effectively.
Modules must be imported in a certain way. Otherwise, Python returns an error:
TypeError: 'module' object is not callable
This happens when you try to import a module as a function.
An Example Scenario
Define a module called “cakes”. This module contains one function: read_file. The read_file function will read the contents of a text file.
Our filename determines the name of our module. Because we want our module to be called “cakes”, we write our code in a file called cakes.py:
def read_file(): all_cakes = [] with open("cakes.txt", "r") as cake_file: cake_list = cake_file.readlines() for c in cake_list: all_cakes.append(c.replace("\n", "")) return all_cakes
This function reads the contents of a file called “cakes.txt”. It then iterates through every line of text in the file and adds each line to a list called “all_cakes”.
The replace() method is used to replace any new line (“\n”) characters with an empty value. This removes all new lines. We return “all_cakes” at the end of our function.
Now, open a file called app.py and paste in this code:
import cakes cake_list = cakes() print(cake_list)
This code uses our “cakes” module to read the contents of the “cakes.txt” file. It then prints out all the cakes the function has found in the file.
Let’s run our code:
Traceback (most recent call last): File "main.py", line 3, in <module> cakes = cakes() TypeError: 'module' object is not callable
Our code returns an error.
The Solution
Let’s take a look at the import statement in our app.py file:
import cakes
We import the module “cakes”. This contains all of the variables, classes, and functions that we declare in the “cakes.py” file.
Now, let’s look at our next line of code:
cake_list = cakes()
Although the “cakes” module only contains one function, we don’t specify what that function is. This confuses Python because it does not know what function it should work with.
To solve this error, we call the name of the function we want to reference instead of the module itself:
import cakes cake_list = cakes.read_file() print(cake_list)
Let’s try to run our code again:
['Cinnamon Babka', 'Chocolate Cupcake']
Our code successfully returns the list of cakes.
In our app.py file, we call cakes.read_file().
Python looks at the file “cakes.py” where our “cakes” module is stored and locates the read_file()
function. Then, Python executes that function.
We assign the result of the read_file()
function to a variable called “cake_list”. Then, we print out that list to the console.
Alternatively, import the read_file function directly into our program:
from cakes import read_file cake_list = read_file() print(cake_list)
Our code returns:
['Cinnamon Babka', 'Chocolate Cupcake']
Our code executes the read_file()
function from the “cakes” module. In this example, we import the whole “cakes” module. Instead of doing so, we import one function from the “cakes” module: read_file.
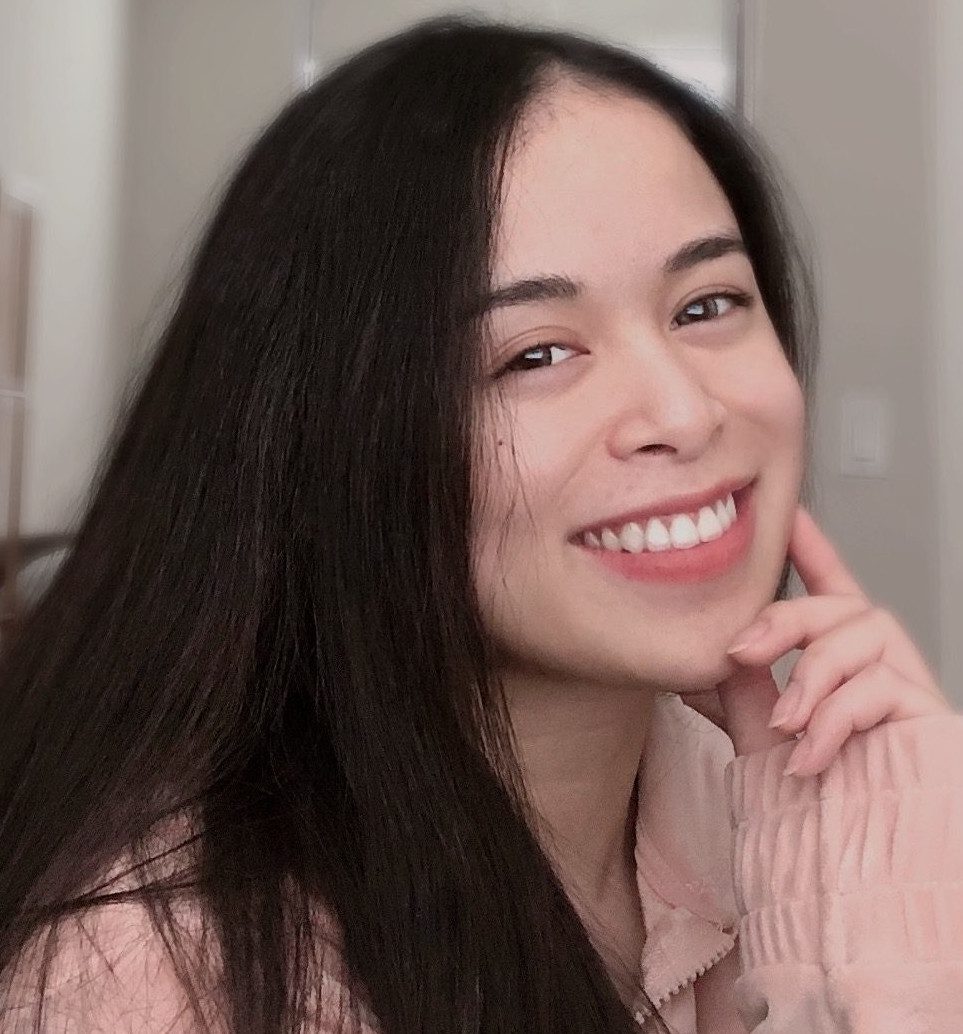
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Notice that when we import read_file, we no longer need to use cakes.read_file()
to call our function. We use read_file()
because we import the read_file()
function directly into our code.
Conclusion
The code for a Python module exists in a different file. There are a few different ways to import functions and values from modules and it’s easy to mess up.
When you work with modules, make sure that you reference the functions, classes, and variables that you want to access correctly. You need to specify the exact function that you want to call from a module if you want to reference a function, rather than calling the module itself.
Now you’re ready to solve this Python TypeError like a professional developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.