String indices must be integers or slices, not strings? What does this error mean? It’s a TypeError, which tells us that we are trying to perform an operation on a value whose type is not compatible with the operation. What’s the solution?
In this guide, we’re going to answer all of those questions. We’ll discuss what causes this error in Python and why it is raised. We’ll walk through an example scenario to help you figure out how to solve it. Without further ado, let’s begin!
The Problem: typeerror: list indices must be integers or slices, not str
Houston, we have a TypeError:
typeerror: list indices must be integers or slices, not str
This error is raised when you try to access a value inside a list using a string value.
Items in lists are indexed using numbers. Consider the following list:
0 | 1 | 2 | 3 | 4 | 5 |
S | t | r | i | n | g |
To access values in this string, we can use index slicing. This is when you call a string followed by an index value to retrieve the character at that position:
example_string = "String" print(example_string[0])
This code returns: S
. Our code retrieves the character at the index position 0 in our list.
If you try to access a list using a letter, like “S”, an error will occur.
An Example Scenario
The error “typeerror: list indices must be integers or slices, not str” is commonly raised when you try to access an item in a list of JSON objects as if it were a list.
Consider the following code snippet:
students = [ { "name": "Lisa Simpson", "age": 8 }, { "name": "Janey Powell", "age": 9 }, { "name": "Ralph Wiggum", "age": 8 } ] to_find = input("Enter the name of the student whose age you want to find: ") for s in students: if students["name"] == to_find: print("The age of {} is {}.".format(students["name"], students["age"]))
This code snippet finds the age of a particular student in a class. First, we have declared a list of students. This list stores each student as a JSON object.
Next, we ask the user to enter the name of the student whose age they want to find. Our program then iterates over each student in the list using a for loop to find the student whose name matches the one we have specified.
If that particular name is found, the age of the student is printed to the console. Otherwise, nothing happens.
Let’s try to run our code:
Traceback (most recent call last): File "main.py", line 19, in <module> if students["name"] == to_find: TypeError: list indices must be integers or slices, not str
Oh no. It’s a TypeError!
The Solution
You can solve the error “typeerror: list indices must be integers or slices, not str” by making sure that you access items in a list using index numbers, not strings.
The problem in our code is that we’re trying to access “name” in our list of students:
students["name"]
This corresponds with no value because our list contains multiple JSON objects. To solve this problem, we’re going to use a range() statement to iterate over our list of students. Then, we’ll access each student by their index number:
for s in range(len(students)): if students[s]["name"] == to_find: print("The age of {} is {}.".format(students[s]["name"], students[s]["age"]))
To start, we have used a range statement. This will allow us to iterate over every student in our list. We’ve replaced every instance of “students[“key_name”]” with “students[s][“key_name”]”. This allows us to access each value in the list.
Let’s run our code and see what happens:
Enter the name of the student whose age you want to find: Lisa Simpson The age of Lisa Simpson is 9.
Our code has successfully found the age of Lisa Simpson.
We access each value in the “students” list using its index number instead of a string. Problem solved!
Conclusion
The error “typeerror: list indices must be integers or slices, not str” is raised when you try to access a list using string values instead of an integer. To solve this problem, make sure that you access a list using an index number.
A common scenario where this error is raised is when you iterate over a list and compare objects in the list. To solve this error, we used a range()
statement so that we could access each value in “students” individually.
Now you’re ready to solve this error like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
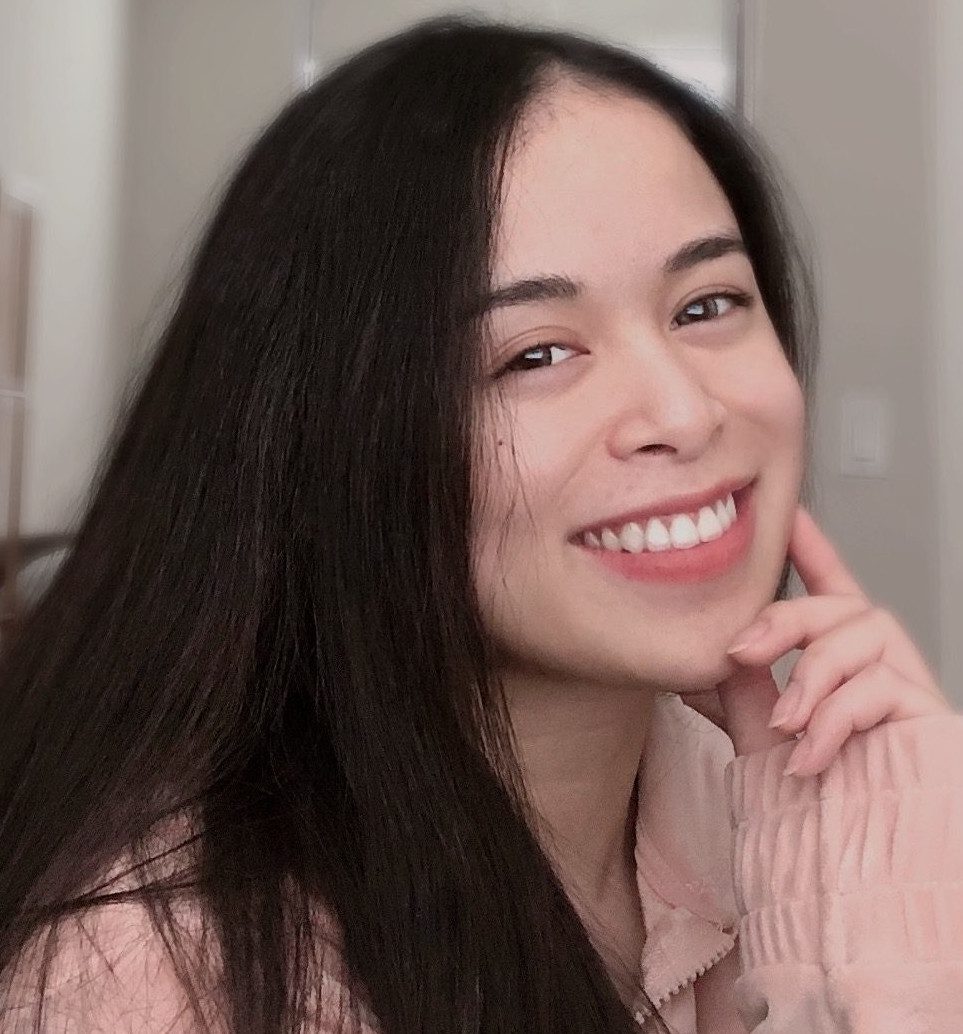
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot