Unlike iterable objects, you cannot access a value from a function using indexing syntax.
Even if a function returns an iterable, you must assign the response from a function to a variable before accessing its values. Otherwise, you encounter an “TypeError: ‘function’ object is not subscriptable” error.
In this guide, we talk about what this error means. We walk through two examples of this error so you can figure out how to solve it in your code.
TypeError: ‘function’ object is not subscriptable
Iterable objects such as lists and strings can be accessed using indexing notation. This lets you access an individual item, or range of items, from an iterable.
Consider the following code:
grades = ["A", "A", "B"] print(grades[0])
The value at the index position 0 is A. Thus, our code returns “A”. This syntax does not work on a function. This is because a function is not an iterable object. Functions are only capable of returning an iterable object if they are called.
The “TypeError: ‘function’ object is not subscriptable” error occurs when you try to access a function as if it were an iterable object.
This error is common in two scenarios:
- When you assign a function the same name as an iterable
- When you try to access the values from a function as if the function were iterable
Let’s analyze both of these scenarios.
Scenario #1: Function with Same Name as an Iterable
Create a program that prints out information about a student at a school. We start by defining a dictionary with information on a student and their latest test score:
student = { "name": "Holly", "latest_test_score": "B", "class": "Sixth Grade" }
Our dictionary contains three keys and three values. One key represents the name of a student; one key represents the score a student earned on their latest test; one key represents the class a student is in.
Next, we’re going to define a function that prints out these values to the console:
def student(pupil): print("Name: " + pupil["name"]) print("Latest Test Score: " + pupil["latest_test_score"]) print("Class: " + pupil["class"])
Our code prints out the three values in the “pupil” dictionary to the console. The “pupil” dictionary is passed as an argument into the student() function.
Let’s call our function and pass the “student” dictionary as a parameter:
student(student)
Our Python code throws an error:
Traceback (most recent call last): File "main.py", line 8, in <module> student(student) File "main.py", line 4, in student print("Name: " + pupil["name"]) TypeError: 'function' object is not subscriptable
This error is caused because we have a function and an iterable with the same name. “student” is first declared as a dictionary. We then define a function with the same name. This makes “student” a function rather than a dictionary.
When we pass “student” as a parameter into the student() function, we are passing the function with the name “student”.
We solve this problem by renaming our student function:
def show_student_details(pupil): print("Name: " + pupil["name"]) print("Latest Test Score: " + pupil["latest_test_score"]) print("Class: " + pupil["class"]) show_student_details(student)
We have renamed our function to “show_student_details”. Let’s run our code and see what happens:
Name: Holly Latest Test Score: B Class: Sixth Grade
Our code successfully prints out information about our student to the console.
Scenario #2: Accessing a Function Using Indexing
Write a program that filters a list of student records and only shows the ones where a student has earned an A grade on their latest test.
We’ll start by defining an array of students:
students = [ { "name": "Holly", "grade": "B" }, { "name": "Samantha", "grade": "A" }, { "name": "Ian", "grade": "A" } ]
Our list of students contains three dictionaries. Each dictionary contains the name of a student and the grade they earned on their most recent test.
Next, define a function that returns a list of students who earned an A grade:
def get_a_grade_students(pupils): a_grade_students = [] for p in pupils: if p["grade"] == "A": a_grade_students.append(p) print(a_grade_students) return a_grade_students
The function accepts a list of students called “pupils”. Our function iterates over that list using a for loop. If a particular student has earned an “A” grade, their record is added to the “a_grade_students” list. Otherwise, nothing happens.
Once all students have been searched, our code prints a list of the students who earned an “A” grade and returns that list to the main program.
We want to retrieve the first student who earned an “A” grade. To do this, we call our function and use indexing syntax to retrieve the first student’s record:
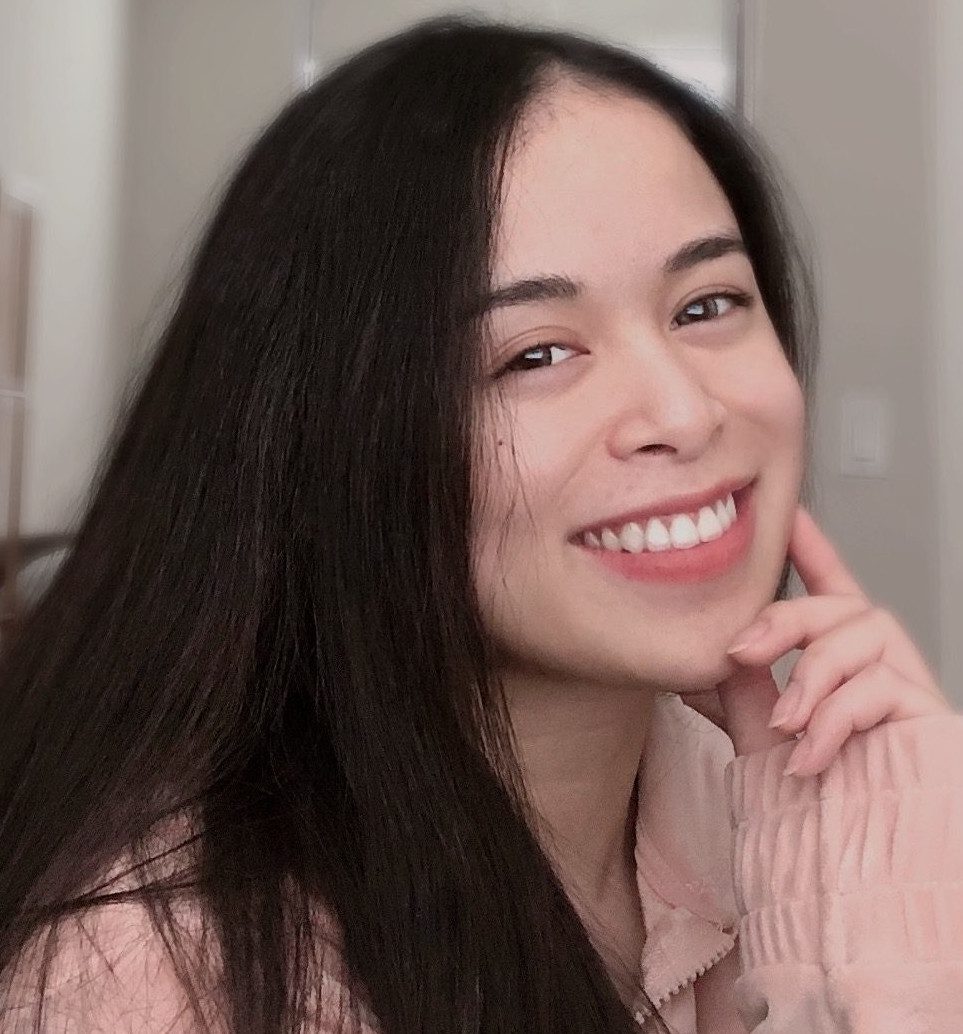
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
first = get_a_grade_students[0] print(first)
Run our code:
Traceback (most recent call last): File "main.py", line 16, in <module> first = get_a_grade_students[0] TypeError: 'function' object is not subscriptable
Our code returns an error. We’re trying to access a value from the “get_a_grade_students” function without first calling the function.
To solve this problem, we should call our function before we try to retrieve values from it:
a_grades = get_a_grade_students(students) first = a_grades[0] print(first)
First, we call our get_a_grade_students()
function and specify our list of students as a parameter. Next, we use indexing to retrieve the record at the index position 0 from the list that the get_a_grade_students()
function returns. Finally, we print that record to the console.
Let’s execute our code:
[{'name': 'Samantha', 'grade': 'A'}, {'name': 'Ian', 'grade': 'A'}] {'name': 'Samantha', 'grade': 'A'}
Our code first prints out a list of all students who earned an “A” grade. Next, our code prints out the first student in the list who earned an “A” grade. That student was Samantha in this case.
Conclusion
The “TypeError: ‘function’ object is not subscriptable” error is raised when you try to access an item from a function as if the function were an iterable object, like a string or a list.
To solve this error, first make sure that you do not override any variables that store values by declaring a function after you declare the variable. Next, make sure that you call a function first before you try to access the values it returns.
Now you’re ready to solve this common Python error like a professional coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.