Values inside a float cannot be accessed using indexing syntax. This means that you cannot retrieve an individual number from a float. Otherwise, you’ll encounter a “typeerror: ‘float’ object is not subscriptable” in your program.
In this guide, we talk about what this error means and why you may see it. We walk through two example scenarios so you can figure out how to fix this problem.
typeerror: ‘float’ object is not subscriptable
You can retrieve individual items from an iterable object. For instance, you can get one item from a Python list, or one item from a dictionary. This is because iterable objects contain a list of objects. These objects are accessed using indexing.
You cannot retrieve a particular value from inside a float. Floating-point numbers, like integers, are not iterable objects.
The “typeerror: ‘float’ object is not subscriptable” is commonly caused by:
- Trying to access a particular value from a floating-point number
- Using the wrong syntax to replace multiple items in a list
Let’s walk through each of these scenarios.
Scenario #1: Accessing an Item from a Float
We’re building a program that checks if a ticket holder in a raffle is a winner. If a user’s ticket number starts with 1 and ends in 7, they are a winner.
Let’s start by asking a user to insert a ticket number that should be checked:
ticket_number = float(input("Enter a ticket number: "))
We’ve converted this value to a float because it is a number.
Next, we use indexing syntax to retrieve the first and last numbers on a ticket:
first = ticket_number[0] last = ticket_number[-1]
The first item in our ticket number is at the index position 0; the last item is at the index position -1. Next, we use an “if” statement to check if a user is a winner:
if first == "1" and last == "7": print("You are a winner!") else: print("You are not a winner.")
If the value of “first” is equal to “1” and the value of “last” is equal to “7”, our “if” statement will run and inform a user they have won. Otherwise, our “else” statement will run, which will inform a user that they are not a winner.
Let’s run our code:
Enter a ticket number: 23 Traceback (most recent call last): File "main.py", line 3, in <module> first = ticket_number[0] TypeError: 'float' object is not subscriptable
Our code does not work. This is because ticket numbers are stored as a float. We cannot use indexing syntax to retrieve individual values from a float.
To solve this error, we remove the float()
conversion from our first line of code:
ticket_number = input("Enter a ticket number: ")
The input()
method returns a string. We can manipulate a string using indexing, unlike a floating-point value. Let’s run our code again:
Enter a ticket number: 23 You are not a winner.
Our code appears to work successfully. Let’s test our code on a winning ticket:
Enter a ticket number: 117 You are a winner!
Our code works whether a ticket is a winner or not.
Scenario #2: Replacing Multiple Items
List slicing allows you to replace multiple items in a list. Slicing is where you specify a list of items in an iterable that you want to access or change.
Let’s build a program that resets the prices of all products in a list of donuts. We must build this program because a store is doing a “Donut Day” promotion where every donut is a particular price. We start by asking the user for the new price of donuts:
price = input("Enter the price of the donuts: ")
Next, we define a list of the current prices of donuts that we must change:
donuts = [2.50, 2.75, 1.80, 1.75, 2.60]
Now that we have these values, we’re ready to change our list. We can do this using indexing:
donuts[0][1][2][3][4] = [float(price)] * 5 print(donuts)
This code resets the values in the “donuts” list at the index positions 0, 1, 2, 3, and 4 to the value of “price”. We’ve converted the value of “price” to a floating point number.
We’ve then enclosed the price in square brackets and multiplied it by five. This creates a list of values which we can assign to our “donuts” list.
Finally, we print the new list of prices to the console. Let’s run our code:
Enter the price of the donuts: 2.50 Traceback (most recent call last): File "main.py", line 3, in <module> donuts[0][1][2][3][4] = [float(price)] * 5 TypeError: 'float' object is not subscriptable
When our code tries to change the values in the “donuts” list, an error is returned. Our code tries to retrieve the item at the index position [0][1][2][3][4]. This does not exist in our list because our list only contains floats. To solve this error, use slicing syntax to update our list.
donuts[0:5] = [float(price)] * 5
This code retrieves all the items in our list from the range of 0 to 5 (exclusive of 5). Then, we assign each value the value of “price”. Let’s try our new code:
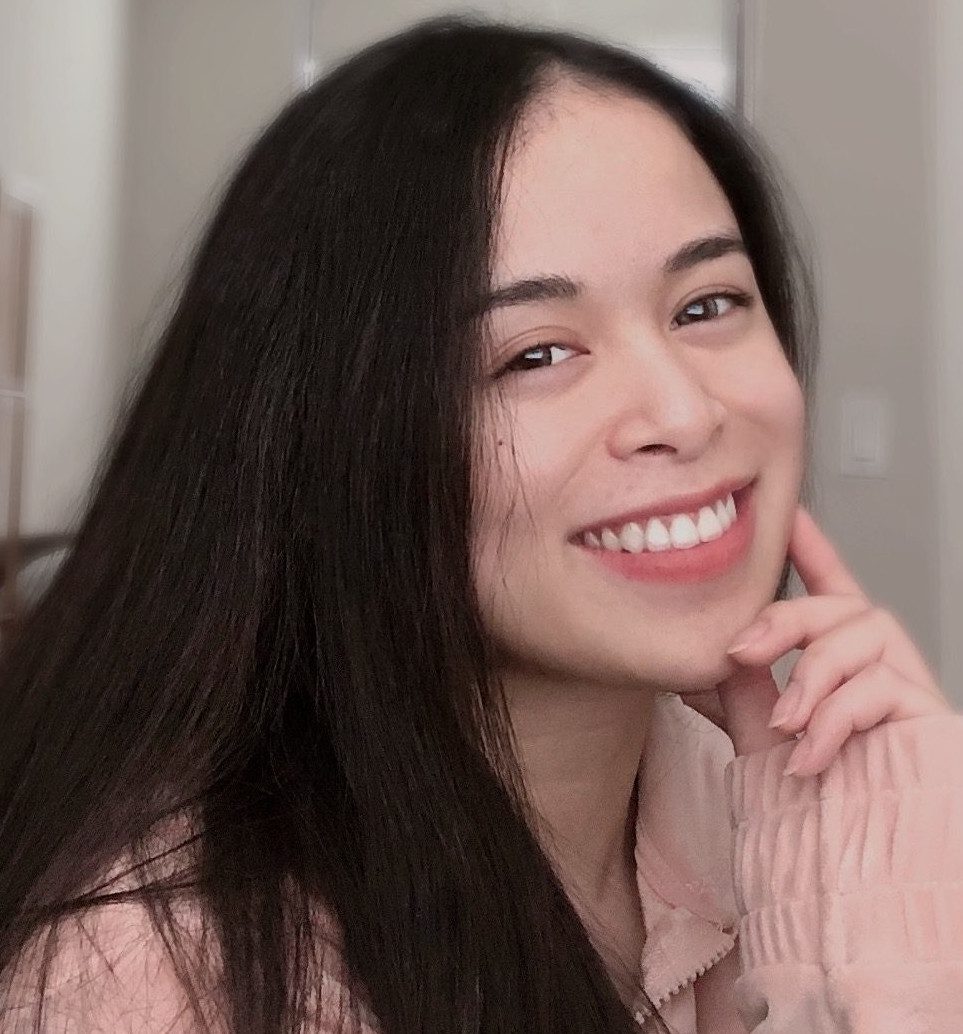
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Enter the price of the donuts: 2.50 [2.5, 2.5, 2.5, 2.5, 2.5]
Our code successfully replaces all the items in our list.
Conclusion
The “typeerror: ‘float’ object is not subscriptable” error occurs when you try to access items from a floating point number as if the number is indexed.
To solve this error, make sure you only use indexing or slicing syntax on a list of iterable objects. If you are trying to change multiple values in a list, make sure you use slicing to do so instead of specifying a list of index numbers whose values you want to change.
Now you’re ready to solve this error in your own code.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.