The join() method lets you transform an iterable object such as a list into a string. It is the opposite of the split() method. If you try to use this method to join a value that is not an iterable object into a list, you’ll encounter the “TypeError: can only join an iterable” error.
In this guide, we’re going to talk about what this error means and how it works. We’ll walk through an example of this list to help you learn how it works.
TypeError: can only join an iterable
Let’s take a look at our error message: TypeError: can only join an iterable.
TypeErrors are raised when you try to perform an operation on a value whose data type does not support that operation. The message after the error type tells us that we’re trying to join a value that is not an iterable object.
The join() method only lets you join iterables like a list or a tuple. This is because the join method traverses through every item in a sequence and adds them to a string. If a value is not iterable, this process cannot occur.
This error is common when you try to join a value that is equal to None.
An Example Scenario
Let’s build a program for a cheesemonger that orders a list of cheeses. We’ll ask a user whether they want the cheeses ordered in ascending or descending order. To start, let’s define a list of cheeses:
cheeses = ["Parmesan", "English Cheddar", "Feta", "Roquefort", "Brie"]
Next, we’re going to ask the user for the order in which they want the cheese list to appear:
order = input("Do you want the cheeses to appear in ascending (ASC) or descending (DESC) order? ")
We’re going to use an “if” statement to translate the answer a user inserts into a boolean:
if order == "ASC": reverse = False else: reverse = True
If a user inserts the value “ASC”, the list will be sorted in ascending order. Otherwise, the list will be sorted in reverse order.
Next, we’re going to use the sort() method to sort our list:
cheeses = cheeses.sort(reverse=reverse)
We refer to the “reverse” variable we defined earlier. This lets us tell the sort() method whether we want our cheeses to be sorted in ascending or descending order.
Now that we’ve sorted our cheeses, we are going to join them into a string and print them to the console:
new_cheeses = ", ".join(cheeses) print("Your sorted cheeses are: " + new_cheeses)
The join() method joins all the cheeses from the “cheeses” list into a string. Each cheese will be separated by a space and a comma. We enclosed these characters in quotation marks in our code before we called the join() method.
Let’s execute our program:
Do you want the cheeses to appear in ascending (ASC) or descending (DESC) order? ASC
Do you want the cheeses to appear in ascending (ASC) or descending (DESC) order? ASC Traceback (most recent call last): File "main.py", line 12, in <module> new_cheeses = " ".join(cheeses) TypeError: can only join an iterable
Our code returns an error.
The Solution
Our code asks the user for the order in which the list of cheeses should appear. Our code stops working on line 12. This is where we join our cheeses list into a string.
The problem is that the value of “cheeses” becomes equal to None in our code. This is because we’ve assigned the value of the sort() method to a new variable.
The sort() method sorts a list in-place. It alters an existing list. The sort() method does not return a new list. This means that when we assign the value of cheeses.sort(reverse=reverse) to a variable, the value of that variable becomes None.
To solve this problem, we’ve got to remove the variable declaration on the line of code where we sort our cheeses:
cheeses.sort(reverse=reverse)
This code will sort our list of cheeses. The value of “cheeses” will be equal to the sorted list rather than None. Let’s run our code and see what happens:
Do you want the cheeses to appear in ascending (ASC) or descending (DESC) order? ASC
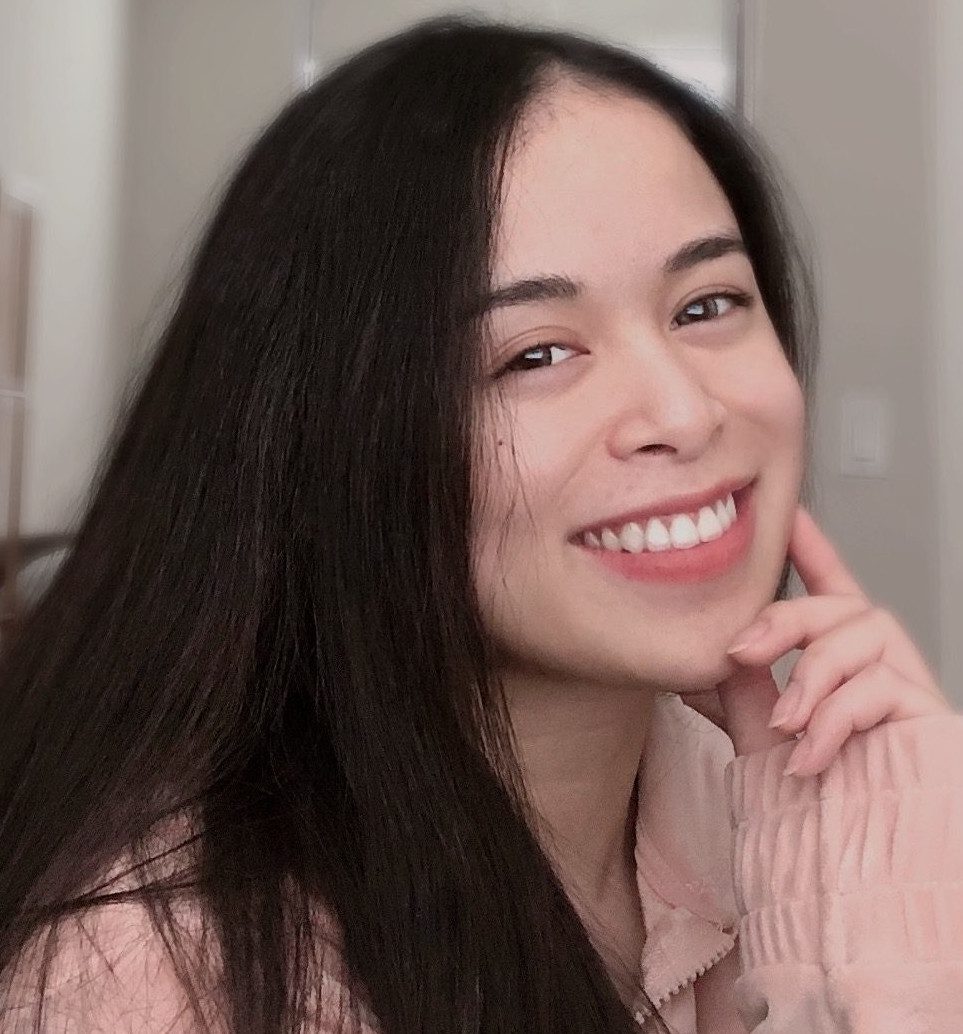
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Your sorted cheeses are: Brie, English Cheddar, Feta, Parmesan, Roquefort
Our code successfully returns a sorted list of cheeses. Let’s try to sort our cheeses in descending order:
Do you want the cheeses to appear in ascending (ASC) or descending (DESC) order? DESC
Your sorted cheeses are: Roquefort, Parmesan, Feta, English Cheddar, Brie
Our code is capable of sorting our lists both in ascending and descending order.
Conclusion
The “TypeError: can only join an iterable” error is caused when you try to join a value that is not iterable to a string.
This can happen if you assign the value of a built-in list method such as sort() to a new variable and try to join the result of that operation to a string. You can fix this error by ensuring that the value that you try to join into a string is an iterable, like a list or a tuple.
Now you’re ready to solve this error in your code like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.