The Python try…except statement catches an exception. It is used to test code for an error which is written in the “try” statement. If an error is encountered, the contents of the “except” block are run.
How to Use Try and Except in Python
You may want to test a specific block of code to ensure it functions correctly before allowing the rest of the program to run. For example, say you have written a large amount of new code for a program. You would want to make sure it works before letting the rest of the program run.
try…except blocks let you test your code and handle an exception if one is raised. You can add finally and else statements to run additional code depending on the outcome of the try…except block.
In this tutorial, we are going to talk about how to use try and except in Python. We’ll refer to an example so you can quickly get started using try and except.
Python Errors and Exceptions Refresher
In Python, there are two kinds of errors you may encounter: syntax errors and exceptions.
Python syntax errors are a type of error that returns when you use the wrong syntax. For example, if you write a while True loop without a colon at the end, the program will report an error.
When syntax errors occur, they return the file name, line number, and an indicator of where an error may be present.
Exceptions are a type of error where code may have the right syntax but still contains a problem. There are many types of exception, but some of the most common you will encounter include: ArithmeticError, ImportError, ZeroDivisionError, NameError, and TypeError.
Python try…except Statement
The Python try…except statement runs the code under the “try” statement. If this code does not execute successfully, the program will stop at the line that caused the error and the “except” code will run.
The try block allows you to test a block of code for errors. The except block enables you to handle the error with a user-defined response.
Here is the syntax for the try…except block:
try: yourcode... except: yourcode...
You can enclose any valid Python code within a try or except statement.
try…except Python Example
Here’s an example of the syntax for try…except blocks:
try: print(ourVariable) except: print('Error returned')
In the above example, we have not declared the Python variable ourVariable, yet we try to use it in our try block.
If we did not have try…except blocks in our code, the program would return an error message. While seeing an error message is fine during debugging, a regular user may get confused if they see an error message.
Because we have try…except blocks, our code knows what to do when an error is encountered.
Here is the result of our code:
Error returned
try…except blocks let you handle exceptions gracefully. You may also want to implement a feature like saving an exception to a log file using a package like Python’s logging module. This would let you keep track of exceptions that have been raised.
try…except Python: Multiple Except Statements
You can repeat except statements for different types of errors to test for multiple exceptions. This is useful if you suspect that one of many exceptions may be raised but you are not sure which one you will encounter.
Here is an example of try…except blocks that look for a NameError:
try: print(ourVariable) except NameError: print('ourVariable is not defined') except: print('Error returned')
In this case, our code returns ourVariable is not defined because our code returns a NameError. We could add more errors, such as a ZeroDivisionError or an OSError, depending on the code we are testing.
For instance, you may check for an IOError and a FileNotFoundError if you want to open a file. Checking for multiple exceptions would ensure your program could continue running even if there was an error opening the file you reference.
try…except Python: Finally
But what if we want a message to print both if an error is returned and if no error is found? That’s where the finally block comes in. If you define a finally clause, its contents will be executed irrespective of whether the try…except block raises an error.
Finally blocks are a useful indicator that you code has executed. Because they do not differentiate between whether a code has successfully executed, they are not as commonly used.
Here’s an example:
try: print(ourVariable) except: print('ourVariable is not defined') finally: print('Code has been run.')
Our program returns the following:
ourVariable is not defined Code has been run.
The code within the except block executes because there is an exception found in our code (ourVariable is not defined). The code within the finally clause executes as well, because our code has finished running.
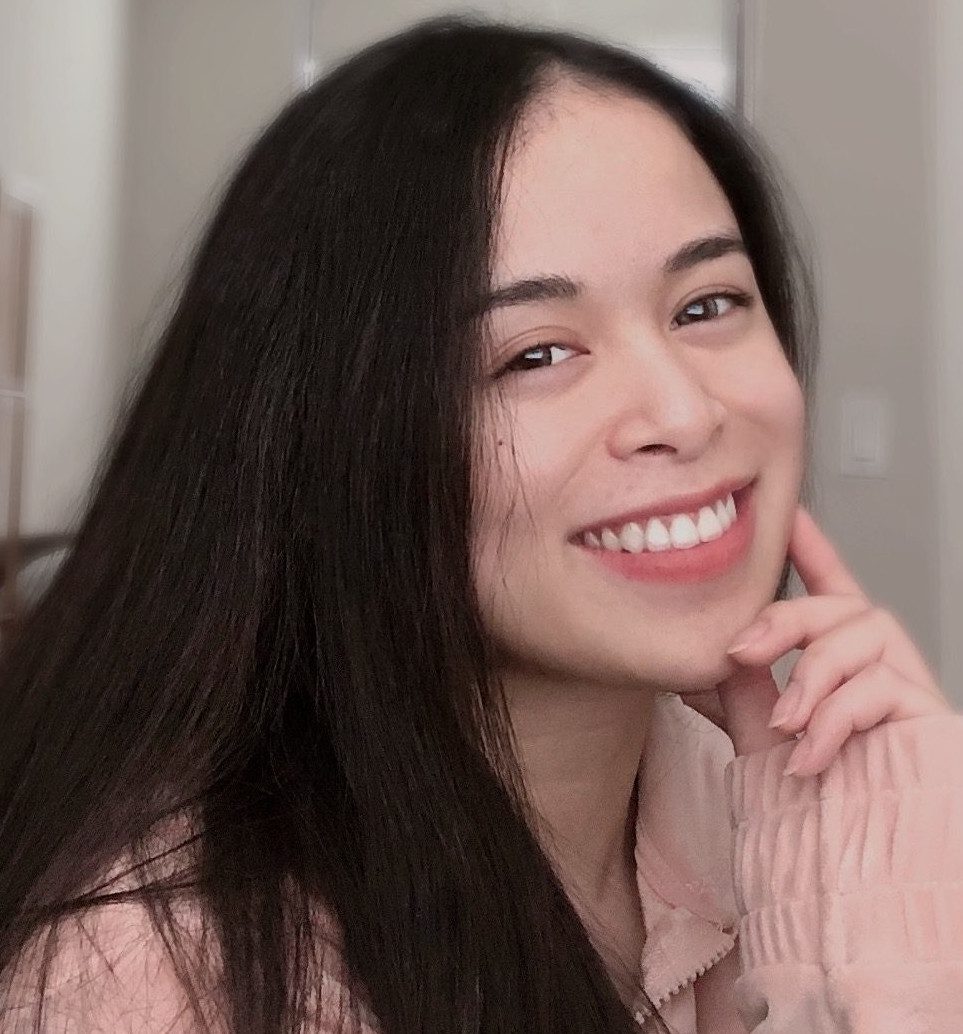
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
try…except Python: Else
By using an else clause, you can define code that will be run in the case that no exceptions are raised. This could be used to inform a user that a program has successfully executed, for instance.
Imagine if you were creating a sign up system for a game. You may include a try…except…else block to check if a username or email address that a user selects is valid. If it is not, the except clause would run. If the username or email address is valid, the else block could run.
Here’s an example:
try: print('Test') except: print('There is a problem.') else: print('There are no problems.')
Our code returns the following:
There are no problems.
Our Python program encounters no exceptions. As a result, the code within the else statement executes. The else statement prints out the message stating there are no problems with our code.
View the Repl.it from this tutorial:
Conclusion
try…except blocks make it easy to debug your Python code. A program tries to run the code in a “try” block. If this fails, the “except” block runs. The code in a “finally” statement runs irrespective of whether an “except” block is executed.
In this tutorial, we have broken down how to use try…except blocks. We have discussed how to use else and except to customize your exception handling.
These blocks can be useful when you’re testing existing code or writing new code. It ensures that your program runs correctly and contains no errors.
For more Python learning resources, check out our comprehensive How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.