The Python time module represents time-based objects in Python. Developers use time() function returns the current time as a UNIX timestamp. The ctime() function translates a UNIX timestamp into a standard year, month, day, and time format.
Working with time is an essential part of many programs. For instance, suppose you’re building a sign-up form for a website. You may want to retrieve the current time so you know when a user signed up for your site.
That’s where the Python time module comes in. The time module allows you to work with time in your Python programs. You can perform actions like retrieving the current time and creating a pause in the program.
This tutorial will discuss, with reference to examples, the basics of the Python time module and how to use it.
Importing the Python Time Module
The time module has a number of time-related functions you can implement in your Python programs. You can use the time module in all versions of Python.
Before we discuss how to use the time module, we first need to import it into our program. We can do so using a Python import statement:
import time
time() Python Function
The Python time() function retrieves the current time. The time is represented as the number of seconds since January 1, 1970. This is the point at which UNIX time starts, also called the “epoch.”
Suppose we want to retrieve the time at this moment. We could do so using this program:
import time current_time = time.time() print(current_time)
Our code returns:
1586944173.957986
Notice that our program did not return a regular time, such as 10:30 A.M. Our program returned a floating-point number (in other words, a decimal number).
This floating-point number is the number of seconds since the epoch started. We printed the time to the console using a print statement.
In programming, epoch time is a standard way of representing time. The time module does not have to account for time zones.
This is because epoch time only stores the number of seconds since the aforementioned date and time. No timezone data is stored, like Coordinated Universal Time, alongside an epoch value.
Python Get Current Time Using ctime()
Epoch time is not readable to most humans. You can concert the time from epoch to local time using the Python ctime() function.
The ctime() function accepts one argument. This argument is the number of seconds since the epoch started and returns a string with the local time. This value is based on the computer’s time zone.
Suppose we want to convert our previous epoch floating-point number into a timestamp. We could do so using this program:
import time epoch_time = 1586944173.957986 local_time = time.ctime(epoch_time) print("The local time is:", local_time)
Our code returns the time:
The local time is: Wed Apr 15 09:49:33 2020
First, we declared a Python variable called epoch_time. This variable stores our epoch timestamp.
Then, we used time.ctime() to convert the timestamp into local time. On the next line of our code, we printed the statement The local time is:, followed by the local time. Also, the comma between The local time is: and the local_time variable adds in a space between the two values.
Python time sleep Function
One of the most common functions in Python’s time library is the time.sleep() function. time.sleep() suspends the execution of a program for a specified number of seconds.
Suppose we want to print out the phrase “Logging out … ”, then wait three seconds before printing “Logout successful!” to the console. We could do so using this code:
import time print("Logging out … ") time.sleep(3) print("Logout successful!")
Here’s how our program works:
- “Logging out … ” is printed to the console.
- The program pauses for 3 seconds.
- “Logout successful!” is printed to the console.
The time.sleep() function accepts floating-point (decimal) or integer (whole) number values. if you want a program to pause for 3.7 seconds, you can do so by specifying 3.7 as a parameter. In our above example, we specified 3.
Python struct_time Object
The Python time library’s struct_time odatetime bject structures non-epochal timestamps. The struct_time object is useful because it allows you to work with a standardized form when you’re using timestamps.
Here are the values the struct_time object can store:
- tm_year: year
- tm_mon: month
- tm_mday: day of the month
- tm_hour: hour
- tm_min: minute
- tm_sec: second
- tm_wday: day of the week (0 is Monday)
- tm_yday: day of the year
- tm_isdst: time in daylight savings time
We can access all of these attributes through a struct_time object. We employ struct_time objects in our examples below.
Using the Python structime_object
Python time.localtime()
Now, suppose we want to convert epoch time to local time and have the outcome return a struct_time object. We could do so using either the localtime() or gmtime() functions.
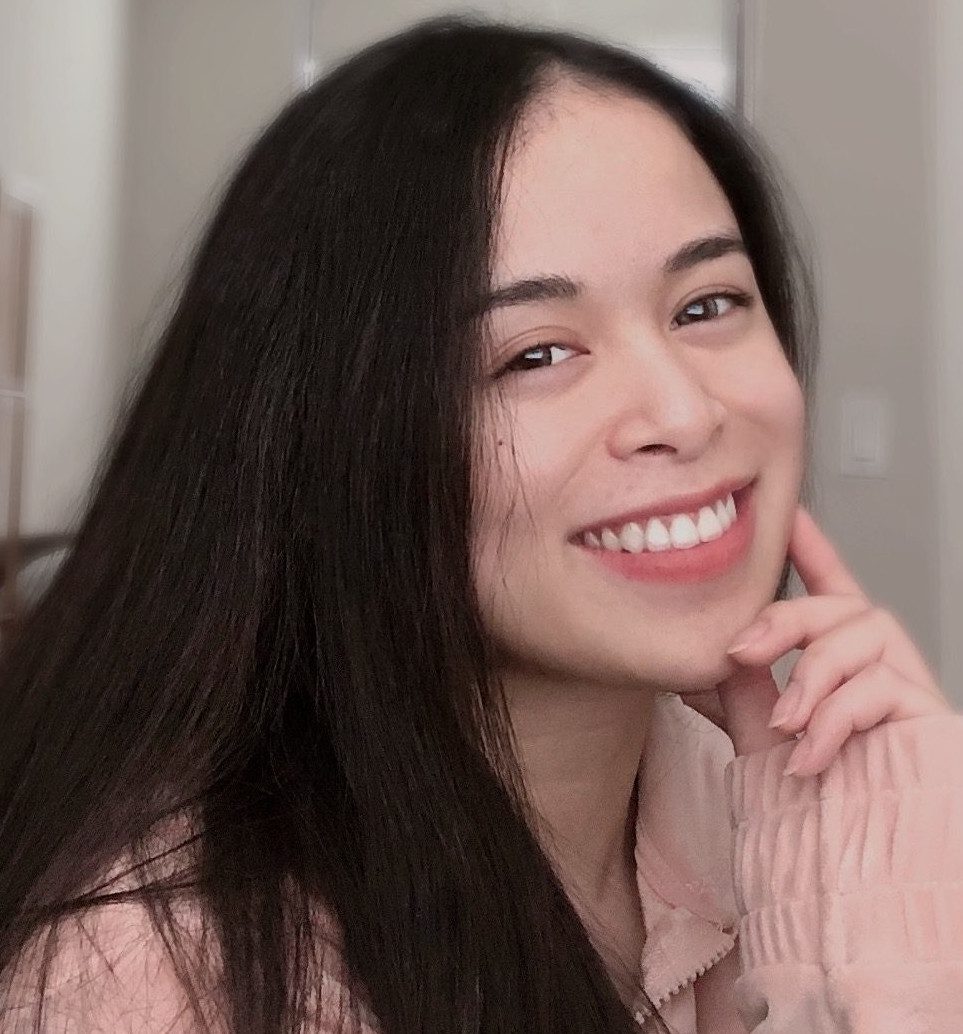
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The localtime() function accepts an epoch time and returns a struct_time object.
Suppose we want to get the current day of the week and minute of the hour. We could do so using this program:
import time current_time = time.localtime(1586944173.957986) print("Day of the week:", current_time.tm_wday) print("Minute of the hour:", current_time.tm_min)
Our code returns:
Day of the week: 2 Minute of the hour: 49
First, we imported the time library. Then, we used localtime() to convert our epoch timestamp from earlier to a struct_time object.
On the next line, we printed the statement Day of the week: to the console. We then printed the day of the week (which is stored in the tm_wday value of our current_time object).
Next, we printed the statement Minute of the hour: to the console. We followed this statement with the current minute of the hour (stored in the tm_min value of our current_time object).
Python time.gmtime()
The gmtime() function works the same way as the localtime() function does, returning a struct_time object from an epoch time.
Suppose we want to find out the month of the year and the day of the month. We could do so using this program:
import time current_time = time.gmtime(1586944173.957986) print("Month of the year:", current_time.tm_mon) print("Day of the month:", current_time.tm_mday)
Our code returns:
Month of the year: 4 Day of the month: 15
In our code, we used gmtime() instead of localtime() to generate a struct_time object. We assigned the value of the gmtime() method to the variable current_time.
Next, we printed the Python string Month of the year: to the console, followed by the current month of the year. This value is stored in the tm_mon value in our current_time object.
Then, we printed Day of the month: to the console, followed by the current day of the month. This value is in the tm_mday value in the current_time object.
Python time.asctime()
The asctime() function convertsa struct_time object into a formatted string. Consider the following example:
import time current_time = time.localtime(1586944173.957986) formatted_time = time.asctime(current_time) print("The current time is:", formatted_time)
Our program returns:
The current time is: Wed Apr 15 09:49:33 2020
In this example, we use the localtime() function to convert epoch time into a struct_time object. Then, we use asctime() to convert our struct_time object into a fully-formatted string representing the current time.
This string is assigned to the variable formatted_time. Then, we print the statement The current time is:, followed by the formatted time.
time.strftime()
The strftime()
function formats the values in a struct_time
object to a particular string.
For instance, suppose we want to turn a struct_time object into a string with the following format: MONTH/DAY/YEAR. We could do so using this code:
import time current_time = time.localtime() final_time = time.strftime("%m/%d/%Y", current_time) print(final_time)
Our code returns:
04/15/2020
We imported the time library. Then, we used localtime() to generate a struct_time object reflecting the current time. We then used strftime() to convert our struct_time object into a string that shows the month, day, and year.
Lastly, we printed out the value of the final_time array. This array stores our formatted time.
In this example:
- %m denotes the month
- %d denotes the day of the month
- %Y denotes the year
Here are a few other values you could use with the strftime function:
- %H denotes the hour of the day
- %M denotes the minute of the hour
- %S denotes the second of the minute
Conclusion
The Python time module allows you to work with time in your Python code. The module comes with a number of different functions.
You can use these to retrieve the current time, convert time between epoch time and more readable values, pause a program, format the current time, and perform other tasks.
Read our guide on How to Code in Python for tips and tricks on how to advance your knowledge of the Python programming language.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.