The Python ternary operator lets you evaluate whether a condition is true or false. The ternary operator takes up one line of code, which means it is shorter and more concise than a full if…else statement.
Conditional statements, such as if statements, allow you to control the flow of your program. The code inside conditional statements only runs when a particular condition is (or set of conditions are) met.
In Python, the most common way to write a conditional statement is to use if. However, the language also offers a way to test for a condition on one-line: the ternary operator.
This tutorial will discuss, with reference to examples, the basics of conditional statements, and how to use the Python ternary operator.
Python Conditionals
When you’re writing a program, you may only want a line or block of code to be executed when a condition is met. This is where conditional statements are useful.
The Python if statement is used to check whether a condition is met.
Suppose we are building an app that checks whether a customer is eligible for a 10% discount at a movie theater. If the customer is aged 65 or over, they should be given a discount, otherwise, no discount should be given. We could build this program using an if…else statement.
But, if statements take up at least two lines of code. There is a more concise way of writing an if statement if you are only evaluating a few conditions: the ternary operator in Python.
Python Ternary Operator
The ternary operator is a type of conditional expression in Python that evaluates a statement. Ternary operators perform an action based on whether that statement is true or false. They are more concise than a traditional if…else statement.
The syntax for the Python ternary statement is as follows:
[if_true] if [expression] else [if_false]
The ternary conditional operator in Python gets its name from the fact it takes in three parameters: if_true, expression, and if_false.
Ternary operators are usually used to determine the value of a variable. The variable takes on the value of “if_true” if the statement evaluates to True or “if_false” if the statement evaluates to false.
One way to think of ternary operators is as what a Python list comprehension is to filtering out a list. Or, you could think of what a lambda function is to defining a function.
Both list comprehensions and lambda functions are more efficient ways of performing an action (filtering lists and defining a function, respectively). This is just like how a ternary operator is a more efficient way of writing an if statement.
But, like ternary operators, they should only be used to improve the readability of your code. You should not go overboard with ternary operators otherwise your code may be harder to read.
Ternary Operator Python Example
Suppose we want to give a customer at our movie theater a discount if they are 65 or older. If a customer is not 65 or older, they are not entitled to a discount. We could check if a customer is eligible to receive a discoutn using the following code:
age = 48 discount = True if age >= 65 else False print(discount)
Our code returns: False.
On the first line, we declare a Python variable called age. This variable is assigned the value 48. Next, we use a ternary operator to calculate whether the customer is eligible for a discount.
Our ternary operator evaluates the expression age >= 65. Because age is equal to 48, this evaluates to False. So, the code that appears after the else statement is executed.
Then, we print the result of our ternary operator to the console. This returns the value False.
If we compare this example to our last one, you can see that it uses significantly fewer lines of code. Our first program used five lines of code, and this one used three.
In this example, our ternary operator returns a Python boolean value (True or False). However, we could allow our ternary operator to return any value.
Ternary Python with Numerical Values
Suppose we want to set a specific discount rate depending on whether the user is eligible for a discount. By default, our movie theater is giving all customers a 5% discount, but seniors (people 65 or older) are eligible for a 10% discount.
The following program allows us to check whether a customer is eligible for the senior discount. If a customer is not eligible for the senior discount, they are given a 5% discount:
age = 22 discount = 5 if age < 65 else 10 print(discount)
Our code returns: 5. In our code, we assign the value 22 to the variable age.
We use a ternary operator to check if the value of the age variable is less than 65. Because our customer is 22, the statement age < 65 evaluates to True. This means that the customer is given the 5% discount rate. If our customer was 65 or older, they would receive the 10% discount rate.
Then, we print the value of the discount variable to the console.
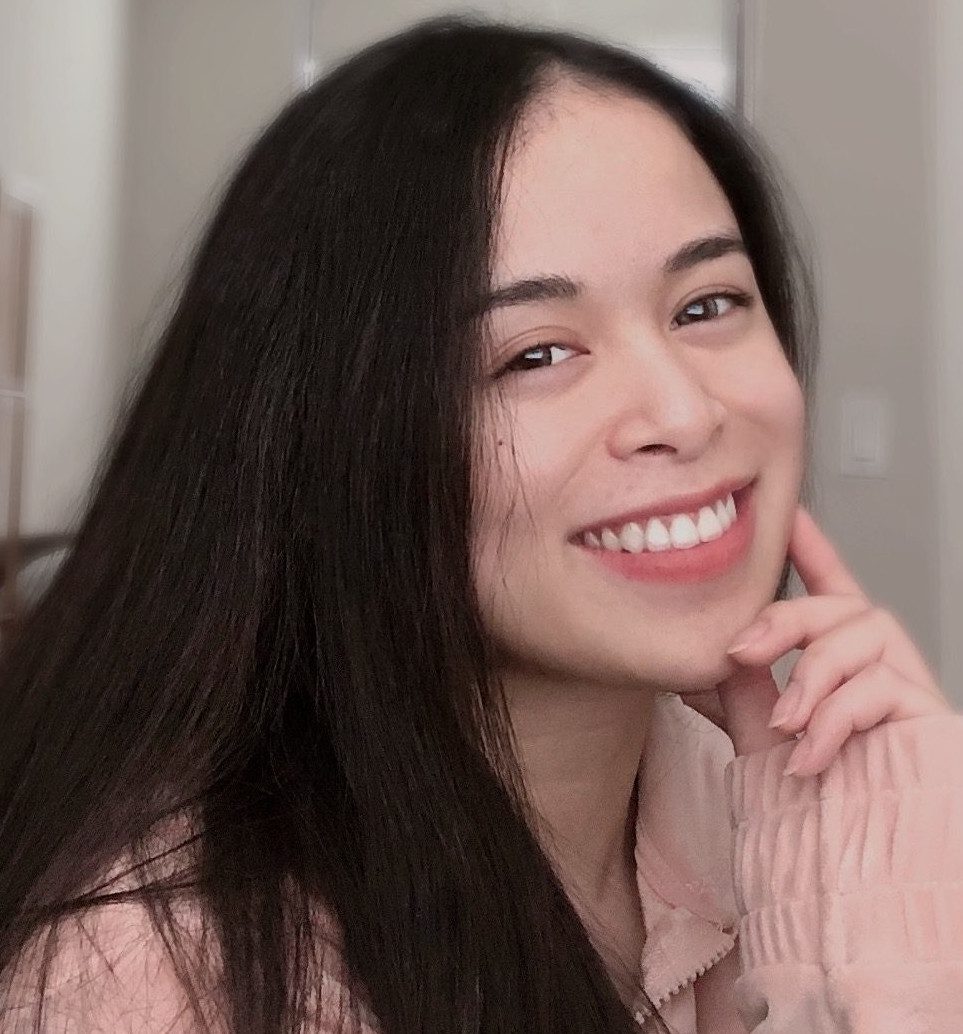
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The Python ternary operator is a more efficient way of performing simple if statements. The ternary operator evaluates a condition, then returns a specific value depending on whether that condition is equal to True or False.
In our examples above, we were able to run an if statement on one line. This is more concise than the multiple lines it would typically take to create an if statement.
However, the ternary operator cannot always be used. If you want to test for multiple expressions, you should write out a full if statement. This will ensure that your code is readable and easy to understand.
This tutorial discussed, with reference to examples, the basics of the Python ternary operator and how it works. Now you have the knowledge you need to use this operator like a Python pro!
To learn more about coding in Python, read our complete guide on How to Learn Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.