Even the best developers make syntax errors all the time when they’re coding. Programming languages have so many rules and even one typo can cause an error.
If you’ve encountered the error “syntaxerror: EOL while scanning string literal”, don’t worry. In this guide, we’re going to talk about what this error means and how to solve it. We’ll walk through a few example scenarios to help you identify potential causes and solutions.
Let’s begin!
The Problem: syntaxerror: EOL while scanning string literal
Syntax is like the grammar of a programming language. English has rules that govern punctuation and spelling; programming languages have similar rules.
Let’s take a look at our error:
syntaxerror: EOL while scanning string literal
The SyntaxError message tells us that we’ve not followed the syntax rules of Python.
The description of the error indicates that Python is expecting a particular character to appear by the end of a line of code that was not found. For instance, Python may be expecting a string close (”) character by the end of a line in which you have opened a string.
If a syntax error is encountered, Python stops executing a program. This is because the Python interpreter needs you to rectify the issue before it can read the rest of your code.
This error is commonly caused by:
- Strings that span multiple lines using the wrong syntax
- Missing quotation marks
- Mismatching quotation marks
Example Scenario: Multi-line Strings
In Python, strings can span multiple lines. The syntax for a multi-line string is different to that of a traditional string. Multi-line strings must be triple quoted, or written using three quotation marks.
Let’s take a look at a multi-line string:
def welcome_hero(): message = "Welcome, Hero! You have just entered the Castle of Doom. Your challenge, should you choose to accept it, is to locate the Sacred Emerald and take it back to the travelling salesman." print(message) welcome_hero()
We have defined a function called welcome_hero()
. This function prints a message to the console. This message is assigned to the variable “message”.
Let’s try to run our code:
File "main.py", line 2 message = "Welcome, Hero! ^ SyntaxError: EOL while scanning string literal
An error is returned. This is because a string using single or double quotes cannot span multiple lines. To solve this problem, we need to enclose our string with three single or double quotes. Any text that appears between these characters will be part of the string:
message = """Welcome, Hero! You have just entered the Castle of Doom. Your challenge, should you choose to accept it, is to locate the Sacred Emerald and take it back to the travelling salesman."""
Let’s try to run our code with this revised line. Our code returns:
Welcome, Hero! You have just entered the Castle of Doom. Your challenge, should you choose to accept it, is to locate the Sacred Emerald and take it back to the travelling salesman.
Success! Our code prints the message without an error.
Example Scenario: Missing Quotation Mark
Strings must be closed after the contents of a string have been declared. Otherwise, Python returns a syntax error. Let’s take a look at a string that is not closed:
def welcome_hero(): message = "Welcome, Hero! print(message) welcome_hero()
Let’s run our code:
File "main.py", line 2 message = "Welcome, Hero! ^ SyntaxError: EOL while scanning string literal
We have forgotten to close our string. If you look at the line of code where we declare the “message” variable, there is no closing string character.
We can fix this error by closing our string using the same quotation mark that we used to open our string.
def welcome_hero(): message = "Welcome, Hero!" print(message) welcome_hero()
Let’s run our code again:
Welcome, Hero!
Our code runs successfully.
Example Scenario: Mismatching Quotation Marks
The type of quote you use to open a string should be the same as the type of quote you use to close a string.
A syntax error is returned when the types of quotes that you use to open and close a string are different. Let’s take a look at a program that opens a string using a single quote mark (‘) and closes a string using a double quote mark (”):
def welcome_hero(): message = 'Welcome, Hero!" print(message) welcome_hero()
Our code returns:
File "main.py", line 2 message = 'Welcome, Hero!" ^ SyntaxError: EOL while scanning string literal
We can fix this problem by making our quotations match. We’re going to change our first quotation mark to use double quotes (“):
def welcome_hero(): message = "Welcome, Hero!" print(message) welcome_hero()
Our code now runs successfully: Welcome, Hero!
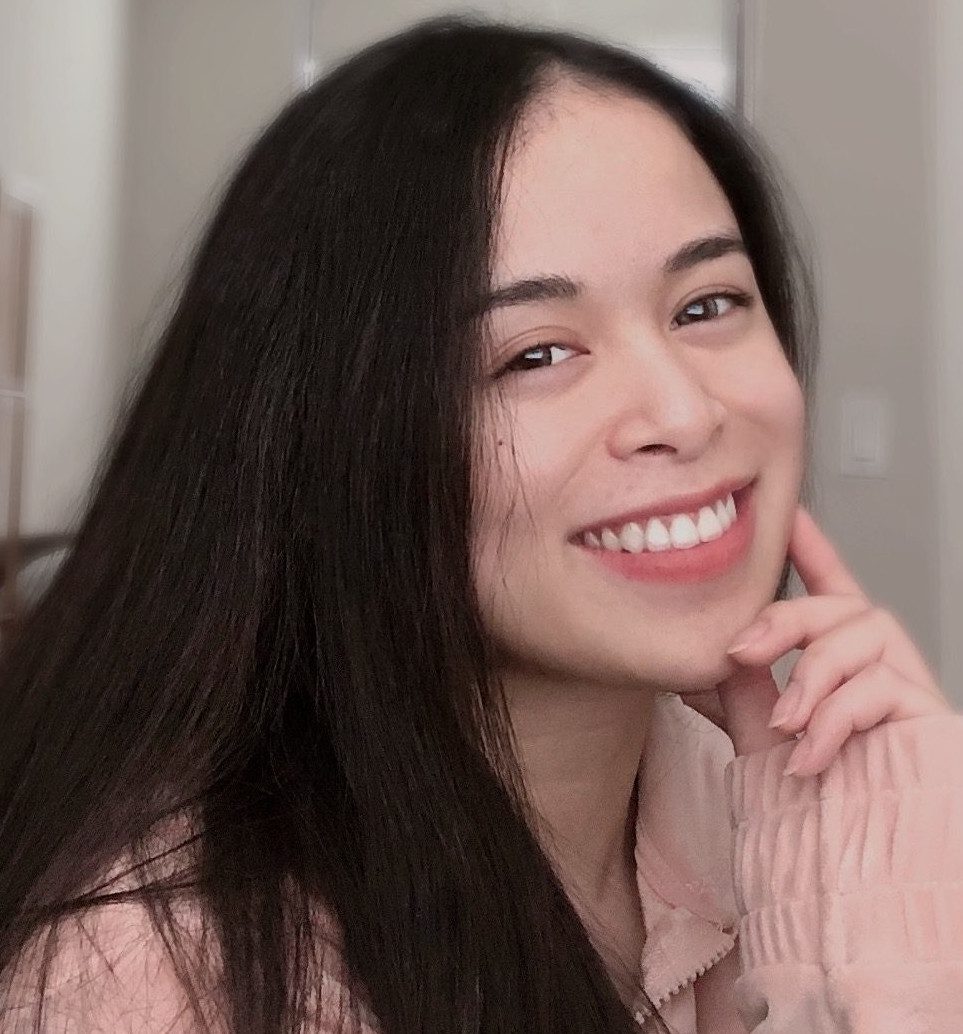
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The “syntaxerror: EOL while scanning string literal” error is experienced by every Python developer. This error happens when:
- You forget to close a string
- You close a string using the wrong symbol
- You declare a multi-line string using one quotation mark instead of three
To solve this error, check if any of the above conditions are true. Then, make the requisite changes to your code. Now you’re ready to start solving this error like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.