To assign the result of a function to a variable, you must specify the variable name, followed by an equals sign, followed by the function you want to call. If you do not follow this order, you’ll encounter a “SyntaxError: can’t assign to function call” error.
This guide discusses what this error means and why it is raised. We’ll walk through an example of this error to help you understand how you can fix it in your code.
SyntaxError: can’t assign to function call
Python variables are defined using the following syntax:
favorite_cheese = “Stilton”
The name of a variable comes first. The variable name is followed by an equals sign. After this equals sign, you can specify the value a variable should hold.
If you read this out loud as if it were a sentence, you’ll hear:
“Favorite cheese is equal to Stilton.”
You must follow this order when you declare a variable. You cannot declare a variable by specifying the value first and the name of a variable last.
The SyntaxError: can’t assign to function call
error occurs if you try to assign a value to a function call. This means that you’re trying to assign a value to a function instead of trying to assign a function call to a variable.
An Example Scenario
We’re building a program that creates a list of all the sandwiches that have been sold more than 1,000 times at a shop in the last month.
To start, define a list of sandwiches:
sandwiches = [ { "name": "Egg Salad", "sold": 830 }, { "name": "Ham and Cheese", "sold": 1128 }, { "name": "Cheese Supreme", "sold": 1203 }, { "name": "Tuna Mayo", "sold": 984 } ]
Our list of sandwiches contains four dictionaries. An individual dictionary contains information about one sandwich. Next, we define a function that calculates which sandwiches have been sold more than 1,000 times:
def sold_1000_times(sandwiches): top_sellers = [] for s in sandwiches: if s["sold"] > 1000: top_sellers.append(s) return top_sellers
This function accepts one argument: a list of sandwiches. We iterate through this list of sandwiches using a for loop. If a sandwich has been sold more than 1000 times in the last month, that sandwich is added to the 1000_sales
list. Otherwise, nothing happens.
Next, we call our function and assign the value it returns to a variable:
sold_1000_times(sandwiches) = top_sellers print(top_sellers)
We’ve assigned our function call to the variable top_sellers
. We then print that value to the console so we can see the sandwiches that have been sold more than 1,000 times.
Let’s run our code:
File "main.py", line 17 sold_1000_times(sandwiches) = top_sellers ^ SyntaxError: cannot assign to function call
We receive an error message.
The Solution
Our code does not work because we’re trying to assign a value to a function call.
When we want to assign the response of a function to a variable, we must declare the variable like any other. This means the variable name comes first, followed by an equals sign, followed by the value that should be assigned to that variable.
Our code tries to assign the value top_sellers
to a variable called sold_1000_times(sandwiches)
:
sold_1000_times(sandwiches) = top_sellers
This is invalid syntax. To solve this error, we need to reverse the order of our variable declaration:
top_sellers = sold_1000_times(sandwiches)
The name of our variable now comes first. The value we want to assign to the variable comes after the equals sign. Let’s run our program:
[{'name': 'Ham and Cheese', 'sold': 1128}, {'name': 'Cheese Supreme', 'sold': 1203}]
Our code returns a list of all the sandwiches that have been sold more than 1,000 times.
Conclusion
The “SyntaxError: can’t assign to function call” error is raised when you try to assign a function call to a variable.
This happens if you assign the value of a function call to a variable in reverse order. To solve this error, make sure you use the correct syntax to declare a variable. The variable name comes first, followed by an equals sign, followed by the value you want to assign to the variable.
Now you’re ready to solve this error in your own Python programs!
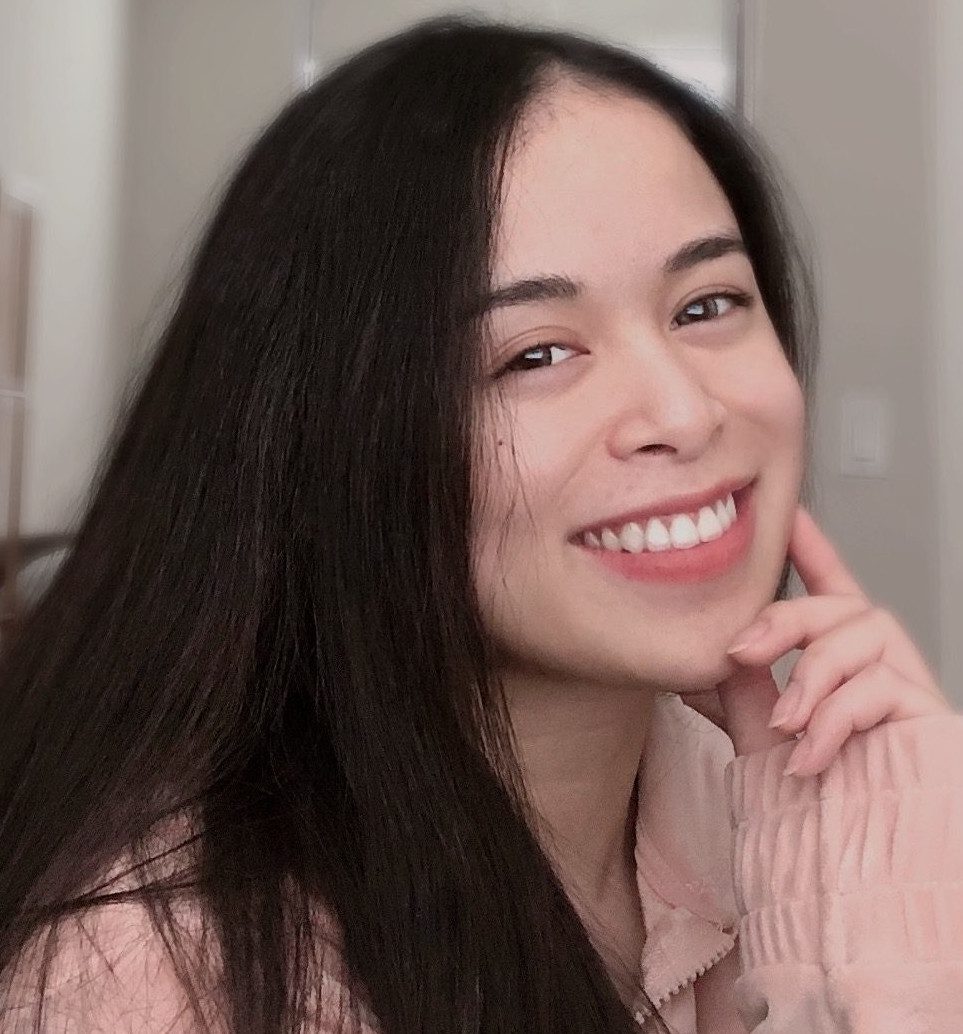
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.