Unlike Java or C#, Python does not have a built-in switch statement. This means you cannot evaluate a switch expression without having to write your own code that mimics a “switch…case” statement.
In this guide, we discuss how to write a “switch…case” in Python, and walk through two examples of a faux-switch statement so you can learn how to write one in your code.
Switch Statements: A Refresher
A switch statement lets you select one of a set of code blocks to run. They are a way of evaluating multiple expressions in a program.
A switch statement works by evaluating a switch statement and comparing the result of that statement with values in “case” statements. If a match is found, the respective block of code is run. Otherwise, nothing will happen.
Optionally, a “default” keyword is used to run a block of code if no statement is found that matches a particular expression.
Python Switch Statement: Returning Individual Values
Let’s write a program that converts a numerical value to a day of the week.
To start, ask a user to insert a number they want to convert into a written day:
convert_to_day = int(input("Insert a day of the week: "))
We convert the value the user inserts into our program to an integer. We’ll discuss why this is necessary later in the tutorial.
Next, we define a dictionary. This dictionary will contain all the days of a week. Each key will store a day as a number and each value will store a day written out (i.e. Tuesday):
days = { 1: "Monday", 2: "Tuesday", 3: "Wednesday", 4: "Thursday", 5: "Friday", 6: "Saturday", 7: "Sunday" }
There are seven keys and values in our dictionary. The key “1” represents “Monday” and so on until the final day of the week.
Next, we use the dictionary get() method to access the item in this dictionary that corresponds with the value a user has inserted:
day_as_written = days.get(convert_to_day, "There is no day with this numerical value.") print(day_as_written)
We’ve converted the value a user inserted into our program to an integer so we can use it in our get()
statement. If there is no key equal to the value that “convert_to_day” stores, our code will return “There is no day with this numerical value.”
If a user inserts the value “1”, our code will evaluate:
days[1]
This will retrieve the value associated with the key “1” in our list.
Let’s run our code and see what happens:
Insert a day of the week: 1 Monday
Our code successfully converts the number a user inserts to a string. Let’s run our code on a day that does not exist in our dictionary:
Insert a day of the week: 8 There is no day with this numerical value.
Our code works even if a user inserts an invalid value.
Python Switch Statement: Calling Functions
We can use this syntax to call functions in our code. We’re going to build an application that displays information about a list of purchases at a sandwich store.
First, let’s define a list of purchases:
purchases = [2.50, 2.50, 2.75, 3.90, 5.60, 2.40]
Next, define three functions. These functions will calculate:
- The number of purchases in our list
- The average purchase value
- The largest purchase
Let’s define these functions:
def number_of_purchases(): total = len(purchases) print(total) def average_value(): average = sum(purchases) / len(purchases) print(average) def largest_purchase(): largest = max(purchases) print(largest)
Now that we have defined these functions, start to write our faux-switch statement. Like we’ve done in our first example, we start by asking a user to insert a value. The value a user inserts should correspond to one of our functions.
print("[1] Display the number of purchases made today") print("[2] Display the average value of all the purchases made today") print("[3] Display the largest purchase made today") to_run = int(input("What would you like to do? "))
Our code prints out three messages. Each one informs a user of an option they can take. Our code then asks a user to insert a value. We convert this value to a number so we can use it later in our program.
Next, define a dictionary that maps our functions to numbers:
options = { 1: number_of_purchases, 2: average_value, 3: largest_purchase }
The value “1” is mapped to the function called “number_of_purchases”, the value “2” is mapped to the function “average_value”, and the value “3” is mapped to the “largest_purchase” function.
Next, use the get()
method to select which function our program should run:
function_to_execute = options.get(to_run) function_to_execute()
This code retrieves the function from our dictionary that we want to execute. We haven’t specified a second value in our get()
method because we need to call a function for our code to work. This means if a user inserts an invalid value, our code returns an error. There are ways to handle this behavior but doing so is outside the scope of this tutorial.
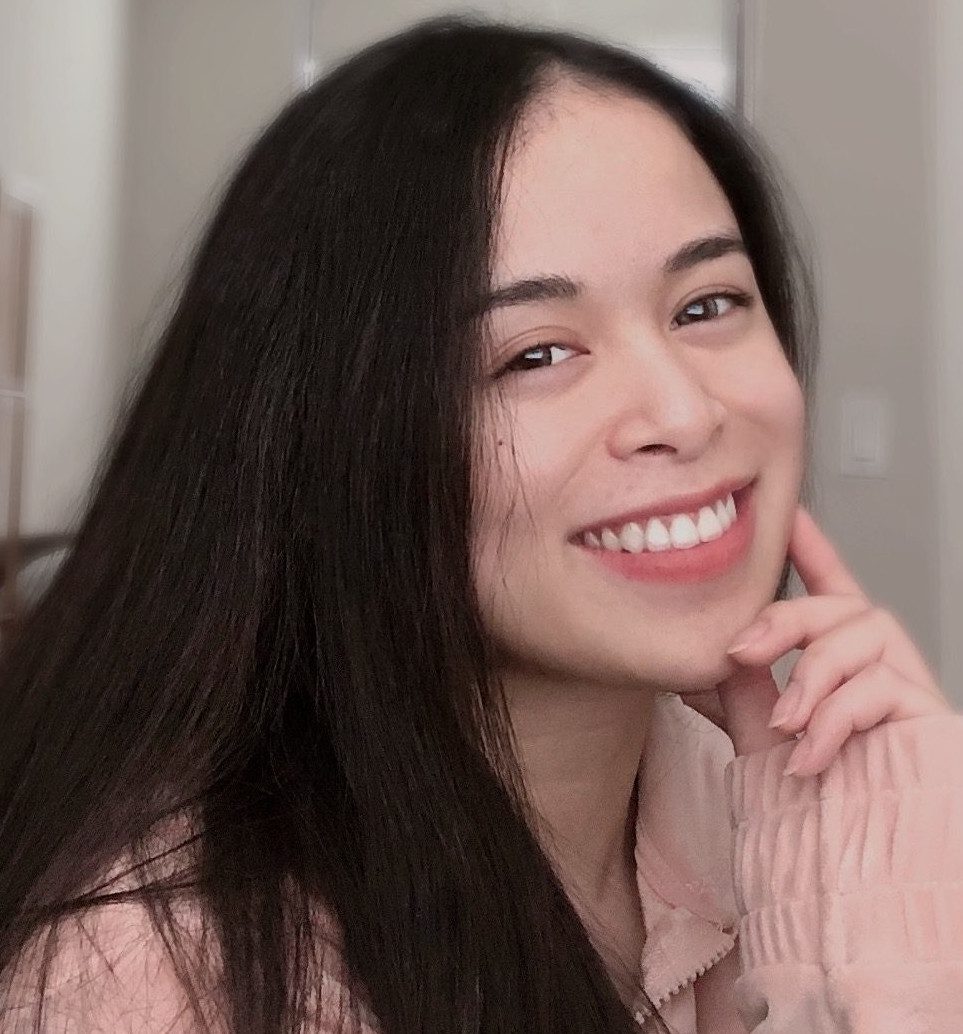
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s run our code:
[1] Display the number of purchases made today [2] Display the average value of all the purchases made today [3] Display the largest purchase made today What would you like to do? 3 5.6
We have selected option 3 from our list. Our code successfully prints out the largest purchase made on a particular day. Let’s try to select option 2:
[1] Display the number of purchases made today [2] Display the average value of all the purchases made today [3] Display the largest purchase made today What would you like to do? 2 3.275
Our code returns the average value of the purchases in our list.
Conclusion
While Python does not have a built-in switch statement, you can create one using a dictionary and the get()
method.
Switch statements are useful if you want to evaluate an expression against multiple potential outcomes.
You can write a faux-switch statement that retrieves values from a dictionary depending on the key you specify. You can also write a faux-switch statement that retrieves a function from a dictionary based on a particular key that you reference.
Now you’re ready to build your own switch statements in the Python programming language like an expert coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.