The Python super() method lets you access methods from a parent class from within a child class. This helps reduce repetition in your code. super() does not accept any arguments.
One core feature of object-oriented programming languages like Python is inheritance. Inheritance is when a new class uses code from another class to create the new class.
When you’re inheriting classes, you may want to gain access to methods from a parent class. That’s where the super() function comes in.
This tutorial will discuss, with examples, the basics of inheritance in Python. Then, we’ll talk about how to use the super() function to inherit values and methods from a parent class.
What is Python Super?
The Python super() method lets you access methods in a parent class. You can think of super() as a way to jump up to view the methods in the class from which another class is inherited.
Here is the syntax for the super() method:
class Food(): def __init__(self, name): self.name = name class Cheese(Food): def __init__(self, brand): super().__init__() self.brand = brand
The super() method does not accept any arguments. You specify the method you want to inherit after the super() method. The above Cheese Python class inherits the values from the Food class __init__() method. We call the super() class method to inherit values from the Food class.
Python inheritance refers to a process where a new class uses code from an existing class to define the structure of the new class.
One way to think about inheritance is to consider how children inherit characteristics from their parents. A child can have the same hair color as their parents, and also inherit their surname from their parents.
In Python, there are two types of classes:
- Child classes (or “sub classes”) inherit methods and variables. Child class are sometimes called sibling classes.
- Parent classes (or “base classes”) are classes whose values can be inherited.
super() Python: Inherit from __init__()
Suppose we are building a bank account system that tracks the credentials of people who hold an account at a bank. To do so, we want to create a class that stores data for checking accounts. We could do so using this code:
class CheckingAccount: def __init__(self, name, balance): self.name = name self.balance = balance def checkBalance(self): print("Balance:", self.balance)
This code creates a blueprint of a checking account.
Now, suppose we want to create a class that stores the blueprint for child bank accounts, which we will call ChildAccount. This class should be based on the CheckingAccount class that we defined earlier. To do so, we can make use of the super() function.
super() allows you to access methods of a parent class inside a child class.
In our example, our parent class is called CheckingAccount, and our child class is called ChildAccount. CheckingAccount creates the blueprint for a bank account, and ChildAccount inherits values from CheckingAccount to create its own class.
Add a New Value to a Class
Now, suppose we want the ChildAccount class to inherit the values stored in our CheckingAccount. But, we also want this account to store a new value called is_child, which should be equal to True by default.
We could make our new ChildAccount class using this code:
class ChildAccount(CheckingAccount): def __init__(self, name, balance, is_child=True): self.is_child = is_child super().__init__(name, balance)
This code creates a blueprint for our new ChildAccount class. We use the super() method to inherit the name and balance values from the CheckingAccount class. We specify CheckingAccount as the parent class in parentheses in the first line of our class.
This shows that super() allows you to inherit all the values from another class. But, in our code, we have also declared a new value called is_child. This value will only be accessible to any ChildAccount because we declared it in the ChildAccount class.
To create an instance of our class for a child account holder, we can use this code:
lynn = ChildAccount("Lynn", 200) print(lynn.name) print(lynn.balance) print(lynn.is_child)
Our code returns:
Lynn 200 True
We have declared an instance of the ChildAccount class assigned to the Python variable lynn. lynn is a class object because we have declared the value of lynn using an object.
Lynn’s account has $200, and the value of is_child is True by default. Then, we have printed out the values of name, balance, is_child associated with Lynn’s account. We print these values to the console using the Python print() method.
Because we inherited name and balance from the CheckingAccount class, we were able to use them in our code. But, Lynn’s account also has a value set for is_child, which is set to True by default for all instances of the ChildAccount.
super() Python: Inherit Methods
super() allows you to inherit classes either using single-level inheritance—inheriting a class from a parent class. Or you can use super() with multilevel inheritance. For this example, we’ll focus on single-level inheritance with super().
In our earlier example, we used super() to inherit values stored in the CheckingAccount class, and make them accessible in the ChildAccount class. But what if we want to inherit a method and modify its response?
Let’s go back to our CheckingAccount class from earlier:
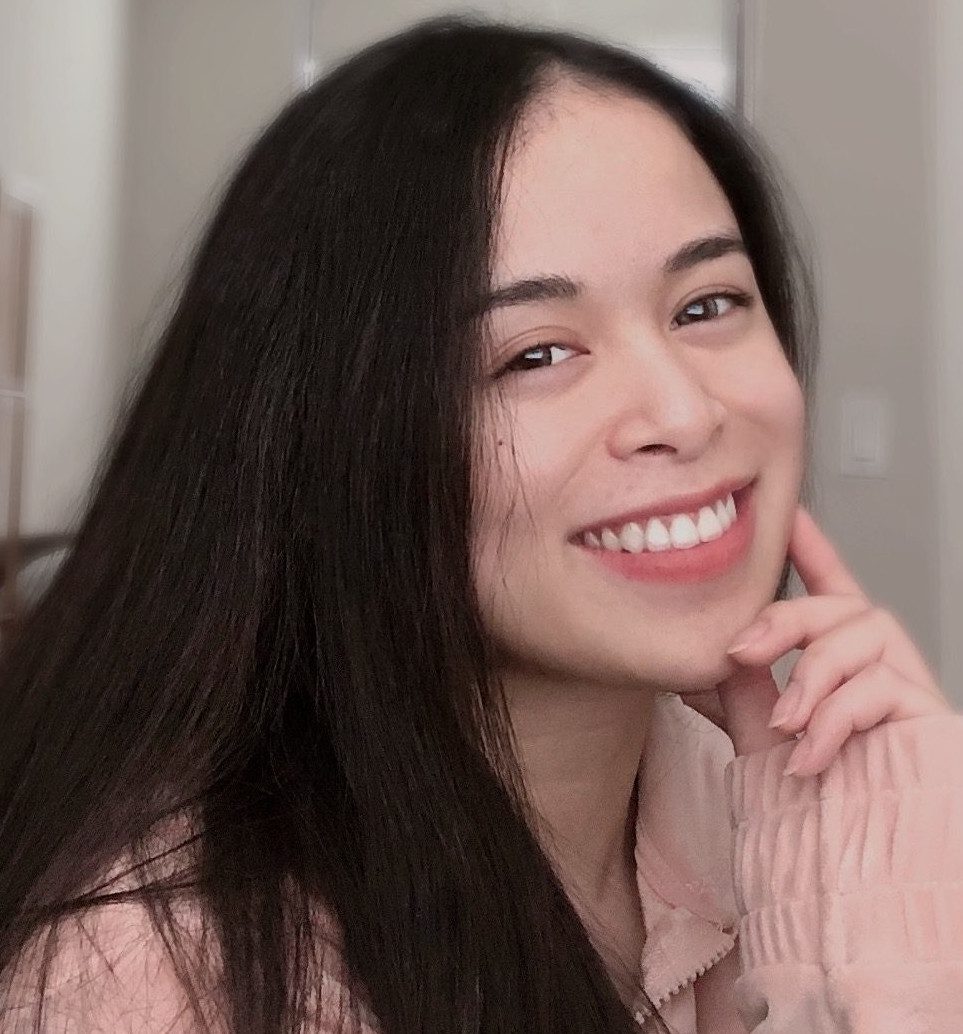
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
class CheckingAccount: def __init__(self, name, balance): self.name = name self.balance = balance def checkBalance(self): print("Balance:", self.balance)
In this class, we have defined the blueprint for values the class should store — name and balance. We defined a method called checkBalance() which allows a user to check their balance.
Now, suppose we want to make checkBalance() accessible to our ChildAccount. We want to also print a message stating “You are a child account holder!” This should appear before the user’s balance is printed to the console.
We could access our inherited methods like so:
class ChildAccount(CheckingAccount): def __init__(self, name, balance, is_child=True): self.is_child = is_child super().__init__(name, balance) def checkBalance(self): print("You are a child account holder!") super().checkBalance()
We have declared a new version of the checkBalance() method in the ChildAccount class. This version first prints “You are a child account holder!” to the console. Then our code it uses super() to execute the checkBalance() method from the class’ parent.
In this case, when super().checkBalance() runs, the checkBalance() function from the CheckingAccount class is executed. This prints out the user’s balance to the console.
Now, let’s try to access our checkBalance() method for Lynn’s account:
lynn = ChildAccount("Lynn", 200) lynn.checkBalance()
Our code returns:
You are a child account holder! Balance: 250
When we call the method lynn.checkBalance(), the code in our ChildAccount.checkBalance() method is run, and then the super object executes the code in the CheckingAccount.checkBalance() method.
The super() function allows us to use parent methods, while still being able to overwrite those methods in our child classes.
Conclusion
The Python super() function lets you inherit methods from a parent class. The super() function helps you write more readable code because you can reuse code from existing functions.
This tutorial discussed, with examples, how to use the super() function to inherit values and methods from a parent class. Now you’re equipped with the knowledge you need to start using the super() function like a Python professional!
For more resources on Python programming, read our complete How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.