Strings are one of the most popular data types in Python. A string is, at a high level, an object consisting of a sequence of characters. A character is a symbol — the English language, for instance, has 26 characters in their alphabet.
Python strings are easy to create and manipulate. You can immediately start learning to do so now. This article takes a look at strings in Python, how they work, and common operations that are used on them.
What are Strings in Python?
You can create a string in Python simply by enclosing characters in quotes — which can be single (‘) or double (“) quotes. Initiate and declare a string by assigning it to a variable:
doubleQuoteString = "I'm a string" singleQuoteString = 'I\'m a string' # needs escaped single quote to treat it as a string value as opposed to end of input.
Remember: if you need to use an apostrophe inside a string that is encapsulated in single quotes, we need to escape it with a “\”. This will treat the quote as part of the string as opposed to the end of the string.
Docstrings and Multi-line Strings
Docstrings are another type of string in Python. They are enclosed in triple quotes (“””) and are treated as a multi-line comment in the first line of a function or class.
def printString(str): ''' takes in a string and prints it to the console ''' print(str)
A docstring describes what a code block’s purpose is. If you happen to want to use the docstring in your logic, you can by assigning the docstring to a variable. If assigned to a variable, the docstring becomes a multi-line string.
multiline = ''' I'm a multi-line string that can be assigned to a variable. The format follows exactly the way YOU input it. ''' print(multiline)
The multi-line string will follow the formatting inside the triple quotes. Without the variable assignment, the string will be treated as a comment and skipped over.
Raw Strings
Python uses a concept called raw strings. This concept treats everything inside the string the way you wrote it — the backslash, in particular, is not used as an escape sequence. Here is a comparison:
notRaw = "I'm a not a raw string, and escape chars DO matter \n\r\t\b\a" rawString = r"I'm a raw string, where escape chars don't matter \n\r\t\b\a" print(notRaw) # I'm not a raw string, and escape chars DO matter. print(rawString) # I'm a raw string, where escape chars don't matter \n\r\t\b\a
The raw string is represented by a regular string prefixed with the letter r:
r”This is a raw string”
Use the raw string when you need the backslash to not indicate a special escaped sequence of some sort.
Unicode Characters in Strings
Unicode is a character specification whose goal is to assign every single character used by any language its own code. In most documentation, unicode values are represented by:
U+<unicode hex value> ⇒ U+2661 ⇒ ♡
U+2661 is an example of one type of unicode character available for us to use. There are tens of thousands of characters to choose from that represent all of the languages of the world.
Writing Unicode in Python 3
To write unicode characters in Python 3, use an escape sequence in a regular string that starts with \u and ends with the code assigned to the character (the number that comes after the “+”).
unicodeString = '\u2661' print(unicodeString) # ♡
You can chain as many unicode characters as you want in a row.
This process of using unicode in a regular string is updated from Python 2. In older versions, you had to have a distinct unicode string that started with u’\u<unicode number here>’
since Python 2 used ASCII values and unicode values.
How to Access Values in Strings
We use square brackets to access values in a string in Python. Strings are zero-based similarly to other programming languages. This means the first character in the string starts on the zeroth index.
hello = "hello world" print(hello[4]) # o
Printing the fourth index in hello will print “o” to the console. If you happen to try to access an index that is not a part of the string, you will see IndexError: string index out of range
. This error means you are trying to access an index that’s larger than or lower than the number of indexes you have.
Note: If you are coming from a programming language like C or Java, you are used to characters having their own data type. This is not the case in Python — individual characters are simply treated as a substring with a length of one. |
Accessing Multiple Characters in a String
Use string slicing to access multiple characters in a string at once. The syntax is:
string_name[first_index? : another_index?]
The last index is non-inclusive. Hello[1:3]
in the previous example will return el
and not ell
.
The interesting thing about slicing in Python is that the index values are not required to slice.
Here are common ways to slice strings in Python:
string_name[:]
Using a colon inside the square brackets will slice the whole string to create a copy.
hello = "hello world" copy = hello[:] + " ⇐ whole string" print(copy) # hello world ⇐ whole string
string_name[first_index:]
You can slice from an index inside the string to the end without knowing the last index value by leaving it empty inside the square brackets.
hello = "hello world" copy = hello[2:] + " ⇐ An index to end of string" print(copy) # llo world ⇐ An index to end of string
string_name[:second_index]
Slicing from the beginning to another index is done by keeping the spot where the first index would go in the brackets empty.
hello = "hello world" copy = hello[:7] + " ⇐ beginning to index inside of string" print(copy) # hello w ⇐ beginning to index inside of string
string_name[first_index:second_index]
Remember that the slice method is non-inclusive if you have two specific indexes that you would like to slice. So if you would like the string from index 1 to index 6, you’ll actually want to format it ashello[1:7]
.
hello = "hello world" copy = hello[1:7] + " ⇐ first index to another index" print(copy) # ello w ⇐ beginning to another index inside of string
Negative numbers can also be used:
hello = "hello world" copy = hello[-7:-2] + " ⇐ negative indexes" print(copy) # o wor ⇐ negative indexes
Using string slicing is great when you would like to manipulate the string, but not mutate the original string. It makes its own copy in memory of the selected indexes so you can update them without changing the original string.
How to Loop Through Strings in Python
In Python, we handle strings in very much the same way we handle arrays. We can loop through strings just as we might do for arrays using the for…in syntax:
#for...in syntax for <index> in <string>: #do logic here #example word = "career karma" for letter in word: print(letter) #prints each individual letter on each iteration
We assign ‘letter’ to the <index> and ‘word’ to <string> to loop through “career karma”. On every iteration, it will print the letter at that iteration to the console.
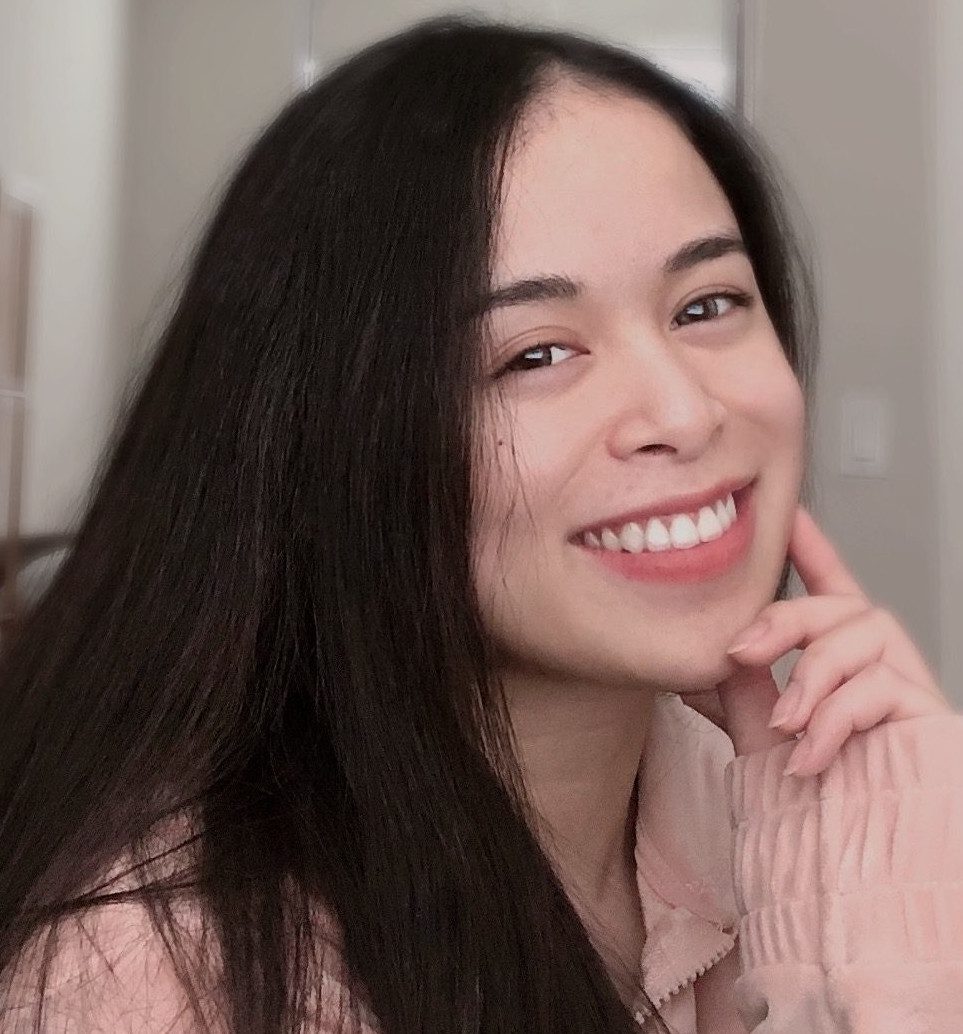
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
How to Find the Length of a String
To find the length of a string, use the len()
method.
lenExample = "The quick brown fox jumps over the lazy dog." print(len(lenExample))
Do not use the len keyword elsewhere in your code. The interpreter will confuse the function with your named variable.
How to Use ‘in’ and ‘not in’
You can use the keywords in and not in with Python strings to check to see if something is contained in the string.
checkString = "Career Karma will help you make a career switch to the tech industry"
checkString is the string we are going to use for the next two examples.
Using in
The in
keyword can be used to test whether or not something is in a string by telling the Python interpreter you want to know if your search term is in the string assigned to the variable.
print("tech" in checkString) # True
Python is a desirable language to learn because it is easily readable. The syntax here is essentially how you might ask that question if you were asking another person:
Q: “Is “tech” in checkString?” (“tech” in checkString)
(scans string)
A: “Yes, yes it is.” (True)
Using not in
The not in
key phrase tests whether something is NOT in a string. This one can be confusing to wrap the mind around, so pay attention. Here, when you ask if something is not in a string, it will return “True” if it’s not in the string, or “False” if it is in the string.
print("tech" not in checkString) # False
Here’s how we might read this syntax if we were talking to another individual:
Q: “Is “tech” not in checkString?” (“tech” not in checkString)
(scans string)
A: “No. The word “tech” is in checkString” (False)
Can We Change the Makeup of a String?
Strings in Python are immutable. The characters that make up the string cannot be mutated or changed in some way after the initial assignment has been made.
delString = "Can we delete this or change this?" delString[2] = "b" print(delString)
Try to run this code in your Python interpreter. This is the error that will result:
Traceback (most recent call last): File "main.py", line no., in <module> delString[2] = "b" TypeError: 'str' object does not support item assignment
The TypeError here tells us that strings in Python do not allow for characters to be reassigned. You need to reassign the whole string to the new string you would like to create if you want to reassign individual characters.
delString = delString[:2] + "b" + delString[3:] print(delString)
The code here is the correct way to do what we intended to do with the delString[2] = “b”
line of code. An entire reassignment is needed to change a string object.
This also means deleting single characters in a string is not possible. You can, however, delete an entire string with the del
keyword.
del delString print(delString) #this will return an error or the linter will catch as being undefined
Popular String Methods
There are several methods associated with Python strings. For the most up-to-date list, check out the Python docs.
Here are some of the most popular methods. testString
is a sample string that will be used to demonstrate most of the methods.
testString = "this is a test string."
capitalize()
The capitalize method will capitalize the first letter of the string:
capitalized = testString.capitalize() print(capitalized) # This is a test string
find()
This built-in method will return the index of where the passed argument begins. If it can’t be found, it returns -1.
found = testString.find("test") notfound = testString.find("not") print(found) # 10 print(notfound) # -1
join()
The join method will take the iterable object passed into the method (could be a string, a set, a tuple, or a list), loop through it, and add the string separator that the method is called on. Here’s an example:
separator = ", " # this is our separator - this string is the one that the method gets called on numbers = "123" # this is our iterable. In this instance, it is a string joinedByComma = separator.join(numbers) print(joinedByComma) # 1, 2, 3
Try playing with the join method to see what you can do with other iterables like lists, sets, or tuples!
lower() and upper()
The lower and upper built-in functions deal with the case of the string. Lower will create a copy of the string in all lowercase; upper will create a copy of the string in all uppercase:
uppercase = testString.upper() print(uppercase) # THIS IS A TEST STRING lowercase = testString.lower() print(lowercase) # this is a test string
These methods are just a few of the methods that you can use when it comes to manipulating strings. After learning these, check out other Python string methods like split()
, replace()
, title()
, and swapcase()
.
Conclusion
In this article, we took a look at what Python strings are, how they are used, and some of the built-in methods that are available for us to use. After you have mastered this guide, take a look at our article on string formatting in Python to take your learning even further!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.