Python has many methods used exclusively for strings. Python string methods include upper()
, lower()
, capitalize()
, title()
, and more. These string methods are useful for manipulating, editing, and working with strings.
Strings are one of the core data types used in programming, and they allow computers to work with text. For example, a string may be used to store a user’s name or their email address in a Python program.
Python includes several string methods that can be used to modify and manipulate strings. These functions are built into Python and so are ready for us to use without importing any libraries.
In this tutorial, we are going to break down a few of the most common string methods you are likely to encounter in Python and explore examples of how they may be used.
String Refresher
Strings allow you to store text when you’re programming and are declared within single quotes ‘’
or double quotes “”
in Python. While you can use either single quotes or double quotes, when you decide which one to use you should keep it consistent throughout your program. Here’s an example of declaring a string:
"This is an example string in Python."
We can print out a string by using the print()
function:
print("This is an example string!")
Our program returns: This is an example string!
When you’re working with a string, you may want to merge it with another string to create a new one. We call this string concatenation in programming. In order to merge two strings, we use the +
operator. Here’s an example of a print()
function that will merge two strings together:
print("Example" + "String")
Our program returns: ExampleString
. Note that when we merge our strings, there are no spaces included. If we want to include a space, we should add a whitespace character within our strings.
Now that we’ve explored the basics of strings, we can start to explore Python string methods.
Upper and Lower Case Strings
Two of the most common string functions in Python are those which allow you to change the cases of your strings to upper or lower case. The functions upper()
and lower()
will return a string with all the original letters in the string converted into either upper or lower case.
When you use the upper()
or lower()
functions, the returned string will be a new string, and your existing string will remain the same.
Here’s an example of the upper()
class being used to convert a string to uppercase:
string_example = "String Example" print(string_example.upper())
Our program returns:
"STRING EXAMPLE".
If we wanted our string to appear in lowercase, we could use the lower()
function like this:
print(string_example.lower())
Our code returns:
"string example".
In addition, you can use the capitalize()
method to capitalize the first character of a string, and the title()
method to capitalize the first letter of every word in a string.
These functions are useful if you want to compare strings and want to keep the cases of those strings consistent. This is important because comparisons in Python are case sensitive. For example, you may want to convert a user’s email address to lower case so that you can compare it with the one you have on file.
String Length
When you’re working with a string, you may want to find out the length of that string. The len()
Python string method can be used to return the number of characters in a string.
This function is useful if you want to set minimum or maximum lengths for a form field, for example. If you want a user’s email address to be no more than 50 characters, you could use len()
to find out the length of the email and present an error if it is more than 50 characters long.
Here’s an example of a program that checks the length of a string:
string_length = "What is the length of this string?" print(len(string_length))
Our program returns: 34. In our code, we declared the variable string_length
which stored the string What is the length of this string?
Then, on the next line, we used the len(string_length)
method to find out the length of our string, and passed it through the print() method so that we could see the length in our console.
Remember that the length function will count every character in a string, which includes letters, numbers, and whitespace characters.
Boolean String Methods
There are a number of Python string methods that return a boolean value. These methods are useful if we want to learn more about the characteristics of a string. For example, if we want to check if a string is uppercase, or if it contains only numbers, we could use a boolean string method that will return true if all characters are uppercase.
Here is a list of the Python string methods that return True if their condition is met:
str.isupper()
: String’s alphabetic characters are all uppercasestr.islower()
: String’s alphabetic characters are all lower casestr.istitle()
: String is in title casestr.isspace()
: String only consists of whitespace charactersstr.isnumeric()
: String only contains numeric charactersstr.isalnum()
: String contains only alphanumeric characters (no symbols)str.isalpha()
: String contains only alphabetic characters (no numbers or symbols)
Let’s explore a few of these boolean string methods. Here’s an example of the isupper()
function being used to check if a string contains only uppercase characters:
full_name = "JOHN APPLESEED" print(full_name.isupper())
Our program returns: True. The full_name
variable contains a value with text that is entirely in uppercase, and so our isupper()
function evaluates to True and returns the True value.
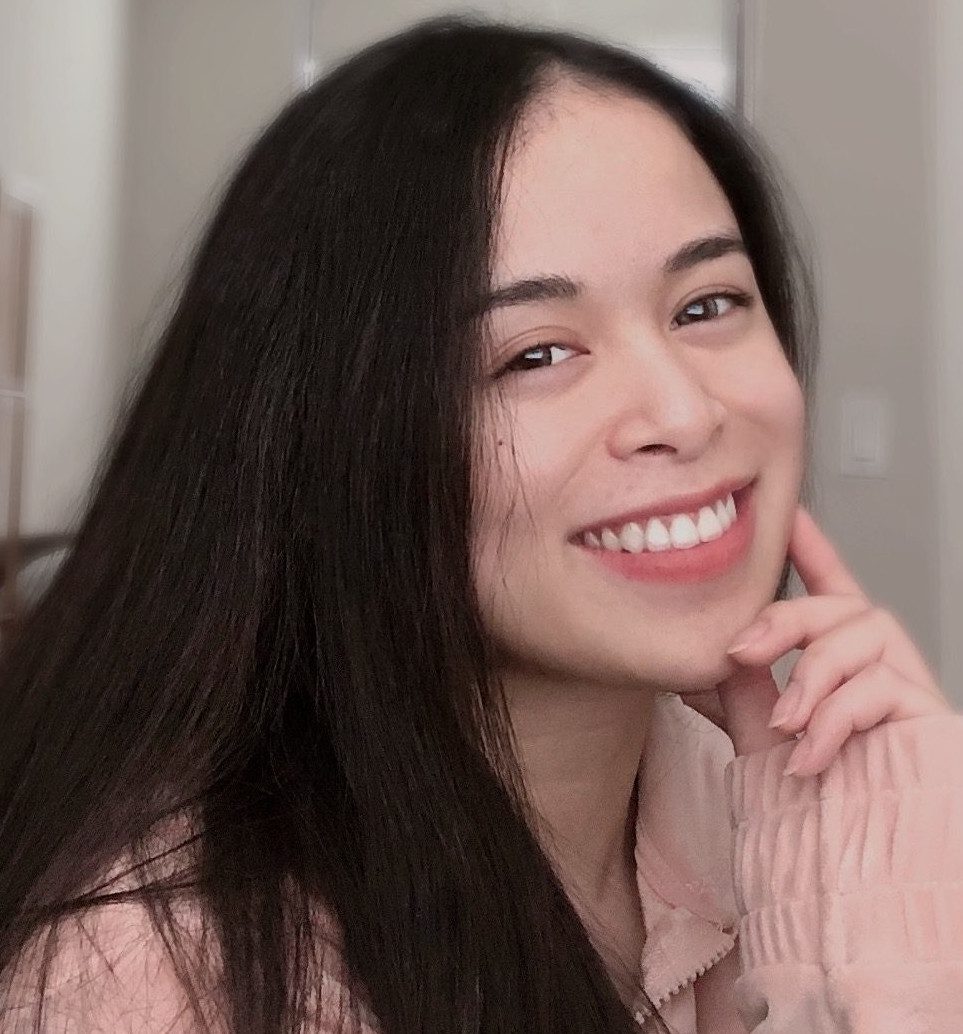
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Similarly, we can use the istitle()
function to check if a string is in title case:
sentence = "This is a sentence." print(sentence.istitle())
Our code returns: False. Because our string is not in title case—each character does not begin with a capital letter—our istitle()
method evaluates to False.
Boolean string methods can be useful when receiving user input that you want to check, or if you have two exact values that you want to compare.
Starts With and Ends With
Let’s say you have a string and you want to check if it starts or ends with a particular value. For example, you may have a user’s name and want to check if it starts with F
. We can use the startswith()
and endswith()
functions to check our strings.
The startswith()
method returns True if a string starts with a specific value, and the endswith()
method returns True if a string ends with a specific value. Otherwise, the string methods return false.
Both methods take three parameters. The first is the value that you are looking for. Optionally, you can specify the starting index within the string where your search should begin with the second parameter, and you can also state the ending index where your search should end with the third.
Here’s an example of the startswith()
function in action:
full_name = "Bill Jefferson" print(full_name.startswith("Bill"))
Our program returns: True.
If we wanted to check if Bill
appeared in our string starting after the fourth letter in our string—the item with the index value 3
, because indexes start at 0
—we could use the following code:
full_name = "Bill Jefferson" print(full_name.startswith("Bill", 3))
Our code returns False
, because Bill
does not appear in the string after the index value 3
.
Split and Join Strings
In addition, you can use the join()
and split()
methods to manipulate strings in Python.
The join()
method takes all the items in an iterable string and joins them to another string. Here’s an example of the join()
Python string method in action:
string = "String" "X".join(string)
Our code returns the following: SXtXrXiXnXg
. What has happened is the join()
string has gone through each letter in the string
variable and added an X
after each letter.
The join()
method can be useful if you want to join a certain value together with a string. The method is also often used to combine a list of strings together. Here’s an example of join()
being used to combine a list of strings into one:
print(",".join(["Lucy", "Emily", "David"])
Our code returns the following: Lucy
,Emily
,David
. Now our list of names is in one string, rather than in an array.
We can also use the split()
string method to split up a string and return a list of strings. Here’s an example of the split()
method being used to divide a sentence into a list of strings:
example_sentence = "This is a sentence." print(example_sentence.split())
Our code returns the following:
["This", "is", "a", "sentence."]
As you can see, our original string has been divided into four items and a new list of those items has been created.
The split()
method returns a list of strings separated by whitespace characters if no other parameter is specified, but if you want to split a string by another character, you can. Here’s an example of a program that divides a string based on the commas in that string:
names = "Lucy,Emily,David" print(names.split(","))
Our code returns:
["Lucy", "Emily", "David"]
As you can see, our program has separated our strings by the comma values in our initial string and created an array with the new separate values.
View the Repl.it from this tutorial:
Conclusion
Strings are a useful data type that allow coders to store text values in their programs. In this tutorial, we explored some of the most common built-in Python string methods that you can use to manipulate strings.
We discussed the upper()
and lower()
methods and how they can help you work with cases in Python, the len()
method and how it can help you get the length of a string, and we explored the boolean string methods available in Python.
We also discussed how to use join()
and split()
, and how to check if a string starts with or ends with a substring.
It’s worth noting that there are a number of other string methods out there such as ljust()
, rjust()
, and zfill()
, and this article only scratches the surface. Now you’re ready to start working with strings in Python like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.