Stacks are an important data structure with a wide range of uses.
In programming, stacks allow you to store data in a last-in, first-out (LIFO) order. This means that the last item stored in a stack is the first one that will be processed.
But how do you create a stack in Python? That’s the question we are going to answer in this guide. By the end of reading this guide, you’ll be an expert at creating and working with stacks in Python.
Python Stacks
Stacks store data in a last-in, first-out (LIFO) order.
To help you understand how this order works, consider a stack of plates. When you have a stack of plates to clean, the first plate you’ll move is the one on the top. Then, as you move plates, you’ll be able to access the ones lower down in the stack.
Stacks are the opposite of queues in Python. Queues remove the least recently added item (because they use the first-in, first-out structure), whereas stacks remove the most recently added item (because they use the last-in, first-out structure).
Stacks typically support two operations: push and pop. Pushing allows you to add an item to the top of a stack, and popping allows you to remove the item at the top of the stack.
In Python, there are two main approaches you can use to create a stack: using built-in lists, and using the collections.deque()
class. Let’s break down how each of these approaches work.
Python Built-In Lists
The built-in list data type allows you to create a stack in Python.
Because Python lists are implemented as arrays, you can add and remove items to them easily. In addition, the order in which you insert values in a list will be preserved, which means that you can easily remove the first and last items in a list.
Suppose we want to create a stack that stores a list of homework assignments in a class. The teacher wants to grade these assignments in the order they appear in the pile (so, the assignment handed in first will be at the bottom of the stack, and the assignment handed in last will be at the top of the stack).
Add Items to Stack
To add items to a stack, we can use the append()
method. We could create our homework assignment stack using the following code:
assignments = [] assignments.append("Hannah") assignments.append("Benny") assignments.append("Gordon") print(assignments)
Our code returns:
['Hannah','Benny','Gordon']
In our code, we first declare a list called assignments
. Then, we use the append()
method to add three names to our list of assignments that have been handed in. The names we add are, in order: Hannah, Benny, Gordon. Because Gordon handed in his assignment last, it appears in the final position in our list.
Remove Items from Stack
Suppose that we have graded Gordon’s assignment, and we want to find out which one is next to grade. This involves removing the item at the top of our stack.
To remove an item from our stack, we can use the pop()
method. Here’s the code we could use to remove the top item on our stack:
assignments = [] assignments.append("Hannah") assignments.append("Benny") assignments.append("Gordon") assignments.pop() print(assignments)
Our code returns:
['Hannah','Benny']
Gordon’s name has been removed from the stack using pop()
, and so our stack now only contains two names: Hannah and Benny.
collections.deque Class
The deque class in the collections library allows you to create a double-ended queue.
The deque object is implemented as a doubly-linked list, which means that it has strong and consistent performance when inserting and deleting elements. In addition, because the collections library is part of the Python Standard Library, you can import it into your code without having to download an external library.
To work with the collections.deque
class, we must first import it into our code using an import statement:
from collections import deque
Let’s return to our homework example from earlier to illustrate how the collections.deque class works.
Add Items to a Deque Stack
To add items to a deque stack, we can use the append()
method. Suppose we want to create a queue with our homework assignments using the deque class. We could do so using this code:
from collections import deque assignments = deque() assignments.append("Hannah") assignments.append("Benny") assignments.append("Gordon") print(assignments)
Our code returns:
deque(['Hannah','Benny','Gordon'])
Let’s break down our code. First, we import the deque class from the collections library. Then, we create a deque using deque()
and assign it to the variable assignments
.
Next, we add three names to our assignments deque: Hannah, Benny, and Gordon. Finally, we print out the contents of our assignments queue to the console.
You can see that, in this example, our data is stored as a deque instead of a stack (denoted by the fact our result is enclosed in deque()
). This is because we are using the deque structure, even though our data still acts like a stack.
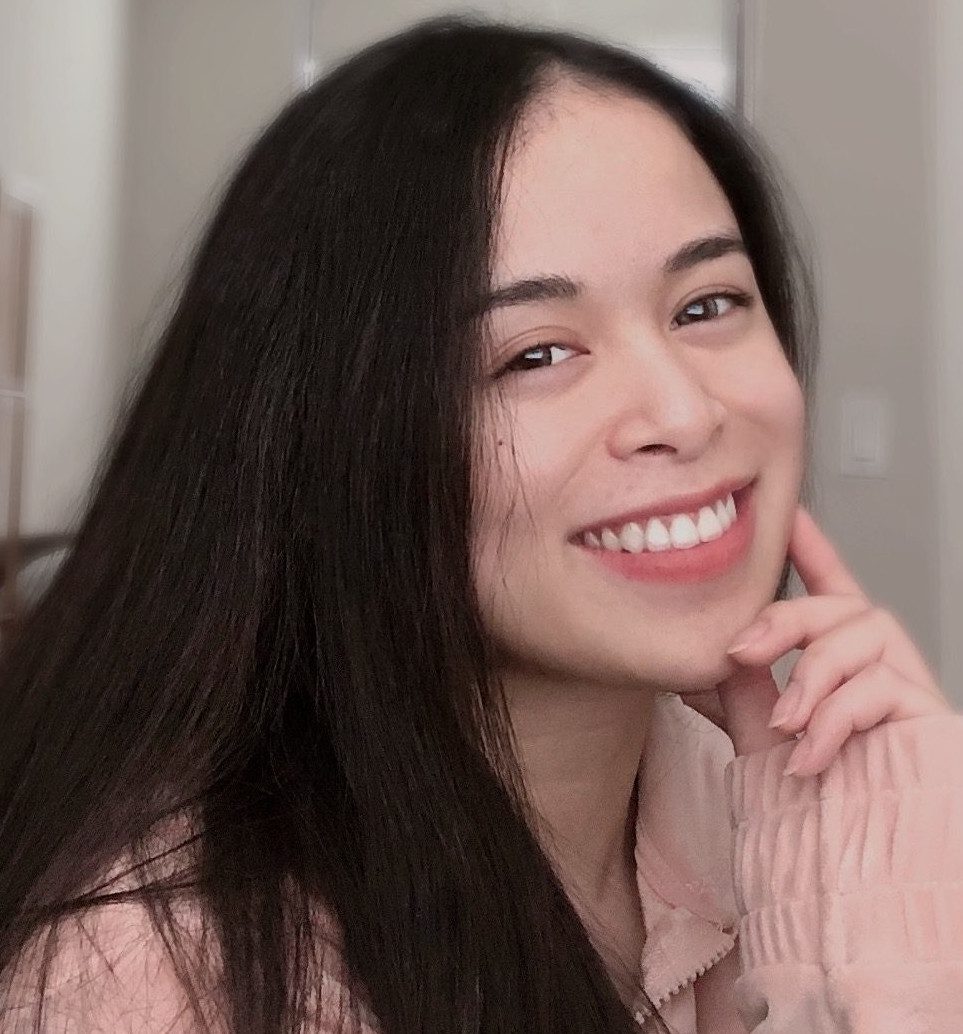
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Remove Items from a Deque Stack
To remove items from a deque stack, you can use the pop()
method.
Suppose we have just graded Gordon and Benny’s assignments. To remove them from our stack, we could use the following code:
from collections import deque assignments = deque() assignments.append("Hannah") assignments.append("Benny") assignments.append("Gordon") assignments.pop() assignments.pop() print(assignments)
Our code returns:
deque(['Hannah'])
In our code, we first create a deque stack with three values. Then, we execute the pop()
statement twice. Each time the pop()
statement runs, the item at the top of our stack is removed. This means that the values Gordon and then Benny are removed from our stack, leaving Hannah as the only item left in our stack.
To learn more about the Python deque class, read our tutorial on Python queues and deques.
Conclusion
Stacks allow you to store data in a last-in, first-out order. There are a number of ways to implement a stack in Python, but the two most practical approaches are to use the Python built-in list structure, or to use the collections.deque()
class.
This tutorial discussed, with reference to examples, how to create a stack in Python using lists and collections.deque()
. Now you’re ready to start creating your own stacks like a professional Python developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.