Unless you’re a math genius, you don’t have all square roots memorized. And even if you did, someone else looking at your code may not know that you are. That means they might have to re-check that you wrote the correct square roots — that’s just re-doing work.
If you have used Python’s square root function, it is clear that a square root is being calculated. Another person looking at your code would know that it is accurate. As an added bonus, no one has to open their calculator!
What is Python sqrt()?
Whether you are using the Pythagorean theorem or working on a quadratic equation, Python’s square root function — sqrt() — can help you solve your problems. As you may have guessed, sqrt()
will return the square of the number you pass in as a parameter.
The sqrt()
method can be useful because it is fast and accurate. This brief tutorial covers what you can pass as a parameter to sqrt()
, ways to get around invalid parameters, and an example to help you understand. You can get the square root of a number by raising it to a power of 0.5 using Python’s exponent operator (**) or the pow()
function.
81 ** 0.5 //The result will be 9.0
When you work with multiple numbers requiring square roots, you will find that using the sqrt()
function is more elegant than using multiple exponent operators with “0.5”. Also, it’s more clear. It can be easy to forget or miss the extra asterisk (‘*’), which will completely change the statement into a multiplication statement, giving you a completely different result.
Python Square Root Function Syntax
The general syntax used for calling the sqrt()
function is:
math.sqrt(x);
In the code snippet above, “x” is the number whose square root you want to calculate. The number you pass in as a parameter to the square root function can be greater than or equal to 0. Note that you can only pass in one number.
But what does the “math” part in the syntax above refer to? The math module is a Python library that holds many useful math-related functions, one of them being the sqrt()
function. To use sqrt()
, you will have to import the math module, as that’s where the code to carry out the function is stored. By prefixing “math” to sqrt()
, the compiler knows you are using a function, sqrt()
, belonging to the “math” library.
The way to import the math module is to write the keyword “import” along with the name of the module — “math” in this case. Your import statement is a simple line that you write before the code containing a sqrt()
function:
import math
The result of the square root function is a floating point number (float). For example, the result of using sqrt()
on 81 would be 9.0, which is a floating point number.
math.sqrt(81) #9.0
Include the math import statement at the top of any file or terminal/console session that contains code which uses sqrt()
.
How to Use Python’s sqrt() Method
You can pass in positive float or int (integer) type positive numbers. We saw an int, 81, as a parameter in the previous example. But we can also pass in floats, 70.5, for example:
math.sqrt(79.5) #8.916277250063503
The result of that calculation is 8.916277250063503. As you can see, the result is pretty precise. Now you can see why it makes sense that the output will always be a double, even if the square root of a number is as simple as “9”.
You can also pass in a variable that represents a number:
yourValue= 90 math.sqrt(yourValue) # 9.486832980505138
And you can save the result in a variable as well:
sqrtOfValue= math.sqrt(yourValue)
Saving this into a variable will make it easier to print to the screen:
print("The square root is " , sqrtOfValue) #The square root is: 9.486832980505138
Working with Negative Numbers with abs()
The square root of any number cannot be negative. This is because a square is the product of the number times itself, and if you multiply two negative numbers the negatives cancel out and the result will always be positive. If you attempt to pass a negative number to sqrt()
, you will get an error message and your calculation will not go through.
The abs()
function returns the absolute value of a given number. The absolute value of -9 would be 9. Likewise, the absolute value of 9 is 9. Since sqrt()
is designed to work with positive numbers, a negative number would throw a ValueError exception.
Suppose you pass in variables to sqrt()
and can’t know if they are all positives without checking through long lines of code to find the variable values. At the same time, you also do not want a ValueError exception thrown at you. Even if you do look, some other programmer can come in and unintentionally add a negative variable, then your code will throw an error. To prevent this madness, you can use abs()
:
negativeValue= -9 math.sqrt(abs(negativeValue)) #3.0
Or , alternatively :
math.sqrt(abs(-81)) # 9.0
The abs()
function will take in your value and translate it to an absolute value (81 in this case). Then the non-negative, absolute value will be passed into the sqrt()
function, which is what we want, so we do not get pesky errors!
List Comprehension and sqrt()
What if you have multiple numbers whose square roots you would like to obtain? You can calculate the square root for all in one line using an in-line for loop called a list comprehension.
First make a list with the values whose square roots you want to obtain.
numbers = [ 21, 30, 9.75, 55, 77]
Secondly, let’s iterate through the list with a for-loop expression in order to get the square root for each value. The syntax for an in-line for-loop expression is for number in numbers, where “number” is each member of the list which we named “numbers”. We will save the results in a list we will call “squaredNumbers”.
squaredNumbers = [ math.sqrt(number) for number in numbers]
Use a print()
statement to see the results of squaring the numbers list.
print("The square roots are: " , squaredNumbers) # The square roots are: [4.58257569495584, 5.477225575051661, 3.122498999199199, # 7.416198487095663, 8.774964387392123]
for-Statements and sqrt()
You can also use a typical for-loop. Although using a typical for-loop means you have to write more lines of code than the above example, for-loops can be easier to read for some people.
First, declare the list you want to save the calculated values in.
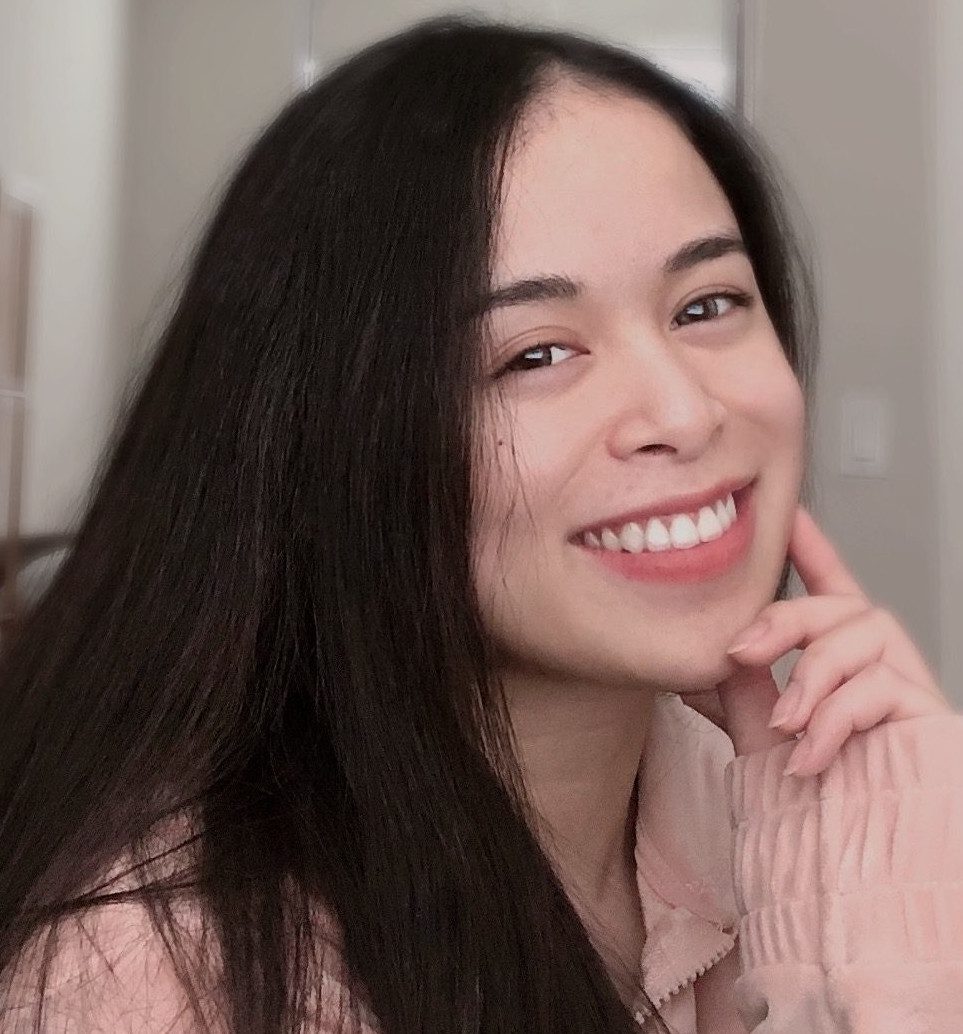
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
squaredNumbers =[ ]
We will use the same values list (“numbers”) as in the previous example, and iterate through each of its elements, which we named “number”.
for number in numbers: squaredNumbers.append(math.sqrt(number))
Now if you print this new list of squared numbers, you get the same output as the earlier example.
print("The square roots are: " , squaredNumbers) # The square roots are: [4.58257569495584, 5.477225575051661, 3.122498999199199, 7.416198487095663, 8.774964387392123]
Example with sqrt(): Diagonal Distances
There are many usages for sqrt()
. One example is you can use it to find the diagonal distance between two points that cross at right angles, such as street corners or points on a field or blueprint schematic.
This is because the diagonal distance between two points that cross at a right angle would be equivalent to the hypotenuse of a triangle, and for that you could use the pythagorean theorem, (a2 + b2) = c2 , which happens to use square roots. This formula is very handy because in city streets, home blueprints, and fields, it can be easy to get length and width measurements but not for the diagonals between them.
You would have to use sqrt()
on the hypotenuse, c2 , to have the length. Another way we could rewrite the pythagorean theorem is c= √a2 + b2. Let’s imagine we ran a circuit at our local park in the form of a triangle.
We ran lengthwise and widthwise, then cut across back to our starting point. To get an accurate count of how many feet you ran, you could calculate the feet of the diagonal way you cut across by using the length and width (whose length in feet you could save as the variables “a” and “b”) of the park:
import math a=27 b=39 math.sqrt(a**2+ b**2)
The result would be 47.43416490252569. So when you add this to the other two lengths, you know and there you have it. The total number of feet you ran in your right-triangle shaped path at the park.
What Else Can You Do with Sqrt()?
Now that you know the basics, the possibilities are endless. For instance:
- Use it in a formula to determine prime numbers.
- Perform any number of operations requiring a precise square root.
- Use it to calculate distances.
In this article, you’ve learned how to use sqrt()
with positive and negative numbers, lists, and how to rework the pythagorean theorem so that four math calculations are done with sqrt()
.
Do you need to work with whole numbers instead of floating point numbers? math.isqrt()
outputs the square as an integer and rounds down to the nearest whole. You can even use sqrt()
with libraries other than the “math” library such as numPy, a python library used for working with arrays.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.